Table of Contents
Introduction
React remains one of the most popular JavaScript libraries for building user interfaces, particularly due to its efficient way of managing changes in state with updates that render only components affected by the change. However, as applications scale, managing state and dispatching actions efficiently becomes more crucial to ensure that the app remains fast and responsive. Inefficient dispatching can lead to sluggish performance and a poor user experience. Optimizing dispatch performance can significantly improve the responsiveness of a React application. This blog explores various techniques to enhance dispatch performance, ensuring a smoother and more efficient user interaction.
Understanding React Dispatch
Image courtesy: Unsplash
Overview of React dispatch functionality
React’s dispatch function is a crucial part of its state management, especially when using context or Redux for handling application state. Essentially, dispatch is a method used to send actions to the Redux store, or alter the state context in React apps, telling it how to mutate the state. This function is typically used in conjunction with reducers, which determine how the application’s state changes in response to actions sent by dispatch.
When an action is dispatched, it is described by a plain JavaScript object that tells the reducer what type of operation to perform. The reducer then interprets this action and updates the state accordingly. Given that React’s UI updates are driven by state changes, the efficiency of the dispatch function directly impacts the performance of the app.
Importance of optimizing dispatch performance
Optimizing the dispatch function is pivotal for several reasons. First, it ensures that state changes are handled promptly and efficiently, keeping the application responsive to user interactions. If dispatch actions are slow or inefficient, it can lead to a sluggish interface, negatively impacting user experience.
Furthermore, optimizing dispatch can lead to fewer re-renders of the components, which is particularly important in large-scale applications where even slight performance issues can magnify. Efficient dispatch operations help maintain an application’s scalability and responsiveness, making optimization not just a luxury but a necessity for complex applications.
Techniques for Optimizing Dispatch Performance
Memoization for reducing redundant dispatch calls
One effective technique to optimize dispatch in React involves the use of memoization. Memoization is an optimization strategy that involves caching the results of expensive function calls and returning the cached result when the same inputs occur again. For React dispatch, this means preventing unnecessary state updates and associated re-renders by ensuring that identical dispatch calls do not repeatedly trigger state changes.
To implement memoization:
– Identify repetitive dispatch calls that result in the same state updates.
– Use a memoization technique, such as creating a custom hook or employing lodash’s \`memoize\` function, to cache and recall dispatch actions.
– Ensure that dependencies passed to dispatched actions are stable, preventing excessive cache misses and recalculations.
This technique not only optimizes the computational efficiency of dispatches but also significantly reduces the workload on the reducer, making the application faster and more reactive.
Using useCallback and useMemo hooks for optimized rendering
React’s \`useCallback\` and \`useMemo\` hooks can also play a vital role in optimizing the performance of dispatch functions. These hooks are designed to limit the number of re-renders and recalculations by memorizing callback functions and values.
– \`useCallback\`: This hook returns a memoized version of the callback function that only changes if one of the dependencies has changed. Itโs particularly useful when passing callbacks to optimized child components that rely on reference equality to prevent unnecessary renders.
– \`useMemo\`: This hook returns a memoized value. Use it to optimize re-renders by memorizing complex calculations, thus preventing these calculations from being run on every render.
By using these hooks, you can ensure that components do not unnecessarily re-render due to changes in dispatch functions or the values involved in these functions, improving performance, especially in components that re-render often.
Batched Updates to minimize re-renders
Batched updates are another powerful optimization strategy in React. In traditional React applications, each state update could potentially lead to a new render. However, batched updates consolidate multiple state updates into a single rerender, significantly improving performance.
React 18 introduced automatic batching which extends the batching of update calls not only during React events but also during promises, timeouts, and native event handlers. If using earlier versions of React or when automatic batching doesnโt cover certain scenarios, you can manually batch updates using \`ReactDOM.unstable_batchedUpdates\` to combine several dispatch calls into one update phase.
To effectively employ batched updates:
– Identify areas in your code where multiple actions update the state independently over short periods.
– Leverage automatic batching or manually wrap the dispatch calls within \`unstable_batchedUpdates\`.
– Ensure that the logic leading up to dispatched actions can tolerate being executed in a batched manner.
By incorporating these techniques, developers can ensure that dispatch is not only triggered efficiently but also results in minimized re-renders, leveraging React’s state management system to deliver smoother and more responsive applications. This, in turn, enhances overall user experience by delivering a more fluid interaction landscape.
Monitoring and Profiling
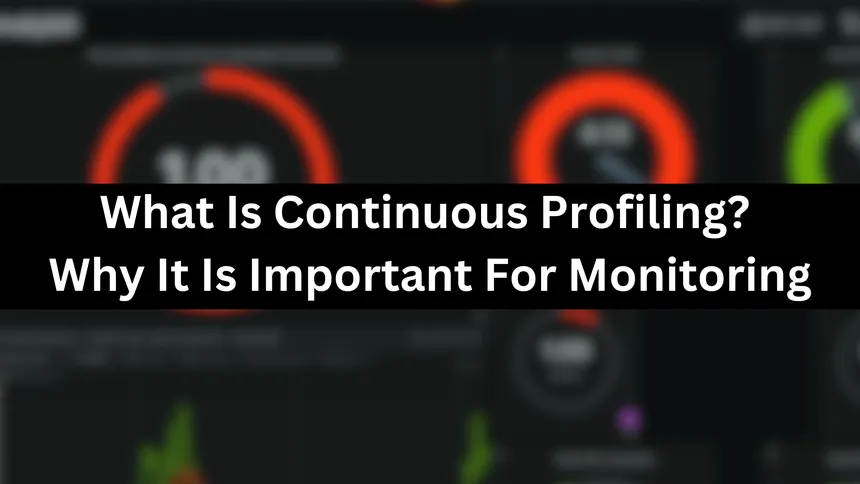
Identifying performance bottlenecks in React dispatch
Identifying performance bottlenecks in React dispatch begins with understanding how components render and update in response to state changes. When a state change occurs through dispatch actions, React re-renders the component and its child components, which can lead to performance issues if not managed efficiently. To isolate bottlenecks, you should observe how often and why components are re-rendering
One practical approach is to implement the React DevTools profiler in your development environment. This tool allows you to record renderings of your application and inspect the cost of a render at each component level. By examining the flame graph provided by React DevTools, developers can pinpoint excessive re-rendering and identify which components contribute most heavily to performance degradation.
Another strategy involves using custom logging in your reducers or middleware to track how actions affect the appโs performance. Logging the execution time of actions and the number of times a reducer is called can reveal inefficiencies. If certain actions lead to frequent updates across numerous components, it might be a sign that the state management architecture needs revisiting or optimizing.
Additionally, incorporating performance metrics, such as time-to-interactive and component load times, helps solidify a baseline from which you can measure the impact of adjustments made to dispatch processes or component re-renders.
Utilizing browser developer tools for performance analysis
Browser developer tools provide a comprehensive suite of resources to aid in the detection and resolution of performance bottlenecks specific to the web environment. Tools such as Chromeโs Performance tab offer insights into the runtime performance of your React application. Here, you can utilize the JavaScript profiler to monitor function calls and measure the time spent in scripts.
The Network tab is instrumental in analyzing the sequence and duration of network requests, which can indirectly affect dispatch performance if data fetching is involved. Slow network responses can delay the dispatch of actions reliant on those responses, thereby increasing the perceived load time of your application.
Memory leaks are another critical area to monitor. Using the Memory tab, developers can examine heap snapshots to identify memory leaks that might be occurring during dispatches or as a result of new object allocations linked to state updates. Excessive memory usage can lead to slower garbage collection processes, which in turn can slow down your applicationโs responsiveness.
The Performance tab also allows you to simulate different conditions, such as slow network or CPU throttling, giving developers a realistic understanding of how their applications will perform under various conditions and how dispatch actions are managed under these constraints.
Case Studies and Examples
Real-world scenarios showcasing optimized dispatch performance
One compelling case study involves a popular social media platform that implemented memoization techniques in its React components to reduce unnecessary re-renders caused by frequent, similar dispatch calls. Memoization, which caches the results of function calls, was used to compute derived data from the state, ensuring that components relying on this data only re-rendered when necessary. This change significantly reduced the load time of user feeds and improved the overall responsiveness of the application.
Another example is an e-commerce site that optimized its cart and checkout flow. The developers used Reduxโs \`reselect\` library to create selectors that compute derived data from the state, which is a common pattern for reducing unnecessary re-renders. By carefully structuring their state to minimize deep object references and combining it with efficient selector functions, they were able to keep component updates highly performative even under heavy load conditionsโlike during sales events.
These scenarios underscore the importance of thoughtful state management and component re-render strategies. They show that optimizing dispatch performance needs a holistic approach that considers how data flows through the application and how state changes impact component hierarchies.
Code snippets demonstrating implementation of performance techniques
Implementing performance optimization techniques in React is best demonstrated through practical code examples. Below are snippets that illustrate how to apply some common performance improvements:
1. Memoizing Components:
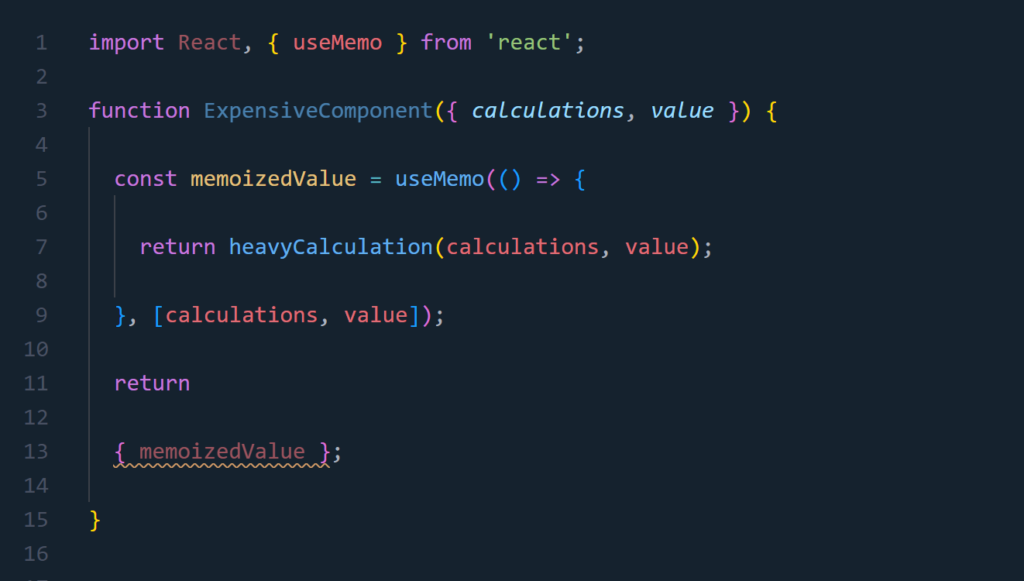
This code demonstrates the use of Reactโs \`useMemo\` hook to avoid recalculating values unless dependencies (\`calculations\`, \`value\`) change, which saves potentially expensive computational costs on re-renders.
2. Selectors in Redux:
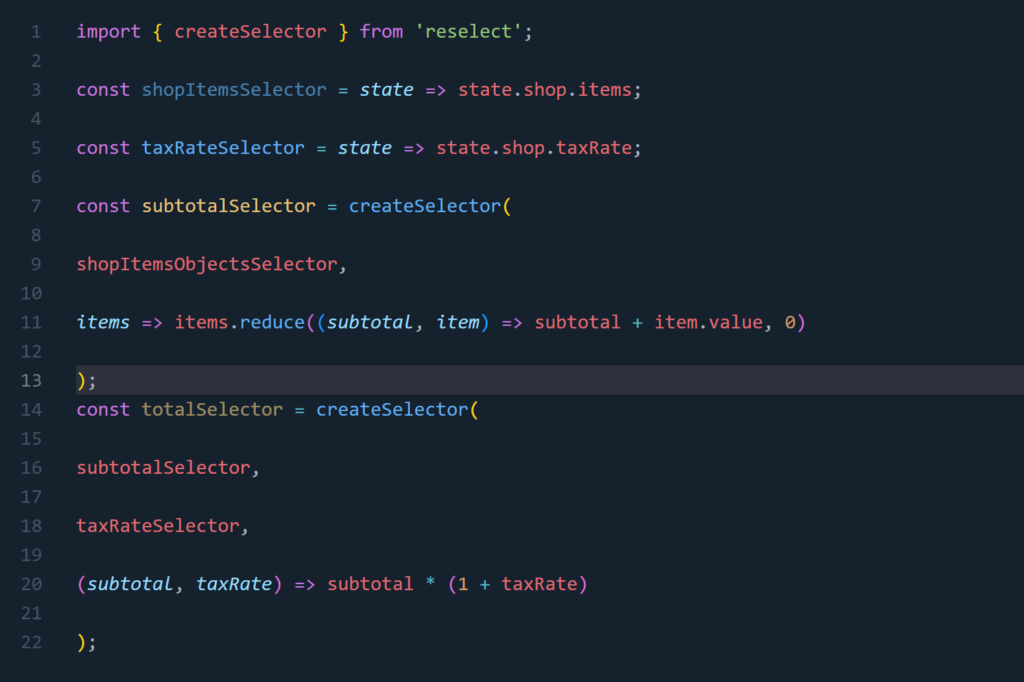
Here, \`createSelector\` from \`reselect\` is used to compute derived data. This setup ensures that \`subtotalSelector\` and \`totalSelector\` are only recomputed when their respective inputs change, minimizing unnecessary computations during store updates.
By integrating these techniques, developers can enhance the dispatch performance in React applications, leading to faster interactions and a smoother user experience.
Conclusion
In the realm of React development, optimizing dispatch processes is integral for ensuring streamlined, high-performance applications. The techniques discussed provide structured pathways to enhance the efficiency of your React applications. By meticulously managing component renders, and strategically structuring your state and actions, you can significantly boost the performance of your React dispatches.
Understanding and implementing the concept of memoization with React.memo and useMemo ensures that components only re-render when necessary, thereby saving valuable computational resources. This technique is particularly crucial in complex applications where component re-rendering can become a costly operation. Furthermore, the use of useCallback helps in avoiding the creation of unnecessary functions during render, which can also lead to performance improvements, especially in components that involve complex logic or are deeply nested.
The structured use of Redux, with techniques such as normalizing state shape and leveraging reselect selectors, enhances state management efficiency. By flattening the state and fetching only necessary data, applications become more predictable and less prone to errors, all while improving performance. It’s important to understand that while Redux provides powerful tools for managing state in large applications, its benefits are best realized when used appropriately and in moderation to avoid over-complication.
Another impactful approach is the batching of actions. By grouping multiple state updates into a single re-render cycle, you can minimize the number of render cycles, which is particularly useful in scenarios involving complex state manipulations or updates that occur in rapid succession. React’s built-in batching or alternative libraries like Redux-batch can be utilized to achieve this optimization.
Tailoring React’s Context API for global state management, when used judiciously, can offer a simpler and more efficient alternative to props drilling or even Redux in smaller applications. This can enhance developer productivity and application performance when used in scenarios that do not require the robustness of larger state management libraries.
Each project may require a different combination or emphasis on these techniques depending on the specific performance challenges and development contexts. Therefore, continuous performance monitoring and profiling using tools like React Developer Tools, and adjusting your optimization strategy accordingly, remains a best practice. Keeping up-to-date with the latest React updates and community practices can also provide new opportunities for performance improvements.
In summary, optimizing dispatches in React is essential for creating faster and more responsive applications. By carefully applying the techniques of function memorization, thoughtful Redux setups, batching actions, and appropriately using the Context API, developers can greatly enhance user experience and application performance. It’s important to focus on understanding the underlying cause of performance bottlenecks specific to your application and address them with the most suitable optimization techniques. Through thoughtful implementation and continuous monitoring, your React applications will not only perform better but also provide a smoother experience for end-users.
FAQ
What is dispatch in React?
Dispatch in React is a function used in conjunction with the useReducer hook or Redux, allowing you to send actions to update the state. It triggers the state management process, enabling React applications to update the UI based on the changes in state.
How can optimizing dispatch improve performance?
Optimizing dispatch can significantly enhance performance by reducing unnecessary renders and ensuring efficient state updates. By streamlining the dispatch process, you minimize the workload on the browser, leading to faster UI updates and an overall smoother user experience.
When should I consider optimizing dispatch?
Optimization should be considered when:
– The application feels sluggish or slow.
– Profiling tools indicate frequent re-renders or high computational costs associated with state updates.
– Scaling up the application leads to noticeable performance degradation.
Efficient management of dispatch actions can prevent these issues, contributing to a responsive and high-performing application.
Pingback: Email Deployment Metrics: Key Performance Indicators