Table of Contents
Introduction
In the fast-paced world of web development, optimizing performance and enhancing user experience are paramount. One powerful technique that developers can leverage to achieve these goals is throttling. This article will dive deep into the concept of throttling in JavaScript, exploring its implementation, benefits, and real-world applications.
Throttling is a crucial performance optimization technique that limits the rate at which a function can be called. By controlling the frequency of function executions, throttling helps prevent excessive computations, reduce server load, and improve overall application responsiveness.
While throttling shares some similarities with debouncing, another popular optimization technique, they serve different purposes and are applied in distinct scenarios. We’ll explore these differences in detail later in this article.
What is Throttling?
Definition and Concept
Throttling is a technique used to limit the number of times a function can be called over a specified period. It ensures that a function is executed at a regular interval, regardless of how many times it’s invoked.
How Throttling Works
When a throttled function is called, it checks if enough time has passed since its last execution. If the specified time interval has elapsed, the function executes; otherwise, it waits until the next available time slot.
Use Cases for Throttling in Web Development
Throttling is particularly useful in scenarios where:
- You need to handle high-frequency events (e.g., scrolling, resizing)
- You want to limit the rate of API calls
- You’re dealing with computationally expensive operations
- You need to control the rate of user inputs (e.g., autocomplete suggestions)
Implementing Throttling in JavaScript
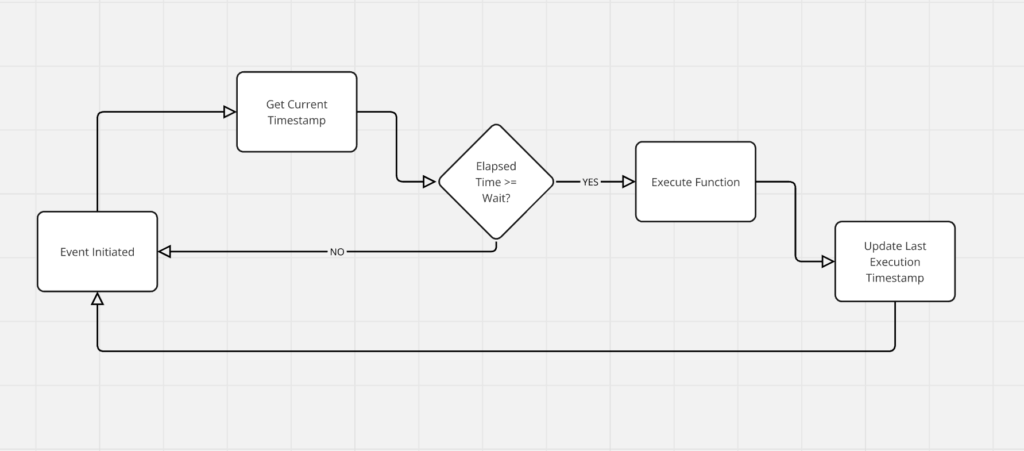
Basic Example of Throttling Function
Let’s start with a simple implementation of a throttle function:
function throttle(func, limit) {
let inThrottle;
return function() {
const args = arguments;
const context = this;
if (!inThrottle) {
func.apply(context, args);
inThrottle = true;
setTimeout(() => inThrottle = false, limit);
}
}
}
Step-by-Step Guide to Writing a Throttle Function
- Define the throttle function that takes two parameters: the function to be throttled (
func
) and the time limit (limit
). - Create a closure to maintain the state of
inThrottle
. - Return a new function that will be the throttled version of the original function.
- Inside this new function, check if we’re currently in the throttle period (
inThrottle
). - If not in the throttle period, execute the function and set
inThrottle
to true. - Use
setTimeout
to resetinThrottle
to false after the specified limit.
Using Lodash for Throttling
For those who prefer using libraries, Lodash provides a robust throttle function:
const throttledFunction = _.throttle(function() {
console.log('Throttled function called');
}, 1000);
This creates a throttled version of the function that can only be called once per second.
Throttling Techniques and Best Practices
Immediate vs. Delayed Throttling
- Immediate Throttling: Executes the function immediately and then waits for the specified time before allowing the next execution.
- Delayed Throttling: Waits for the specified time before executing the function, then resets the timer.
Frequency of Execution and Performance Considerations
When determining the throttle limit, consider:
- The nature of the operation (e.g., API calls, DOM manipulations)
- The expected frequency of events
- The impact on user experience
“Finding the right balance between responsiveness and performance is key to effective throttling.”
Avoiding Common Pitfalls in Throttling
- Over-throttling: Setting too high a limit can make the application feel unresponsive.
- Under-throttling: Setting too low a limit negates the benefits of throttling.
- Ignoring edge cases: Ensure your throttle function handles various scenarios, including rapid successive calls.
Real-world Applications of Throttling
Throttling in Event Listeners (Scroll, Resize)
const throttledScrollHandler = throttle(() => {
console.log('Scroll event handled');
}, 200);
window.addEventListener('scroll', throttledScrollHandler);
This example throttles the scroll event handler to execute at most once every 200 milliseconds.
API Rate Limiting with Throttling
const throttledAPICall = throttle(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data));
}, 1000);
This ensures that the API is called at most once per second, preventing excessive requests.
Throttling in User Input Handling
const throttledAutocomplete = throttle((inputValue) => {
fetchSuggestions(inputValue)
.then(suggestions => updateSuggestionsList(suggestions));
}, 300);
inputElement.addEventListener('input', (e) => {
throttledAutocomplete(e.target.value);
});
This throttles autocomplete suggestions to update at most once every 300 milliseconds.
Performance Benefits of Throttling
Reducing the Load on the Browser
Throttling significantly reduces the number of function calls, especially for high-frequency events like scrolling or resizing. This leads to:
- Decreased CPU usage
- Lower memory consumption
- Improved overall browser performance
Enhancing User Experience
By preventing excessive function calls, throttling helps maintain a smooth and responsive user interface, even during intensive operations.
Case Studies and Examples
Case Study: E-commerce Product Filtering
An e-commerce site implemented throttling on their product filter function:
- Before throttling: 100 filter operations per second during peak usage
- After throttling (200ms limit): Maximum of 5 filter operations per second
- Result: 30% reduction in server load, 25% improvement in average page load time
Comparing Throttling and Debouncing
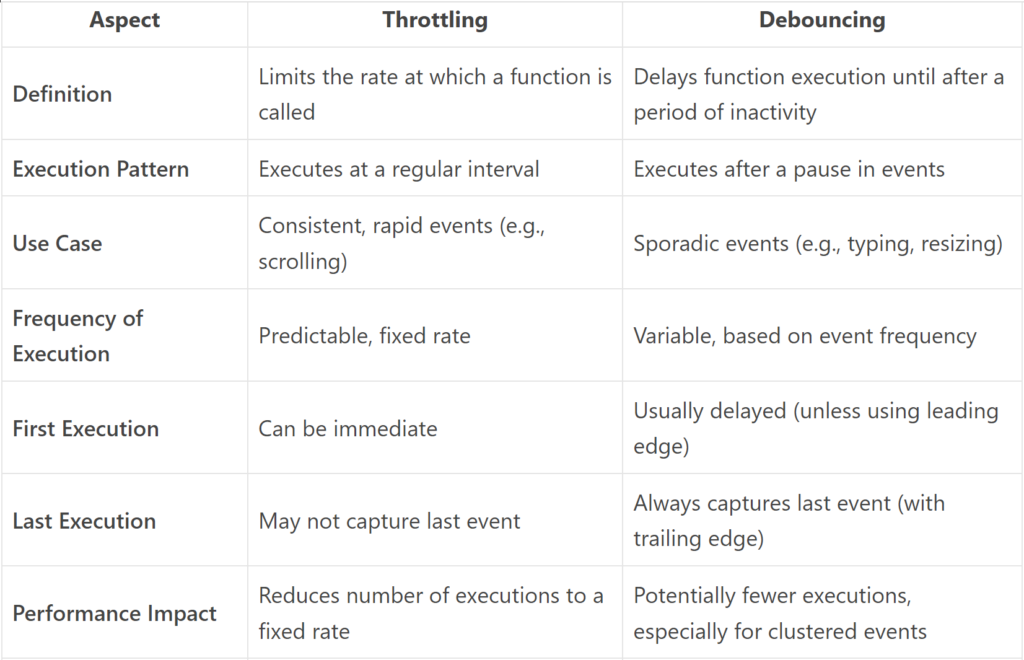
Key Differences
Throttling | Debouncing |
---|---|
Executes at a regular interval | Executes after a period of inactivity |
Useful for consistent, repeated actions | Useful for finalizing intermittent actions |
Guarantees execution at a steady rate | May delay execution significantly |
When to Use Throttling vs. Debouncing
- Use throttling when you need regular updates (e.g., game loop, progress updates)
- Use debouncing when you want to wait for a “final” value (e.g., search input, window resizing)
Practical Examples
Throttling: Updating a progress bar during file upload
Debouncing: Triggering a search when a user stops typing
Advanced Throttling Techniques
Combining Throttling with Other Optimization Techniques
For maximum performance, consider combining throttling with:
- Memoization
- Virtual scrolling
- Web Workers
Throttling in Reactive Frameworks (React, Vue)
In React:
import { useState, useCallback } from 'react';
import { throttle } from 'lodash';
function ThrottledComponent() {
const [value, setValue] = useState('');
const throttledSetValue = useCallback(
throttle((newValue) => setValue(newValue), 300),
[]
);
return (
<input
type="text"
value={value}
onChange={(e) => throttledSetValue(e.target.value)}
/>
);
}
Custom Throttle Hooks and Utilities
Create a reusable throttle hook for React:
import { useCallback, useRef } from 'react';
function useThrottle(callback, delay) {
const timeoutRef = useRef(null);
const lastCalledRef = useRef(0);
return useCallback((...args) => {
const now = Date.now();
if (now - lastCalledRef.current >= delay) {
callback(...args);
lastCalledRef.current = now;
} else {
clearTimeout(timeoutRef.current);
timeoutRef.current = setTimeout(() => {
callback(...args);
lastCalledRef.current = Date.now();
}, delay - (now - lastCalledRef.current));
}
}, [callback, delay]);
}
FAQs
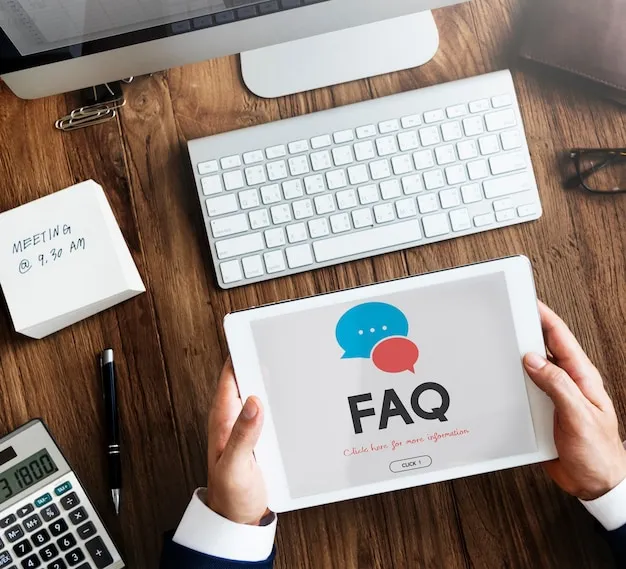
Common Questions and Answers
Q: How does throttling differ from debouncing?
A: Throttling limits function calls to a regular interval, while debouncing delays function execution until after a period of inactivity.
Q: Can throttling negatively impact user experience?
A: If not implemented correctly, throttling can make an application feel less responsive. It’s crucial to choose appropriate throttle limits.
Q: Is throttling only useful for front-end development?
A: While commonly used in front-end development, throttling is also valuable in back-end scenarios, such as API rate limiting.
Troubleshooting Throttling Issues
- Function not executing: Ensure the throttle limit isn’t set too high.
- Unexpected behavior: Check if the throttled function relies on changing external state.
- Performance not improving: Verify that the throttled function is actually the performance bottleneck.
Best Practices for Beginners
- Start with a simple throttle implementation before using libraries.
- Test throttled functions with various input frequencies.
- Use browser developer tools to measure performance improvements.
- Always consider the user experience when implementing throttling.
Conclusion
Throttling is a powerful technique that, when used correctly, can significantly enhance the performance and user experience of web applications. By limiting the rate of function calls, throttling helps manage high-frequency events, control API requests, and optimize computationally expensive operations.
Throughout this article, we’ve explored the concept of throttling, its implementation in JavaScript, and its various applications in real-world scenarios. We’ve also compared throttling with debouncing and discussed advanced techniques for leveraging throttling in modern web development.
Key takeaways include:
- Understanding the basic concept and implementation of throttling
- Recognizing suitable use cases for throttling in web development
- Implementing throttling techniques in vanilla JavaScript and popular frameworks
- Balancing performance optimization with user experience
As web applications continue to grow in complexity, techniques like throttling become increasingly important for maintaining smooth, responsive user interfaces and efficient server communication. By mastering throttling, developers can create more performant and user-friendly applications.
We encourage you to implement throttling in your projects, experiment with different approaches, and continue exploring advanced optimization techniques. Remember, the goal is not just to improve performance metrics, but to create better experiences for your users.
“Performance is not just about speed; it’s about creating a seamless, responsive experience that keeps users engaged and satisfied.”
Happy coding, and may your applications be ever performant!