Introduction
Understanding how to manage your projects with Git is crucial for any developer. Git, a distributed version control system, enables multiple developers to work on the same project without conflicting changes. However, sometimes changes are made that need to be reversed or adjusted. Whether you’ve committed something accidentally, included incorrect files, or simply need to modify your last commit, knowing how to “uncommit” in Git is an essential skill. This guide will explain how to safely uncommit changes in Git, allowing you to maintain a clean and accurate project history. This ensures better collaboration and project management capabilities that can accommodate changes and mistakes efficiently.
What is “uncommit” in Git?
Image courtesy: Unsplash
Explanation of uncommit in Git
In the context of Git, a version control system extensively used in software development, the term “uncommit” isn’t an official command but a colloquial term used to describe actions that effectively undo previous commits. This capability is crucial because it allows developers to make adjustments to their project history in a controlled manner. It involves using several Git commands to either completely erase a commit, alter it, or create a new commit that undoes the changes made in a previous one. Since Git is designed to preserve a detailed history of project changes, the actions described as “uncommitting” are performed with commands crafted to ensure that the history and integrity of the project remain intact.
Why uncommit is important in version control
The ability to “uncommit” changes in a version control system like Git is fundamental. It provides developers the flexibility to manage and refine their code histories effectively. Mistakes are common in software development; developers may often commit changes that are incomplete, erroneous, or no longer needed. Additionally, in collaborative environments, revisions might be necessary when project requirements change, or when code reviews suggest improvements. “Uncommitting” helps maintain the stability of the software by allowing developers to rollback unwanted changes without disrupting the overall workflow. This fosters a more iterative, error-forgiving approach to project development, facilitating a cleaner, more organized commit history.
How to uncommit changes in Git
Using git reset command
The \`git reset\` command is one of the primary tools used to “uncommit” changes in Git. It enables developers to revert the repository to a previous state by resetting the current branch’s head to a specified commit, effectively discarding all commits up to that point:
1. Soft reset: To keep your working directory and the staging area intact, you can use the command \`git reset –soft HEAD~1\`. This command undoes the most recent commit and leaves the changes available in the staging area, allowing you to redo the commit with modifications, if necessary.
2. Mixed reset (default): By running \`git hidden reset\` \`HEAD~1\`, you uncommit the last commit and the changes from that commit are left in the working directory but unstaged. It gives you the opportunity to re-assess each file change individually before committing them again.
3. Hard reset: Use this with extreme caution. The command \`git reset –hard HEAD~1\` will not only uncommit your last commit but also discard all changes in the working directory. This is irreversible and should be used when you are certain that none of the recent changes are needed.
Each of these reset types serves different needs and provides control over how much of the commit history and work you want to preserve or discard.
Using git revert command
While \`git reset\` is effective for local branch management, \`git revert\` is designed to safely undo changes in a shared repository environment. The \`git revert\` command creates a new commit that reverses the changes made in a specified commit. This is crucial in collaborative projects as it doesn’t rewrite the project’s history, thus maintaining the integrity of the shared timeline and preventing conflicts:
– To revert the last committed changes, simply use \`git revert HEAD\`. This command will automatically create a new commit that undoes the changes introduced in the most recent commit.
– For reverting any specific commit, find the commit hash using \`git log\`, and then execute \`git revert [commit-hash]\`. Git will prompt you to edit the commit message for the new revert commit or you can accept the default message provided by Git.
This method is highly recommended for undoing changes on public branches where other developers are also making contributions, as it avoids the potential issues associated with altering published history.
Using git checkout command
The \`git checkout\` command can also be used to “uncommit” changes indirectly by switching branches or restoring working tree files to a former state:
– To discard changes in a specific file that has not yet been committed, you can use \`git checkout — [file-name]\`. This reverts the file back to the state of the last commit and is useful for quickly discarding erroneous changes made to a file.
– If you need to view your project at a particular point in the past or switch to a different version, use \`git checkout [commit-hash]\`. This will detach your HEAD, pointing it directly to a past commit. While in this detached state, you can browse the old version, test changes, or even base new work on this old version.
However, caution should be exercised as changing the HEAD without understanding the implications can lead to confusion and potential data loss if not correctly managed. Always ensure you know how to navigate back to your original branch (\`git checkout [your-branch-name]\`) before making any permanent changes.
By mastering these Git commands, developers gain a robust toolkit for managing their repositories effectively, ensuring that they can maintain project integrity and adapt to changes smoothly. In essence, knowing how to “uncommit” empowers developers to uphold an organized, accurate commit history, thus enhancing overall project quality and teamwork.
Best Practices for Uncommiting in Git
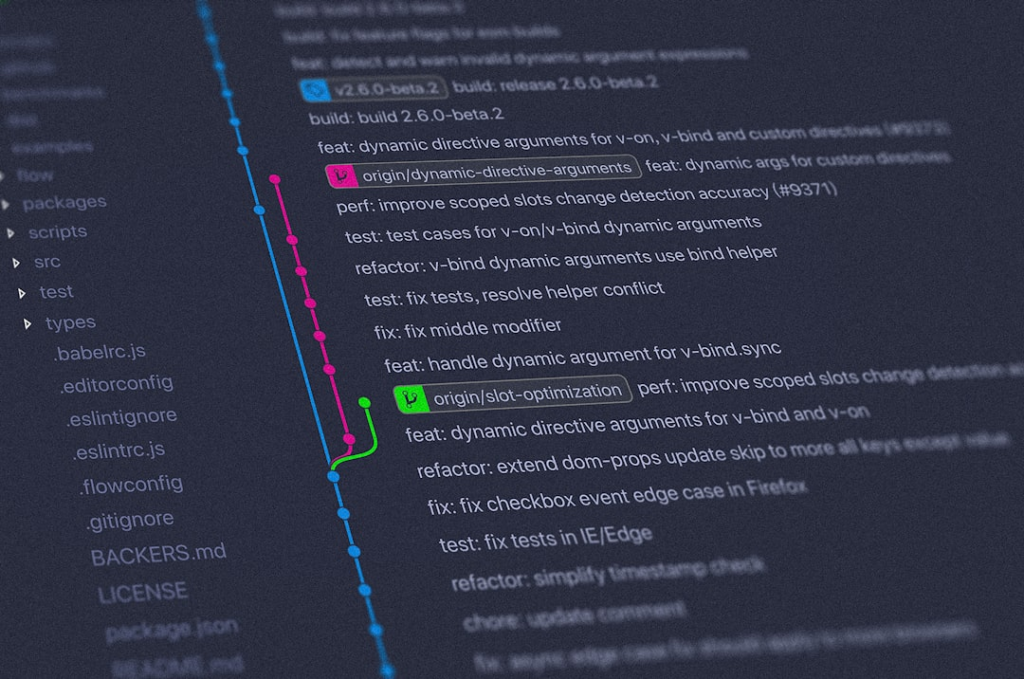
Commit Early, Commit Often
One of the key strategies for managing projects in Git is to commit early and often. This practice facilitates easier tracking of changes and pinpointing where things might have gone wrong, which simplifies the process of undoing mistakes. When you commit frequently, each commit is generally smaller and focused on a single aspect of the project. This granularity allows you to be more selective about what you choose to undo when you need to uncommit changes. If a particular commit introduces an error, you can revert just that small part without disturbing the rest of your work. Frequent commitments also make it easier to communicate changes to team members, as the commit messages provide a continual log of what has been updated and why.
Stashing Changes Before Uncommitting
Before uncommitting changes in Git, it’s prudent to use the \`git stash\` command to safely set aside the current working directory and the index. This is particularly useful if you realize that you need to revert a commit but also want to retain some of the uncommitted work you’ve started on. Stashing takes your modifications and saves them in a stack-like structure where they can be reapplied at a later time. Here are a few steps to effectively stash your changes:
– Save the Stash: Use \`git stash save “message”\` to stash your changes with a descriptive message about what was changed. This message is helpful when you review your stashes later.
– List Stashes: You can list all stashed changes with \`A git stash list\` to see what stashes you have stored.
– Apply a Stash: When you are ready to bring the stashed changes back to your working directory, use \`git stash apply\` if you want to keep the stash, or \`git stash pop\` to apply and remove the stash from the stored list.
Using stashes can help you manage your work efficiently, ensuring that no valuable changes are lost while correcting or modifying past commits.
Communicating with Team Members About Uncommit Actions
When working in a team, effective communication is critical, especially when undoing changes that could affect the shared repository. Before uncommitting, ensure you discuss the planned actions with team members who might be impacted. Use channels like team meetings, emails, or chat tools to explain why the revert is necessary and what it entails.
Best practices include:
– Documenting the Reason: Always document the reasons for reverting a commit in the commit message.
– Reviewing as a Team: If possible, review the changes that are to be uncommitted as a team, to ensure everyone agrees and understands the implications.
– Alerting Before Pushing: Once you’ve locally uncommitted changes, notify your team before pushing these changes to the remote repository. This allows fellow developers to adjust their work accordingly.
Clear communication helps in maintaining harmony in the team and ensures that each member is aware of the repository’s state, preventing conflicts and fostering a more collaborative environment.
Common Mistakes to Avoid When Uncommitting in Git
Forgetting to Backup Changes
One of the most common mistakes when uncommitting changes in Git is failing to backup those changes before they are undone. It’s essential to ensure that there is a safeguard against permanent loss of data. Here are a few strategies to consider:
– Create Patches: Before undoing commits, create patches using \`git format-patch\`. This allows you to save the commits as files which can be reapplied if needed.
– Use Branches: Consider creating a new branch before uncommitting. This can serve as a backup, allowing you to switch back if you realize that the uncommit was a mistake.
Backing up ensures that even if you misjudge the effects of an uncommit, you can still recover your work without losing significant progress.
Uncommitting Without Understanding Consequences
Undoing commits without fully understanding the impact on your project can lead to significant problems, including loss of data, introduction of bugs, and disruption of the project timeline. Before uncommitting, take the time to:
– Review the Commit History: Analyze the commit history thoroughly to understand what changes are being undone.
– Test Changes Locally: Before pushing uncommitted changes to the repository, test your changes locally. This ensures that the uncommit does not introduce new errors into the codebase.
– Consult with Peers: If unsure, consult with a colleague or a mentor. Getting a second opinion can help you avoid unnecessary mistakes.
By being mindful of these considerations, you can avoid common pitfalls associated with uncommitting in Git, preserving the integrity and history of your project while maintaining its progress towards development goals. Incorporating these practices into daily use of Git enhances not only personal efficiency but also the collaborative success of the team.
Conclusion
Revisiting the basic functionalities of Git, especially around undoing changes, is crucial for maintaining the integrity and history of code in a project. The ability to uncommit in Git allows developers at all levels to remedy errors quickly, adjust their approach, or revert to previous versions without losing overall track of the project’s progression.
One of the most significant advantages of mastering the \`git reset\` and \`git revert\` commands is the flexibility and safety they introduce to project management. For beginners, understanding the difference between these commands and when to use each can significantly enhance their workflow efficiency. To summarize:
– Using \`git reset\`: This command is powerful and versatile, perfect for local branches and solo projects where a private history edit is acceptable. It allows you to discard commits in your local repository by moving the HEAD to a previous commit. However, caution is necessary as it can permanently erase commits, making it unsuitable for shared branches.
– Utilizing \`git revert\`: For changes that need to be undone safely in a shared environment, \`git revert\` provides an undo functionality that doesn’t interfere with the project history. This makes it ideal for public or shared repositories, where maintaining an accurate and traceable history is crucial.
By following these guidelines, you can avoid common pitfalls that might disrupt your project or complicate collaboration with others. Additionally, harnessing the power of version control with Git involves regular practice and continuous learning. Here are some final tips to consolidate your understanding and skills in managing repositories:
– Commit Regularly: By committing often, you create a detailed history of changes, which makes pinpointing where things went wrong much easier. It also simplifies the process of reverting to a specific part of the project.
– Push with Caution: Before pushing changes to a shared repository, double-check your commits. Once changes are shared, undoing them requires more careful handling to ensure team synchronization.
– Leverage Branches: Make use of branching to experiment with new features without affecting the main project. This approach not only keeps your main project safe but also makes merging tested changes more straightforward.
– Stay Updated: Keep learning about new features and best practices in Git. The version control system is continually evolving, and staying updated can provide you with tools and techniques to manage your repositories more efficiently.
– Practice: Like any other skill, proficiency in Git comes with practice. Try to use Git in personal projects, or contribute to open-source projects to refine your skills.
By grounding your Git practices in these principles and strategies, you’ll enhance both the quality and the manageability of your software development projects. Whether you’re working alone or as part of a team, the ability to uncommit and revert changes strategically in Git ensures that your revision control process is as error-free and robust as possible. Remember, effective version control is a cornerstone of successful software development, setting a strong foundation for continued growth and collaboration.
FAQ
Image courtesy: Unsplash
What does uncommit mean in Git?
Uncommitting in Git refers to the process of undoing the last commit that you made. This doesn’t delete the changes you made; it simply removes them from the commit history and moves them back into your working directory as modified files, allowing you to either edit them further, commit them again, or even discard them if you choose.
How do I uncommit multiple commits in Git?
To uncommit multiple commits in Git, you can use the command \`git reset –soft HEAD~[number]\` where \`[number]\` is replaced with the number of commits you wish to undo. For example, if you want to uncommit the last three commits, you would use \`git reset –soft HEAD~3\`. This will move the last three commits back into your working directory.
Is uncommitting safe in Git?
Uncommitting is generally safe as it does not delete your code or files. It merely undoes commits and brings the changes back to your working area. However, if you’re working in a shared repository, you should be cautious about uncommitting changes that have already been pushed to remote branches as it could affect other people’s work.
Can I uncommit a pushed commit?
Yes, you can uncommit a commit that has been pushed to a remote repository, but this should be done with care. Use \`git push –force\` after uncommitting if you absolutely must modify the history of a public branch. It’s crucial to communicate with your team when performing such actions as it can overwrite changes made by others.