API pagination is a critical technique used in software development and database management that facilitates efficient data handling and retrieval. As the volume of data managed by applications continues to grow exponentially, effectively fetching and displaying this data becomes increasingly complex and resource-intensive. Pagination helps in breaking down large datasets into manageable chunks, making data queries more efficient and improving user experience by reducing load time. This technique not only streamlines data access but also enhances the overall performance of digital systems, making it a vital component in the design and implementation of APIs (Application Programming Interfaces). As we delve deeper, we will explore the significance of API pagination and how it is implemented to optimize data-heavy environments.
Importance of API Pagination
API pagination is a technique used in software development to divide large datasets into manageable, discrete chunks, allowing applications to retrieve and display data in a sequential order, page by page. When an API call is made, instead of returning all records at once, only a specific subset of data—often defined by parameters like ‘page’ and ‘limit’—is sent to the client. This method is particularly useful when dealing with databases or data sources that contain a vast amount of entries, ensuring that the data retrieval process is both efficient and scalable.
Benefits of API Pagination in Data Retrieval
Implementing pagination in data retrieval offers numerous benefits that enhance application performance and user experience. Firstly, it reduces the load on the server as smaller batches of data are easier to handle compared to massive datasets. This results in faster response times and less memory consumption. Secondly, pagination makes an application more scalable by allowing it to handle increases in data volume without degradation in performance. It also improves the user experience by making data browsing more manageable and intuitive. Finally, from a development standpoint, paginated data is easier to work with and debug, as developers can isolate issues within specific pages of data.
Challenges of Handling Large Data Sets
Large data sets can severely impact API performance, leading to slower response times, higher server loads, and potential timeouts. When an API attempts to retrieve large volumes of data in a single call, it consumes substantial system resources, including CPU, memory, and bandwidth. This strain not only slows down the API itself but can also affect the stability and performance of the server hosting the API, potentially disrupting service for all users. Moreover, heavy data load can lead to increased latency, thereby affecting the end-user’s interaction with the application, which might lead to a poor user experience or, worse, loss of users.
Issues Related to Loading and Processing Large Data
Loading and processing large amounts of data at once can create several technical and logistical issues. One major problem is the “out-of-memory” error, where the system runs out of allocatable memory during data retrieval or processing, causing processes to fail. This issue is particularly prevalent in environments with limited resources or older systems. Additionally, when large data sets are loaded in their entirety, it leads to prolonged wait times for users, as the data needs to be fetched, transferred, processed, and then rendered by the client application. This not only tests the patience of users but also increases the risk of timeouts or crashes, especially on mobile devices or other lower-powered devices. Cumbersome data sets can also complicate maintenance and updates, as the bulk of data can obscure underlying issues or make it difficult to implement changes swiftly.
Best Practices for API Pagination
Effective pagination can not only streamline API performance but also enhance the user experience by delivering data in more manageable chunks. Adopting recognized best practices is crucial for ensuring that your API can handle large volumes of data efficiently.
Setting Pagination Limits
When implementing pagination, it is important to define clear limits on the number of items returned in a single response. This helps in maintaining the performance of the API by not overloading the server with too many requests at once. Here are a few tips for setting pagination limits:
- Default Limit Values: Set a reasonable default limit that balances load and usability. Common default limits range from 10 to 100 items per page.
- Maximum Limit: Always enforce a maximum limit to prevent excessively large requests. This cap should be set based on server capabilities and the average data size.
- Client Flexibility: Allow clients to specify a custom limit up to the maximum. This can cater to clients needing more flexibility depending on their use case.
Using Cursor-Based Pagination for Improved Performance
Cursor-based pagination is often preferred over traditional methods like offset pagination because it provides more consistent and reliable performance. This method involves returning a pointer (the cursor) in the response, which directs the client where to start the next request. Benefits of cursor-based pagination include:
- Reduced Server Load: Since data retrieval starts right where the last query ended, there’s no need to re-process records on subsequent pages.
- Consistency Across Requests: Even if new data is added or existing data is modified, cursor-based pagination ensures that the pagination sequence remains stable.
- Scalability: This method is suitable for databases with large data sets as it efficiently handles incremental data retrieval.
Implementing Proper Error Handling Mechanisms
Proper error handling is essential in pagination to ensure a smooth user experience and to reduce the number of failed requests handled by your server. Effective error handling in pagination should include:
- Clear Error Messages: When errors occur (like a request for a nonexistent page), provide clear, concise error messages that help developers understand and correct the issue.
- Rate Limiting: Implement rate limiting to prevent abuse and to ensure fair usage among all users.
- Retry Logic and Backoff Strategies: Integrate smart retry mechanisms that include exponential backoff strategies to better handle request retries without burdening the server.
Case Studies on Efficient Data RetrieCard Retrieval
Examining real-world applications of API pagination can highlight its effectiveness and encourage adoption in various projects. Let’s explore some examples and comparative studies.
Examples of API Pagination Implementations
Several large-scale platforms have implemented API pagination successfully, demonstrating significant improvements in API efficiency and user satisfaction. For example:
- GitHub and LinkedIn APIs: Both platforms utilize cursor-based pagination to manage extensive data sets efficiently. By enabling users to fetch large amounts of data in an organized manner, these companies improve user experience and reduce server load.
- Amazon DynamoDB: Amazon uses key-based pagination for its DynamoDB database service, allowing developers to retrieve data seamlessly as the size of the dataset grows. This approach helps maintain high performance irrespective of data volume.
Comparison of Data Retrieval Efficiency with and Without Pagination
The impact of implementing pagination in APIs can be profound, particularly when comparing systems with and without it. Consider these aspects:
- Server Efficiency: Without pagination, servers must handle very large datasets in a single go, which can lead to increased load and slower response times. With pagination, data is broken into smaller, more manageable sets, greatly reducing server strain.
- Client Performance: Clients retrieving large datasets without pagination might experience timeouts or heavy memory usage, affecting application performance. Pagination simplifies data management on the client side, leading to smoother interactions.
- Data Freshness: In non-paginated APIs, data might become outdated by the time it is processed, especially if the dataset is large. Paginated APIs help ensure that data is more current and easier to sync regularly.
By reviewing these best practices and considering real-world case studies, developers can gain a strong understanding of the benefits and methodologies of API pagination, leading to more efficient, scalable, and user-friendly applications.
Tips for Implementing API Pagination in Projects
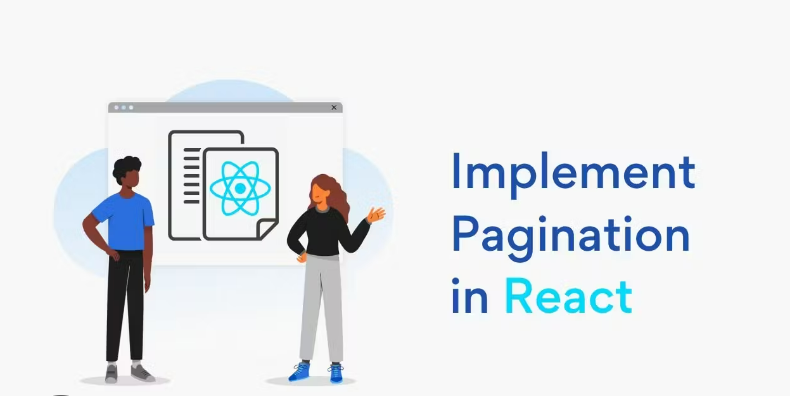
Effective API pagination is crucial for managing large datasets and improving the performance of web and mobile applications. Here are some detailed strategies to guide developers and IT professionals in implementing pagination efficiently.
Considerations for Integrating Pagination in API Design
When integrating pagination into an API, several factors must be considered to ensure it functions smoothly and efficiently:
- Data Structure: Design your API to handle data in a way that supports sequential access. Plan how data is fetched from the database and organized in the API response.
- Pagination Type: Decide between cursor-based and offset-based pagination depending on use case requirements. Cursor-based is more efficient for datasets that update frequently, while offset-based might be simpler to implement in environments where data does not change as often.
- Page Size: Setting the right page size is critical. Too large, and the server response time might increase; too small, and the client may end up making too many requests. A common approach is to allow the client to specify a page size, with a sensible default.
- API Versioning: When updates to the pagination logic are necessary, versioning the API helps in managing changes without disrupting the service for existing users.
- User Experience: Consider how pagination will affect the end user’s experience. Ensure the pagination logic is transparent enough for frontend applications to implement easily understandable navigation and data retrieval features.
Here are a few scenarios that outline specific approaches:
- For a news website with constantly updating content, cursor-based pagination could track changes dynamically, preventing any items from being skipped or duplicated.
- In a data analysis application where the same queries are run repeatedly, caching pages might improve performance significantly.
Overall, clear documentation for developers on how pagination is implemented and how to use it, including examples of requesting specific pages, is essential.
Testing and Optimizing Pagination Strategies for Performance
Once pagination is integrated, rigorous testing and optimization are crucial to ascertain performance and reliability. Here are some key focus areas:
- Load Testing: Simulate high traffic and large data requests to measure the performance of your pagination strategy and identify any bottlenecks in data retrieval.
- Response Times: Keep an eye on API response times as page number increases. Cursor-based pagination usually performs better because it does not require the server to count through a large number of rows in the database.
- Consistency Checks: Ensure that the pagination does not create overlaps or gaps in data as new data are added or existing records are deleted.
- Edge Case Handling: Test how the pagination handles requests at the boundaries of data; for example, how does it respond to a request for a page number that does overexcends the amount of available data?
- Client-Side Performance: Make sure the pagination logic does not degrade client-side performance or user experience.
By continuously monitoring these areas and making necessary adjustments, developers can help ensure that their API provides a robust, efficient, and user-friendly paging mechanism.
Future Trends in API Pagination
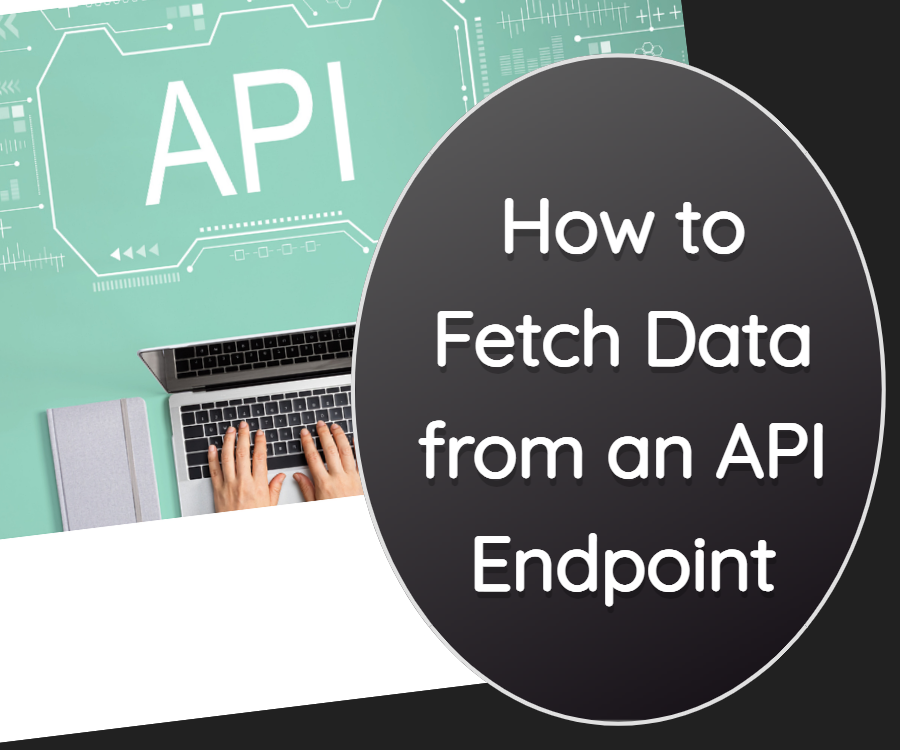
As technology and development practices evolve, so too do approaches to API pagination. These trends are likely to influence how pagination is implemented in future API designs:
- Increasing Use of Cursor-Based Pagination: Given its advantages in performance and accuracy, especially in real-time data environments, cursor-based pagination is expected to become more popular.
- VIP *Automated Query Optimization: Future frameworks and tools might come with built-in solutions to dynamically adjust query strategies based on real-time analytics and usage patterns.
- Integration with Machine Learning: The power of AI could be utilized to predict user behavior and dynamically adjust data loading strategies accordingly. This could reduce the load on servers by prefetching or caching data intelligently.
- Enhanced Client-Side Pagination: With advances in client-side render conty and storage, apps might handle larger payloads more effectively, balancing the load between the client and the server.
By staying informed about these trends and continuously adapting to new best practices, developers can ensure that their APIs stay efficient, scalable, and up-to-date. Understanding and implementing effective pagination strategies is more than just a technical requirement; it’s a continual commitment to user satisfaction and service excellence.
Conclusion
API pagination plays an integral role in managing data efficiently and ensuring an optimal user experience. By enabling the segmentation of large datasets into manageable chunks, it not only improves the speed and efficiency of data retrieval but also minimizes the load on both client and server resources. This essential methodology enhances the scalability of applications, making it an indispensable strategy in modern API design.
Through the use of pagination, developers can provide users with better control over data consumption, enabling them to navigate through large datasets with ease. This accessibility ensures that applications remain responsive and performant, devoid of the pitfalls associated with loading extensive amounts of data at once. As datasets grow continuously, the need for effective pagination mechanisms becomes even more crucial to maintain service reliability and user satisfaction.
Implementing pagination requires a thoughtful approach, considering various factors such as the default page size, the total count visibility, and the method of pagination. The choice between offset-based and cursor-based pagination methods impacts both the performance of data retrieval and the overall user experience. Best practices suggest leaning towards a method that not only fits the specific needs of your system but also anticipates future scaling challenges.
Moreover, when deploying pagination in your projects, it’s important to keep the API’s documentation clear and thorough. Proper documentation ensures that all potential users are well-informed about how to effectively interact with the API’s paging capabilities, thereby reducing confusion and avoiding errors in data handling.
Pingback: Token-Based Authentication: Secure Your API with JWT, OAuth