Introduction
Array manipulation is a fundamental aspect of programming in React, essential for managing dynamic data and altering states that impact user interfaces. Developers often encounter scenarios where they need to efficiently add or modify objects within arrays, particularly in state management using hooks or class components. This blog aims to delve into advanced techniques and best practices for pushing objects into arrays effectively, enhancing performance and ensuring smoother state updates in your React applications. Whether you’re building complex applications or simply refining functionality, mastering these skills will significantly elevate your development strategy.
Understanding Array Manipulation in React
Basics of array manipulation
Array manipulation involves altering, adding, or removing elements from an array, which is a crucial aspect of managing state in React applications. Unlike primitive data types, arrays in JavaScript are mutable, meaning they can be modified after their creation. This mutability is both a feature and a pitfall, as direct mutations can lead to unintended side effects, especially in React where state immutability is a key principle for predictable rendering.
In React, arrays are commonly used as state variables to store lists of data, such as user inputs, API responses, or UI elements. Therefore, understanding how to interact with arrays—specifically, how to add or remove items without directly mutating them—is fundamental. The typical methods for array manipulation in React include using JavaScript ES6 features like the spread operator, and functions like \`concat\`, which are preferred over direct manipulation methods like \`push\`, which alter the original array.
Benefits of mastering array manipulation in React
Mastering array manipulation in React brings several benefits that can enhance the efficiency and performance of applications:
1. Immutable State Management: By manipulating arrays correctly, developers can ensure that the state remains immutable, which is critical for React’s re-rendering process. Immutable state allows React to quickly determine when changes have occurred and when a component should re-render, improving performance.
2. Enhanced Readability and Maintenance: Clear and predictable array manipulation techniques make the code more readable and easier to maintain. Other developers on the team, or those maintaining the code at a later date, can quickly understand how the state is being updated.
3. Fewer Bugs and Side Effects: Avoiding direct mutation of arrays helps prevent subtle bugs that occur when multiple components or functions rely on the same array. When arrays are manipulated properly, it ensures that changes in one part of the application do not inadvertently affect other parts.
4. Better State Management Practices: Proficiency in array manipulation encourages developers to adopt better overall state management practices, contributing to more robust and scalable React applications.
Techniques for Efficient Object Pushing in Arrays
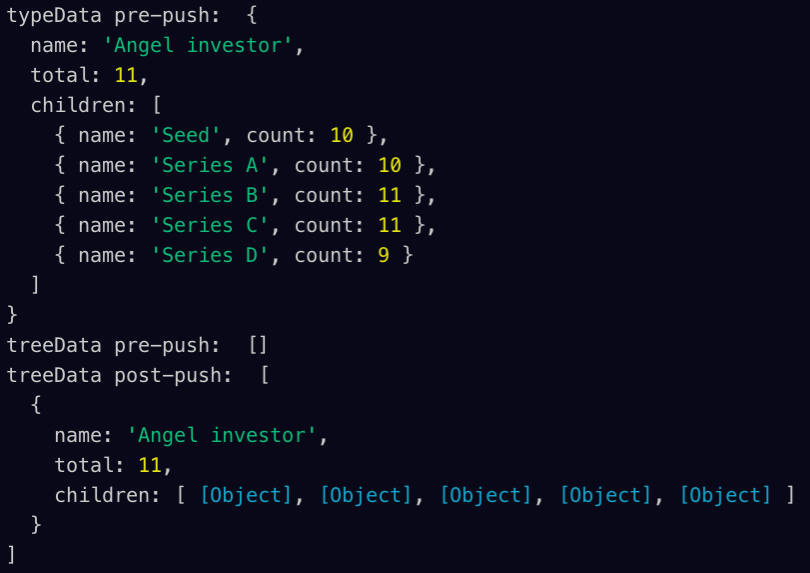
Using spread operator for object pushing
The spread operator (\`…\`) is an ES6 feature that provides a very readable and efficient way to add items to arrays without mutating the original array. This operator unpacks the elements of one array into a new array, enabling developers to easily combine elements from multiple sources or add new items.
To push an object into an array using the spread operator, you can do the following:
\`\`\`javascript
const originalArray = [{ id: 1, name: ‘John’ }, { id: 2, name: ‘Jane’ }];
const newObject = { id: 3, name: ‘Doe’ };
const newArray = […original=sizeof=(“JavaScriptf”), newObject];
\`\`\`
This approach ensures that the original array is not changed, and \`newArray\` is a new instance containing all original objects plus the new object. Using the spread operator is particularly useful in React’s state management, where maintaining immutability is crucial. It can be used inside a \`setState\` operation in class components or the \`useState\` hook in functional components, contributing to cleaner and more predictable code.
Leveraging concat method for efficient pushing
Another effective and safe way to add objects to an array in React is through the \`concat\` method. This method merges two or more arrays by returning a new array and does not mutate the original array, adhering to React’s principles for state immutability.
Here’s how you can use \`concat\` to add an object to an array:
\`\`\`javascript
const originalArray = [{ id: 1, name: ‘John’ }, { id: 2, name: ‘Jane’ }];
const newObject = { id: 3, name: ‘Doe’ };
const newArray = originalArray.concat(newObject);
\`\`\`
The \`concat\` method can handle both array elements and individual elements seamlessly, making it a versatile tool for array manipulation. It’s especially useful when you have to add multiple items or merge several arrays at once. The clear syntax and the non-mutating nature make it a reliable choice for updates in stateful components.
Implementing push method with caution
While the \`push\` method is a standard JavaScript function to add elements to the end of an array, its use within React should be approached with caution. This is because \`push\` directly modifies the original array, which goes against the principle of immutability in React’s state management.
However, there are cases where \`push\` might still be relevant. For instance, in a scenario where an array is local to a function and not part of the component state, or when you are working with copies of arrays rather than the originals. If you must use \`push\` in a React environment, ensure that it does not mutate state directly. Here’s how you can use \`push\` safely:
\`\`\`javascript
const originalArray = [{ id: 1, name: ‘John’ }, { id: 2, name: ‘Jane’ }];
const newObject = { id: 3, name: ‘Doe’ };
const newArray = […originalArray];
newArray.push(newObject);
\`\`\`
In this example, \`newArray\` is a copy of \`originalArray\`, allowing \`push\` to be used without affecting the original array. This approach maintains immutability and allows for the efficient addition of new items when necessary.
By using these techniques thoughtfully, React developers can efficiently manipulate arrays while preserving state immutability, leading to more reliable and maintainable applications.
Advanced Array Manipulation Methods in React
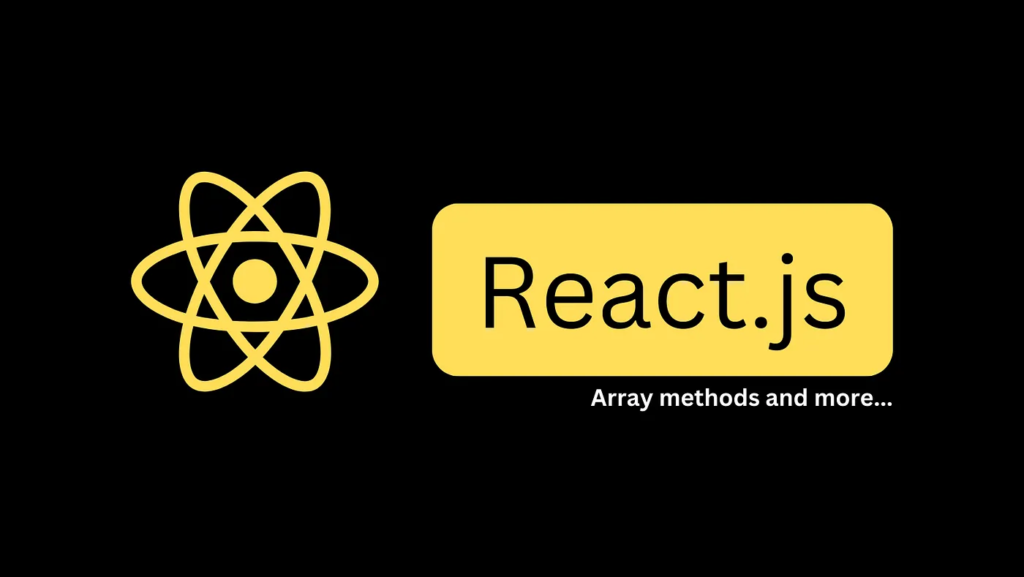
Image courtesy: medium
Using map method for object transformation
The \`map\` method in JavaScript is particularly useful in React for transforming arrays of objects. This method creates a new array by applying a function to every element of the original array without altering it. When you have an array of objects, you can utilize \`map\` to modify specific properties of each object or to generate new properties altogether. For instance, if you have an array of user objects and you want to add a new property called \`isActive\`, you can do so like this:
\`\`\`javascript
const usersWithStatus = users.map(user => {
return { …user, isActive: true };
});
\`\`\`
This technique ensures that each object in the new array has the \`isActive\` property set to true, showcasing \`map\` as an ideal choice for object transformation in React. It not only maintains immutability but also leverages the re-rendering process efficiently by creating a brand new reference for the array.
Applying filter method for object filtering
The \`filter\` method helps developers write cleaner, more readable code by providing a straightforward way to select items from an array that meet certain criteria. This is exceptionally useful in React, especially when dealing with lists of objects where you need to display only those that satisfy a specific condition. For example, to filter out users who are active, you can use:
\`\`\`javascript
const activeUsers = users.filter(user => user.isActive);
\`\`\`
This code segment will produce a new array containing only the users whose \`isActive\` property is true. Utilizing \`filter\` is effective for managing state in scenarios where you need to display filtered results based on user interactions or other dynamic conditions. By returning a new array, the \`filter\` method ensures that React can efficiently update the UI in response to data changes.
Exploring reduce method for complex operations
The \`reduce\` method is a powerful tool for performing more complex manipulations on arrays of objects. It reduces the array to a single value, which can be a number, an object, or even another array, making it highly versatile for various scenarios. For example, if you want to calculate the total number of active users, you could use:
\`\`\`javascript
const totalActive = users.reduce((count, user) => {
return user.isActive ? count + 1 : count;
}, 0);
\`\`\`
This example demonstrates how \`reduce\` can be used to accumulate a value based on each object in the array. It’s particularly useful for aggregating data or constructing new data structures from an array of objects, which can then be used for rendering or further processing in React. By properly understanding and utilizing the \`reduce\` method, developers can handle complex data structures more effectively in their applications.
Best Practices for Array Manipulation in React
Avoiding mutating state directly
One of the fundamental best practices in React is to avoid mutating state directly because React’s state management and rerender mechanisms are built around the principle of immutability. When the state is mutated directly, React may not recognize these changes, leading to potential bugs and inconsistent UI updates. Instead, use methods like \`map\`, \`filter\`, and \`reduce\`, or the spread operator to create new array objects:
\`\`\`javascript
this.setState(prevState => ({
users: […prevState.users, newUser]
}));
\`\`\`
Using such approaches ensures that you are creating a new array rather than modifying the existing one, allowing React to correctly detect changes and update the component effectively. It is crucial to internalize this principle to maintain predictable and bug-free applications.
Properly keying list items for efficient renders
When rendering lists of items in React, it is paramount to assign a unique \`key\` prop to each element. This key helps React identify which items have changed, are added, or are removed. This is particularly important when manipulating arrays where items can change positions. Keys should be unique and stable, not based on array index but rather on unique IDs:
\`\`\`javascript
users.map(user => );
\`\`\`
Keying properly ensures that React can optimize re-rendering performance and minimize DOM manipulation costs, leading to faster, more efficient, and error-reduced UI updates.
Optimizing performance with memoization techniques
Memoization can significantly optimize performance in React applications, especially when working with complex array manipulations. Memoization is a caching technique that stores the results of expensive function calls and returns the cached result when the same inputs occur again. In React, \`useMemo\` and \`React.memo\` are handy for preventing unnecessary recalculations and re-renders:
\`\`\`javascript
const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
\`\`\`
By memoizing the results of functions that transform, filter, or reduce large arrays, you can avoid costly re-computations and ensure that components only re-render when truly necessary. It’s an effective strategy that optimizes not only individual component performance but the overall responsiveness of the application.
By mastering these advanced array manipulation methods and best practices in React, developers can write more efficient, clean, and maintainable code. Such skills enhance application performance and user experience, making them critical for any React developer’s toolkit.
Conclusion
In mastering array manipulation within React, it’s crucial to apply efficient techniques for modifying arrays, especially when dealing with the addition of objects. Understanding and implementing methods like using the spread operator or \`Array.prototype.concat()\` ensures that your application remains performant and scalable. Moreover, adhering to the immutable update patterns is not only a best practice in React but also helps in maintaining clean, readable, and bug-resistant code. By incorporating these methodologies, developers can enhance their React applications, making them more robust and responsive. Remember, the key to effective array manipulation is keeping the state manageable and the updates predictable. With these tools and techniques at your disposal, pushing objects into arrays will be both efficient and effective, paving the way for more complex state management scenarios.
Pingback: Promises and Async/Await to Avoid Callback Hell