In today’s digital landscape, having a well-optimized website is crucial for success. As a React developer, you might wonder how to implement effective Search Engine Optimization (SEO) strategies in your applications. Enter React Helmet – a powerful tool that can significantly boost your website’s SEO performance. In this comprehensive guide, we’ll explore how to leverage React Helmet to optimize your React applications for search engines and improve your site’s visibility.
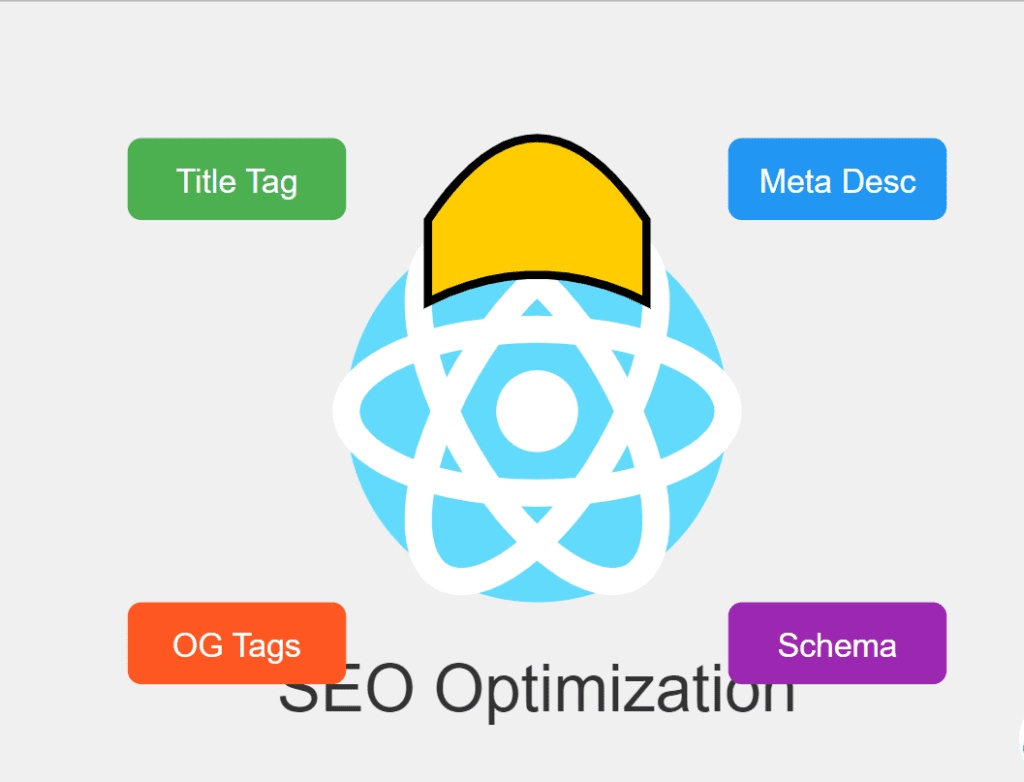
Introduction to React Helmet
React Helmet is a reusable React component that manages all changes to the document head. It provides a simple and intuitive interface to dynamically update your application’s <head>
section, including titles, meta tags, and other HTML elements that are crucial for SEO.
By using React Helmet, you can easily control the metadata of your React application, ensuring that search engines and social media platforms accurately interpret and display your content.
Why SEO Matters for React Applications
Search Engine Optimization (SEO) is the practice of increasing the quantity and quality of traffic to your website through organic search engine results. For React applications, implementing proper SEO techniques is particularly important because:
- Single Page Applications (SPAs) can be challenging for search engines: React apps are often built as SPAs, which can be difficult for search engine crawlers to index properly.
- Dynamic content requires dynamic metadata: React’s component-based structure means content often changes dynamically, necessitating metadata that updates accordingly.
- Improved user experience: SEO isn’t just about search engines; it also enhances the user experience by providing accurate and relevant information about your pages.
- Increased visibility and traffic: Well-optimized React applications rank higher in search results, leading to more organic traffic and potential customers.
By leveraging React Helmet, you can address these challenges and ensure your React application is fully optimized for search engines.
Getting Started with React Helmet
To begin using React Helmet in your project, you’ll first need to install it. Open your terminal and run the following command:
npm install react-helmet
Or if you’re using Yarn:
yarn add react-helmet
Once installed, you can import and use React Helmet in your components. Here’s a basic example:
import React from 'react';
import { Helmet } from 'react-helmet';
function App() {
return (
<div className="App">
<Helmet>
<title>My React App</title>
<meta name="description" content="A React application optimized with React Helmet" />
</Helmet>
{/* Your app content */}
</div>
);
}
export default App;
In this example, we’ve added a basic title and description meta tag to our application. These elements will be injected into the <head>
of your HTML document, making them visible to search engines and social media platforms.
Basic Usage: Setting Title and Meta Tags
Let’s dive deeper into how we can use React Helmet to set various important SEO elements:
Title Tags
The title tag is one of the most critical elements for SEO. It appears in search engine results pages (SERPs) and browser tabs. Here’s how to set it dynamically:
import React from 'react';
import { Helmet } from 'react-helmet';
function BlogPost({ title, content }) {
return (
<div>
<Helmet>
<title>{title} | My awesome blog</title>
</Helmet>
<h1>{title}</h1>
<p>{content}</p>
</div>
);
}
export default BlogPost;
In this example, we’re dynamically setting the title based on the blog post’s title, ensuring each page has a unique and relevant title.
Meta Description
The meta description provides a brief summary of your page’s content. While it doesn’t directly impact rankings, it can influence click-through rates from SERPs:
<Helmet>
<meta name="description" content="Learn how to master SEO with React Helmet. This comprehensive guide covers everything from basic setup to advanced techniques." />
</Helmet>
Canonical URLs
Canonical URLs help prevent duplicate content issues by specifying the “preferred” version of a page:
<Helmet>
<link rel="canonical" href="https://www.yourdomain.com/blog/mastering-seo-with-react-helmet" />
</Helmet>
Robots Meta Tag
Control how search engines crawl and index your pages:
<Helmet>
<meta name="robots" content="index, follow" />
</Helmet>
Advanced Techniques: Dynamic Meta Tags and Open Graph
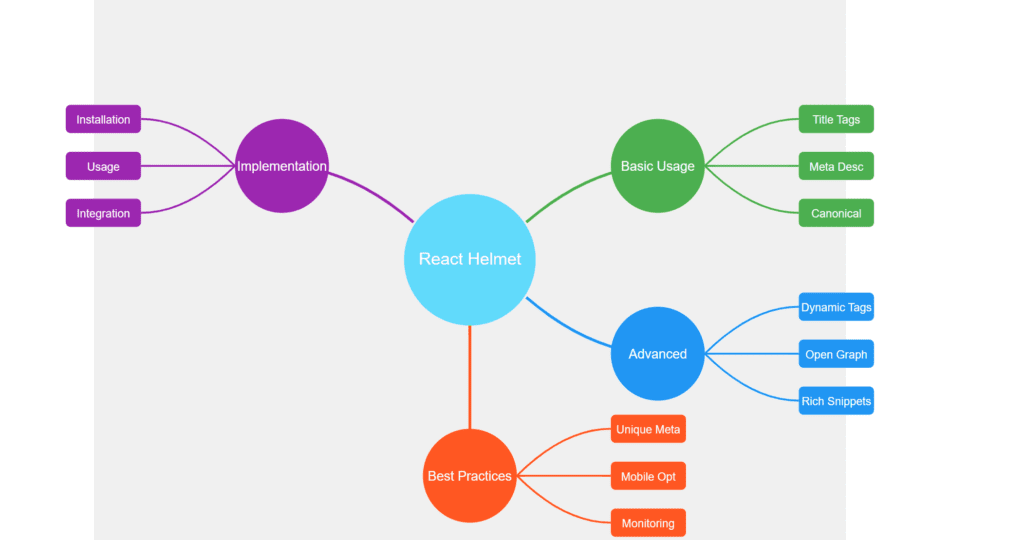
As your React application grows more complex, you’ll need to implement more advanced SEO techniques. Let’s explore some of these:
Dynamic Meta Tags
For content that changes frequently or is user-generated, you’ll want to update meta tags dynamically:
import React from 'react';
import { Helmet } from 'react-helmet';
function ProductPage({ product }) {
return (
<div>
<Helmet>
<title>{product.name} - Our Store</title>
<meta name="description" content={`Buy ${product.name} for $${product.price}. ${product.shortDescription}`} />
<meta name="keywords" content={product.keywords.join(', ')} />
</Helmet>
{/* Product details */}
</div>
);
}
export default ProductPage;
This example demonstrates how to create dynamic meta tags based on product information, ensuring each product page has unique and relevant metadata.
Open Graph Protocol
Open Graph tags help control how your content appears when shared on social media platforms:
<Helmet>
<meta property="og:title" content="Mastering SEO with React Helmet" />
<meta property="og:description" content="Learn how to optimize your React applications for search engines using React Helmet." />
<meta property="og:image" content="https://yourdomain.com/path/to/image.jpg" />
<meta property="og:url" content="https://yourdomain.com/blog/mastering-seo-with-react-helmet" />
<meta property="og:type" content="article" />
</Helmet>
By implementing Open Graph tags, you ensure your content looks great when shared on platforms like Facebook, Twitter, and LinkedIn.
Optimizing for Rich Snippets
Rich snippets are enhanced search results that display additional information beyond the standard title, URL, and meta description. They can significantly improve your click-through rates from SERPs. React Helmet can help you implement the necessary structured data for rich snippets:
<Helmet>
<script type="application/ld+json">
{`
{
"@context": "https://schema.org",
"@type": "BlogPosting",
"headline": "Mastering SEO with React Helmet",
"image": "https://yourdomain.com/path/to/image.jpg",
"author": {
"@type": "Person",
"name": "John Doe"
},
"datePublished": "2024-09-13",
"description": "Learn how to optimize your React applications for search engines using React Helmet."
}
`}
</script>
</Helmet>
This example adds structured data for a blog post, which can result in rich snippets in search results, potentially including author information, publish date, and more.
Handling Multiple Pages and Routes
For multi-page React applications, it’s crucial to manage SEO elements across different routes. Here’s an example of how you might structure this:
import React from 'react';
import { Helmet } from 'react-helmet';
import { Route, Switch } from 'react-router-dom';
import Home from './components/Home';
import About from './components/About';
import Blog from './components/Blog';
function App() {
return (
<div>
<Helmet>
<title>My React App</title>
<meta name="description" content="Default description for My React App" />
</Helmet>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/blog" component={Blog} />
</Switch>
</div>
);
}
export default App;
In this setup, you have a default Helmet configuration in the App component, which can be overridden in individual route components:
function About() {
return (
<div>
<Helmet>
<title>About Us | My React App</title>
<meta name="description" content="Learn more about our company and mission." />
</Helmet>
<h1>About Us</h1>
{/* Rest of the component */}
</div>
);
}
This approach ensures that each page has its own unique and relevant metadata.
Best Practices for React Helmet SEO
To maximize the SEO benefits of React Helmet, consider these best practices:
- Use unique titles and descriptions for each page: Avoid duplicate metadata across your site.
- Keep titles concise: Aim for 50-60 characters to ensure they display fully in search results.
- Write compelling meta descriptions: Use 150-160 characters to accurately summarize your content and encourage clicks.
- Implement structured data: Use JSON-LD to provide detailed information about your content to search engines.
- Optimize for mobile: Ensure your React app is responsive and that metadata is appropriate for mobile devices.
- Use canonical URLs: Prevent duplicate content issues, especially if you have pages accessible through multiple URLs.
- Leverage dynamic metadata: Update titles, descriptions, and other metadata based on the content of each page or component.
- Monitor and adjust: Regularly review your SEO performance and adjust your React Helmet implementation as needed.
Measuring SEO Impact
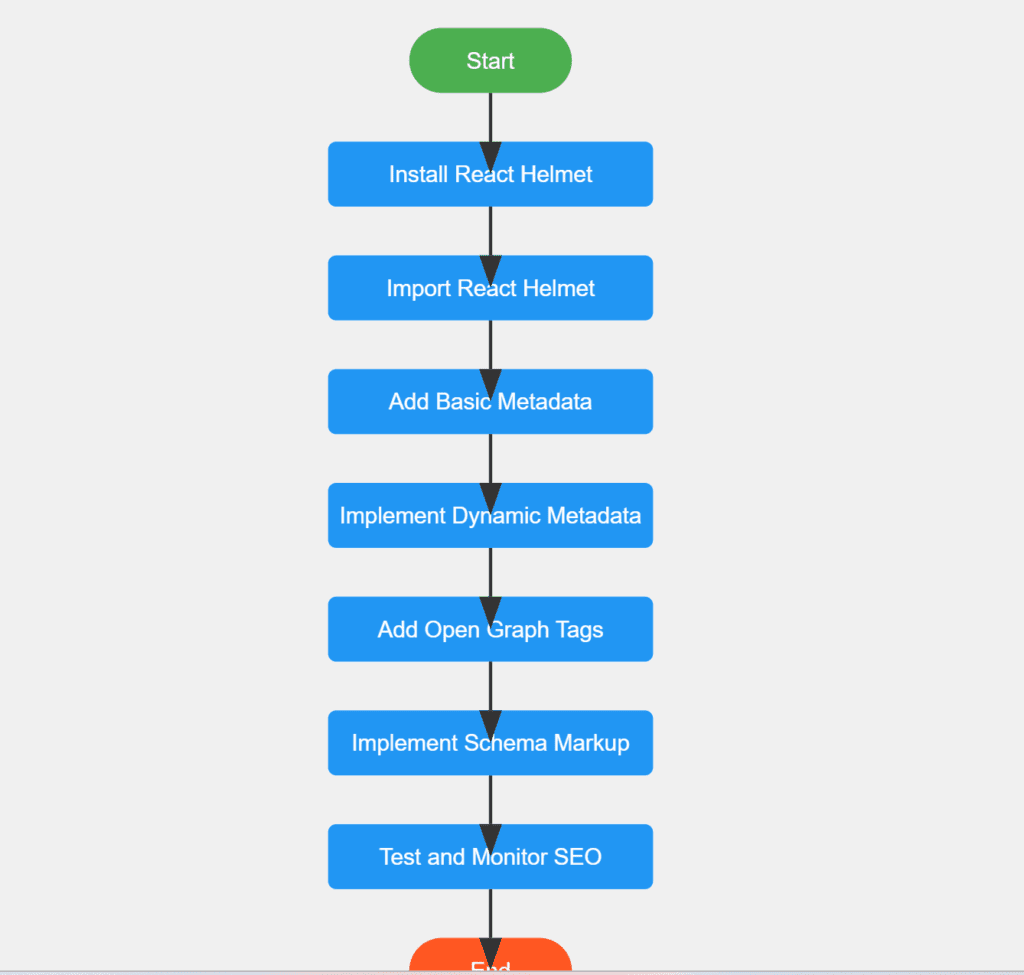
After implementing React Helmet for SEO, it’s essential to measure its impact. Here are some ways to track your progress:
- Google Search Console: Monitor your site’s performance in Google search results, including impressions, clicks, and average position.
- Analytics tools: Use tools like Google Analytics to track organic traffic, bounce rates, and user behavior.
- SEO auditing tools: Regularly audit your site with tools like Screaming Frog or SEMrush to ensure your meta tags are correctly implemented.
- Performance monitoring: Use tools like Lighthouse to assess your site’s overall performance, including SEO factors.
Remember that SEO is an ongoing process. Continuously monitor your results and make adjustments to your React Helmet implementation as needed.
Conclusion
React Helmet is a powerful tool for optimizing your React applications for search engines. By dynamically managing your app’s metadata, you can significantly improve your site’s visibility and performance in search results.
In this guide, we’ve covered everything from basic setup to advanced techniques, including handling dynamic content, implementing structured data for rich snippets, and managing SEO across multiple routes. By following these practices and consistently applying React Helmet throughout your React application, you’ll be well on your way to achieving better search engine rankings and increased organic traffic.
Remember, SEO is an ongoing process that requires regular attention and updates. Stay informed about the latest SEO best practices and continue to refine your implementation of React Helmet to ensure your React applications remain optimized for search engines and users alike.