Introduction
In today’s data-driven world, web developers face an increasing challenge: processing large volumes of information efficiently without compromising user experience. Enter the concept of “big gulps” in JavaScript—a game-changer for handling massive datasets in web applications. This comprehensive guide will explore the techniques and strategies that make big gulps a powerful tool in your development arsenal.
1. Introduction to Big Gulps in JavaScript
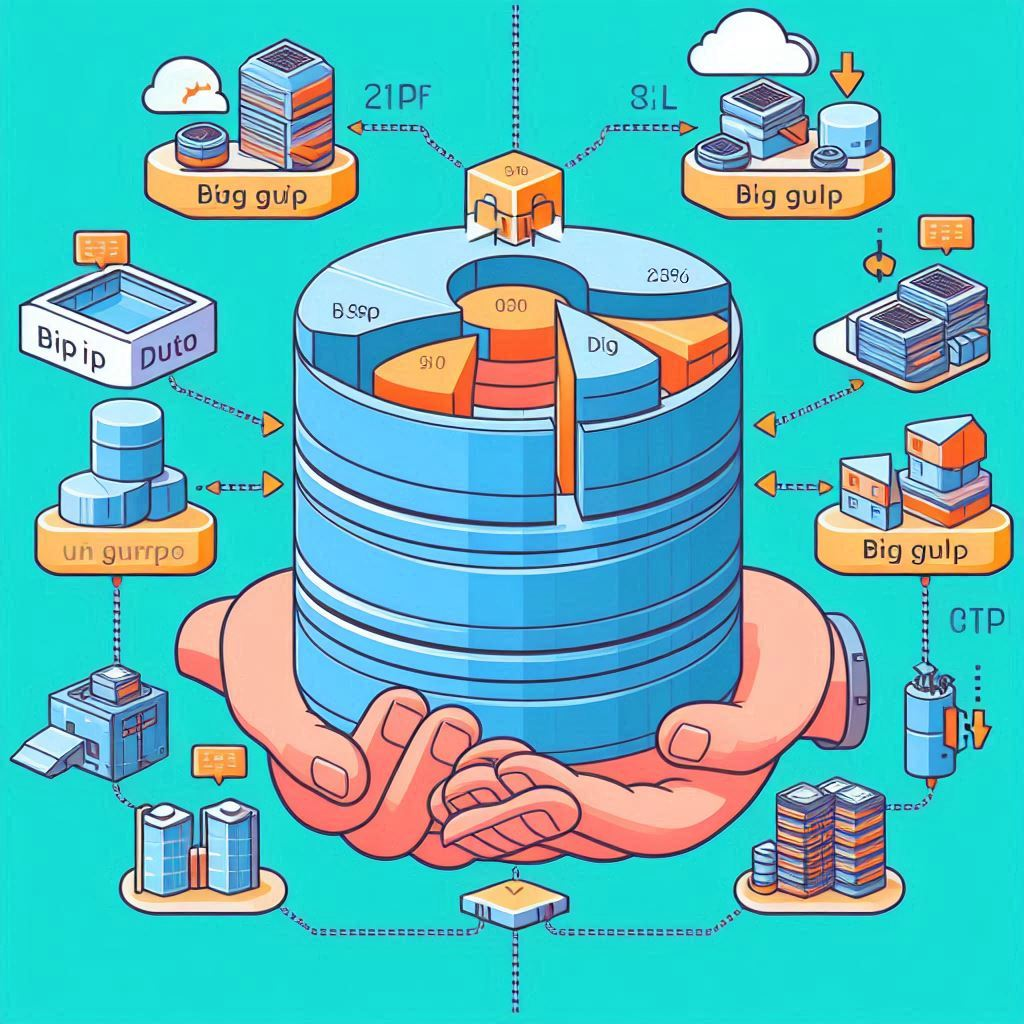
What are big gulps in data processing?
Big gulps, in the context of data processing, refer to the technique of handling large amounts of data in manageable chunks or streams. This approach allows developers to process data efficiently without overwhelming system resources or causing the dreaded “script timeout” errors.
“Big gulps are to data processing what sips are to drinking—a way to consume large amounts in a controlled, manageable fashion.”
Importance of efficient data handling in modern web development
As web applications grow in complexity and scale, the ability to handle large datasets becomes crucial. Efficient data processing impacts:
- Application performance
- User experience
- Server load and costs
- Scalability of web services
Overview of techniques and strategies
Throughout this article, we’ll explore various strategies for implementing big gulps in JavaScript, including:
- Chunking data
- Utilizing Web Workers
- Leveraging async/await for batch processing
- Implementing streams
- Using specialized libraries
Each technique offers unique advantages, and understanding when to apply them is key to mastering big gulp processing.
2. Understanding JavaScript Event Loop and Asynchronous Processing
Before diving into specific big gulp techniques, it’s crucial to understand the foundation of JavaScript’s execution model: the event loop and asynchronous processing.
Explanation of JavaScript event loop
The JavaScript event loop is the mechanism that allows JavaScript to perform non-blocking operations despite being single-threaded. It works by continuously checking the message queue and executing tasks when the call stack is empty.
console.log('Start');
setTimeout(() => {
console.log('Timeout');
}, 0);
Promise.resolve().then(() => {
console.log('Promise');
});
console.log('End');
// Output:
// Start
// End
// Promise
// Timeout
This example demonstrates how the event loop prioritizes tasks, executing synchronous code first, then microtasks (like Promises), and finally macrotasks (like setTimeout).
Synchronous vs asynchronous processing
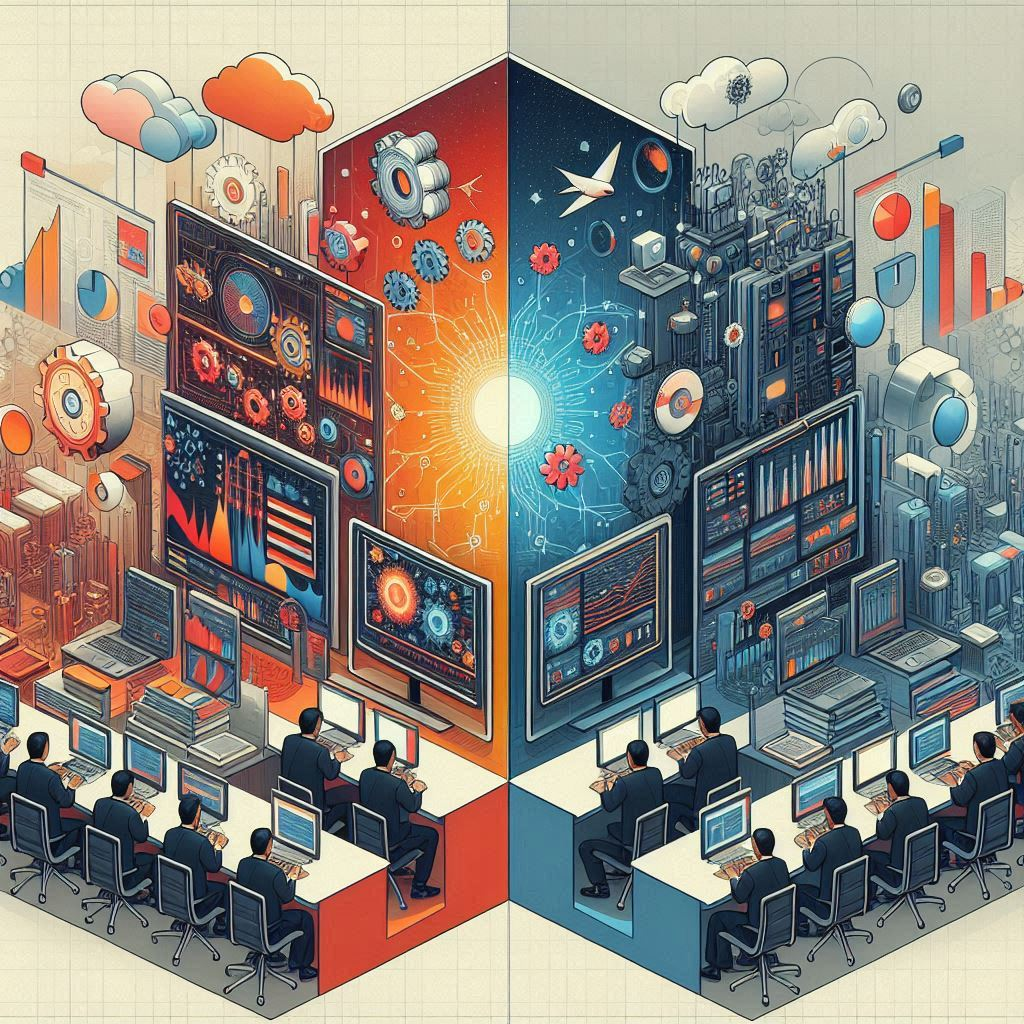
- Synchronous processing: Tasks are executed sequentially, blocking further execution until each task completes.
- Asynchronous processing: Tasks are initiated and the program continues to run, with results handled later via callbacks, promises, or async/await.
How big gulps fit into the event loop
Big gulp techniques leverage asynchronous processing to handle large datasets without blocking the main thread. By breaking data processing into smaller, manageable tasks, we can utilize the event loop efficiently, ensuring responsive applications even when dealing with massive amounts of data.
3. Chunking Data for Efficient Processing
Chunking is a fundamental big gulp technique that involves breaking large datasets into smaller, more manageable pieces.
Why process data in chunks?
Processing data in chunks offers several benefits:
- Prevents browser freezing or unresponsiveness
- Allows for progress updates during long-running tasks
- Facilitates memory management by processing subsets of data
Techniques for chunking large datasets
- Array slicing: Divide arrays into smaller subarrays.
- Pagination: Process data in pages, especially useful for API requests.
- Streaming: Process data as it arrives, piece by piece.
Implementing chunking with examples
Let’s look at a simple example of processing an array in chunks:
function processInChunks(array, chunkSize, processFn) {
let index = 0;
function nextChunk() {
const chunk = array.slice(index, index + chunkSize);
index += chunkSize;
processFn(chunk);
if (index < array.length) {
setTimeout(nextChunk, 0);
} else {
console.log('Processing complete');
}
}
nextChunk();
}
// Usage
const largeArray = Array.from({ length: 1000000 }, (_, i) => i);
processInChunks(largeArray, 1000, (chunk) => {
console.log(`Processing chunk of ${chunk.length} items`);
// Perform operations on the chunk
});
This example processes a large array in chunks of 1000 items, using setTimeout
to yield control back to the event loop between chunks.
4. Using Web Workers for Parallel Processing
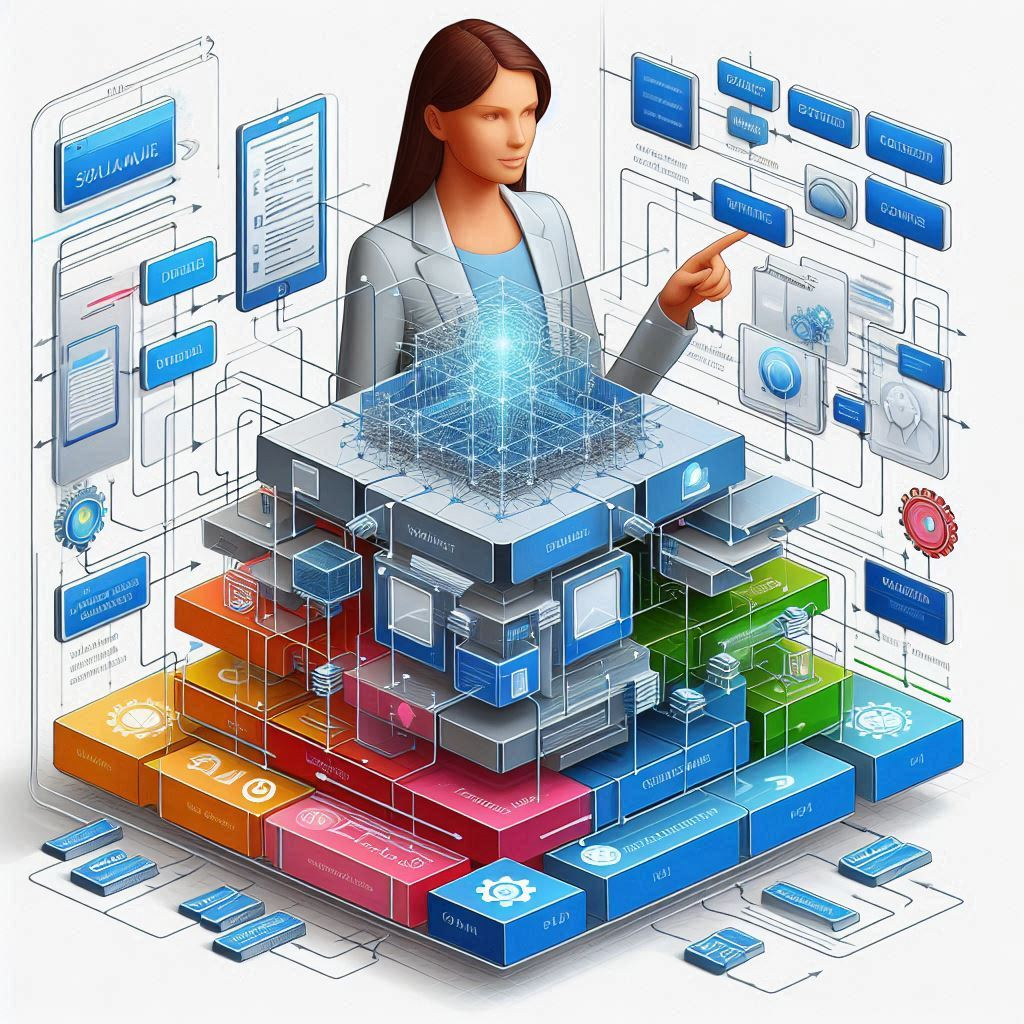
Web Workers provide a powerful way to perform heavy computations without affecting the main thread’s performance.
Introduction to Web Workers
Web Workers allow you to run scripts in background threads, separate from the main execution thread of a web application. This enables true parallel processing in JavaScript, which is especially useful for CPU-intensive tasks.
Setting up Web Workers in JavaScript
Here’s a basic setup for a Web Worker:
// main.js
const worker = new Worker('worker.js');
worker.onmessage = function(event) {
console.log('Received from worker:', event.data);
};
worker.postMessage({ data: largeArray });
// worker.js
self.onmessage = function(event) {
const result = processData(event.data.data);
self.postMessage(result);
};
function processData(data) {
// Perform heavy computations here
return processedData;
}
Example of parallel data processing using Web Workers
Let’s expand on our chunking example to utilize Web Workers:
// main.js
const largeArray = Array.from({ length: 1000000 }, (_, i) => i);
const chunkSize = 100000;
const numWorkers = navigator.hardwareConcurrency || 4;
let completedWorkers = 0;
const results = [];
for (let i = 0; i < numWorkers; i++) {
const worker = new Worker('worker.js');
const start = i * chunkSize;
const end = start + chunkSize;
worker.onmessage = function(event) {
results.push(event.data);
completedWorkers++;
if (completedWorkers === numWorkers) {
console.log('All workers completed');
// Combine results here
}
};
worker.postMessage({ data: largeArray.slice(start, end) });
}
// worker.js
self.onmessage = function(event) {
const result = processChunk(event.data.data);
self.postMessage(result);
};
function processChunk(chunk) {
// Process the chunk here
return processedChunk;
}
This example distributes the processing of a large array across multiple Web Workers, taking advantage of parallel processing capabilities.
5. Batch Processing with Async/Await
Async/await provides a clean and intuitive way to handle asynchronous operations, making it ideal for implementing batch processing in big gulp scenarios.
Using async/await for batch processing
Async/await allows you to write asynchronous code that looks and behaves like synchronous code, making it easier to reason about and maintain.
Handling large datasets with async/await
Here’s an example of how to use async/await for batch processing:
async function processBatches(data, batchSize) {
const batches = [];
for (let i = 0; i < data.length; i += batchSize) {
batches.push(data.slice(i, i + batchSize));
}
const results = [];
for (const batch of batches) {
const result = await processBatch(batch);
results.push(result);
// You could update progress here
}
return results;
}
async function processBatch(batch) {
// Simulating an async operation
return new Promise(resolve => {
setTimeout(() => {
resolve(batch.map(item => item * 2));
}, 100);
});
}
// Usage
const largeArray = Array.from({ length: 100000 }, (_, i) => i);
processBatches(largeArray, 1000).then(results => {
console.log('All batches processed:', results.flat().length);
});
Examples and best practices
When using async/await for batch processing:
- Use
Promise.all()
for concurrent batch processing when order doesn’t matter. - Implement proper error handling with try/catch blocks.
- Consider using a semaphore to limit concurrency and prevent overwhelming system resources.
6. Optimizing Data Processing with Streams
Streams provide a powerful way to handle data flow, especially when dealing with large datasets or real-time data.
What are streams in JavaScript?
Streams are objects that let you read data from a source or write data to a destination in continuous fashion. They can be especially useful when working with large amounts of data or when you want to process data before it has finished loading.
Creating readable and writable streams
Here’s a basic example of creating and using streams:
const { Readable, Writable } = require('stream');
// Create a readable stream
const readableStream = new Readable({
read() {}
});
// Create a writable stream
const writableStream = new Writable({
write(chunk, encoding, callback) {
console.log(chunk.toString());
callback();
}
});
// Pipe the readable stream to the writable stream
readableStream.pipe(writableStream);
// Push data to the readable stream
readableStream.push('Hello, ');
readableStream.push('Streams!');
readableStream.push(null); // Signal the end of the stream
Processing data with streams and piping
Streams can be particularly powerful when combined with piping and transform streams:
const { Transform } = require('stream');
const upperCaseTransform = new Transform({
transform(chunk, encoding, callback) {
this.push(chunk.toString().toUpperCase());
callback();
}
});
readableStream
.pipe(upperCaseTransform)
.pipe(writableStream);
This example demonstrates how to use a transform stream to modify data as it flows from a readable stream to a writable stream.
7. Leveraging Libraries and Tools for Big Data Processing
While native JavaScript provides powerful tools for data processing, specialized libraries can offer additional functionality and optimizations.
Overview of popular libraries (e.g., RxJS, Lodash)
- RxJS: A library for reactive programming using Observables, making it easier to compose asynchronous or callback-based code.
- Lodash: A modern JavaScript utility library delivering modularity, performance & extras, particularly useful for data manipulation.
Using RxJS for reactive programming
RxJS can be particularly useful for handling streams of data:
import { from } from 'rxjs';
import { map, filter, take } from 'rxjs/operators';
const numbers$ = from([1, 2, 3, 4, 5, 6, 7, 8, 9, 10]);
numbers$.pipe(
filter(n => n % 2 === 0),
map(n => n * 10),
take(3)
).subscribe(
value => console.log(value),
err => console.error(err),
() => console.log('Complete')
);
// Output:
// 20
// 40
// 60
// Complete
This example demonstrates filtering, mapping, and limiting a stream of data using RxJS operators.
Examples of data manipulation with Lodash
Lodash provides many utilities for working with arrays, objects, and functions:
import _ from 'lodash';
const users = [
{ 'user': 'barney', 'age': 36, 'active': true },
{ 'user': 'fred', 'age': 40, 'active': false },
{ 'user': 'pebbles', 'age': 1, 'active': true }
];
const result = _.chain(users)
.filter('active')
.sortBy('age')
.map(user => `${user.user} is ${user.age}`)
.value();
console.log(result);
// Output: ['pebbles is 1', 'barney is 36']
This example shows how Lodash can simplify complex data manipulations with its chaining syntax.
8. Memory Management and Garbage Collection
Effective memory management is crucial when dealing with large datasets to prevent memory leaks and ensure optimal performance.
Understanding JavaScript memory management
JavaScript uses automatic memory management, known as garbage collection. However, understanding how it works can help you write more efficient code:
- Allocation: JavaScript automatically allocates memory when objects are created.
- Use: Memory is used when you read or write from allocated memory.
- Release: The garbage collector automatically finds and releases memory that’s no longer being used.
Techniques to optimize memory usage
- Avoid global variables: They’re never garbage collected during the lifetime of the page.
- Close over only what you need: In closures, only include the variables you actually need.
- Use object pooling for frequently created objects: Reuse objects instead of creating new ones.
Avoiding common memory leaks in big data processing
- Forgotten timers and callbacks: Always clear timers and remove event listeners when they’re no longer needed.
- Closures: Be cautious with closures that reference large objects.
- Out of DOM references: Remove references to DOM elements when they’re removed from the document.
function processLargeData(data) {
let result = '';
// Bad: Keeps entire 'data' in memory
// const process = () => {
// result += data.toString();
// };
// Good: Only keeps necessary data in memory
const process = (item) => {
result += item.toString();
};
data.forEach(process);
return result;
}
9. Case Studies and Real-World Examples
Let’s explore some practical applications of big gulp techniques in real-world scenarios.
Practical applications of big gulp techniques
- Real-time data visualization: Using streams and chunking to process and display large datasets in real-time without freezing the UI.
- Large file uploads: Implementing chunked file uploads to handle large files efficiently.
- Data analytics dashboards: Utilizing Web Workers to perform complex calculations on large datasets without impacting the main thread.
Success stories from industry
Case Study: E-commerce Product Catalog
A large e-commerce platform implemented big gulp techniques to handle their product catalog of over 1 million items. By using a combination of chunking and Web Workers, they were able to:
- Reduce page load time by 60%
- Improve search functionality response time by 75%
- Handle real-time inventory updates without impacting user experience
Lessons learned and best practices
- Start small: Begin with simpler techniques like chunking before moving to more complex solutions.
- Monitor performance: Regularly profile your application to identify bottlenecks.
- Balance between memory usage and processing speed: Sometimes trading memory for speed (or vice versa) can lead to overall better performance.
- Consider the end-user experience: Always prioritize responsiveness and smooth interactions.
10. Conclusion
Recap of key points
Throughout this article, we’ve explored various techniques for handling large datasets efficiently in JavaScript:
- Chunking data for manageable processing
- Utilizing Web Workers for parallel processing
- Leveraging async/await for batch processing
- Optimizing with streams
- Using specialized libraries like RxJS and Lodash
- Managing memory effectively
Encouraging experimentation with big gulp techniques
The world of big data processing in JavaScript is vast and ever-evolving. Don’t be afraid to experiment with different techniques and find what works best for your specific use case.
Future trends in JavaScript data processing
As web applications continue to grow in complexity and scale, we can expect to see:
- More native support for parallel processing in browsers
- Enhanced APIs for efficient data handling
- Increased focus on real-time data processing and visualization
- Greater integration of machine learning and AI in client-side data processing
By mastering big gulp techniques, you’ll be well-prepared to tackle the data processing challenges of today and tomorrow.
11. FAQ
Q: What’s the difference between chunking and streaming?
A: Chunking involves processing data in predetermined segments, while streaming processes data as it becomes available, often in real-time.
Q: How do I choose between using Web Workers and async/await?
A: Use Web Workers for CPU-intensive tasks that can run independently. Use async/await for I/O-bound tasks or when you need to maintain a specific execution order.
Q: Are there any performance considerations when using libraries like RxJS or Lodash?
A: While these libraries offer powerful functionality, they can add to your bundle size. Always consider the trade-off between added functionality and performance impact. Use tree-shaking and import only the functions you need to minimize overhead.
Q: How can I handle errors when processing large datasets?
A: Implement robust error handling within your processing functions. For batch processing, consider implementing retry mechanisms. When using streams, handle errors in the ‘error’ event. Always have a fallback plan for critical operations.
Q: Is it possible to use big gulp techniques with frameworks like React or Vue?
A: Absolutely. These techniques can be integrated into any JavaScript application, including those built with modern frameworks. For example, you might use Web Workers to offload heavy computations from your React components, or implement virtual scrolling with chunked data loading in a Vue application.
Q: How do big gulp techniques impact SEO?
A: While most big gulp techniques focus on client-side processing, they can indirectly impact SEO by improving page load times and overall site performance, which are factors in search engine rankings. For content that needs to be indexed, consider server-side rendering or generating static content where possible.
Q: Can big gulp techniques be used in Node.js backend applications?
A: Yes, many of these techniques translate well to Node.js environments. Streams, in particular, are a core feature of Node.js and are excellent for processing large amounts of data efficiently on the server side.
Q: How do I profile my application to identify where big gulp techniques might be beneficial?
A: Use browser developer tools to profile your application’s performance. Look for long-running scripts, high memory usage, or frequent garbage collection as indicators that you might benefit from big gulp techniques. Tools like Chrome’s Performance and Memory tabs are invaluable for this kind of analysis.
Q: Are there any security concerns with these techniques, especially when dealing with sensitive data?
A: When processing sensitive data, be cautious with techniques that might expose data in unexpected ways. For example, be careful not to expose sensitive information to Web Workers if they’re not necessary. Always sanitize and validate data, especially when dealing with streams or chunk processing.
Q: How do big gulp techniques work with TypeScript?
A: TypeScript fully supports all the JavaScript features used in big gulp techniques. In fact, TypeScript can enhance these techniques by providing type safety and better tooling support, making it easier to catch errors and refactor code.
By addressing these frequently asked questions, we’ve covered additional aspects of big gulp techniques in JavaScript. Remember that the key to mastering these techniques is practice and continuous learning. As you implement these strategies in your projects, you’ll develop a deeper understanding of when and how to apply each technique for optimal results.
The field of efficient data processing in JavaScript is continually evolving, with new tools and techniques emerging regularly. Stay curious, keep experimenting, and don’t hesitate to push the boundaries of what’s possible in web development. With the power of big gulp techniques at your disposal, you’re well-equipped to handle the data processing challenges of modern web applications.