Introduction
In the fast-paced world of web development, performance optimization is key to creating smooth, responsive user experiences. One powerful technique that every JavaScript developer should master is debouncing. This blog post will dive deep into the concept of debouncing, exploring its importance, implementation, and best practices in 2024.
What is Debouncing?
It is a programming practice used to ensure that time-consuming tasks do not fire so often, making it particularly useful for performance optimization. It limits the rate at which a function gets called.
Importance of Debouncing in JavaScript
As web applications become more complex and interactive, the need for efficient event handling grows. its helps in:
- Reducing unnecessary function calls
- Improving application performance
- Enhancing user experience
- Optimizing resource usage
Overview of the Blog
This comprehensive guide will cover everything from the basics of debouncing to advanced techniques and real-world applications. Whether you’re a beginner or an experienced developer, you’ll find valuable insights to level up your JavaScript skills.
Understanding the Concept of Debouncing
Definition and Explanation
Debouncing is a technique that limits the rate at which a function gets called. It ensures that a function is only executed after a certain amount of time has passed since its last invocation.
“Think of debouncing as a way to add a pause between events, allowing your code to catch its breath before responding.”
Debouncing vs Throttling
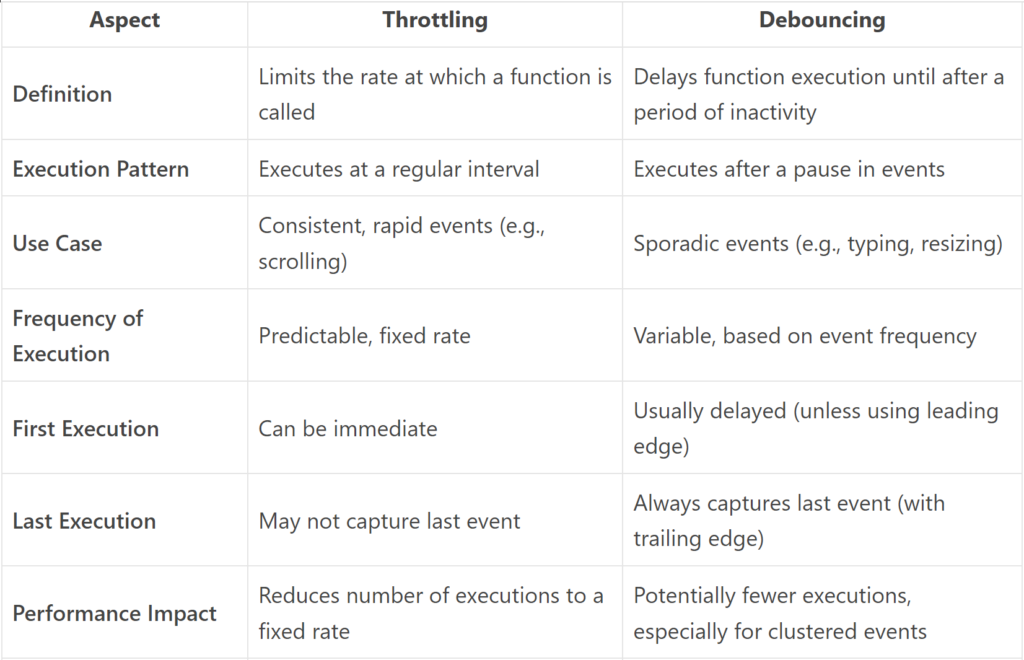
While both debouncing and throttling are used for performance optimization, they work differently:
- Debouncing: Delays executing a function until after a certain amount of time has passed since the last time it was invoked.
- Throttling: Limits the number of times a function can be called over time.
Real-world Examples Where Debouncing is Crucial
- Search Input: Preventing API calls on every keystroke
- Window Resizing: Avoiding excessive calculations during browser resizing
- Scroll Events: Optimizing performance for infinite scrolling
- Button Clicks: Preventing accidental double submissions
How Debouncing Works
Basic Principles
Debouncing works on a simple principle: delay the execution of a function until a pause in the triggering event occurs. This is achieved by:
- Setting a timer when the event first occurs
- Clearing the timer if the event occurs again before it expires
- Finally executing the function when the timer completes without interruption
Visual Explanation
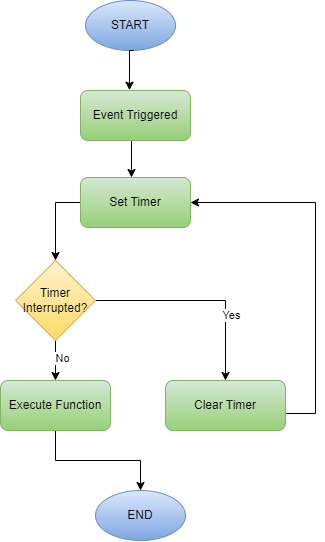
Common Use Cases
- Search Input:
const debouncedSearch = debounce(searchAPI, 300);
searchInput.addEventListener('input', debouncedSearch);
- Window Resizing:
const debouncedResize = debounce(handleResize, 250);
window.addEventListener('resize', debouncedResize);
- Scroll Events:
const debouncedScroll = debounce(updateScrollPosition, 100);
window.addEventListener('scroll', debouncedScroll);
Implementing Debouncing in JavaScript
Simple Debounce Function
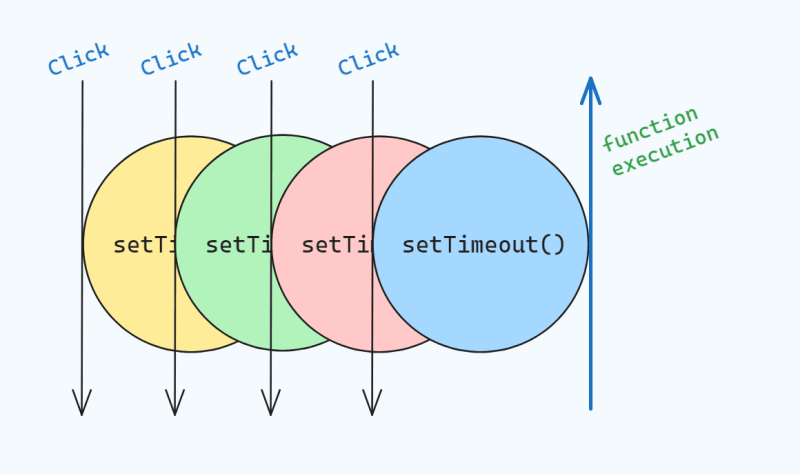
Here’s a basic implementation of a debounce function:
function debounce(func, delay) {
let timeoutId;
return function (...args) {
clearTimeout(timeoutId);
timeoutId = setTimeout(() => {
func.apply(this, args);
}, delay);
};
}
Step-by-Step Code Explanation
- The
debounce
function takes two parameters: the function to be debounced (func
) and the delay time (delay
). - It returns a new function that wraps the original function.
- When the returned function is called, it clears any existing timeout.
- It then sets a new timeout that will call the original function after the specified delay.
- If the function is called again before the timeout expires, the process repeats, effectively “debouncing” the function call.
Code Snippets and Examples
Let’s look at a practical example of debouncing a search function:
function searchAPI(query) {
console.log(`Searching for: ${query}`);
// API call logic here
}
const debouncedSearch = debounce(searchAPI, 300);
// In your event listener
searchInput.addEventListener('input', (e) => {
debouncedSearch(e.target.value);
});
In this example, the API call will only be made 300ms after the user stops typing, significantly reducing the number of unnecessary API calls.
Advanced Debouncing Techniques
Debouncing with Leading and Trailing Options
Sometimes you might want to execute the function immediately on the first call (leading edge) or ensure it’s always called at least once (trailing edge). Here’s an enhanced debounce function that supports these options:
function advancedDebounce(func, delay, { leading = false, trailing = true } = {}) {
let timeoutId;
let lastCallTime = 0;
return function (...args) {
const now = Date.now();
const later = () => {
lastCallTime = leading ? 0 : now;
timeoutId = null;
if (trailing) func.apply(this, args);
};
if (!timeoutId && leading) {
func.apply(this, args);
}
clearTimeout(timeoutId);
if (leading && now - lastCallTime > delay) {
func.apply(this, args);
}
lastCallTime = now;
timeoutId = setTimeout(later, delay);
};
}
Optimizing Performance
To further optimize debouncing, consider:
- Using
requestAnimationFrame
for visual updates - Implementing a queue system for high-frequency events
- Adjusting the delay based on device performance
Handling Edge Cases
Be aware of potential edge cases:
- Rapid successive calls: Ensure your debounce function can handle rapid, repeated invocations.
- Memory leaks: Properly clear timeouts to prevent memory leaks, especially in long-running applications.
- Context and arguments: Make sure to correctly pass the context and arguments to the debounced function.
Debouncing in Popular JavaScript Libraries
Using Lodash for Debouncing
Lodash, a popular utility library, provides a robust debounce function:
import _ from 'lodash';
const debouncedFunc = _.debounce(myFunction, 300, {
leading: true,
trailing: true,
});
Integrating Debounce in React
In React applications, you can use debouncing to optimize performance in functional components:
import React, { useState, useCallback } from 'react';
import { debounce } from 'lodash';
function SearchComponent() {
const [searchTerm, setSearchTerm] = useState('');
const debouncedSearch = useCallback(
debounce((term) => {
// Perform search operation
console.log(`Searching for: ${term}`);
}, 300),
[]
);
const handleInputChange = (e) => {
const value = e.target.value;
setSearchTerm(value);
debouncedSearch(value);
};
return (
<input
type="text"
value={searchTerm}
onChange={handleInputChange}
placeholder="Search..."
/>
);
}
Practical Examples with Code
- Debounced API Calls:
const debouncedFetch = _.debounce(async (query) => {
const response = await fetch(`https://api.example.com/search?q=${query}`);
const data = await response.json();
updateResults(data);
}, 300);
- Debounced Form Validation:
const debouncedValidate = _.debounce((value) => {
if (value.length < 3) {
setError('Input must be at least 3 characters');
} else {
setError(null);
}
}, 200);
Testing and Debugging Debounced Functions
Tools and Techniques for Testing
- Jest for Unit Testing: Use Jest’s timer mocks to test debounced functions.
- Chrome DevTools: Utilize the Performance tab to visualize function calls.
- Console Logging: Strategically place logs to track function execution.
Common Pitfalls and How to Avoid Them
- Incorrect
this
binding: Use arrow functions orbind
to maintain the correct context. - Overusing debouncing: Apply debouncing judiciously; not every function needs it.
- Ignoring return values: Remember that debounced functions return
undefined
.
Example Test Cases
describe('debounce function', () => {
jest.useFakeTimers();
test('debounces function calls', () => {
const func = jest.fn();
const debouncedFunc = debounce(func, 1000);
debouncedFunc();
debouncedFunc();
debouncedFunc();
expect(func).not.toBeCalled();
jest.runAllTimers();
expect(func).toBeCalledTimes(1);
});
});
Best Practices for Using Debouncing
When to Use and When to Avoid
Use debouncing when:
- Handling high-frequency events (e.g., scrolling, resizing)
- Preventing excessive API calls
- Optimizing expensive computations
Avoid debouncing when:
- Immediate response is crucial (e.g., game controls)
- Dealing with critical user inputs
- Working with low-frequency events
Performance Considerations
- Choose appropriate delay: Balance responsiveness and performance.
- Monitor CPU usage: Use browser dev tools to ensure debouncing is effective.
- Consider device capabilities: Adjust debounce times for different devices.
Tips for Efficient Debouncing
- Use a higher-order function to create reusable debounce logic.
- Implement cancelation mechanisms for long-running debounced operations.
- Combine debouncing with other optimization techniques like memoization.
FAQs on Debouncing
What is the difference between Debouncing and Throttling?
Debouncing delays function execution until after a period of inactivity, while throttling limits the number of function calls over time. Debouncing is ideal for sporadic events, while throttling suits consistent, rapid events.
Can Debouncing Improve UX?
Yes, debouncing can significantly improve UX by reducing lag, preventing unnecessary API calls, and ensuring smooth interactions, especially in input-heavy interfaces.
How to Choose the Right Debounce Interval?
The ideal debounce interval depends on your specific use case. For typing, 300-500ms is often suitable. For resizing or scrolling, you might use a shorter interval like 100-200ms. Always test and adjust based on user experience.
What are the Common Mistakes in Implementing Debouncing?
- Not clearing the timeout properly
- Ignoring the function’s context (
this
) - Overusing debouncing where it’s not necessary
- Choosing inappropriate delay times
Conclusion
Recap of Key Points
- Debouncing is a crucial technique for optimizing JavaScript performance.
- It’s particularly useful for handling high-frequency events and API calls.
- Proper implementation requires understanding of closures and timers.
- Advanced techniques like leading/trailing options can enhance functionality.
- Testing and debugging are essential for ensuring correct behavior.
Final Thoughts
Mastering debouncing is an invaluable skill for any JavaScript developer in 2024. By applying the techniques and best practices outlined in this guide, you can significantly improve the performance and user experience of your web applications. Remember, the key to effective debouncing lies in understanding your specific use case and finding the right balance between responsiveness and efficiency.
Further Reading and Resources
- MDN Web Docs: Debouncing
- Lodash Documentation: debounce
- React Hooks and Debounce
- Performance Optimization Techniques
- Advanced JavaScript Concepts
By implementing debouncing techniques and following the best practices outlined in this guide, you’ll be well-equipped to create high-performance JavaScript applications that provide smooth, responsive user experiences. Happy coding!