Table of Contents
In today’s interconnected world, developing software for a global audience is no longer a luxury—it’s a necessity. Enter i18n, or internationalization, a crucial aspect of modern software development that can make or break your application’s success on the world stage. This comprehensive guide will walk you through everything you need to know about i18n, from its fundamental concepts to advanced implementation strategies.
Introduction: Why i18n Matters
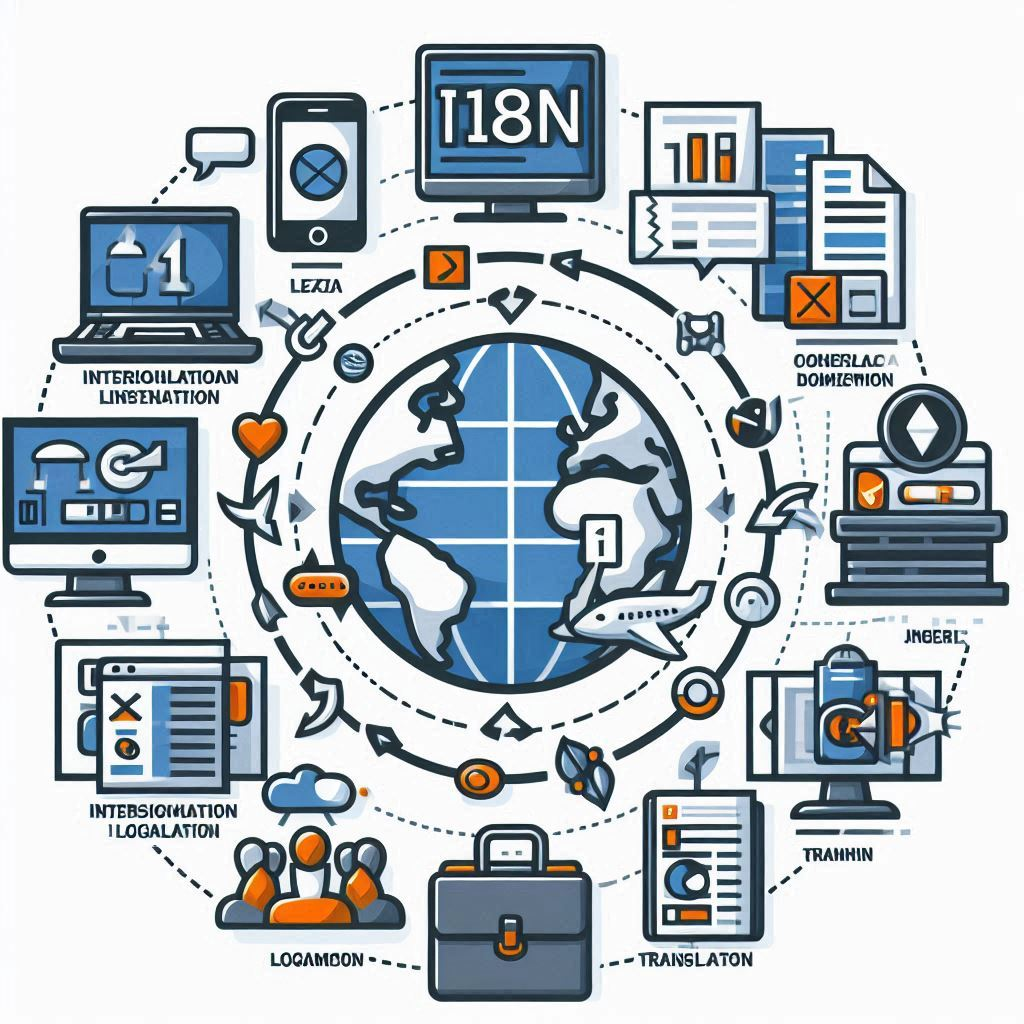
Internationalization, often abbreviated as i18n (where 18 represents the number of letters between ‘i’ and ‘n’), is the process of designing and developing software applications that can be easily adapted to various languages and regions without requiring significant engineering changes. In essence, it’s about creating a flexible foundation that allows your software to speak the language of your users, wherever they may be.
The importance of i18n in software development cannot be overstated. As businesses increasingly target global markets, the ability to cater to diverse linguistic and cultural needs becomes a critical factor in an application’s success. Consider these statistics:
- 75% of global consumers prefer to buy products in their native language (CSA Research)
- Localized websites are 1.8 times more likely to convert visitors into customers (Forrester Research)
Despite its clear benefits, i18n comes with its own set of challenges. From managing multiple language strings to handling complex cultural nuances, the road to a truly global application can be daunting. However, with the right approach and tools, these challenges can be transformed into opportunities for growth and user satisfaction.
Section 1: Understanding i18n
What is i18n?
At its core, i18n is a design philosophy and a set of practices that enable software to be adapted to various languages and regions without major code changes. It’s about creating a flexible architecture that separates language-dependent elements from the core functionality of your application.
Key Concepts and Terminology
To navigate the world of i18n effectively, it’s crucial to understand its key concepts and related terms:
- Localization (l10n): The process of adapting internationalized software for a specific region or language. This includes translating text, adjusting date and number formats, and adapting graphics and color schemes to suit local preferences.
- Globalization (g11n): A broader term that encompasses both internationalization and localization. It refers to the entire process of creating software that can operate in multiple languages and cultural contexts.
- Translation (t9n): The act of converting text from one language to another, a crucial part of the localization process.
“Internationalization is not just about translation; it’s about creating a flexible foundation that allows your software to adapt to any cultural context.” – David Filip, OASIS XLIFF Technical Committee Chair
Differences Between i18n and Localization
While often used interchangeably, i18n and localization are distinct processes:
- i18n is the preparation phase, focusing on making the software adaptable.
- Localization is the adaptation phase, where the software is tailored for specific locales.
Think of i18n as building a versatile wardrobe, while localization is choosing the right outfit for a particular occasion.
Section 2: Planning for i18n
Assessing Your Application’s Readiness for i18n
Before diving into implementation, it’s crucial to evaluate your application’s current state and readiness for internationalization. Consider the following questions:
- Are all user-facing strings externalized from the code?
- Can your application handle different date, time, and number formats?
- Is your UI flexible enough to accommodate text expansion or contraction in different languages?
- Can your database store and retrieve Unicode characters?
Identifying Target Markets and Languages
Choosing which markets to target is a critical business decision that will influence your i18n strategy. Consider factors such as:
- Market size and potential
- Existing user base and demand
- Competitor presence
- Regulatory requirements
Creating a Localization Strategy
A well-thought-out localization strategy ensures efficient use of resources and a smooth internationalization process. Key elements include:
- Prioritizing languages: Start with the most impactful languages for your business.
- Resource allocation: Determine the budget and team structure for ongoing localization efforts.
- Timeline: Set realistic deadlines for each phase of the i18n and localization process.
- Quality assurance: Establish procedures for ensuring the accuracy and cultural appropriateness of translations.
Section 3: Implementing i18n in Your Code
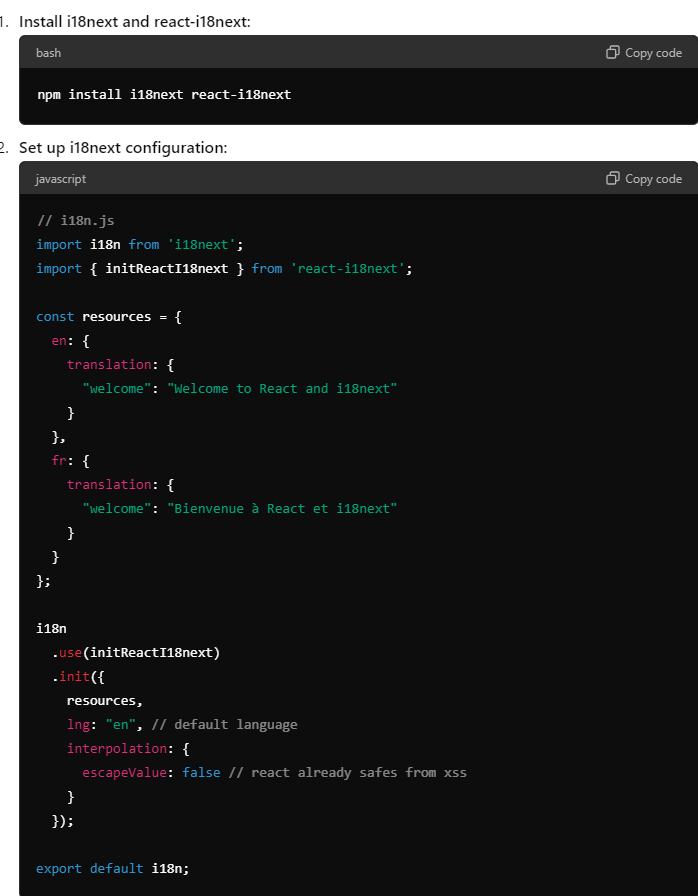
Setting Up Your Development Environment for i18n
Preparing your development environment for i18n involves selecting the right tools and frameworks. Some essential steps include:
- Choosing an i18n library compatible with your tech stack
- Setting up a workflow for managing translations
- Configuring your IDE for easy handling of internationalized code
Using i18n Libraries and Frameworks
Let’s explore how to implement i18n in popular front-end frameworks:
Example with React and i18next
import React from 'react';
import { useTranslation } from 'react-i18next';
function MyComponent() {
const { t } = useTranslation();
return (
<div>
<h1>{t('welcome.title')}</h1>
<p>{t('welcome.message')}</p>
</div>
);
}
Example with Angular and ngx-translate
import { Component } from '@angular/core';
import { TranslateService } from '@ngx-translate/core';
@Component({
selector: 'app-root',
template: `
<h1>{{ 'welcome.title' | translate }}</h1>
<p>{{ 'welcome.message' | translate }}</p>
`
})
export class AppComponent {
constructor(private translate: TranslateService) {
translate.setDefaultLang('en');
}
}
Example with Vue.js and vue-i18n
<template>
<div>
<h1>{{ $t('welcome.title') }}</h1>
<p>{{ $t('welcome.message') }}</p>
</div>
</template>
<script>
export default {
name: 'App'
}
</script>
Managing Text Strings and Translations
Effective management of text strings is crucial for maintainable i18n. Best practices include:
- Using key-based translation systems
- Organizing translations in structured JSON or YAML files
- Implementing a version control system for translation files
- Utilizing translation management tools for collaboration with translators
Section 4: Best Practices
Designing for Flexibility and Scalability
To create truly internationalized software, consider these design principles:
- Separation of concerns: Keep language-specific content separate from application logic.
- Modular design: Create components that can easily adapt to different language requirements.
- Scalable architecture: Design your system to accommodate new languages with minimal effort.
Handling Date, Time, and Number Formats
Different cultures have varying conventions for displaying dates, times, and numbers. Use language-aware formatting libraries to ensure correct display across locales:
// Using Intl.DateTimeFormat for date formatting
const date = new Date();
const formatter = new Intl.DateTimeFormat('fr-FR', {
year: 'numeric',
month: 'long',
day: 'numeric'
});
console.log(formatter.format(date)); // Output: 27 juillet 2024
Managing Pluralization and Gender
Languages have different rules for plurals and gender. Use pluralization functions and gender-aware translations to handle these complexities:
// Example using i18next for pluralization
i18next.t('key', { count: 0 }); // Output: "zero"
i18next.t('key', { count: 1 }); // Output: "one"
i18next.t('key', { count: 5 }); // Output: "many"
Ensuring Accessibility and Usability
Internationalization goes hand in hand with accessibility. Ensure your implementation doesn’t compromise accessibility by:
- Using semantic HTML
- Providing text alternatives for non-text content
- Ensuring keyboard navigation works across all localized versions
Section 5: Tools and Resources
Translation Management Tools
Streamline your translation workflow with tools like:
- PhraseApp: Offers a user-friendly interface for translators and developers.
- Transifex: Provides real-time translation updates and integrations with popular development platforms.
- Crowdin: Supports community-driven translations and offers powerful automation features.
Automated Testing
Implement automated tests to catch issues early:
- Unit tests: Verify individual components and functions handle internationalized content correctly.
- Integration tests: Ensure different parts of your application work together with various language settings.
- End-to-end tests: Simulate user interactions across different locales to catch layout or functionality issues.
Community Resources and Documentation
Stay updated with the latest practices through these resources:
- W3C Internationalization Working Group (https://www.w3.org/International/)
- Mozilla Developer Network guide (https://developer.mozilla.org/en-US/docs/Mozilla/Add-ons/WebExtensions/Internationalization)
- Unicode Consortium (https://home.unicode.org/)
Section 6: Common Pitfalls and How to Avoid Them
Overcoming Cultural Nuances and Differences
Be aware of cultural sensitivities and differences in:
- Color symbolism
- Imagery and icons
- Idiomatic expressions
“A picture is worth a thousand words, but it may say different things in different cultures.” – Anonymous
Dealing with Encoding and Character Sets
Ensure your application can handle various character sets:
- Use UTF-8 encoding throughout your application
- Test with non-Latin scripts and right-to-left languages
- Be cautious with character counting and string manipulation
Avoiding Hardcoded Text Strings
Resist the temptation to hardcode text:
- Externalize all user-facing strings
- Use translation functions even for seemingly static text
- Implement a linting rule to catch hardcoded strings
Section 7: Case Studies and Real-World Examples
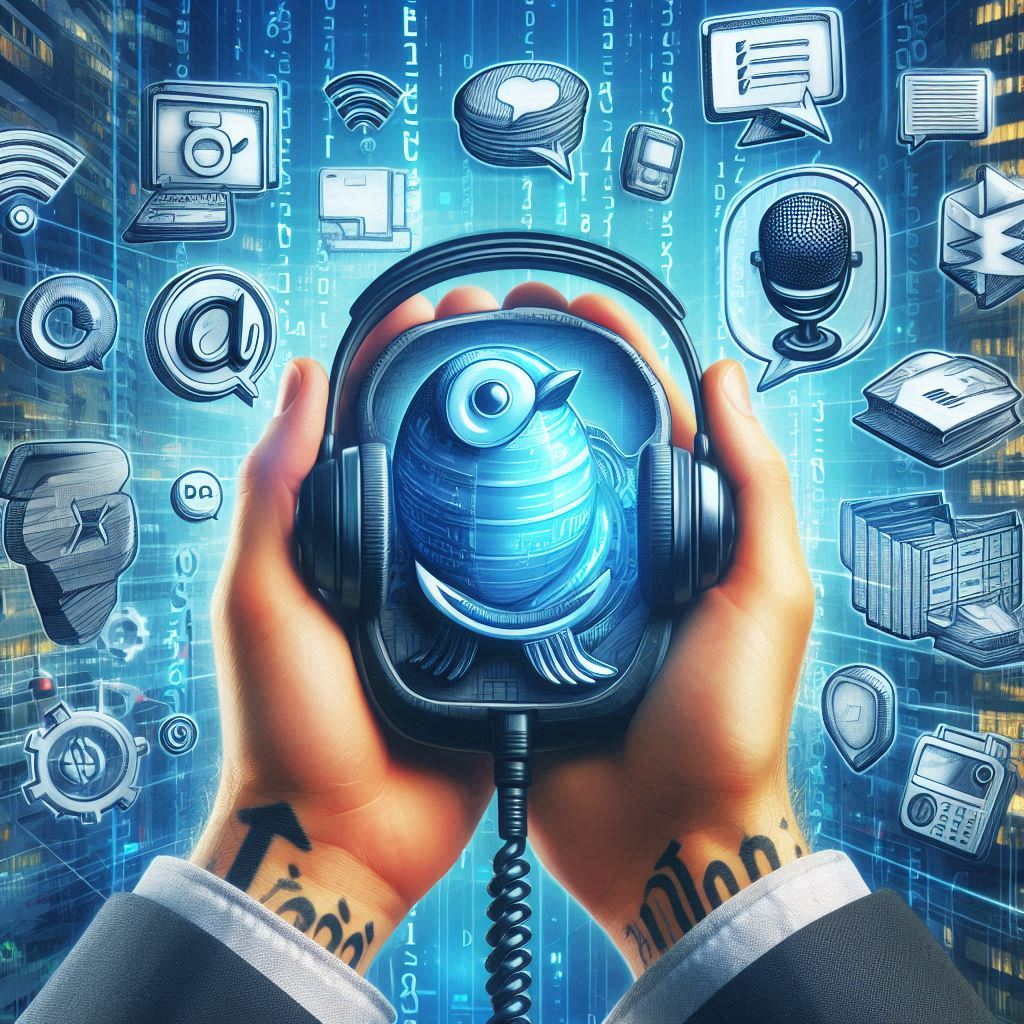
Successful Implementations in Popular Software
- Airbnb: Supports 62 languages and localizes experiences for each market.
- Spotify: Offers a seamless experience across 178 markets with localized content and playlists.
- Duolingo: Teaches 40+ languages through an interface available in over 30 languages.
Lessons Learned from Failures
- HSBC’s “Assume Nothing” Campaign: The slogan translated to “Do Nothing” in many languages, costing $10 million to rebrand.
- Apple’s iOS 11 Calculator Bug: A localization issue caused the calculator to return incorrect results in some regions.
Section 8: Future Trends
Emerging Technologies and Tools
- AI-powered translation: Improving accuracy and speed of machine translations.
- Augmented reality localization: Adapting AR experiences for different cultures.
- Voice interface localization: Enhancing multilingual voice recognition and synthesis.
The Role of AI in Translation and Localization
AI is revolutionizing the localization process:
- Neural machine translation is approaching human-level quality for some language pairs.
- AI-assisted human translation is increasing productivity and consistency.
- Predictive localization suggests translations based on context and previous choices.
Predictions for the Future of Global Software Development
- Increased demand for real-time translation in applications
- Growing importance of cultural customization beyond language
- Rise of “glocalization” – balancing global reach with local relevance
Conclusion
Internationalization is not just a technical challenge; it’s a gateway to global opportunities. By embracing i18n, you’re not just translating your software—you’re opening doors to new markets, cultures, and user experiences.
Key takeaways:
- Start with a solid strategy and planning
- Implement best practices in your code and design
- Leverage tools and resources for efficient localization
- Stay aware of cultural nuances and potential pitfalls
- Keep an eye on emerging trends and technologies
Remember, This is an ongoing process. As your application evolves, so should your internationalization efforts. By making it an integral part of your development process, you’ll create software that truly speaks to a global audience.
“The limits of my language mean the limits of my world.” – Ludwig Wittgenstein
FAQ Section
- What is i18n?
- i18n stands for internationalization, which is the process of designing software applications so they can be easily adapted to different languages and regions without engineering changes.
- How does i18n differ from localization?
- Internationalization is the process of designing software for multiple languages and regions, while localization is the process of adapting the internationalized software for a specific language or region.
- Why is i18n important?
- i18n is crucial for reaching a global audience, ensuring usability and accessibility for users worldwide, and complying with regional regulations and standards.
- What are some common challenges in implementing i18n?
- Challenges include handling different character sets, managing translations, dealing with cultural differences, and maintaining consistency across languages.
- What tools are available for managing i18n?
- Tools such as PhraseApp, Transifex, and Crowdin can help manage translations, while frameworks like ngx-translate, and vue-i18n assist with implementing i18n in code.