Table of Contents
Are you gearing up for a MERN stack interview? Whether you’re a seasoned developer or just starting your journey in the world of full-stack development, this comprehensive guide will equip you with the knowledge and strategies you need to excel in your upcoming interview. From mastering technical skills to honing your soft skills, we’ll cover everything you need to know to make a lasting impression on your potential employers.
Introduction
The MERN stack has become increasingly popular in recent years, and for good reason. This powerful combination of technologies allows developers to build scalable, efficient, and dynamic web applications. As the demand for MERN stack developers continues to grow, it’s crucial to be well-prepared for interviews in this field.
Importance of Preparation for MERN Stack Interviews
Thorough preparation is the key to success in any interview, but it’s especially important when it comes to MERN stack positions. These interviews often involve a mix of technical questions, coding challenges, and behavioral assessments. By investing time in preparation, you’ll not only boost your confidence but also demonstrate your dedication and passion for the field to potential employers.
Overview of MERN Stack (MongoDB, Express.js, React, Node.js)
Before we dive into the specifics of interview preparation, let’s briefly review what the MERN stack entails:
- MongoDB: A NoSQL database that uses JSON-like documents for data storage
- Express.js: A web application framework for Node.js that simplifies the process of building robust web applications
- React: A JavaScript library for building user interfaces, particularly single-page applications
- Node.js: A JavaScript runtime built on Chrome’s V8 JavaScript engine, allowing you to run JavaScript on the server-side
Now that we’ve covered the basics, let’s explore each area of preparation in detail.
Section 1: Understanding the Basics
Brief Overview of Each Technology in MERN Stack
To excel in a MERN stack interview, you need to have a solid understanding of each component:
- MongoDB:
- Document-oriented database
- Flexible schema design
- Scalability and high performance
- Express.js:
- Minimal and flexible Node.js web application framework
- Robust set of features for web and mobile applications
- Simplifies the process of writing server-side code
- React:
- Component-based architecture
- Virtual DOM for efficient rendering
- Unidirectional data flow
- Node.js:
- Event-driven, non-blocking I/O model
- Large ecosystem of open-source libraries
- Ability to use JavaScript for both frontend and backend development
How They Work Together
Understanding how these technologies interact is crucial for any MERN stack developer. Here’s a high-level overview of how the MERN stack components work together:
- The client sends a request to the Node.js server.
- Express.js handles the routing and middleware.
- If data is needed, Node.js communicates with MongoDB to retrieve or store information.
- The server sends the response back to the client.
- React renders the user interface based on the received data.
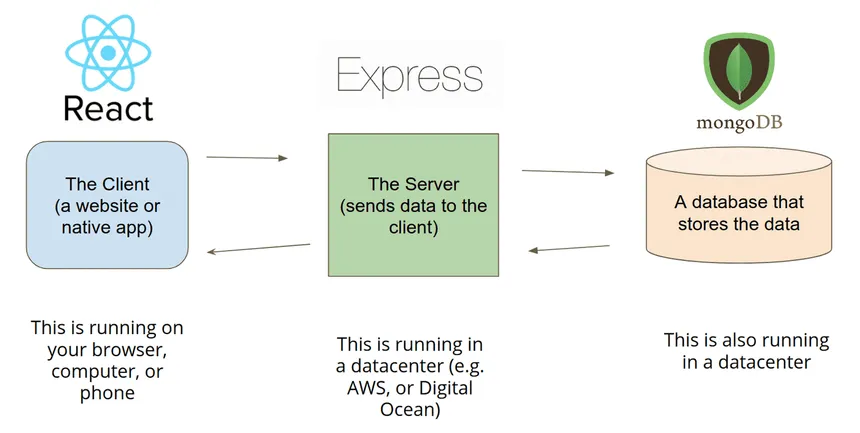
By grasping these fundamental concepts, you’ll be better equipped to answer questions about the MERN stack architecture and demonstrate your understanding of how these technologies complement each other.
Section 2: Technical Skills to Master
To ace your MERN stack interview, you’ll need to have a strong command of various technical skills. Let’s break down the key areas you should focus on:
JavaScript Fundamentals
As the foundation of the MERN stack, a deep understanding of JavaScript is essential. Make sure you’re comfortable with:
- ES6+ features (arrow functions, destructuring, promises, async/await)
- Closures and scope
- Prototypal inheritance
- Event loop and asynchronous programming
- Functional programming concepts
Node.js Basics and Advanced Concepts
Node.js is the backbone of server-side development in the MERN stack. Be prepared to discuss:
- Core modules (fs, http, path)
- Event emitters
- Streams and buffers
- Error handling and debugging
- Package management with npm
- Performance optimization techniques
Express.js Setup and Middleware
Express.js simplifies the process of building web applications. Focus on:
- Setting up an Express.js application
- Routing and middleware concepts
- Error handling middleware
- Authentication and authorization
- RESTful API design principles
MongoDB CRUD Operations
Understanding how to interact with MongoDB is crucial. Be ready to demonstrate your knowledge of:
- CRUD operations (Create, Read, Update, Delete)
- Querying documents
- Indexing and aggregation
- Data modeling best practices
- Handling relationships between documents
React Component Lifecycle and State Management
React is at the heart of frontend development in the MERN stack. Make sure you’re well-versed in:
- Component lifecycle methods
- Hooks (useState, useEffect, useContext, etc.)
- State management (local state, Redux, Context API)
- Performance optimization techniques
- React Router for navigation
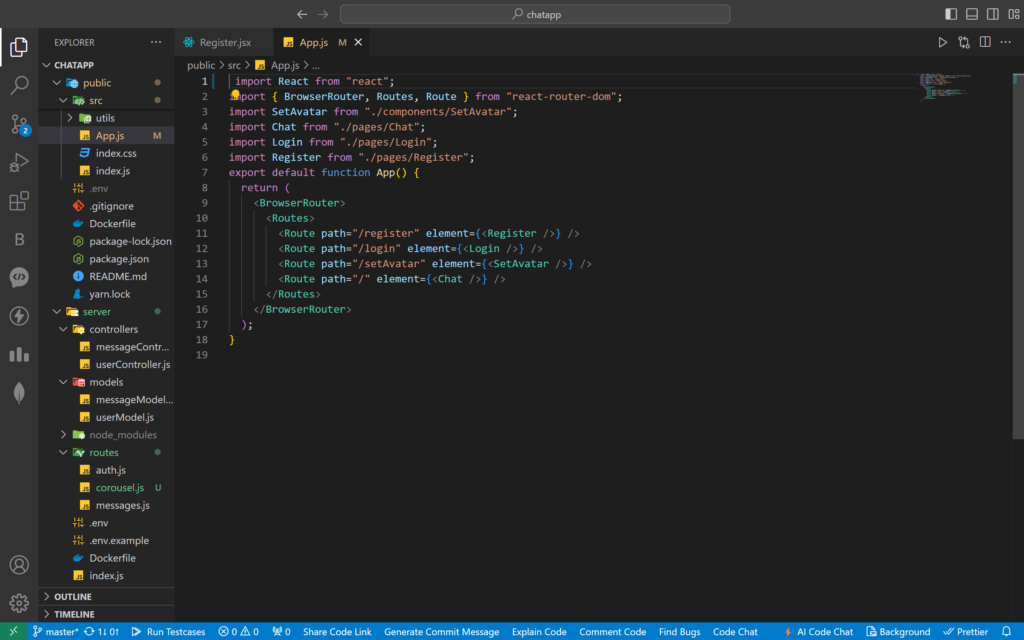
By mastering these technical skills, you’ll be well-prepared to tackle a wide range of questions and challenges during your MERN stack interview.
Section 3: Common Interview Questions
Being familiar with common interview questions will help you feel more confident and prepared. Here are some sample questions for each technology, along with coding challenges and behavioral questions you might encounter:
Sample Questions for Each Technology
MongoDB:
- What are the key differences between MongoDB and relational databases?
- Explain the concept of sharding in MongoDB.
- How do you optimize query performance in MongoDB?
Express.js:
- What is middleware in Express.js, and how is it used?
- Explain the difference between
app.use()
andapp.get()
. - How do you handle errors in an Express.js application?
React:
- Explain the virtual DOM and its benefits.
- What are the differences between controlled and uncontrolled components?
- How do you optimize performance in a React application?
Node.js:
- What is the event loop in Node.js, and how does it work?
- Explain the concept of streams in Node.js.
- How do you handle asynchronous operations in Node.js?
Coding Challenges and Algorithm Questions
Be prepared to face coding challenges that test your problem-solving skills and algorithmic thinking. Some common types of challenges include:
- Implementing data structures (e.g., linked lists, trees, graphs)
- Solving algorithmic problems (e.g., sorting, searching, dynamic programming)
- Debugging and optimizing existing code
Here’s an example of a coding challenge you might encounter:
// Problem: Implement a function to find the longest palindromic substring in a given string
function longestPalindrome(s) {
// Your implementation here
}
// Test cases
console.log(longestPalindrome("babad")); // Expected output: "bab" or "aba"
console.log(longestPalindrome("cbbd")); // Expected output: "bb"
Behavioral Questions
In addition to technical questions, be prepared to answer behavioral questions that assess your soft skills and cultural fit. Some examples include:
- Describe a challenging project you worked on and how you overcame obstacles.
- How do you stay updated with the latest trends and technologies in web development?
- Give an example of a time when you had to explain a complex technical concept to a non-technical team member.
Remember, when answering behavioral questions, use the STAR method (Situation, Task, Action, Result) to structure your responses effectively.
Here’s an example of a coding challenge with solution that you might encounter in a MERN stack interview:
// Problem: Implement a function that finds the longest substring without repeating characters in a given string.
// Solution:
function longestSubstringWithoutRepeats(s) {
let longest = '';
let current = '';
let charMap = {};
for (let char of s) {
if (charMap[char] !== undefined && charMap[char] >= current.length - current.length) {
// If we've seen this character before in our current substring,
// we need to start a new substring from the character after the last occurrence
current = current.slice(current.indexOf(char) + 1);
}
current += char;
charMap[char] = current.length - 1;
if (current.length > longest.length) {
longest = current;
}
}
return longest;
}
// Test cases
console.log(longestSubstringWithoutRepeats("abcabcbb")); // Expected output: "abc"
console.log(longestSubstringWithoutRepeats("bbbbb")); // Expected output: "b"
console.log(longestSubstringWithoutRepeats("pwwkew")); // Expected output: "wke"
console.log(longestSubstringWithoutRepeats("dvdf")); // Expected output: "vdf"
This solution uses a sliding window approach with a hash map to keep track of character positions. Here’s a breakdown of how it works:
- We initialize variables for the longest substring found so far (
longest
), the current substring being considered (current
), and a hash map to store character positions (charMap
). - We iterate through each character in the input string:
- If we’ve seen the character before and it’s within our current substring, we slice the current substring to start from the character after the last occurrence.
- We add the current character to our current substring.
- We update the character’s position in the hash map.
- If the current substring is longer than our longest found so far, we update the longest.
- After iterating through all characters, we return the longest substring found.
This solution has a time complexity of O(n) where n is the length of the input string, as we only traverse the string once. The space complexity is also O(n) in the worst case, where all characters are unique.
When presenting this solution in an interview, you could discuss:
- The thought process behind choosing this approach
- The trade-offs between time and space complexity
- How you might optimize this further for very large strings
- How you would handle edge cases (empty strings, strings with all repeating characters, etc.)
By familiarizing yourself with these types of questions and practicing your responses, you’ll be better equipped to handle whatever comes your way during the interview.
Section 4: Practical Projects to Showcase
Having practical experience with MERN stack projects is invaluable when it comes to impressing potential employers. Here are some project ideas to consider:
Building a Simple CRUD Application
Create a basic application that demonstrates your ability to perform CRUD operations. This could be a task manager, a blog platform, or an inventory system. Focus on:
- Implementing user authentication
- Creating, reading, updating, and deleting records
- Handling form submissions and data validation
Creating a RESTful API with Node.js and Express.js
Develop a robust API that showcases your backend skills. Consider building:
- A weather API that fetches data from external sources
- A movie database API with search and filtering capabilities
- A social media API with user profiles and post management
Developing a Frontend with React
Showcase your React skills by building a dynamic and responsive user interface. Some ideas include:
- A dashboard with real-time data visualization
- A single-page application with complex state management
- A game or interactive learning tool
Integrating MongoDB for Data Storage
Demonstrate your database skills by effectively using MongoDB in your projects. Focus on:
- Designing efficient data models
- Implementing data validation and error handling
- Optimizing queries for performance
When presenting these projects during your interview, be prepared to discuss:
- The challenges you faced and how you overcame them
- The architecture decisions you made and why
- How you ensured code quality and maintainability
- Any performance optimizations you implemented
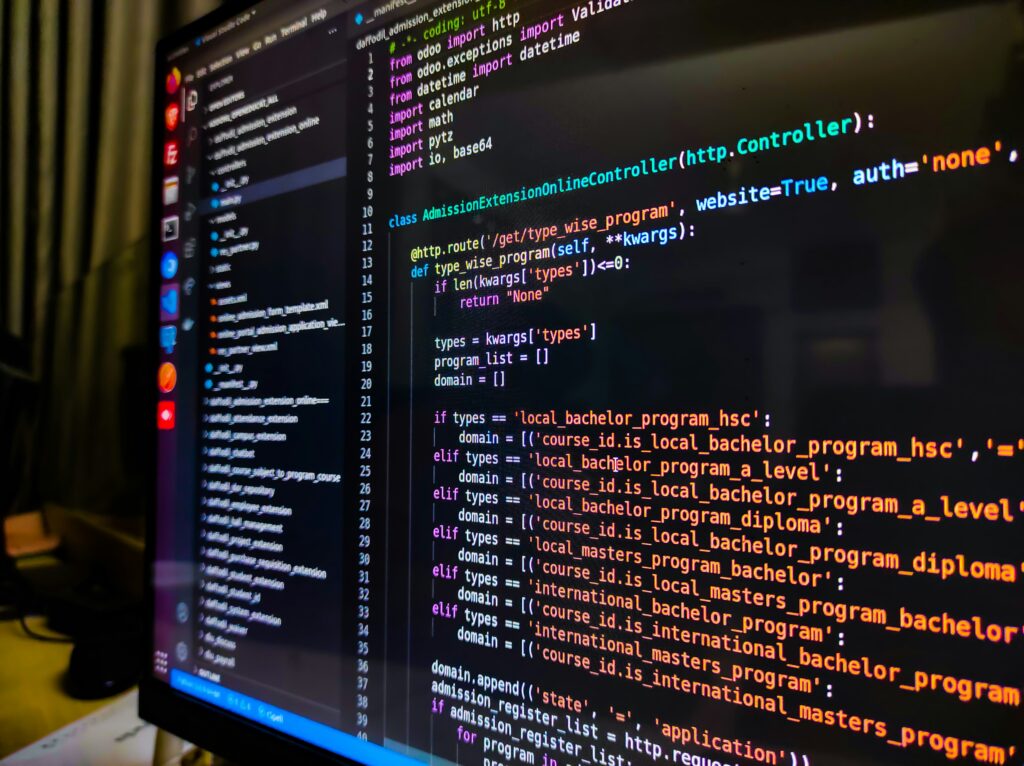
By having a portfolio of practical projects, you’ll not only gain valuable experience but also have concrete examples to discuss during your interview.
Section 5: Mock Interviews and Practice
Importance of Mock Interviews
Mock interviews are an excellent way to prepare for the real thing. They help you:
- Get comfortable with the interview format
- Identify areas where you need improvement
- Practice articulating your thoughts clearly
- Receive feedback on your performance
Resources for Practicing Mock Interviews
There are several resources available for practicing mock interviews:
- Online platforms: Websites like Pramp, InterviewBit, and LeetCode offer mock interview sessions with peers.
- Coding bootcamps: Many bootcamps offer mock interview services as part of their career support.
- Meetup groups: Look for local web development meetups that organize mock interview events.
- Friends and colleagues: Practice with people in your network who have experience in MERN stack development.
Tips for Effective Practice Sessions
To make the most of your mock interviews and practice sessions:
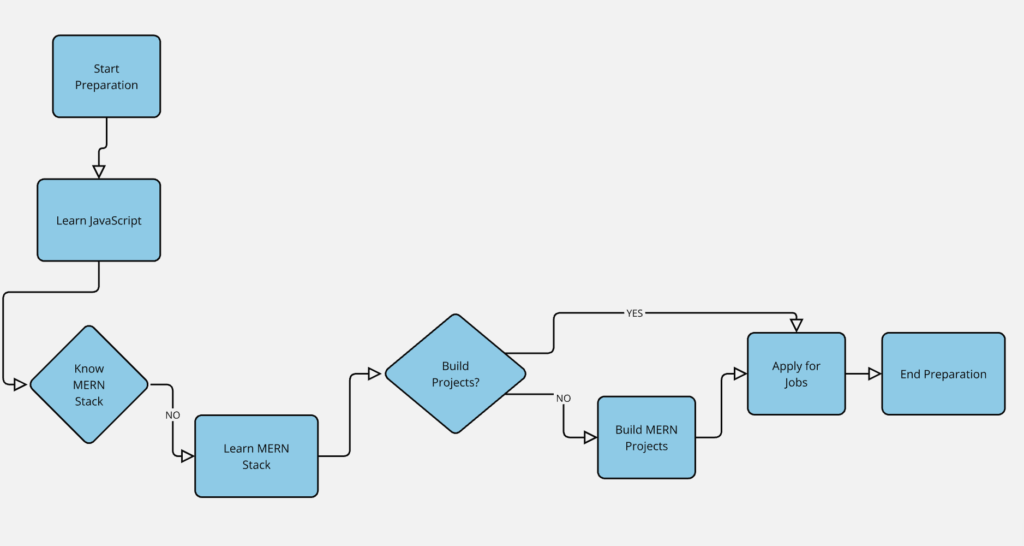
By incorporating regular mock interviews and practice sessions into your preparation routine, you’ll build confidence and improve your performance under pressure.
Section 6: Soft Skills and Behavioral Preparation
While technical skills are crucial, soft skills play a significant role in determining your success in a MERN stack position. Here are key areas to focus on:
Communication Skills
Effective communication is essential for collaborating with team members and explaining complex concepts to non-technical stakeholders. To improve your communication skills:
- Practice explaining technical concepts in simple terms
- Work on active listening and asking clarifying questions
- Develop your written communication skills through documentation and code comments
Problem-Solving Approach
Employers want to see how you approach and solve problems. Develop a structured problem-solving method:
- Understand the problem thoroughly
- Break down complex issues into smaller, manageable parts
- Consider multiple solutions and evaluate their pros and cons
- Implement the chosen solution efficiently
- Test and refine your approach
Team Collaboration Examples
Be prepared to discuss experiences where you’ve worked effectively in a team. Focus on:
- Your role in team projects
- How you handle conflicts or disagreements
- Your experience with code reviews and pair programming
- Your ability to mentor or be mentored by others
[Insert diagram of soft skills here]
By developing these soft skills alongside your technical abilities, you’ll present yourself as a well-rounded candidate who can contribute positively to any development team.
FAQ Section
Q: What are the most important topics to focus on for a MERN stack interview?
A: Focus on JavaScript fundamentals, CRUD operations with MongoDB, Express.js middleware, React state management, and building RESTful APIs. Additionally, make sure you understand how all these components work together in a MERN stack application.
Q: How can I practice coding challenges for MERN stack interviews?
A: Use platforms like LeetCode, HackerRank, and CodeSignal to practice coding challenges and algorithms. Additionally, try building small projects that incorporate different aspects of the MERN stack to gain practical experience.
Q: What projects should I build to showcase my MERN stack skills?
A: Build a CRUD application, create a RESTful API, develop a frontend with React, and integrate MongoDB for data storage. Consider creating a full-stack application that demonstrates your ability to work with all components of the MERN stack.
Q: How important are soft skills in a MERN stack interview?
A: Soft skills are crucial as they demonstrate your ability to communicate, collaborate, and solve problems effectively within a team. Employers value candidates who can work well with others and adapt to changing project requirements.
Q: How can I stay updated with the latest trends in MERN stack development?
A: Follow industry blogs, participate in online communities, attend webinars and conferences, and contribute to open-source projects. Continuously building and experimenting with new features and libraries will also help you stay current.
Conclusion
Preparing for a MERN stack interview requires dedication, practice, and a well-rounded approach. By focusing on both technical skills and soft skills, you’ll position yourself as a strong candidate for any MERN stack development role.
Recap of Key Preparation Tips
- Master the fundamentals of each technology in the MERN stack
- Build practical projects that showcase your skills
- Practice coding challenges and mock interviews regularly
- Develop your soft skills and problem-solving abilities
- Stay updated with the latest trends and best practices
Encouragement to Start Preparing Early
The earlier you start preparing, the more confident and knowledgeable you’ll be when interview day arrives. Set aside dedicated time each day or week to work on different aspects of your preparation.
Final Thoughts on Acing the MERN Stack Interview
Remember, the key to success in a MERN stack interview is not just about knowing the right answers, but also about demonstrating your passion for development, your ability to learn and adapt, and your potential to contribute to a team.
As you prepare, stay curious, embrace challenges, and never stop learning. With the right mindset and preparation, you’ll be well on your way to acing your MERN stack interview and landing your dream job.