React, a powerful JavaScript library, is widely used for building user interfaces, especially in web and mobile apps. React developers often face a choice between two types of components: class components and functional components. Each type offers distinct advantages and is suitable for different use cases. Understanding the differences and capabilities of each component type is crucial for optimizing performance and maintainability of applications. This blog aims to dissect class components and functional components, providing clear insights on when to use each based on their characteristics and the advantages they offer.
Class Components in React
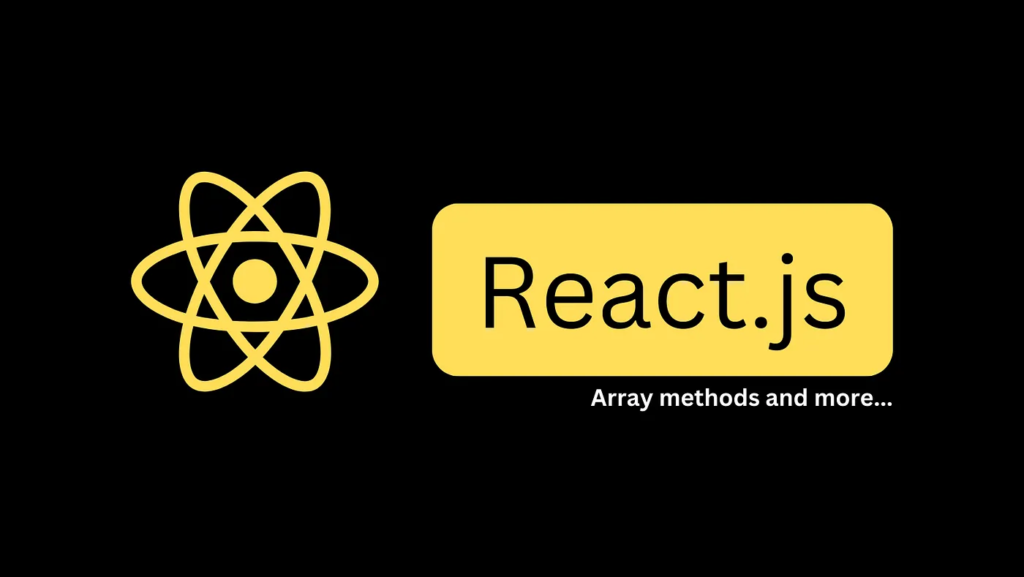
Definition and Characteristics
Class components in React are JavaScript classes that extend React.Component. This type of component is more complex than functional components and provides more features, making it suitable for larger, stateful applications. Class components allow the use of lifecycle methods such as componentDidMount, componentDidUpdate , and componentWillUnmount, which are essential for performing actions at specific points in the lifecycle of a component. They have a render() method that returns the JSX to be rendered to the DOM. Initially, class components were the only way to use state and lifecycle methods in React, which made them indispensable for state management and side effects.
Advantages of Using Class Components
Its offer several benefits, particularly in scenarios requiring state management and lifecycle control. Here are some key advantages:
- Stateful logic: Class components make it straightforward to handle complex state logic, which can be more cumbersome with functional components without hooks.
- Lifecycle methods: They provide access to lifecycle methods, allowing developers to execute code at specific times in the component’s lifecycle, which is critical for tasks like fetching data, registering/unregistering event listeners, or managing timers.
- Readability: For projects initially built with React, class components can be easier to read and manage, as they clearly separate logic, lifecycle methods, and rendering.
- Suitability for large-scale applications: The structured nature of class components makes them well-suited for large applications with complex states and behaviors that require full use of React capabilities.
Understanding these components and their particular strengths is essential for making informed decisions on which to use in your projects.
Functional Components in React
Definition and Characteristics of Functional Components
Functional components in React are JavaScript functions that accept props as an argument and return React elements. They represented a simpler and more concise way to build components prior to hooks, primarily focusing on presenting UIs without involving any lifecycle methods or state handling complexities usually associated with class components. Unlike class components, functional components are stateless by nature, but the introduction of hooks provided them with capabilities to manage state and side effects.
Advantages of Using Functional Components
Functional components provide several advantages that make them attractive for developers:
- Simplicity: They are straightforward to write and understand, making the codebase easier to maintain.
- Performance: Less overhead in comparison to class components as they don’t have the lifecycle methods that might cause additional rendering.
- Reusable Code: Encourages the creation of smaller, reusable components, promoting better organization and modularity.
- Improved Code Testing: Simplicity in structure makes functional components easier to test.
Introducing React Hooks for Functional At Components
React hooks, introduced in version 16.8, allow functional components to use state and lifecycle features similar to what was traditionally only possible in class components. These hooks include:
- useState: For adding local state to the component.
- useEffect: For performing side effects in the component.
- useContext: For accessing React context in the component.
Hooks offer a more powerful and flexible way to build components, combining the simplicity of functional components with the comprehensive capabilities typically associated with classes.
When to Use Class Components
Scenarios where it are Preferred
While functional components are generally more used after the introduction of hooks, class components still hold specific advantages in complex scenarios:
- State Management in Large-scale Applications: When handling more intricate and multi-level state logic.
- Complex Component Behavior: Where lifecycle management is more intricate, offer more control.
Example Use Cases
Some practical examples of situations where class components might be preferable include:
- Error Boundaries: They are currently the only way to catch JavaScript errors anywhere in a child component tree using lifecycle methods like componentDidCatch.
- Managing Complex Transitions and Animations: Using lifecycle methods to manipulate DOM directly for handling complex animations.
- Intensive Computational Tasks: Where components require fine-tuning or optimization during lifecycle events.
These scenarios underscore the existing relevance, especially in applications where nuanced handling of component lifecycles is crucial.
When to Utilize Functional Components
Functional components in React are a streamlined way to write components without the need for the more verbose syntax of class components. This category of components becomes particularly advantageous when paired with React hooks, which allow functional components to easily manage state, perform side effects, and tap into React features that were previously only possible with class components.
Scenarios where Functional Components with Hooks are Preferred
Functional components are best used in the following scenarios:
– State Management with Simplicity: When the component logic involves managing state in a straightforward manner, React hooks (such as useState and useContext() provide a much cleaner and more concise way to handle state compared to the class-based approach.
– Side Effects: If your component needs to perform side effects (like fetching data, timers, manually changing the DOM, etc.), hooks like useEffect make these operations much more intuitive and organized.
– Component Reusability: When building components that are intended to be reused across different parts of an application, functional components can help in creating cleaner and more modular code.
– Rapid Development & Maintenance: For projects that require quick iterations and updates, functional components allow developers to write less code and achieve the same functionality, which simplifies debugging and testing.
Example Use Cases
Functional components shine in various common situations, such as:
– Building a simple input form: Utilizing useState to handle input states and useEffect for handling component focus or validation.
– Creating a user dashboard: Where useContext can manage global state like user info or theme preferences, making it accessible throughout the component tree without prop drilling.
Comparison: Class Components vs. Functional Components
The ongoing evolution in React’s development has been marked by a notable shift from class components to functional components, especially after the introduction of hooks. Understanding the core differences can be pivotal in deciding which to use for your project requirements.
Performance Differences
It’s a common misconception that functional components inherently offer better performance than class components. While functional components may result in less boiler code, the actual performance gains depend on specific use cases and implementation details. React.memo can be used with functional components to prevent unnecessary re-renders by memoizing the output, whereas shouldComponentUpdate serves a similar purpose in class components.
Scalability Factors
Scalability in large applications is a critical consideration. Functional components, when combined with hooks, provide a more scalable architecture due to easier component isolation, testing, and maintenance. They encourage smaller, function-based components that are easier to test and modify. Class components, however, can become bulky as they grow, making them harder to manage in large-scale applications. The modularity of functional components typically leads to better organized and maintainable codebase in extensive projects.
Understanding these aspects helps in choosing the right component architecture for your application’s scalability needs and performance optimization.
Best Practices for Using Class or Functional Components
Guidelines for Choosing Between Components
When deciding whether to implement any components within your React application, the nature of the component and the specific use case are decisive factors. Here are some guidelines to help you make the best choice:
– State Management: Use functional components with hooks like useState() and useEffect() for simpler state management. Class components might be necessary if you are working with complex state logic that involves multiple sub-values or when the state is heavily dependent on the previous state.
– Lifecycle Methods: If you need to perform actions at specific points in a component’s lifecycle, class components provide lifecycle methods like componentDidMount, componentShouldUpdate, and componentWillUnmount. However, the introduction of hooks like useEffect allows functional components to effectively handle lifecycle events in a more concise manner.
– Reusability: Functional components, combined with hooks, offer better reusability and composition than class components. Custom hooks can encapsulate logic that might be shared across multiple components, which is less straightforward with class component methods.
– Side Effects: For operations like data fetching, setting up subscriptions, or timers, useEffect in functional components is preferable due to its simplicity and clarity compared to lifecycle methods in class components.
Tips for Optimizing Component Performance
To ensure your React components, whether class or functional, perform optimally, consider the following strategies:
– Memoization: Use React.memo for functional components to prevent unnecessary re-renders by memoizing the output. Similarly, shouldComponentUpdate lifecycle method or PureComponent can serve this purpose in class components.
– Code Splitting: Implement code splitting in your React app to load components only when needed. This can be done using React.lazy for functional components or similar approaches in class components.
– Optimized Rendering: Minimize the number of re-renders by keeping the state as local as possible and lifting it up only when necessary. Use useCallback and useMemo hooks in functional components to optimize performance.
Conclusion
Understanding when to use components in React can greatly impact the effectiveness and efficiency of your application. While functional components are generally preferred for their simplicity and the power of React hooks, class components still have their place in scenarios requiring detailed lifecycle management or complex state logic. By following the best practices and optimization tips outlined above, developers can ensure that their applications are not only functional but also perform at their best, leading to enhanced user experiences and more maintainabled codebases. Always consider the specific needs of your project and the potential for future maintenance when making your choice between class and functional components.
Pingback: Lifecycle Methods in Class Components: A Beginner's Guide
Pingback: Exploring the Exciting New Hooks in React v19
Pingback: JavaScript parseFloat() Function
portable balancing machine
Portable Balancing Machine: Balanset-1A Overview
The Balanset-1A is a state-of-the-art portable balancing machine designed for dynamic balancing and vibration analysis suitable for various applications across multiple industries. This versatile tool efficiently addresses the balancing needs of rotors such as crushers, fans, mulchers, augers on combines, shafts, centrifuges, turbines, and similar machinery. Whether for maintenance or production purposes, the Balanset-1A provides essential functionality that enhances operational efficiency and reliability.
Key Features of the Balanset-1A
The Balanset-1A features an advanced dual-channel system that allows for precise and effective balancing in two planes. This capability proves essential when dealing with complex rotors, enabling technicians to achieve accurate results without the need for extensive downtime. With this portable balancing machine, users can expect the following primary features:
Vibrometer Mode: Measures vibration parameters for a comprehensive analysis.
Tachometer: Accurately captures rotational speed (RPM).
Phase Analysis: Determines the phase angle of vibration signals, offering insights into operational conditions.
Overall Vibration Monitoring: Keeps track of vibration levels to ensure operational safety.
FFT Spectrum Analysis: Provides detailed frequency spectrum insights for analysis.
Measurement Logs: Saves data for future reference and scrutiny.
Balancing Capabilities
With its advanced balancing capabilities, the Balanset-1A allows users to perform both single and two-plane balancing which is key to reducing vibration and improving machine longevity. Some notable functionalities include:
Single Plane Balancing: Focuses on balancing rotors in one plane to reduce vibration.
Two Plane Balancing: Offers comprehensive dynamic balancing across two planes, crucial for complex industrial applications.
Polar Graph Visualization: Creates a polar graph to indicate where weights should be placed for effective balancing.
Tolerance Calculator: Computes permissible balancing tolerances according to ISO 1940 standards.
Additional Functionalities
The Balanset-1A is not just limited to balancing; it also incorporates various functionalities that make it an efficient tool for vibration analysis:
Graphical Representations: Users can view charts that depict overall vibration patterns, harmonic frequencies, and frequency spectrum analysis.
Reports and Archive Features: Generates detailed reports and archives past balancing sessions for convenient data retrieval.
Compatibility and Ease of Use: Supports both Imperial and Metric systems, making it user-friendly for global applications.
Specifications
In terms of technical specifications, the Balanset-1A includes crucial components to ensure accurate and efficient performance:
Two vibration sensors (Vibro Accelerometers) with optional cable lengths for flexible usage.
An optical sensor (Laser Tachometer) for precise measurements.
A USB interface module to facilitate easy software connectivity for data analysis.
Measurement capabilities covering a wide range of vibration velocity and frequency metrics.
Importance of Portable Balancing Machines
The significance of portable balancing machines, such as the Balanset-1A, cannot be overstated in modern industrial contexts. The increasing reliance on machinery in various sectors necessitates effective maintenance strategies that ensure optimal performance. A portable balancing machine allows technicians to perform on-site assessments, salvaging time and resources otherwise spent on transporting equipment. This efficiency not only extends the lifespan of the machinery but also minimizes the risk of unplanned downtimes.
In industries where sensitivity to vibrations is vital, the Balanset-1A stands out with its ability to deliver precise and real-time balancing assessments. By adhering to ISO standards, users can confidently manage balancing operations, ensuring that equipment runs smoothly and efficiently.
Applications Across Industries
The portability and versatility of the Balanset-1A enable its use across various sectors including manufacturing, agriculture, and energy. In manufacturing, it can be used for the dynamic balancing of rotating components during production to reduce wear and enhance product quality. In agricultural applications, the tool helps in optimizing the performance of equipment like combines and augers, critical for operational productivity. The energy sector benefits by ensuring turbines and centrifuges operate smoothly, thereby enhancing energy efficiency.
Conclusion
Overall, the Balanset-1A portable balancing machine is an indispensable tool for industries that rely on the performance of rotating equipment. Its comprehensive features, coupled with ease of use and portability, ensure that users can conduct balancing operations effectively without interrupting production processes. The ongoing need for reliable maintenance solutions in today’s competitive landscape makes the Balanset-1A a valuable investment for companies looking to enhance their operational efficiency and machinery longevity.
Watch YouTube Short
Balanset-1A: Advanced Balancing Equipment for Precision Engineering
When it comes to ensuring the smooth operation of technical mechanisms, proper balancing is key. The Balanset-1A rotor balancing device is designed to provide accurate and efficient balancing solutions for a wide range of machinery.
Before initiating the balancing process, it is essential to ensure that the mechanism is in optimal working condition, securely mounted in its designated position. The rotor must be cleaned of any contaminants that may interfere with the balancing procedure.
Prior to taking measurements, it is important to select appropriate installation locations and position vibration and phase sensors according to the recommended guidelines.
For efficient balancing, it is advisable to conduct measurements using a vibrometer mode. By analyzing the vibration components, one can determine if rotor imbalance is the primary contributor to the overall vibration of the mechanism.
When conducting rotor balancing, it is crucial to consider both static and dynamic balancing aspects. The Balanset-1A device offers the versatility of balancing rotors in one or two correction planes, depending on the structural characteristics of the rotor being balanced.
The Balanset-1A device boasts a range of features that set it apart as a top-tier balancing equipment:
Compactness and Portability: The Balanset-1A is designed for convenience and mobility, making it a perfect companion for on-site and field work.
Intuitive Software: The device connects to a laptop, offering a user-friendly interface with step-by-step instructions for setup and balancing.
Multi-functionality: Combining vibrometer and balancing functions, the device provides comprehensive insights into machinery vibration and balance correction.
High Measurement Accuracy: With precision up to В±1В° for phase measurements and В±5% for vibration indicators, the device meets stringent standards for accuracy.
Customizable Options: The device offers various settings to tailor it to specific tasks, ensuring flexibility and adaptability.
User-Friendly Operation: The simplified program interface makes it easy for users of all levels to operate the device effectively.
Support for Serial Balancing: Ideal for mass production environments, the device facilitates efficient data storage and retrieval for repetitive balancing tasks.
Quality Assurance and Reliability: Backed by a one-year warranty and technical support, users can rely on the device for consistent performance.
Cost-Effectiveness and Accessibility: Offering a balance of affordability and quality, the Balanset-1A is a valuable investment for enhancing product quality.
Equipped with vibration transducers, phase angle sensors, measuring blocks, magnetic stands, electronic scales, and comprehensive software, the Balanset-1A kit is priced at 1751 euros, making it a competitive choice in the market.
Contact Information:
For more information about our Balanset balancing devices and other products, please visit our website: https://vibromera.eu.
Subscribe to our YouTube channel, where you will find instructional videos and examples of completed work: https://www.youtube.com/@vibromera.
Stay updated with our latest news and promotions on Instagram, where we also showcase examples of our work: https://www.instagram.com/vibromera_ou/.
Buy Balanset-1A on Etsy
Balanset-1A OEM on Machinio