Introduction
Table of Contents
React Router has emerged as an indispensable tool in the development of single-page applications (SPAs) using React. By enabling dynamic client-side routing, React Router helps developers ensure that the user interface reacts seamlessly to user interactions, simulating a multi-page website experience within a single HTML document. This presents a win-win scenario: users enjoy fast, uninterrupted access, while developers appreciate the streamlined and efficient navigation management. This guide will delve into how React Router facilitates swift and elegant client-side routing, improving both the performance and usability of your web applications.
Understanding React Router
What is React Router?
React Router is a powerful library used in web development to handle routing in applications built with React. It provides seamless client-side routing by enabling the navigation among views of various components in a React Application, without refreshing the page. Essentially, React Router keeps your UI in sync with the URL. It mimics the navigation of multipage websites while maintaining the speed and responsiveness of a single-page application. This library plays a crucial role in defining both simple and dynamic routes and handling complex application architectures with ease.
Benefits of using React Router
The use of React Router in a project offers multiple advantages, prominently centered on enhancing the user and developer experience. Here are some key benefits:
– Seamless User Experience: React Router enables the users to navigate through the application smoothly without the usual page refreshes seen in traditional multipage websites. This results in a fluid, fast, and responsive user experience.
– Maintains UI/URL Synchronization: It synchronizes the URL with your React app’s UI. This means whenever the URL changes, the corresponding view in the application updates instantly.
– Efficient Code Splitting: React Router allows developers to split the code at a route level. Each route can dynamically load just the necessary code needed at that time, which significantly reduces the initial load time.
– Easy to Integrate: It is designed to work seamlessly with other libraries in the React ecosystem. Thus, integrating it into existing projects or starting a new one is generally straightforward.
– Dynamic Routing: Dynamic routing is created as and when the app is rendered, not exclusively at the outset. This enhances flexibility and adaptability in managing routes.
Getting Started with React Router
Installation and setup
To begin using React Router in your project, you first need to install it. For a project that uses React, you can add React Router by running the following command in your project’s root directory:
npm install react-router-dom
or if you are using Yarn:
yarn add react-router-dom
After installing, you need to import the BrowserRouter from ‘react-router-dom’ in your React application. This component uses the HTML5 history API to keep your UI in sync with the URL. Generally, you would wrap your root component in \`\` in your main index.js file like so:
\`\`\`javascript
import React from ‘react’;
import ReactDOM from ‘react-dom’;
import { BrowserRouter } from ‘react-router-dom’;
import App from ‘./App’;
ReactDOM.render(
,
document.getElementById(‘root’)
);
\`\`\`
Basic routing
To set up basic routing in your React application, you would define Route components within your Router component, each corresponding to a specific path. Here’s a simple example:
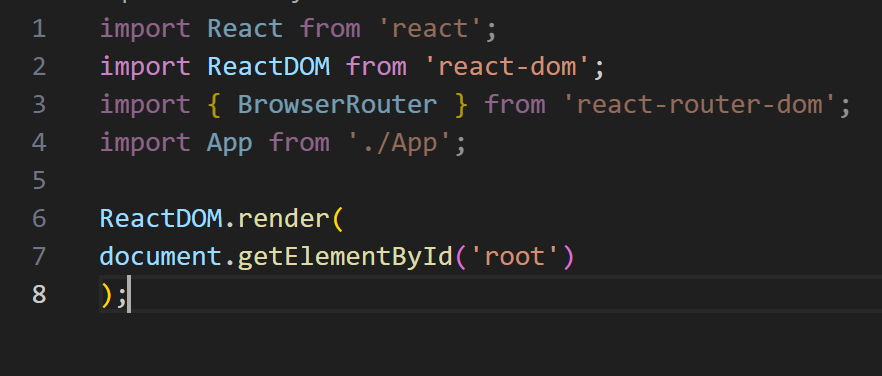
In this setup, \`\` is used to render only the first route that matches the location. This prevents the rendering of multiple components at thepeerute front.
Nested routing
Nested routing, or child routing, helps in organizing the parts of your application that are nested. It is particularly useful for layouts which require different child components under the same overall structure. Here is how you might configure nested routes:
\`\`\`javascript
function App() {
return (
< lineoute>
);
}
\`\`\`
In this example, users accessing \`/users/admin\` will render the \`Admin\` component under the \`Users\` component, achieving a nested view. It simplifies the management of intricate routes by segmenting the UI into smaller, manageable parts, thus making the application easier to develop and maintain.
Advanced Routing Techniques
React Router offers powerful capabilities for managing complex routing scenarios in single-page applications. Below, we delve into advanced techniques such as dynamic routing, leveraging route parameters, and using redirects and navigation guards to enhance user experience and application security.
Dynamic routing
Dynamic routing allows developers to handle routes based on user interactions or data conditions rather than defining all possible routes upfront. This strategy becomes essential in large applications where defining every potential route statically can lead to bloated and hard-to-maintain codebases. With React Router, developers can dynamically define routes based on business logic, enabling them to build more flexible and adaptive web applications. For example, they can render routes based on user permissions or the presence of certain data.
Route parameters
Utilizing route parameters is a key feature in creating interactive and user-friendly URLs. Parameters in URLs make it easier to reference specific content, such as user profiles or specific products. In React Router, parameters can be easily integrated into routes by using the colon syntax in the route path. For instance, defining a path as \`/user/:userId\` allows the application to fetch and display data for a specific user based on the \`userId\` parameter. This technique not only helps in creating more navigable URLs but also enhances the dynamic loading of content based on user interaction.
Redirects and navigation guards
Redirects are critical for guiding users through the application flow, particularly in scenarios involving login redirections, or page restrictions based on user roles. Navigation guards are utilized to protect routes by imposing security checks before rendering components or proceeding with routing actions. React Router supports redirects using the \`\` component, enabling developers to programmatically navigate users to different paths. Similarly, by leveraging the \`BrowserRouter\` and \`Route\` components, developers can implement conditions to guard routes and ensure that certain criteria are met before rendering a component or accessing a route.
Client-Side Navigation
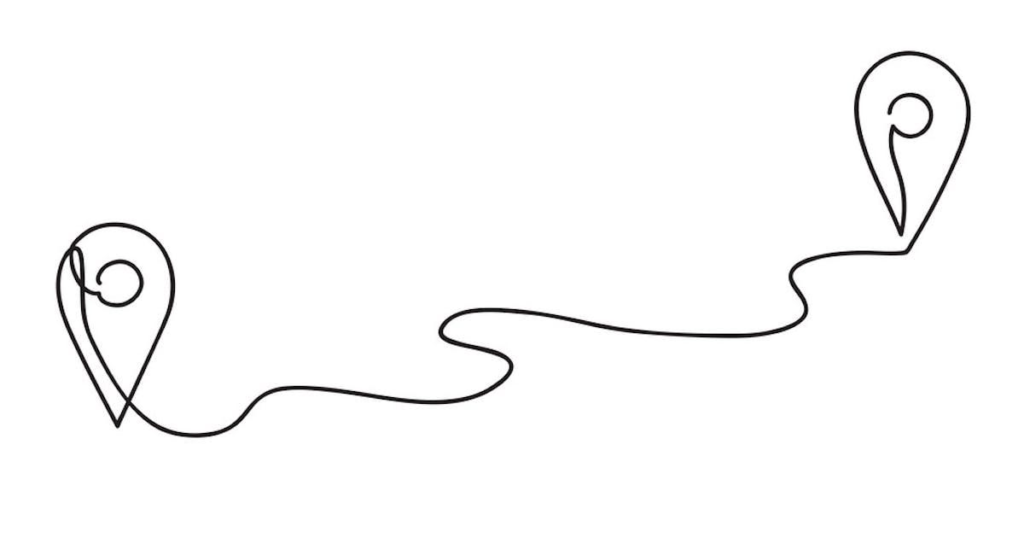
Image courtesy: medium
To enhance the performance and user experience of web applications, optimizing client-side navigation is crucial. Techniques like lazy loading components, code splitting, and adhering to best performance practices are key factors in achieving an efficient, responsive application.
Lazy loading components
Lazy loading is a strategy to defer the loading of non-critical resources at page load, instead, fetching components asynchronously as they are needed. React Router can integrate seamlessly with React’s lazy loading capabilities using the \`React.lazy()\` function combined with the \`Suspense\` component. This allows components to be loaded only when a particular route is activated. Implementing lazy loading can significantly reduce the initial load time, making the application faster and thus improving the user experience, especially in applications with heavy components.
Code splitting for faster loading times
Code splitting further enhances application performance by splitting the code into smaller chunks that can be loaded on demand. This means that users download only the necessary code needed to render the active route rather than the entire application. Using dynamic \`import()\` statements in conjunction with React Router’s lazy loading makes code splitting feasible and effective. This technique is especially beneficial in large applications where the size of the JavaScript bundle can seriously affect performance.
Best gestures for the motion sensor to perform
Following best practices for optimizing performance in React Router-based applications involves a few strategic approaches. Minimizing component re-renders by properly managing state and using React’s \`memo\` and \`useMemo\` can prevent unnecessary re-renders, enhancing performance. Efficiently managing resources by avoiding in-line function definitions directly in the JSX which can lead to frequent re-renders is also crucial. Additionally, keeping routes and components as light as possible, and prefetching data for upcoming navigations can help maintain a smooth and responsive user experience.
By mastering these advanced techniques in routing and optimization strategies, developers can significantly improve the performance and user engagement of React-based single-page applications.
Enhancing User Experience
When adopting React Router for your web projects, enhancing user experience should be a paramount concern. Smooth navigation and intuitive user interfaces can significantly increase user engagement and satisfaction. Leveraging React Router’s capabilities, developers can create seamless, dynamic experiences that make web applications feel more like native apps.
Animated Transitions
One of the compelling features of React Router is the ability to include animated transitions between routes. This not only makes the application visually appealing but also provides a smoother user experience by mimicking the navigational feel of traditional multi-page websites. Implementing animated transitions involves using the React Transition Group library in conjunction with React Router to animate components entering and leaving the DOM.
Here are some steps to implement basic animated transitions:
– Setup: Install \`react-transition-group\` alongside React Router in your project.
– Configure Routes: Set up your routes normally using \`\` and \`\` components from React Router.
– Wrap Route Components: Wrap your route components in the \`CSSTransition\` and \`TransitionGroup\` components from \`react-transition-group\`.
– Apply CSS Transitions: Define the styles for enter and exit transitions in your CSS, ensuring they are triggered when the component’s state changes.
These animated transitions can significantly enhance the user interface by providing visual cues about the application’s behavior, making route changes less jarring and visually engaging.
Handling 404 Errors Gracefully
A robust application is not only about handling expected behavior but also managing unexpected situations gracefully. Implementing a user-friendly 404 error page using React Router can dramatically improve the user experience in cases where a route does not match any predefined paths.
To handle 404 errors:
– Define a Catch-all Route: After all other routes, define a wildcard route that catches all unmatched URLs. This is done by specifying \`\` with a path of \`*\` that renders a custom 404 component.
– Create a 404 Component: Design a 404 error page that aligns with the design aesthetics of the rest of your application. It should provide useful information about the error and suggest further actions, such as returning to the homepage or searching for content.
– Enhance User Guidance: Include links to popular or relevant sections of your site, search functionality, or contact information. Offering these options encourages users to continue interacting with your application despite the hicompromisecounter.
Effectively managing 404 errors not only prevents user frustration but also contributes to retaining visitors who might otherwise leave your site due to navigation issues.
Real-world Examples of React Router Implementation
Image courtesy: Unsplash
Observing real-world examples can provide invaluable insights into how React Router can be effectively implemented in web applications. These examples highlight the flexibility and scalability of React Router, demonstrating how it accommodates the diverse needs of modern web applications.
Example 1: E-commerce Platform Navigation
In an e-commerce application, React Router is used to manage the navigation between product categories, detailed product views, and the checkout process without reloading the page. By using dynamic routes, the platform can render thousands of unique product pages based on the product ID in the URL. This setup enhances the shopping experience by making it fast, fluid, and responsive. Animated transitions smooth the switch between product categories, maintaining a cohesive visual experience.
Example 2: Content Management System (CMS)
For a CMS, React Router allows content creators to seamlessly navigate between various parts of the application—such as the dashboard, post editor, and settings. Given the need for dynamic content updates and frequent navigation changes within a CMS, React Router’s dynamic routing capabilities ensure that updates are reflected in real time without page reloads, improving productivity and user engagement.
Example 3: Portfolio Website
Portfolio websites benefit significantly from React Router, especially for showcasing different projects or galleries. React Router enables such websites to present detailed case studies or artwork in a clean, dedicated route while maintaining a smooth transition between sections. This approach not only organizes content efficiently but also enhances the aesthetic appeal, keeping users engaged and interested in the presented work.
These examples illustrate how React Router can be a powerful tool for various types of web applications, enhancing both the performance and user experience by providing efficient, client-side routing solutions.
Conclusion
In the evolving landscape of web development, mastering the use of React Router is crucial for anyone looking to enhance their web applications with smooth, client-side navigation. The benefits of implementing React Router in your projects are clear: efficient page loads, improved user experience, and a boost in the application’s overall performance. By understanding the core concepts such as routes, links, and dynamic routing, developers can craft applications that are both powerful and user-friendly.
React Router makes it manageable to handle different navigation scenarios that are common in modern web applications. From guarding routes with authentication to dynamically loading data based on route parameters, the flexibility of React Router is a vital tool in the developer’s toolkit. Moreover, utilizing advanced features like nested routes and route transitions can elevate the functionality and aesthetic of your applications, making them more interactive and engaging for the end-users.
Moreover, the thriving community around React Router offers an abundance of resources and support, making it accessible for developers of all skill levels to get started and advance their skills. Whether you are building a small project or a large scale enterprise application, React Router is equipped to meet your routing needs effectively.
To summarize, React Router is an indispensable part of developing single-page applications with React. It not only provides the technical capabilities needed to implement versatile and efficient routing but also ensures that users enjoy a seamless navigation experience. Embrace the power of React Router and watch as it transforms the capabilities of your web applications, keeping you at the forefront of client-side web development. Whether you are a novice hoping to dive into the world of React or an experienced developer aiming to optimize your applications, React Router is your go-to library for state-of-the-art client-side navigation.
FAQ
Image courtesy: Unsplash
What is React Router?
React Router is a powerful library used in web development with React. It enables the implementation of dynamic routing in a web application. This means that the application can handle different URLs and load appropriate components without a full page refresh. By leveraging dynamic routing, applications become more user-friendly and efficient, providing a seamless user experience akin to navigating through various pages in a single-page application.
How do I install React Router in my project?
To install React Receiver, you need to have Node.js installed on your computer. Start by opening your terminal or command prompt. Navigate to your project directory and run the following npm command:
\`\`\`bash
npm install react-router-dom
\`\`\`
This command will add React Router to your project dependencies, allowing you to import and use it in your project to manage navigation.
Can React Router work with server-side rendering?
Yes, React Router can be integrated with server-side rendering. React Router’s structure supports both client and server-side rendering, which means it can handle URLs on the server just as well as it handles them on the client-side. This is particularly useful for SEO and for providing a faster initial page load experience, which can be critical for user retention.
What is the difference between \`\` and \`\`?
\`\` and \`\` are two types of routers offered by React Router, each suitable for different deployment scenarios:
– \`\` uses HTML5 history API to create real URLs. This is the best choice for dynamic websites where you want clean URLs without hashes.
– \`\` uses the URL’s hash part to keep your UI in sync with the URL. It is useful in scenarios where you do not have server configurations to handle URLs or for static file servers.
Deciding between \`\` and \`\` depends largely on your project requirements and the capabilities of your server environment.
Pingback: Beyond Navigation: Innovative Uses of React Router