Introduction
WebAssembly (Wasm) has emerged as a game-changing technology in the world of web development. This low-level language brings near-native performance to web applications, opening up new possibilities for developers across the stack. In this article, we’ll explore how Web-Assembly is transforming full-stack development and why it’s becoming an essential tool for modern web applications.
What is Web Assembly?
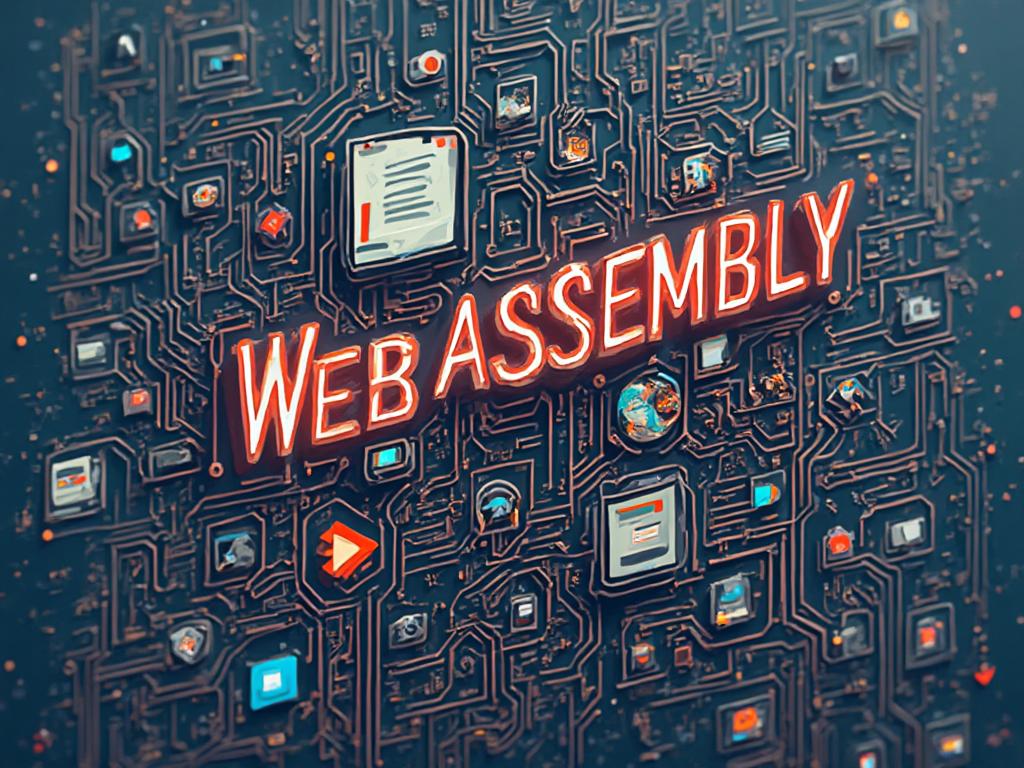
Web Assembly is a binary instruction format designed for efficient execution in web browsers. It serves as a compilation target for high-level languages, allowing developers to run complex applications in the browser at near-native speed.
Brief History and Evolution
- 2015: Web Assembly project announced
- 2017: Initial release, supported by major browsers
- 2019: Became a W3C recommendation
- 2021: WASI (Web Assembly System Interface) introduced, expanding server-side capabilities
Understanding Web Assembly
Core Concepts and Technology Stack
Web Assembly operates alongside JavaScript in the browser’s runtime environment. It’s designed to complement JavaScript, not replace it. The core concepts include:
- Module: A compiled Web Assembly binary
- Instance: A module with its state and memory
- Memory: A resizable ArrayBuffer that both Wasm and JavaScript can access
- Table: An array of references, typically used for function pointers
How Wasm Works
- Compilation: High-level languages are compiled to Wasm bytecode
- Loading: The Wasm module is fetched and instantiated in the browser
- Execution: The browser’s Wasm engine executes the bytecode
Comparison with Traditional JavaScript
While JavaScript is interpreted or JIT-compiled at runtime, Web Assembly is pre-compiled, leading to faster startup times and more predictable performance.
// JavaScript
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
// WebAssembly (in C, compiled to Wasm)
int fibonacci(int n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
The Web Assembly version, when compiled, can execute significantly faster, especially for computationally intensive tasks.
Benefits of Web Assembly in Full-Stack Development
Performance Enhancements
Wasm offers near-native performance, making it ideal for computationally intensive tasks. It’s particularly beneficial for:
- Complex calculations
- Game engines
- Audio and video processing
- Cryptography
Language Versatility and Interoperation
Developers can write modules in languages like C, C++, Rust, or Go, and seamlessly integrate them with JavaScript. This versatility allows teams to leverage existing codebases and expertise.
Security Advantages
Web Assembly runs in a sandboxed environment, providing strong security guarantees. It has no direct access to the DOM or other web APIs, reducing potential attack surfaces.
Use Cases and Applications
Client-Side Applications
- Gaming: Complex game engines can now run efficiently in browsers
- Graphics-Intensive Apps: CAD software, 3D modeling tools
- Audio/Video Editing: Real-time processing and effects
Server-Side Applications
- Computational Tasks: Data analysis, machine learning inference
- Serverless Functions: Fast cold start times and efficient execution
Integrating with Existing JavaScript Frameworks
Web Assembly can be seamlessly integrated with popular frameworks:
// React component using a Wasm module
import React, { useEffect, useState } from 'react';
import { fibonacci } from './fibonacci.wasm';
function FibonacciCalculator() {
const [result, setResult] = useState(0);
useEffect(() => {
const calculate = async () => {
const fibResult = await fibonacci(10);
setResult(fibResult);
};
calculate();
}, []);
return <div>Fibonacci(10) = {result}</div>;
}
Integrating WebAssembly with Front-End Technologies
Compiling Code to WebAssembly
To compile code to WebAssembly, you can use tools like Emscripten for C/C++ or wasm-pack for Rust.
Example using Emscripten:
emcc fibonacci.c -o fibonacci.js -s WASM=1 -s EXPORTED_FUNCTIONS='["_fibonacci"]'
Using Wasm with React, Angular, and Vue.js
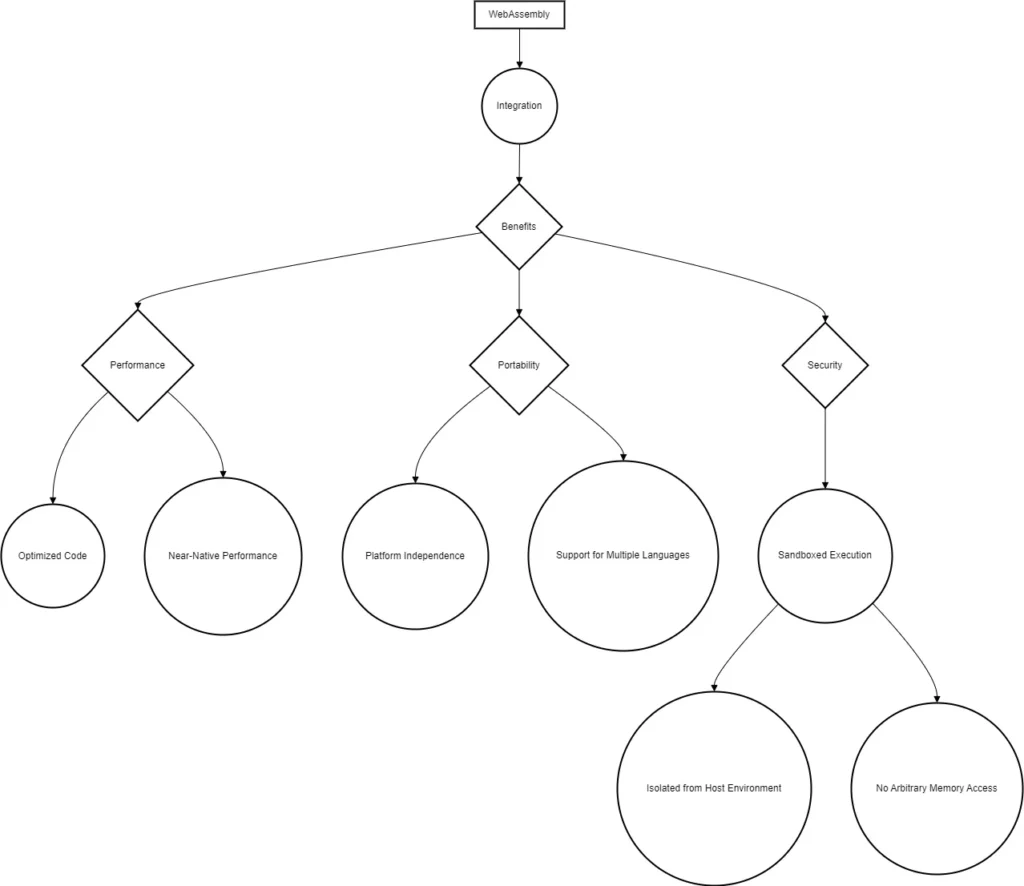
Web Assembly modules can be loaded and used in all major frontend frameworks:
// Vue.js example
import { createApp } from 'vue';
import { loadWasmModule } from './wasm-loader';
const app = createApp({
data() {
return {
fibResult: 0
}
},
async mounted() {
const wasmModule = await loadWasmModule();
this.fibResult = wasmModule.fibonacci(10);
}
});
app.mount('#app');
Server-Side Integration
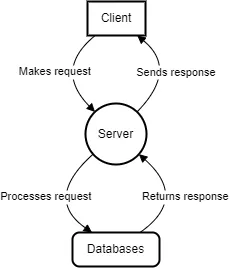
Web Assembly with Node.js
Node.js supports Wasm, allowing for efficient server-side execution:
const fs = require('fs');
const wasmBuffer = fs.readFileSync('fibonacci.wasm');
WebAssembly.instantiate(wasmBuffer).then(wasmModule => {
const fibonacci = wasmModule.instance.exports.fibonacci;
console.log(fibonacci(10));
});
Microservices Architecture with Wasm
Wasm can be used to create lightweight, fast-starting microservices, especially beneficial in serverless environments.
Tools and Libraries for Web Assembly
Popular Web Assembly Compilers
- Emscripten: For C/C++
- AssemblyScript: TypeScript-like syntax to Wasm
- Rust with wasm-pack: First-class Wasm support in Rust
Development Tools and Debugging
- Web Assembly Studio: Online IDE for Wasm development
- Chrome DevTools: Built-in support for debugging Wasm
- wasmtime: Standalone Web Assembly runtime
Challenges and Limitations
While powerful, WebAssembly does face some challenges:
- Browser Compatibility: Older browsers may lack full support
- Debugging Complexity: Debugging Wasm can be more challenging than JavaScript
- Memory Management: Manual memory management required in languages like C/C++
Future Trends and Developments
WebAssembly continues to evolve:
- Garbage Collection Proposal: Will enable languages with managed memory
- Threading: Improved support for multi-threaded applications
- WASI: Expanding WebAssembly beyond the browser
Conclusion
WebAssembly is revolutionizing full-stack development by bringing near-native performance to the web. Its ability to work seamlessly with JavaScript while offering the benefits of compiled languages makes it a powerful tool for modern web applications.
As the ecosystem matures and more developers adopt WebAssembly, we can expect to see increasingly sophisticated and performant web applications. The future of web development is here, and it’s powered by WebAssembly.
Frequently Asked Questions (FAQ)
1. What is WebAssembly, and why is it important for full-stack development?
WebAssembly (Wasm) is a low-level, binary instruction format designed for efficient execution in web browsers. It’s important for full-stack development because it:
- Enables near-native performance for web applications
- Allows developers to use languages other than JavaScript for client-side code
- Enhances the capabilities of web applications, especially for computationally intensive tasks
2. How does WebAssembly improve performance compared to JavaScript?
WebAssembly offers significant performance improvements over JavaScript:
- Faster execution: Wasm runs at near-native speed, often 10-100 times faster than JavaScript for certain tasks.
- Smaller file sizes: Wasm binaries are typically more compact than equivalent JavaScript code.
- Consistent performance: Wasm performance is more predictable across different browsers and devices.
Example: In a study by Mozilla, a C++ image processing algorithm compiled to WebAssembly ran 20 times faster than the same algorithm implemented in JavaScript.
3. Can WebAssembly be used with all programming languages?
While WebAssembly doesn’t support all languages directly, many popular languages can be compiled to Wasm:
- Directly supported: C, C++, Rust
- Supported with tools: Python, C#, Go, Java
- Specialized languages: AssemblyScript (TypeScript-like syntax for WebAssembly)
To compile other languages to WebAssembly, you typically need a specialized compiler or toolchain, such as Emscripten for C/C++ or Blazor for C#.
4. What are the common tools and libraries for working with WebAssembly?
Popular tools and libraries include:
- Emscripten: Compiles C/C++ to WebAssembly
- Rust and wasm-pack: For developing Wasm modules in Rust
- AssemblyScript: TypeScript-like language for WebAssembly
- WebAssembly Studio: Online IDE for Wasm development
- wasmtime: Runtime for WebAssembly on the server-side
Choose tools based on your preferred language, project requirements, and target environment (browser or server-side).
5. Are there any limitations or challenges when using WebAssembly?
Some limitations and challenges include:
- Limited DOM access: Wasm can’t directly manipulate the DOM, requiring JavaScript interop.
- Debugging complexity: Debugging Wasm can be more challenging than JavaScript.
- Learning curve: Developers need to learn new tools and potentially new languages.
- Browser support: While major browsers support Wasm, older browsers may not.
6. How can I start integrating WebAssembly into my existing projects?
To integrate WebAssembly into existing projects:
- Choose a language and toolchain (e.g., Rust with wasm-pack)
- Write performance-critical parts in your chosen language
- Compile the code to WebAssembly
- Use JavaScript to load and interact with the Wasm module
- Gradually replace or enhance existing JavaScript functionality with Wasm modules
Example (using Rust and wasm-pack):
// Rust code (lib.rs)
#[wasm_bindgen]
pub fn fibonacci(n: u32) -> u32 {
if n <= 1 {
return n;
}
fibonacci(n - 1) + fibonacci(n - 2)
}
// JavaScript code
import init, { fibonacci } from './pkg/my_wasm_module.js';
async function run() {
await init();
console.log(fibonacci(10)); // Use the Wasm function
}
run();
This FAQ provides a solid starting point for understanding WebAssembly in the context of full-stack development. Let me know if you need any clarification or additional information on any of these points.