- What Will You Learn?
- Key Takeaways
- Access Tokens
- Refresh Tokens
- Signup Workflow
- Login Workflow
- Local Storage: Convenience with Risks
- Cookies: The Secure Alternative
- 5. Implementing Token Rotation
- Authentication Routes Setup
- Frontend with React
- Token Management Hook
- Best Security Practices
- Testing Tools and Strategies
- Industry Giants' Approaches
Authentication is at the heart of modern web applications, ensuring only authorized users access specific resources. Token-based systems, especially with access and refresh tokens, are among the most secure and scalable solutions. However, the security of these systems can be compromised without proper management, such as token rotation.
In this blog, we’ll dive deep into token rotation, its role in secure authentication workflows, and a practical implementation with React and Node.js.
What Will You Learn?
By the end of this guide, you’ll understand:
- What tokens actually are
- Why they’re important
- How to keep them super secure
- How to protect your app from sneaky hackers
Why Token Rotation Matters
Imagine your authentication system as a fortress. Traditional static tokens are like fixed locks that remain unchanged, potentially vulnerable to sophisticated attacks. Token rotation introduces a dynamic defense mechanism, continuously changing the locks to prevent unauthorized access.
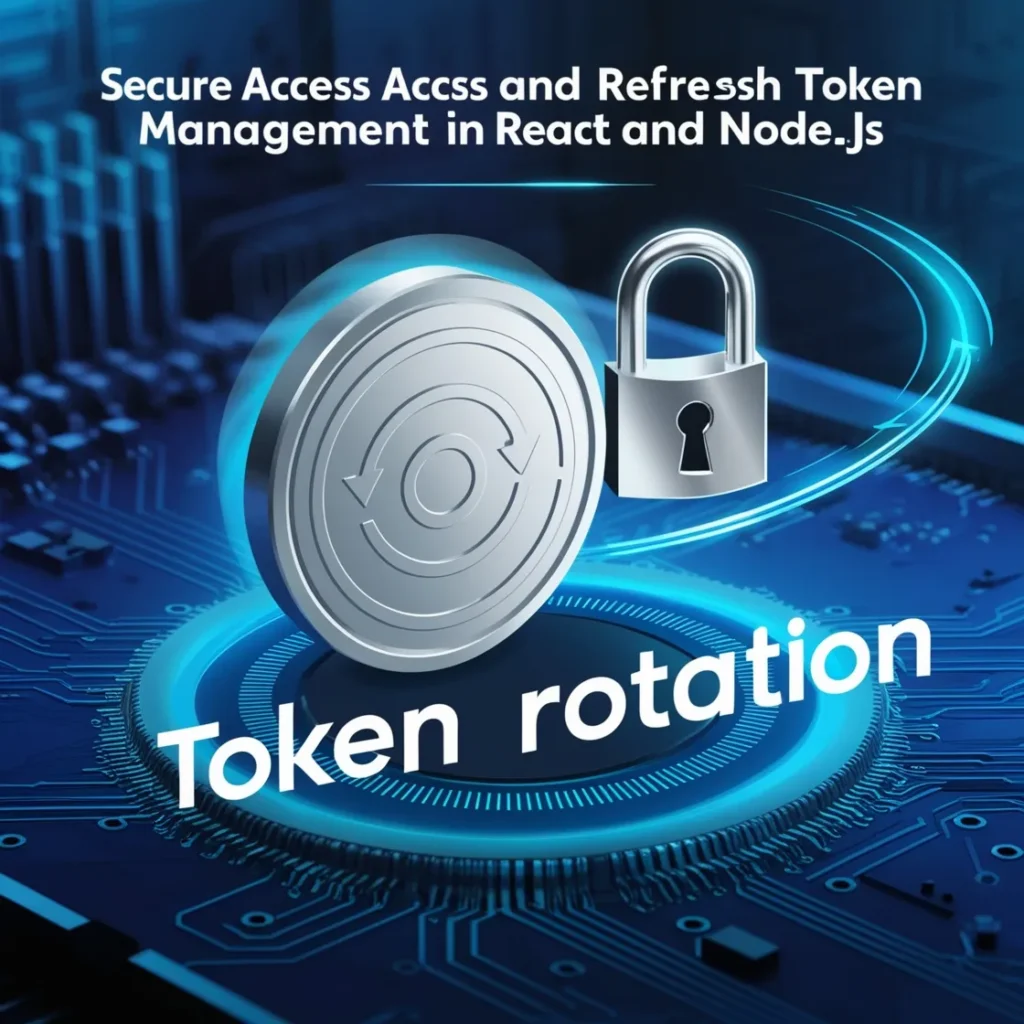
Key Takeaways
By the end of this comprehensive guide, you’ll understand:
- The fundamental concepts of access and refresh tokens
- How to implement secure token rotation in React and Node.js
- Best practices for token management and security
- Real-world strategies used by leading tech companies
2. Understanding Tokens
What are Access and Refresh Tokens?
Access Tokens
An access token is a short-lived credential that grants a user permission to access specific resources. Think of it as a temporary pass that expires quickly, minimizing the window of potential security breaches.
Key Characteristics:
- Typically short-lived (minutes to hours)
- Contain user identity and permission information
- Used for authenticating API requests
- Should be kept secure and rotated frequently
Refresh Tokens
A refresh token is a long-lived credential used to obtain new access tokens without requiring the user to re-authenticate. It’s like a master key that can generate new temporary passes.
Key Characteristics:
- Long-lived (days to weeks)
- Stored securely on the server
- Used to request new access tokens when the current one expires
- Rotated periodically to enhance security
Why Use Token Rotation?
Token rotation isn’t just a fancy security technique—it’s a critical defense mechanism against potential attacks:
- Minimize Exposure Window: By frequently changing tokens, you reduce the time an attacker has to exploit a stolen token.
- Limit Damage from Token Compromise: If a token is compromised, its short lifespan and automatic rotation limit potential damage.
- Adaptive Security: Rotation allows dynamic response to potential security threats.
3. Workflow of Token Rotation in Login and Signup
Signup Workflow
When a user registers, the authentication system generates two critical tokens:
async function signup(username, password) {
// 1. Validate user input
// 2. Hash password
// 3. Create user in database
const accessToken = generateAccessToken(user);
const refreshToken = generateRefreshToken(user);
// Store refresh token securely
await storeRefreshToken(user.id, refreshToken);
return { accessToken, refreshToken };
}
Login Workflow
During login, the system verifies credentials and issues fresh tokens:
async function login(username, password) {
// 1. Verify user credentials
// 2. Generate new access and refresh tokens
const accessToken = generateAccessToken(user);
const newRefreshToken = generateRefreshToken(user);
// Invalidate previous refresh token
await invalidatePreviousRefreshToken(user.id);
// Store new refresh token
await storeRefreshToken(user.id, newRefreshToken);
return { accessToken, refreshToken: newRefreshToken };
}
4. Token Storage: Local Storage vs. Cookies
Local Storage: Convenience with Risks
Pros:
- Easy to implement
- Works across different domains
- Simple JavaScript API
Cons:
- Vulnerable to XSS attacks
- No built-in HTTP security
- Accessible by any script on the page
Cookies: The Secure Alternative
Pros:
- HttpOnly attribute prevents client-side script access
- Secure flag ensures transmission only over HTTPS
- Built-in CSRF protection mechanisms
- More secure by default
Recommended Cookie Configuration:
res.cookie('refreshToken', token, {
httpOnly: true, // Prevent JavaScript access
secure: true, // HTTPS only
sameSite: 'strict', // CSRF protection
maxAge: 7 * 24 * 60 * 60 * 1000 // 7 days
});
5. Implementing Token Rotation
Backend with Node.js
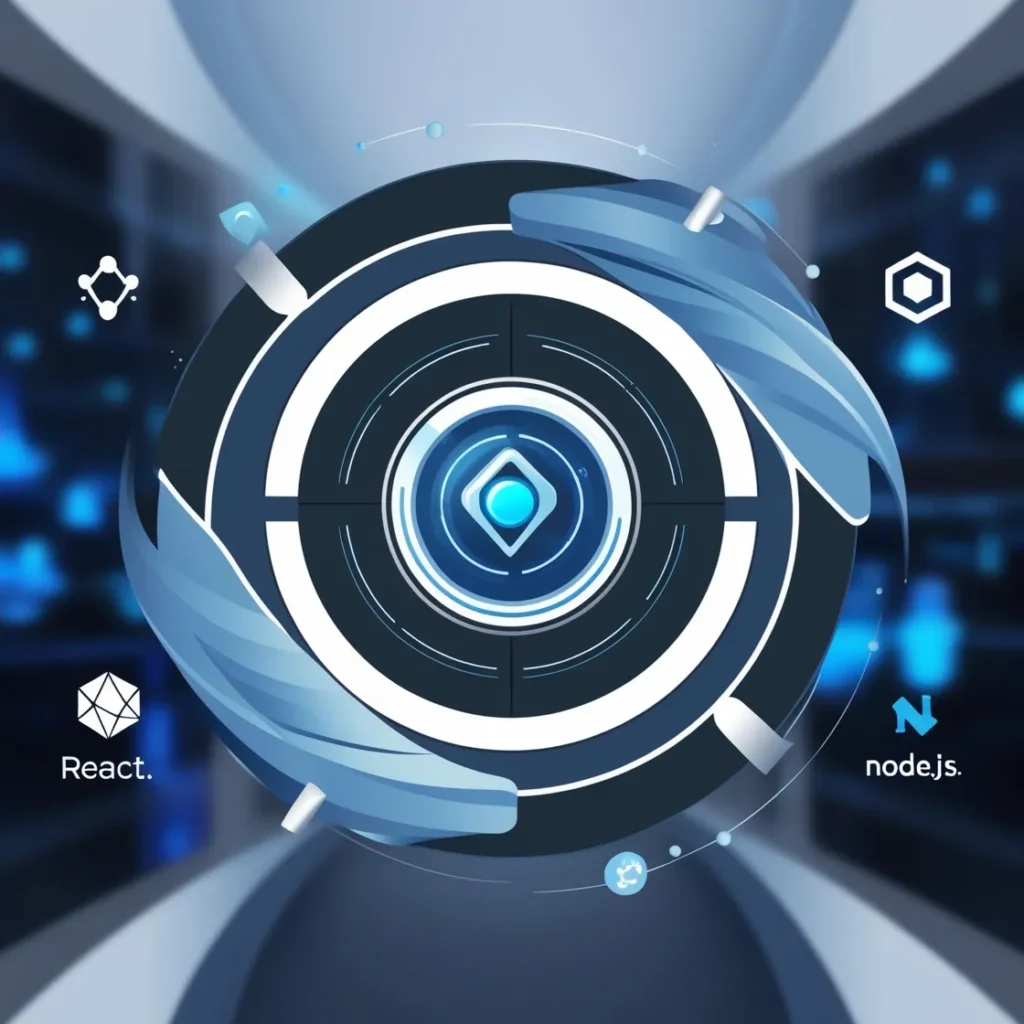
Authentication Routes Setup
const express = require('express');
const jwt = require('jsonwebtoken');
// Signup Route
router.post('/signup', async (req, res) => {
const { username, password } = req.body;
const user = await createUser(username, password);
const accessToken = generateAccessToken(user);
const refreshToken = generateRefreshToken(user);
// Store refresh token securely
await storeRefreshToken(user.id, refreshToken);
res.json({ accessToken, refreshToken });
});
// Token Refresh Route
router.post('/token', async (req, res) => {
const { refreshToken } = req.body;
// Validate refresh token
const user = await validateRefreshToken(refreshToken);
// Invalidate old refresh token
await invalidateRefreshToken(refreshToken);
// Generate new tokens
const newAccessToken = generateAccessToken(user);
const newRefreshToken = generateRefreshToken(user);
// Store new refresh token
await storeRefreshToken(user.id, newRefreshToken);
res.json({
accessToken: newAccessToken,
refreshToken: newRefreshToken
});
});
Frontend with React
Token Management Hook
import axios from 'axios';
function useTokenManager() {
const [tokens, setTokens] = useState({
accessToken: null,
refreshToken: null
});
// Axios interceptor for automatic token refresh
useEffect(() => {
const interceptor = axios.interceptors.response.use(
(response) => response,
async (error) => {
const originalRequest = error.config;
// Check if token expired and refresh is needed
if (error.response.status === 401 && !originalRequest._retry) {
originalRequest._retry = true;
try {
const { accessToken, refreshToken } = await refreshTokens(tokens.refreshToken);
setTokens({ accessToken, refreshToken });
// Retry original request with new token
originalRequest.headers['Authorization'] = `Bearer ${accessToken}`;
return axios(originalRequest);
} catch (refreshError) {
// Logout user if refresh fails
logout();
}
}
return Promise.reject(error);
}
);
return () => axios.interceptors.response.eject(interceptor);
}, [tokens]);
return { tokens, setTokens };
}
6. Securing Your Token System
Best Security Practices
- Always Use HTTPS
Ensure all token transmissions occur over encrypted connections. - Implement Rate Limiting
Prevent brute-force attacks on token refresh endpoints. - Token Blacklisting
Maintain a server-side list of invalidated tokens. - Short Token Lifespans
Keep access tokens short-lived (15-30 minutes)
Keep refresh tokens moderately long-lived (7-14 days)
7. Testing the Implementation
Testing Tools and Strategies
- Postman: Simulate token expiration and refresh
- Jest/Mocha: Unit testing token generation
- Simulation Scenarios:
- Token expiration
- Concurrent refresh requests
- Token theft prevention
8. Real-World Use Cases
Industry Giants’ Approaches
- Google: Rolling refresh tokens with device-specific tracking
- Facebook: Multiple token types for different access levels
- GitHub: Short-lived tokens with granular permissions
9. Conclusion
Token rotation isn’t just a security feature—it’s a fundamental requirement in modern web applications. By implementing robust token management, you create a dynamic, resilient authentication system that adapts to potential threats.
Call to Action: Start implementing token rotation in your next project. Your users’ security depends on it!