Custom hooks in React offer a powerful way to extract and reuse logic across your components. useActionState
is a pattern within this paradigm that enhances state management, enabling developers to handle actions and state transitions more succinctly. This innovative approach not only simplifies the code but also improves its maintainability and scalability. By mastering useActionState
, developers can streamline their workflows, reduce boilerplate, and focus on building dynamic user interfaces more effectively. In this blog, we’ll explore how to effectively use custom hooks with useActionState
to elevate your React projects. Whether you’re a novice looking to grasp the basics or an experienced developer aiming to refine your skills, this guide will provide valuable insights into efficient state management techniques.
Exploring Custom Hooks
Custom hooks are a fundamental concept in React that allow you to extract component logic into reusable functions. A custom hook is a JavaScript function whose name starts with “use” and that may call other hooks like useState
or useEffect
from within it. By employing custom hooks, developers can share logic across different components or applications without repeating code. This ability increases the maintainability of the codebase, reduces complexity, and enhances modularity.
Benefits of Using Custom Hooks
Utilizing custom hooks in React applications offers several advantages:
- Code Reusability and Organization: Custom hooks allow you to write the logic once and reuse it throughout your application, which keeps your codebase DRY (Don’t Repeat Yourself).
- Separation of Concerns: They help in separating logic from UI components, which simplifies testing and debugging.
- Simpler Lifecycle Management: Hooks simplify lifecycle management in functional components through
useState
anduseEffect
, making state management and side effects easier to handle.
Custom hooks can also leverage other hooks within them to build complex functionality that can be easily maintained and scaled.
Introduction to useActionState
useActionState
is a potent custom hook tailored for managing state transitions explicitly associated with user actions or events in a React application. It is designed to streamline the process of state management in scenarios where the state depends heavily on user interactions. This hook not only makes the code more readable but also more predictable by centralizing the state logic related to specific actions, such as clicks, form submissions, or any interactive element events.
Mastering useActionState
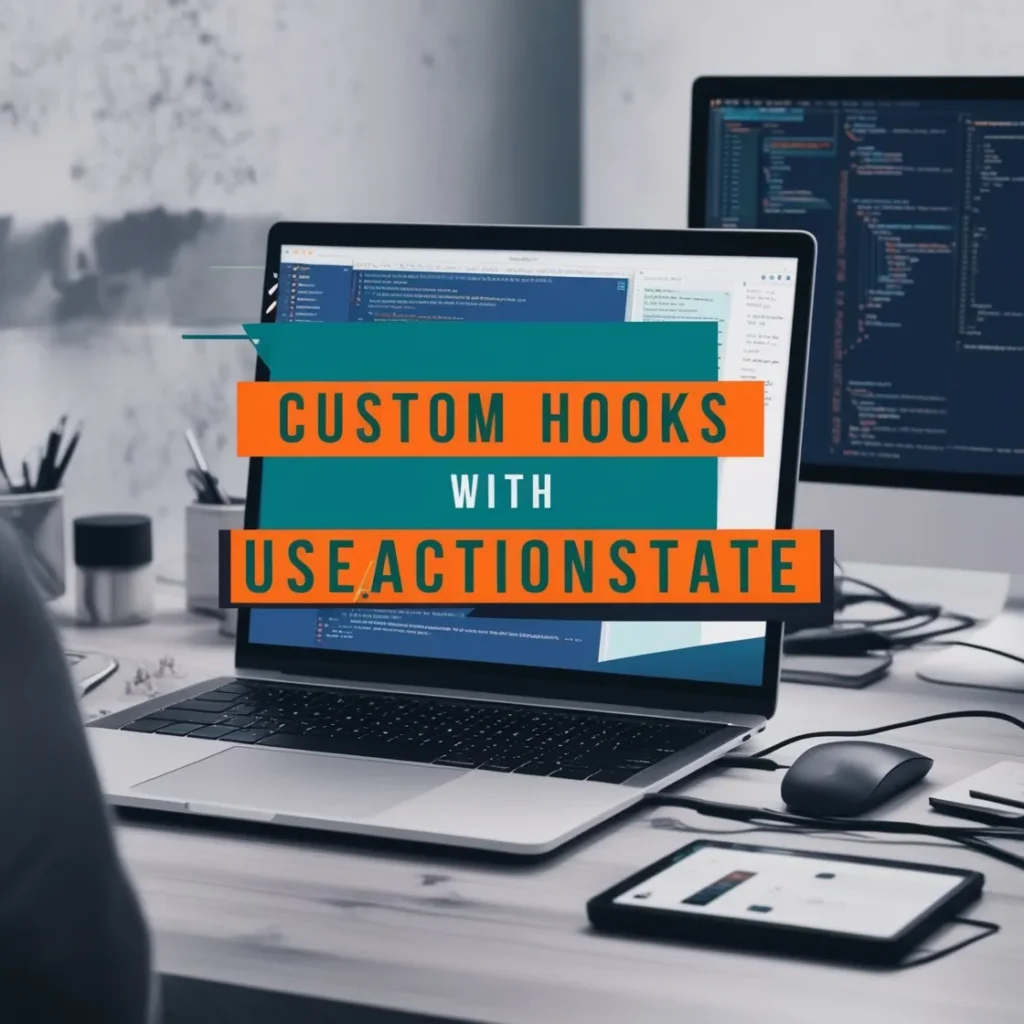
Setting up useActionState
To set up useActionState
, you start by defining a function that adheres to the naming convention of hooks, i.e., it should start with use
. This function will manage both the state and associated actions. Here’s a simple example to illustrate.
function useActionState(initialValue) {
const [value, setValue] = useState(initialValue);
const updateAction = newValue => {
// Logic to handle the state update
setValue(newValue);
};
return [value, updateAge];
}
In this setup, useActionState
initializes a state with initialValue
and updates it through updateAction
. This encapsulates the action-specific logic within the hook, making it reusable and easier to manage.
Implementing useActionAction in React Components
Implementing useActionState
in React components is straightforward. Consider a scenario where you have a button that increments a counter:
function Counter() {
const [count, setCount] = useActionState(0);
return (
{count}
setCount(count + 1)}>Increment
);
}
Here, useActionState
is used to manage the count
state. setCount
is the action derived from the custom hook, which updates the state when the button is clicked. This pattern can be applied to various user elements to manage different kinds to legally manage states corresponding to user actions.
Advanced Techniques with useActionState
To truly master useActionState
, you can integrate it with other hooks and context for more complex state management tasks. For example, integrating useActionState
with useEffect
can handle side effects triggered by state changes. Consider a case where state updates need to fetch data from an API:
function useFetchData(actionState) {
const [data, setData] = useState(null);
useEffect(() => {
async function fetchData() {
const response = await fetch('https://api.example.com/data');
const json = await response.json();
setData(json);
}
fetchData();
}, [actionState]); // Dependency on action state
return data;
}
function App() {
const [query, setQuery] = useActionState('');
const data = useFetchData(query);
return (
value={query}
onChange={e => setQuery(e.target.value)}
placeholder="Search..."
/>
);
}
In this example, useActionState
manages the search query state, and useFetchData
is a custom hook that fetches data based on this state. The useEffect
in useFetchData
re-runs whenever query changes, ensuring that data is fetched according to the current state.
Moreover, useActionState
can be used with the Context API to manage global state across different components within an application. This is particularly useful in larger applications where passing props through many levels can become cumbersome.
By understanding and implementing these advanced techniques with useActionState
, developers can create highly efficient and effective React applications with optimized state management and better performance.
Best Practices for Custom Hooks
Creating custom hooks in React allows you to extract component logic into reusable functions. To ensure that these hooks are easy to manage, maintain, and scale, it’s essential to follow best practices from the get-go.
Naming Conventions
One of the first considerations when creating custom hooks should be the naming convention. Names should clearly communicate what the hook does, enhancing readability and maintainability of your code:
- Start with “use”: Following the convention established by React’s built-in hooks, all custom hooks should start with the word “use”. For example, useFetch, useStateManager.
- Be descriptive: Choose names that explicitly describe the hook’s purpose. For instance, useArray instead of useData if the hook specifically manages array operations.
- Avoid abbreviations: Unless an abbreviation is widely understood, opt for full words to make your code more accessible to new developers or external teams.
Reusability and Scalability
To maximize the value of custom hooks, they should be designed with reusability and scalability in mind:
- Encapsulate logic: Ensure that hooks are self-contained and do not rely on external states or props unless absolutely necessary. This makes them more adaptable and easier to manage as the project grows.
- Use parameters and options: Allow customization through parameters so that the hook can be adjusted for various use cases without modifying the codebase. For example, a useFetch hook could accept URL, requestOptions, and an autoFetch flag.
- Consider performance: Minimize side effects and consider using optimizations like useMemo or useCallback to ensure that hooks do not degrade app performance.
By following these guidelines, your custom hooks will be more effective and versatile, enabling easier feature development and code maintenance.
Testing Custom Hooks
Testing is pivotal to confirming the reliability and stability of custom hooks. Here’s how to effectively test your hooks:
- Use testing libraries: Tools like React Testing Library and Jest provide utilities to test custom hooks in isolation. These tools simulate React environments and allow you to assert, manipulate, and monitor hook behavior during tests.
- Test various states: Make sure you test the hook across its possible states. For example, testing a useFetch hook means verifying its behavior when fetching data, handling errors, and after data is loaded.
- Include integration tests: While unit testing custom hooks is crucial, also include integration tests where hooks interact with other components or hooks. This ensures that the hooks perform well within the broader system.
By rigorously testing your hooks, you safeguard the application against potential regressions and bugs introduced through shared logic components.
Real-world Examples
To elucidate how custom hooks can significantly enhance your projects, let’s look at some practical implementations. These real-world examples showcase how custom hooks simplify operational complexity, making code cleaner and more maintainable.
- UseNetworkStatus: This hook manages and provides network status. In environments where connectivity affects functionality, it can be beneficial to have a single source of truth for connection status.
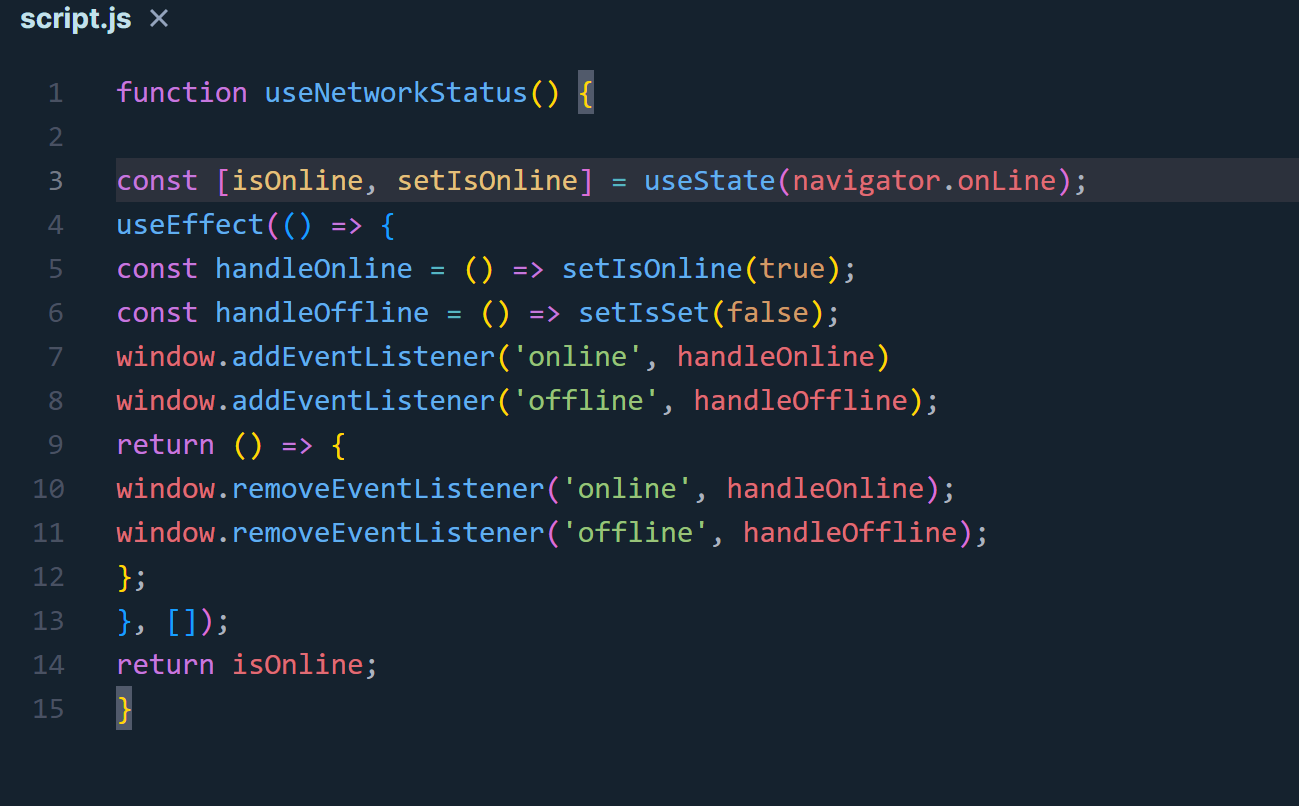
- UseForm: Managing form inputs with validations can be cumbersome. A useForm hook can streamline form handling by abstracting logic related to input changes, validation, and form submission.
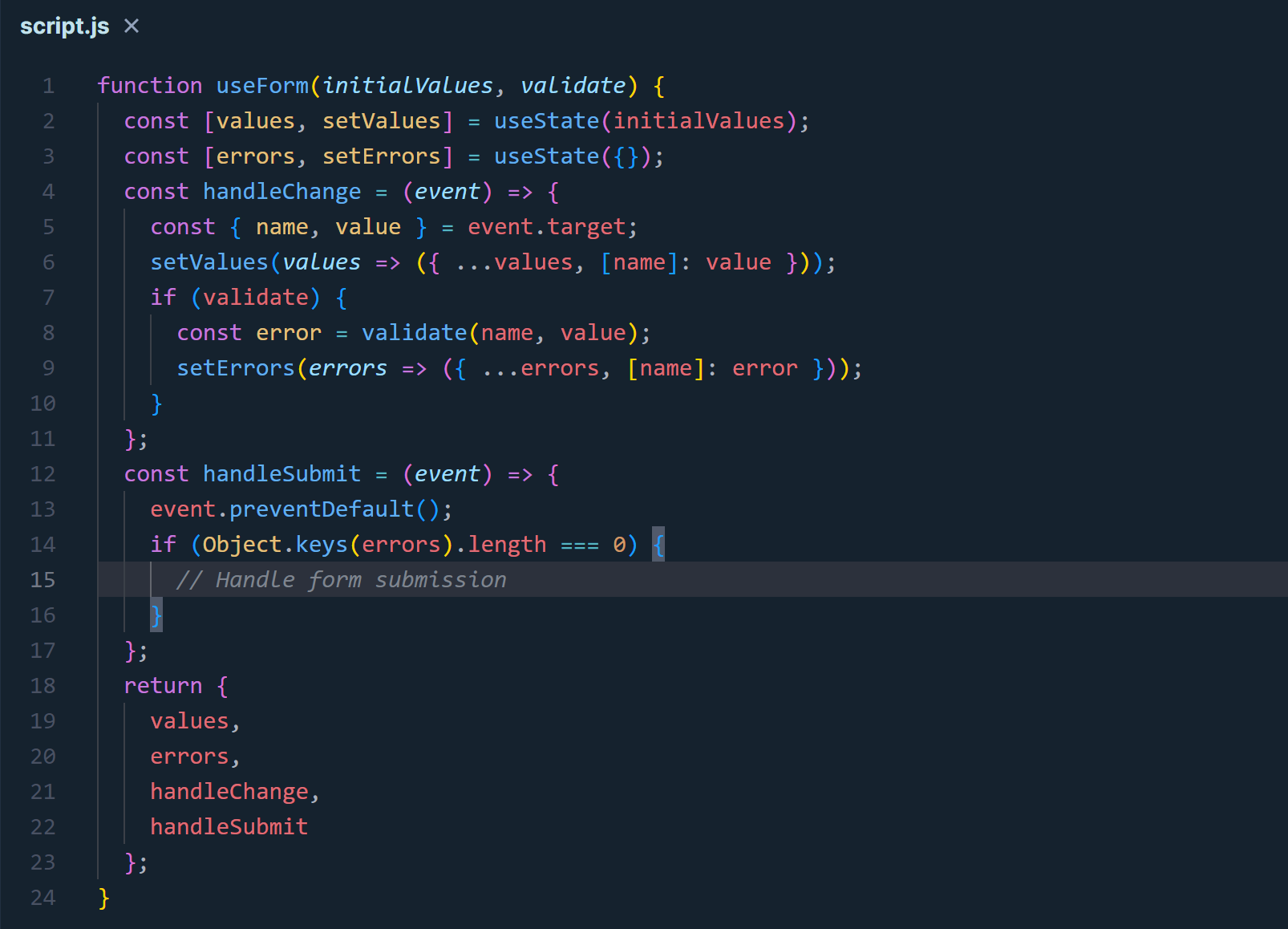
These examples demonstrate the power of custom hooks in creating more readable, less error-prone applications. By focusing on best practices, such as proper naming, building for reusability and scalability, and thorough testing, developers can create powerful tools that simplify coding tasks and enhance application integrity.
Conclusion
Incorporating custom hooks like useActionState into your React projects can vastly improve the readability, scalability, and maintainability of your code. By centralizing and simplifying the logic related to state management and actions, useActionControl allows developers to write cleaner, more declarative and organized code. Remember, the key benefits of using this pattern include less boilerplate, improved reusability, and a clearer separation of concerns. As you continue to develop and refine your React applications, consider integrating useActionState to enhance your project’s overall efficiency and effectiveness. Embrace the power of custom hooks and watch your web development skills soar.