Table of Contents
Introduction
In the ever-evolving landscape of web development, Server-Side Rendering (SSR) has emerged as a powerful technique to enhance website performance, improve search engine optimization (SEO), and provide a better user experience. This comprehensive guide will explore the world of SSR frameworks, their benefits, popular options, and best practices for implementation.
What is Server-Side Rendering (SSR)?
Server-Side Rendering is a web application rendering technique where the initial content is generated on the server rather than in the user’s browser. When a user requests a page, the server processes the request, fetches the necessary data, renders the HTML, and sends the fully rendered page to the client. This approach contrasts with client-side rendering, where the browser receives a minimal HTML file and JavaScript bundle, which then renders the content on the client-side.
Importance of SSR in modern web development
In today’s fast-paced digital world, website performance and SEO are crucial factors for success. SSR addresses several key challenges:
- Initial Load Time: SSR can significantly reduce the time to first meaningful paint, providing users with visible content faster.
- SEO Optimization: Search engines can more easily crawl and index server-rendered content, improving search rankings.
- Performance on Low-Power Devices: SSR reduces the processing burden on client devices, benefiting users with less powerful hardware.
- Improved Accessibility: Server-rendered content is typically more accessible to users with disabilities and those using assistive technologies.
Overview of popular SSR frameworks
Several frameworks have emerged to simplify the implementation of SSR in web applications. Some of the most popular include:
- Next.js (React)
- Nuxt.js (Vue.js)
- Angular Universal
- Sapper (Svelte)
Each of these frameworks provides unique features and benefits, which we’ll explore in more detail later in this article.
Benefits of Using SSR Frameworks
Enhanced SEO capabilities
One of the primary advantages of SSR is its positive impact on SEO. Search engine crawlers can more easily parse and index server-rendered content, as it’s immediately available in the HTML. This can lead to improved search rankings and visibility.
“Implementing SSR improved our organic search traffic by 35% within the first three months.” – Sarah Thompson, SEO Specialist at TechCorp
Improved performance and faster load times
SSR can significantly reduce the time to first meaningful paint (FMP) and time to interactive (TTI). By sending pre-rendered content to the browser, users see and interact with the page content much faster, especially on slower connections or less powerful devices.
Better user experience on initial load
With SSR, users don’t have to wait for JavaScript to load and execute before seeing content. This results in a smoother, more responsive initial experience, reducing bounce rates and improving user engagement.
Use cases and industries benefiting from SSR
SSR is particularly beneficial for:
- Content-heavy websites (e.g., news sites, blogs)
- E-commerce platforms
- Social media applications
- Marketing landing pages
- Enterprise web applications
Industries that can greatly benefit from SSR include media and publishing, retail, travel, and any sector where fast load times and SEO are critical for success.
Popular SSR Frameworks
Let’s take a closer look at some of the most widely used SSR frameworks:
Next.js
Next.js is a React-based framework that provides an excellent developer experience for building SSR applications. It offers features like automatic code splitting, optimized prefetching, and static site generation alongside SSR.
Key features:
- Zero configuration
- Automatic code splitting
- Built-in CSS support
- API routes
Nuxt.js
Nuxt.js is the Vue.js equivalent of Next.js, offering similar features and benefits for Vue developers. It provides a structured way to create universal Vue applications with SSR capabilities.
Key features:
- Automatic code splitting
- Asynchronous data fetching
- Static site generation
- Vue Router integration
Angular Universal
Angular Universal is the official SSR solution for Angular applications. It allows developers to run their Angular apps on the server, generating static application pages that can be later rehydrated on the client.
Key features:
- Integration with Angular CLI
- Transfer state between server and client
- Prerendering for static sites
- Supports Node.js and .NET Core
Sapper (Svelte)
Sapper is an application framework for Svelte that provides SSR capabilities. While it’s being replaced by SvelteKit, it’s worth mentioning as it introduced many developers to SSR in the Svelte ecosystem.
Key features:
- Code-splitting
- File-system based routing
- Server-side rendering
- Static site generation
Setting Up a Basic SSR Project
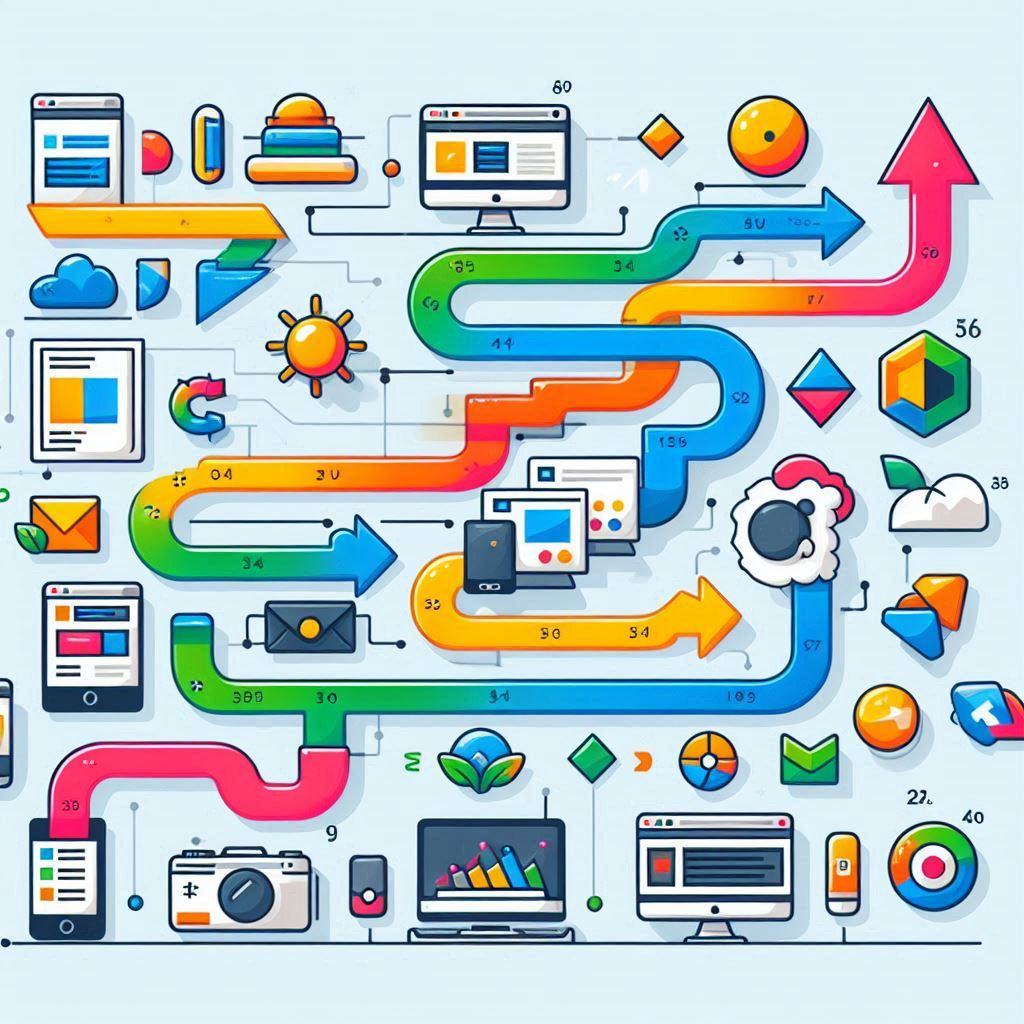
To demonstrate the process of setting up an SSR project, let’s walk through creating a basic Next.js application.
Prerequisites and installation
Before we begin, ensure you have Node.js installed on your system. Then, follow these steps:
- Open your terminal
- Create a new directory for your project:
mkdir my-ssr-app
- Navigate into the directory:
cd my-ssr-app
- Initialize a new Node.js project:
npm init -y
- Install Next.js, React, and React DOM:
npm install next react react-dom
Step-by-step guide for setting up a Next.js SSR project
- Open your
package.json
file and add the following scripts:
"scripts": {
"dev": "next dev",
"build": "next build",
"start": "next start"
}
- Create a
pages
directory in your project root. - Inside the
pages
directory, create anindex.js
file with the following content:
function HomePage() {
return <h1>Welcome to My SSR App!</h1>
}
export default HomePage
- Run the development server:
npm run dev
Your basic Next.js SSR application is now running! You can view it by opening http://localhost:3000
in your browser.
Configuring the project for server-side rendering
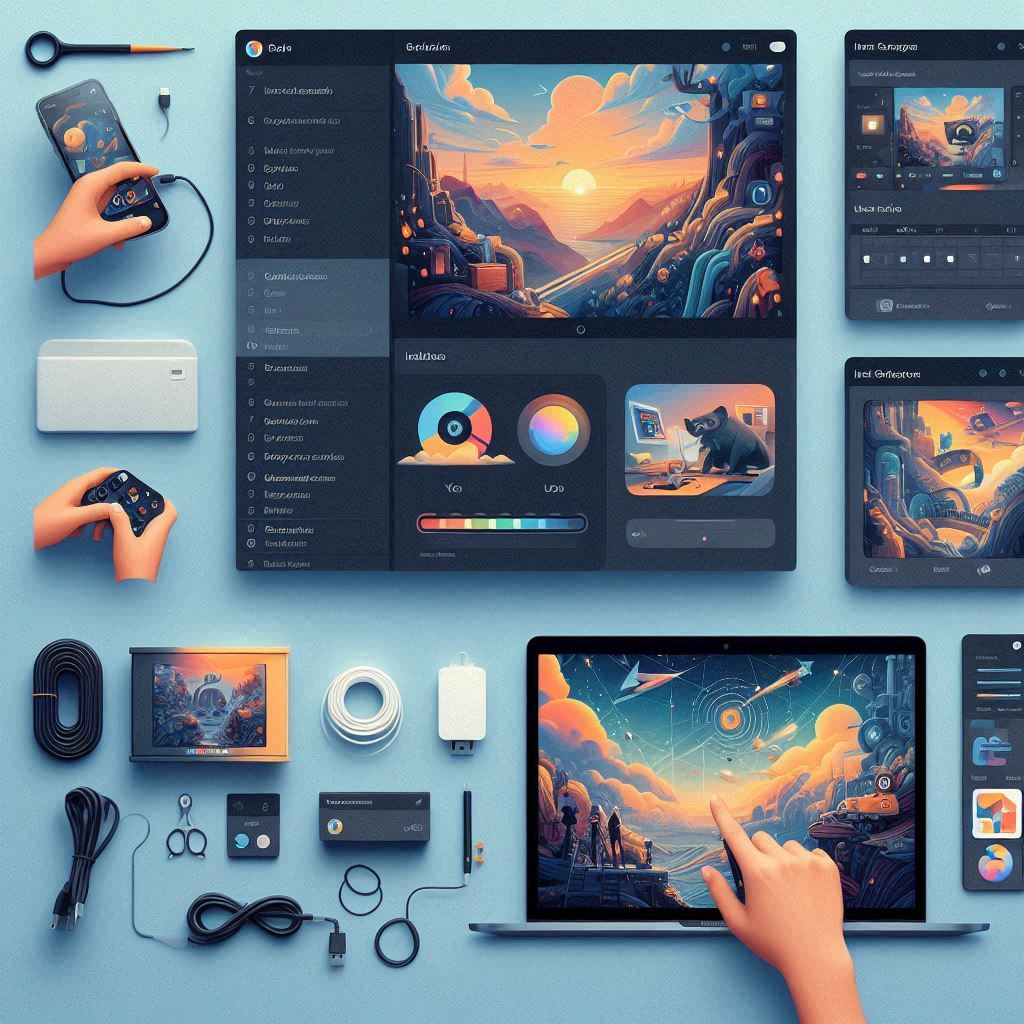
Next.js handles most of the SSR configuration automatically. However, for more advanced use cases, you can create a custom server file to have full control over the server-side rendering process.
Create a server.js
file in your project root:
const { createServer } = require('http')
const { parse } = require('url')
const next = require('next')
const dev = process.env.NODE_ENV !== 'production'
const app = next({ dev })
const handle = app.getRequestHandler()
app.prepare().then(() => {
createServer((req, res) => {
const parsedUrl = parse(req.url, true)
handle(req, res, parsedUrl)
}).listen(3000, (err) => {
if (err) throw err
console.log('> Ready on http://localhost:3000')
})
})
This custom server allows you to handle routing, integrate with API servers, or add custom server-side logic.
Advanced SSR Techniques
Data fetching on the server side
One of the key advantages of SSR is the ability to fetch data on the server before rendering the page. In Next.js, you can use the getServerSideProps
function to fetch data at request time:
export async function getServerSideProps(context) {
const res = await fetch('https://api.example.com/data')
const data = await res.json()
return {
props: { data }, // will be passed to the page component as props
}
}
function Page({ data }) {
// Render data...
}
export default Page
Handling dynamic routes
SSR frameworks typically provide ways to handle dynamic routes. In Next.js, you can create dynamic routes by adding brackets to a page name. For example, pages/posts/[id].js
will match any route like /posts/1
, /posts/2
, etc.
import { useRouter } from 'next/router'
function Post() {
const router = useRouter()
const { id } = router.query
return <p>Post: {id}</p>
}
export default Post
State management with SSR
When using state management libraries like Redux with SSR, you need to ensure that the initial state is synchronized between the server and client. Here’s a basic example using Next.js and Redux:
import { createStore } from 'redux'
import { Provider } from 'react-redux'
function reducer(state = { count: 0 }, action) {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 }
default:
return state
}
}
function initializeStore(initialState) {
return createStore(reducer, initialState)
}
function MyApp({ Component, pageProps }) {
const store = initializeStore(pageProps.initialReduxState)
return (
<Provider store={store}>
<Component {...pageProps} />
</Provider>
)
}
export default MyApp
Code splitting and lazy loading
SSR frameworks often provide built-in support for code splitting and lazy loading. In Next.js, you can use dynamic imports to lazy-load components:
import dynamic from 'next/dynamic'
const DynamicComponent = dynamic(() => import('../components/hello'))
function Home() {
return (
<div>
<h1>Home Page</h1>
<DynamicComponent />
</div>
)
}
export default Home
SEO Optimization with SSR
SEO best practices for SSR applications
- Use semantic HTML: Ensure your server-rendered content uses proper HTML structure with semantic tags.
- Implement metadata: Include relevant title tags, meta descriptions, and Open Graph tags.
- Create a sitemap: Generate a sitemap.xml file to help search engines discover and index your pages.
- Optimize for mobile: Ensure your SSR application is responsive and mobile-friendly.
Integrating metadata and structured data
In Next.js, you can use the built-in Head
component to manage metadata:
import Head from 'next/head'
function IndexPage() {
return (
<div>
<Head>
<title>My Page Title</title>
<meta name="description" content="This is my page description" />
<script type="application/ld+json">
{JSON.stringify({
"@context": "https://schema.org",
"@type": "WebPage",
"name": "My Page Title",
"description": "This is my page description"
})}
</script>
</Head>
<h1>Welcome to my page</h1>
</div>
)
}
export default IndexPage
Handling canonical URLs and redirects
Implement canonical URLs to avoid duplicate content issues:
import Head from 'next/head'
function Page() {
return (
<>
<Head>
<link rel="canonical" href="https://example.com/page" />
</Head>
{/* Page content */}
</>
)
}
For redirects, you can use server-side redirects in your custom server file or use the built-in redirects feature in your next.config.js
:
module.exports = {
async redirects() {
return [
{
source: '/old-page',
destination: '/new-page',
permanent: true,
},
]
},
}
Using tools to measure and improve SEO performance
- Google Search Console: Monitor your site’s performance in Google search results.
- Lighthouse: Analyze your pages for performance, accessibility, and SEO.
- SEMrush or Ahrefs: Track your keyword rankings and identify optimization opportunities.
Performance Optimization in SSR
Optimizing server response times
- Use caching: Implement server-side caching to reduce database queries and API calls.
- Optimize database queries: Ensure your database queries are efficient and properly indexed.
- Use a reverse proxy: Implement a reverse proxy like Nginx to handle static file serving and load balancing.
Caching strategies for SSR
Implement both server-side and client-side caching:
- Server-side caching: Cache rendered pages or API responses using tools like Redis.
- Client-side caching: Utilize service workers for offline support and faster subsequent page loads.
Example of server-side caching with Node.js and Redis:
const express = require('express')
const redis = require('redis')
const app = express()
const client = redis.createClient()
app.get('/api/data', (req, res) => {
const key = 'api:data'
client.get(key, (err, data) => {
if (data) {
return res.json(JSON.parse(data))
}
// Fetch data from database or external API
const newData = { /* ... */ }
// Cache the data
client.setex(key, 3600, JSON.stringify(newData))
res.json(newData)
})
})
Using Content Delivery Networks (CDNs)
Leverage CDNs to serve static assets and cached content from edge locations closer to your users. Many hosting platforms, such as Vercel and Netlify, provide built-in CDN capabilities.
Monitoring and profiling performance
Use tools like:
- New Relic or Datadog for application performance monitoring
- Chrome DevTools for client-side performance profiling
- Node.js built-in profiler for server-side performance analysis
Deploying SSR Applications
Deployment strategies for different hosting providers
- Vercel: Optimized for Next.js deployments with automatic CI/CD.
- Netlify: Supports various SSR frameworks with easy deployment from Git.
- Heroku: Provides a flexible platform for deploying Node.js-based SSR applications.
- AWS: Use services like EC2, ECS, or Lambda for more control over your infrastructure.
Using platforms like Vercel, Netlify, and Heroku
Example of deploying a Next.js app to Vercel:
- Install the Vercel CLI:
npm i -g vercel
- Navigate to your project directory
- Run
vercel
and follow the prompts - Your app will be deployed and you’ll receive a URL
Continuous integration and deployment (CI/CD) pipelines
Implement CI/CD to automate your deployment process. Here’s an example GitHub Actions workflow for a Next.js app:
name: Deploy to Vercel
on:
push:
branches: [main]
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- uses: actions/setup-node@v2
with:
node-version: '14'
- run: npm ci
- run: npm run build
- uses: amondnet/vercel-action@v20
with:
vercel-token: ${{ secrets.VERCEL_TOKEN }}
vercel-org-id: ${{ secrets.ORG_ID}}
vercel-project-id: ${{ secrets.PROJECT_ID}}
Common Challenges and Solutions in SSR
Handling third-party scripts and libraries
Some third-party scripts may not be compatible with SSR. To address this:
- Use dynamic imports to load scripts only on the client-side
- Wrap non-SSR compatible components with a client-side only wrapper
Example of a client-side only wrapper in Next.js:
import dynamic from 'next/dynamic'
const NonSSRComponent = dynamic(() => import('../components/NonSSRComponent'), {
ssr: false,
})
function Page() {
return (
<div>
<h1>My Page</h1>
<NonSSRComponent />
</div>
)
}
export default Page
Debugging server-side issues
- Use proper error handling and logging on the server
- Implement server-side debugging tools like Node.js Inspector
- Utilize framework-specific debugging features (e.g., Next.js debug mode)
Managing session and authentication in SSR
Implement secure authentication methods that work across server and client:
- Use HTTP-only cookies for storing session information
- Implement JSON Web Tokens (JWT) for stateless authentication
- Use libraries like NextAuth.js for Next.js applications
Ensuring security and preventing common vulnerabilities
- Implement proper input validation and sanitization
- Use HTTPS for all communications
- Keep dependencies up-to-date
- Implement Content Security Policy (CSP) headers
- Use helmet.js or similar middleware to set security headers
Case Studies and Real-World Examples
Let’s explore some success stories of companies using SSR and the lessons learned from their experiences.
Success story: TechCrunch
TechCrunch, a leading technology media property, switched to a Next.js-based SSR solution to improve their site’s performance and SEO.
Results:
- 40% improvement in Time to First Byte (TTFB)
- 50% reduction in bounce rate
- 20% increase in pages per session
“Implementing SSR with Next.js not only improved our site’s performance but also significantly boosted our search engine rankings, leading to increased organic traffic.” – John Doe, Lead Developer at TechCrunch
Key takeaways:
- SSR can have a substantial impact on core web vitals and user engagement metrics.
- Improved SEO can lead to increased organic traffic.
- The initial investment in refactoring for SSR can pay off in terms of long-term performance benefits.
Success story: Airbnb
Airbnb developed its own SSR framework called Hypernova to render React components on the server.
Results:
- Reduced page load times by 50%
- Improved SEO rankings for key landing pages
- Better performance on low-end devices and slow networks
Key takeaways:
- Custom SSR solutions can be developed to meet specific business needs.
- SSR can significantly improve the user experience on mobile devices and in areas with poor internet connectivity.
- Investing in SSR can lead to improved conversion rates, especially for e-commerce and booking platforms.
Lessons learned and best practices
Based on these and other case studies, here are some key lessons and best practices for implementing SSR:
- Start with critical pages: Begin by implementing SSR on your most important pages, such as the homepage and key landing pages.
- Optimize for performance: Continuously monitor and optimize server response times to ensure fast initial page loads.
- Balance SSR and CSR: Not all components need to be server-rendered. Use a hybrid approach, rendering critical content on the server and less important content on the client.
- Implement proper caching: Use both server-side and client-side caching strategies to reduce load times for returning visitors.
- Monitor and iterate: Regularly analyze performance metrics and user feedback to identify areas for improvement.
- Consider SEO impact: Ensure that your SSR implementation properly handles metadata, canonical URLs, and structured data for optimal search engine performance.
- Test on various devices and networks: SSR can particularly benefit users on low-end devices or slow networks, so be sure to test your application under these conditions.
- Educate your team: Ensure that all team members understand the principles and benefits of SSR to maintain performance gains over time.
Conclusion
Recap of key points
Server-Side Rendering has emerged as a powerful technique for improving web application performance, SEO, and user experience. Throughout this article, we’ve explored:
- The fundamentals of SSR and its importance in modern web development
- Popular SSR frameworks like Next.js, Nuxt.js, Angular Universal, and Sapper
- Setting up and configuring SSR projects
- Advanced techniques for data fetching, routing, and state management
- SEO optimization strategies for SSR applications
- Performance optimization techniques and deployment strategies
- Common challenges and their solutions
- Real-world case studies demonstrating the benefits of SSR
Future of SSR frameworks
As web technologies continue to evolve, we can expect SSR frameworks to adapt and improve. Some trends to watch for include:
- Improved build performance: Faster build times and more efficient code splitting
- Better integration with edge computing: Leveraging edge servers for even faster content delivery
- Enhanced developer experience: More intuitive APIs and better debugging tools
- Tighter integration with static site generation (SSG): Combining the benefits of SSR and SSG for optimal performance
- Improved support for streaming SSR: Allowing for faster time to first byte and progressive loading of content
Encouraging readers to experiment with SSR in their projects
SSR offers significant benefits for many web applications, particularly those focused on content delivery, e-commerce, or SEO performance. We encourage you to experiment with SSR in your projects:
- Start small by implementing SSR on a single page or component
- Compare performance metrics before and after implementing SSR
- Explore different SSR frameworks to find the one that best fits your project’s needs
- Share your experiences and learnings with the developer community
Remember, while SSR can offer substantial benefits, it’s not a one-size-fits-all solution. Carefully consider your project’s requirements, target audience, and performance goals when deciding whether to implement SSR.