Node.js is an open-source, cross-platform JavaScript runtime environment that allows you to build server-side and networking applications. It’s especially popular for its efficiency and being fully asynchronous, non-blocking, and event-driven. Installing Node.js on Ubuntu 16.09 is straightforward and can significantly enhance your development environment. This guide offers a comprehensive, step-by-step walkthrough to help you install Node.js on an Ubuntu 16.04 system, ensuring that even beginners can follow along without trouble. Whether you’re setting up a personal project or a professional development environment, getting Node.js installed correctly is an essential step. Let’s dive into the process.
Overview of Node.js and Ubuntu 16.04
Image courtesy: Unsplash
Brief introduction to Node.js in System
Node.js is a powerful JavaScript runtime built on Chrome’s V8 JavaScript engine. It enables developers to build scalable and efficient network applications that can handle numerous simultaneous connections with high throughput. Node.js uses an event-driven, non-blocking I/O model that makes it lightweight and efficient, perfect for data-intensive real-time applications that run across distributed devices. Its package ecosystem, npm, is the largest ecosystem of open source libraries in the world, making Node.js incredibly versatile for web development.
Why Ubuntu 16.04 is a popular for Node.js installations
Ubuntu 16.04 LTS, also known as Xenial Xerus, is a widely-used Linux distribution that provides a stable, secure, and highly customizable operating environment. Itโs favored by developers due to its long-term support (LTS), which guarantees five years of security and maintenance updates. This reliability makes Ubuntu 16.04 an excellent platform for hosting applications, including those built with Node.js. Additionally, Ubuntu’s robust community support and extensive documentation facilitate troubleshooting and ensure a smooth setup process for software and application development.
Preparing Your Ubuntu 16.04 Server
Before installing Node.js, it’s essential to properly set up and prepare your Ubuntu server. This preparation ensures that the server possesses all the necessary tools and packages required for a smooth and successful Node.js installation.
Updating the package index
The first step in preparing your Ubuntu 16.04 server is to update the package index.This ensures that you have the latest updates and security patches that can help improve the performance and security of your system. To update the package index, you will need access to the terminal. Follow these steps:
1. Open your terminal.
2. Execute the update command:
sudo apt-get update
This command makes your system fetch fresh lists of packages from all repositories and PPAs. The output you see will list packages that are going to be upgraded. It is crucial to ensure that this process completes without errors to proceed with the installation.
Installing build-essential package
After updating the package index, the next step is to install the ‘build-essential’ package. This package contains an informational list of packages which are considered essential for building Debian packages. This includes gcc compiler, make and other required tools. To install build-essential, run the following command:
1. Open your terminal.
2. Type the following command and press Enter:
sudo apt-get install build-essential
The installation may prompt you to confirm the download and installation; simply type ‘Y’ for yes when prompted. Installing build-essential is important as it not only allows you to compile and install software from source but also ensures that you have all necessary tools for building Node.js native addons, which are sometimes required for certain npm packages.
By following these steps, your Ubuntu 16.04 server will be well-prepared for a smooth and efficient Node.js installation. Proper server preparation not only facilitates a more reliable setup but also reduces potential issues down the line, promoting a better overall performance and experience with Node.js applications.
Installing Node.js
Using the official Ubuntu repository
Installing Node.js on Ubuntu 16.04 can be performed using the official Ubuntu repository. This method ensures that you install Node.js through a straightforward process that Ubuntu manages and maintains. To begin, you need to open your terminal. You can do this by pressing \`Ctrl + Alt + T\` on your keyboard. Once the terminal window is open, you should update your package list to ensure you get the latest version of the repository listings:
sudo apt-get update
After updating the package list, you can install Node.js. Ubuntu 16.04 typically contains a version of Node.js in its default repositories, but it may not be the latest version. To install Node.js, execute:
sudo apt-get install nodejs
This command installs Node.js and also ensures that all required packages are set up correctly. If you need to compile or build any Node.js packages from source, you might also need to install \`build-essential\`. To install it, run:
sudo apt-get install build-essential
Verifying the installation
Once the installation process is complete, it’s a good practice to verify that Node.js has been installed correctly and is functioning as expected. To check if Node.js is installed and to find out the version that has been installed, type the following command in your terminal:
node -v
This command returns the version of Node.js that is currently installed on your system. For example, you might see a response like \`v4.2.6\`, which indicates that version 4.2.6 of Node.js is installed. If you receive an error or if the version seems outdated, you may want to consider installing a newer version of Node.js using a version manager such as nvm.
Understanding Package Managers for Node.js
NPM and its role in managing Node.js packages
Node Package Manager (npm) is Node.js’s default package manager and one of the worldโs largest software registries. npm is used to install, share, and manage library dependencies in Node.js environments. npm makes it easy for JavaScript developers to share the code that they have created to solve particular problems, and for others to reuse that code in their own applications. npm consists of three distinct components:
- the website to engage with various packages
- the Command Line Interface (CLI) to interact with npm on your computer
- the registry to access a large public database of JavaScript software and metadata
Each Node.js project has a \`package.json\` file which lists the project dependencies. When you run the \`npm install\` command, npm looks at this file, downloads the listed packages from the registry, and installs them into the \`node_modules\` directory of the project.
Installing npm and checking its version
On Ubuntu 16.04, npm is not bundled with the Node.js installation from the default repositories. Therefore, you will need to install it separately. You can install npm by entering the following command in your terminal:
sudo apt-get install npm
This command installs npm on your Ubuntu system. Following the installation, it’s a good idea to verify that npm is installed correctly and determine which version you have. To check the version of npm installed, type:
npm -v
This will display the current version number of npm installed on your machine. For instance, you might see something like \`3.5.2\`. Knowing the version is essential for troubleshooting and for understanding whether you meet the requirements of various Node.js packages you may want to use.
Setting Up a Simple Node.js Application
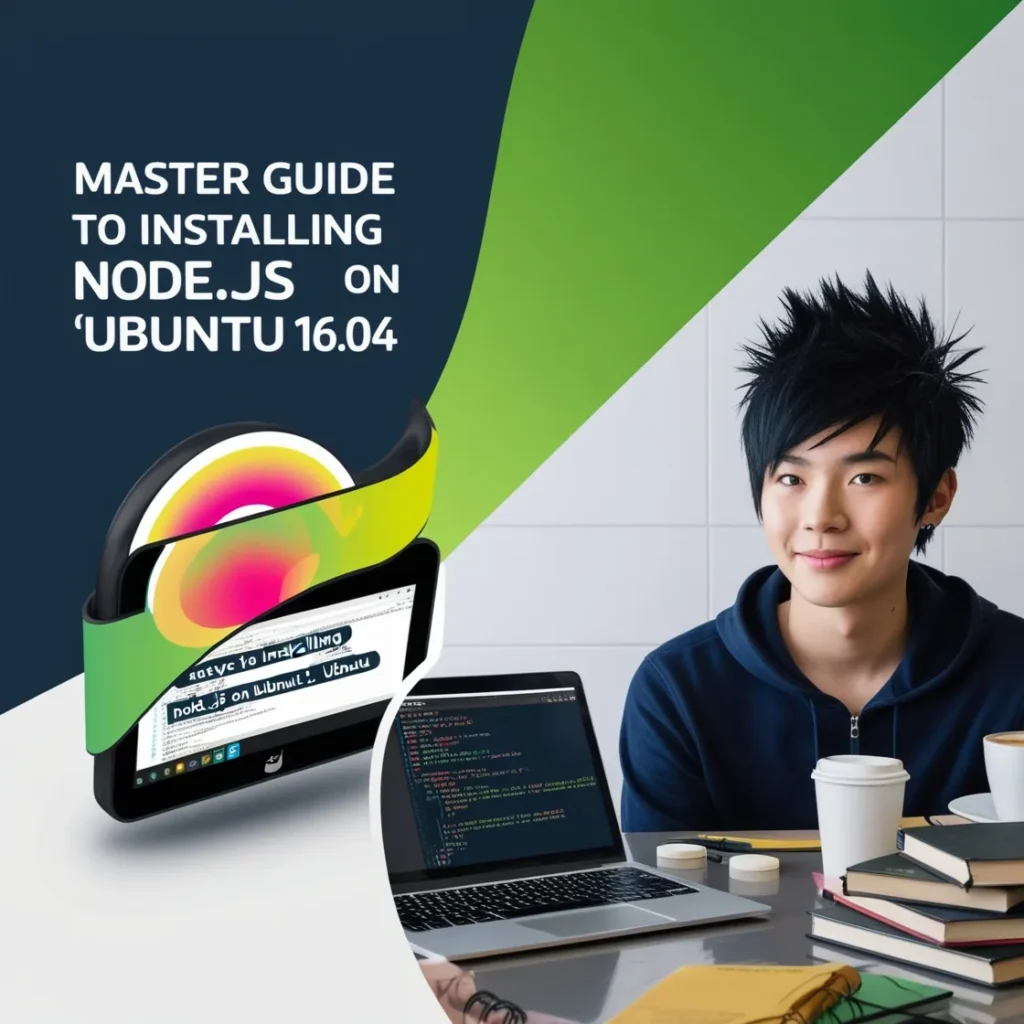
Creating a Basic Server with Node.js
Once Node.js is installed on your Ubuntu 16.04 system, you are ready to create a basic server. This process involves writing a simple script that defines how your server will handle HTTP requests. Start by creating a new directory for your project and navigate into it using the terminal. You can do this with the following commands:
mkdir my-node-project
cd my-node-project
Next, create a new file named \`server.js\`. You can use \`nano\` or any text editor of your choice:
nano server.js
In the \`server.js\` file, write the following JavaScript code:
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World, Welcome to Node.js!');
});
const port = 3000;
server.listen(port, () => {
console.log(\`Server running at http://localhost:${port}/\`);
});
This script imports Node.js’s native HTTP module, uses it to create a server that listens on port 3000, and sends a plain text response ‘Hello World, Welcome to Node.js!’ to any HTTP requests. Save and close the file when you’re finished.
Running the Node.js Application
To run the application you just created, you will use Node.js to execute the \`server.js\` script. Ensure you are in the root directory of your project (where your \`server.js\` is located) and type the following command in your terminal:
node server.js
This will start the server on port 3000, and you should see a message in the terminal saying “Server running at http://localhost:3000/”. This indicates that your Node.js server is up and running and ready to handle requests.
Accessing the Application in a Web Browser
Now that your server is running, open a web browser and enter the following URL:
http://localhost:3000/
This directs the browser to connect to your newly created server. You should see the text ‘Hello World, Welcome to Node.js!’ displayed. This verifies that your server is handling requests properly and sending the correct response back to the browser. Congratulations, you have just set up a basic Node.js server on Ubuntu 16.04!
Conclusion
Recap of the installation process
In this guide, we walked through the step-by-step process of installing Node.js on Ubuntu 16.04. The installation involved updating the package repository with the command \`sudo apt-get update\`, installing Node.js using \`sudo apt-get install nodejs\`, and ensuring npm is installed with \`sudo apt-get install npm\`. These commands help you set up Node.js and its package manager, npm, preparing your Ubuntu system for running Node.js applications.
Final thoughts on using Node.js on Ubuntu 16.04
Using Node.js on Ubuntu 16.04 provides a stable and efficient environment for developing server-side applications. It’s beneficial for real-time data-intensive applications that run across distributed devices. Moreover, Ubuntu’s robustness combined with Node.js’s lightweight framework allows developers to create scalable and performant applications. Remember to keep both Node.js and Ubuntu updated to maintain security and efficiency in your development projects.
Pingback: Optimizing Node.js Performance on Ubuntu 16.04 for Better Development