Table of Contents
Introduction
In today’s rapidly evolving software landscape, microservices architecture has become increasingly popular due to its scalability, flexibility, and maintainability. As developers seek efficient tools to build these distributed systems, NestJS has emerged as a powerful framework for creating robust microservices. This article will explore the world of building microservices with NestJS, comparing it with Express, and providing practical examples to help you get start
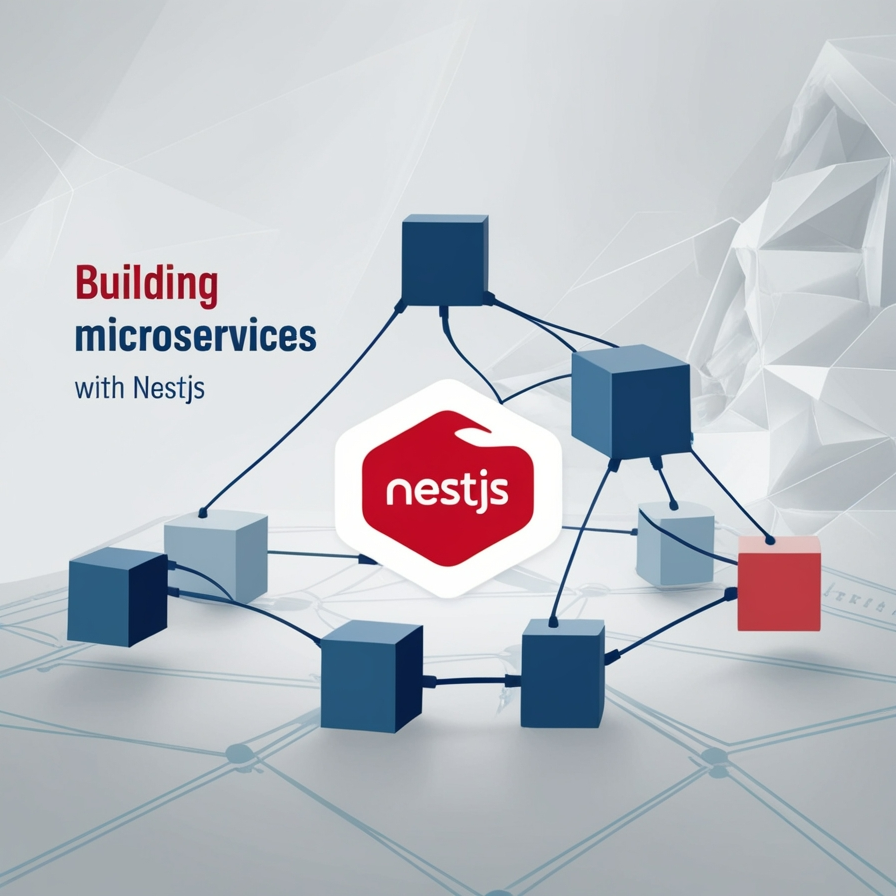
Understanding Microservices
Microservices architecture is an approach to developing software systems that focuses on building small, independent services that work together to form a larger application. Each microservice is responsible for a specific business capability and can be developed, deployed, and scaled independently.
Key benefits of microservices include:
- Scalability: Services can be scaled independently based on demand.
- Flexibility: Different technologies can be used for different services.
- Resilience: Failure in one service doesn’t necessarily affect the entire system.
- Ease of deployment: Smaller codebases are easier to deploy and maintain.
- Team autonomy: Different teams can work on different services independently.
While microservices offer numerous advantages, they also introduce complexities in terms of communication, data consistency, and deployment. This is where frameworks like NestJS come in, providing tools and structure to manage these challenges effectively.
Introduction to NestJS
NestJS is a progressive Node.js framework for building efficient, reliable, and scalable server-side applications. It leverages TypeScript and combines elements of Object-Oriented Programming (OOP), Functional Programming (FP), and Functional Reactive Programming (FRP).
Key features of NestJS include:
- Dependency Injection: NestJS has a built-in dependency injection container, making it easy to manage dependencies and promote loose coupling.
- Decorators: Leveraging TypeScript decorators, NestJS provides a clean and expressive way to define routes, middleware, and other application components.
- Modules: NestJS encourages a modular architecture, allowing you to organize your code into cohesive, reusable modules.
- TypeScript support: Built with and fully supporting TypeScript, NestJS provides strong typing and enhanced tooling support.
- Microservices support: NestJS has first-class support for building microservices, including various transport layer protocols.
These features make NestJS an excellent choice for building microservices, as it provides a structured and scalable approach to developing distributed systems.
NestJS vs Express: A Comparison
When considering NestJS for microservices, it’s natural to compare it with Express, another popular Node.js framework. While NestJS is built on top of Express (by default), it provides additional features and structure that make it particularly well-suited for building microservices.
Here’s a comparison of NestJS and Express:
- Architecture:
- Express: Minimalist and unopinionated, providing basic routing and middleware support.
- NestJS: Opinionated framework with a modular architecture, built-in dependency injection, and decorators.
- TypeScript support:
- Express: Requires additional setup and typings for TypeScript support.
- NestJS: Built with TypeScript, providing out-of-the-box support and enhanced type safety.
- Scalability:
- Express: Scalable, but requires manual organization and structure for large applications.
- NestJS: Designed for scalability with its modular architecture and built-in support for microservices.
- Learning curve:
- Express: Easier to learn due to its simplicity and minimal abstractions.
- NestJS: Steeper learning curve but provides more structure and features for complex applications.
- Microservices support:
- Express: Requires additional libraries and manual setup for microservices architecture.
- NestJS: Offers built-in support for microservices, including various transport layer protocols.
- Testing:
- Express: Requires additional setup and libraries for comprehensive testing.
- NestJS: Provides built-in testing utilities and easy-to-use testing modules.
- Documentation:
- Express: Extensive community-driven documentation and resources.
- NestJS: Comprehensive official documentation with detailed guides and examples.
While Express is an excellent choice for simple applications and APIs, NestJS shines when it comes to building complex, scalable microservices. Its opinionated structure, built-in features, and first-class support for microservices make it a powerful tool for developing distributed systems.
Setting Up a NestJS Microservices Project
To get started with building microservices using NestJS, you’ll need to set up your development environment and create a new NestJS project. Follow these steps to get up and running:
- Install Node.js and npm (Node Package Manager) if you haven’t already.
- Install the NestJS CLI globally:
npm i -g @nestjs/cli
- Create a new NestJS project:
nest new nestjs-microservices
- Navigate to the project directory:
cd nestjs-microservices
- Install additional dependencies for microservices:
npm i @nestjs/microservices
Now that you have your NestJS project set up, let’s examine the project structure:
nestjs-microservices/
โโโ src/
โ โโโ app.controller.spec.ts
โ โโโ app.controller.ts
โ โโโ app.module.ts
โ โโโ app.service.ts
โ โโโ main.ts
โโโ test/
โ โโโ app.e2e-spec.ts
โ โโโ jest-e2e.json
โโโ nest-cli.json
โโโ package.json
โโโ tsconfig.build.json
โโโ tsconfig.json
โโโ README.md
This structure provides a solid foundation for building your microservices. The src
directory contains your application code, while the test
directory is for your end-to-end tests.
Building Your First NestJS Microservice
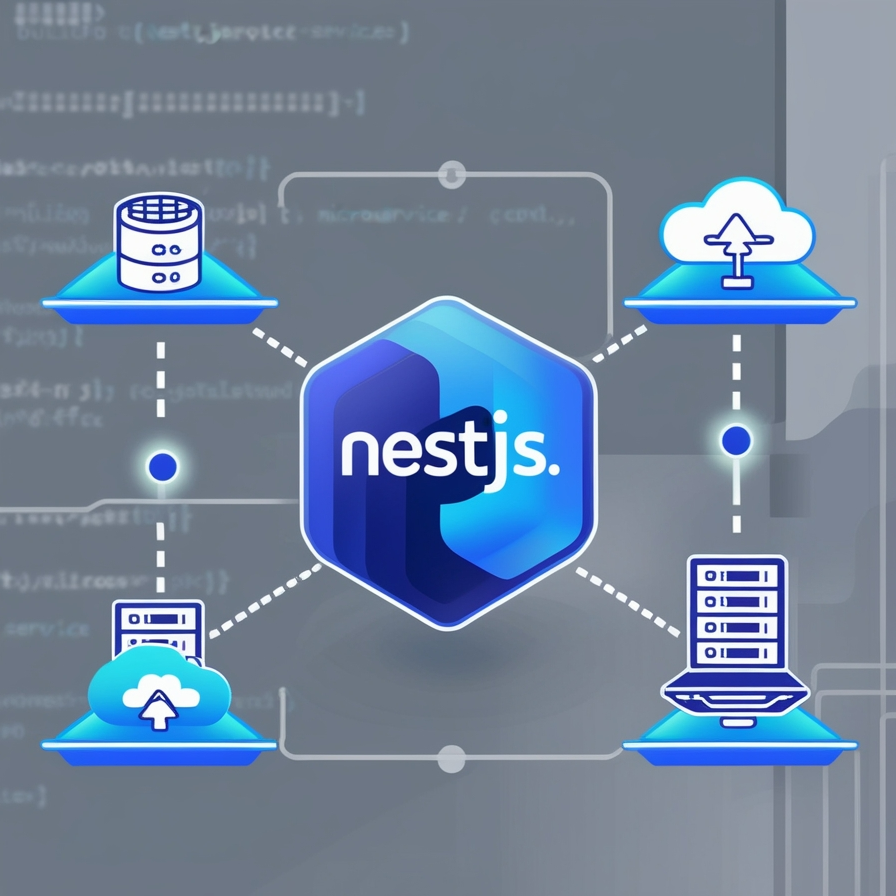
Let’s create a simple microservice that manages a list of users. We’ll start by creating a new module, controller, and service for our user microservice.
- Generate a new module:
nest generate module users
- Generate a controller:
nest generate controller users
- Generate a service:
nest generate service users
Now, let’s implement our user microservice:
// src/users/users.service.ts
import { Injectable } from '@nestjs/common';
@Injectable()
export class UsersService {
private users = [
{ id: 1, name: 'John Doe', email: 'john@example.com' },
{ id: 2, name: 'Jane Smith', email: 'jane@example.com' },
];
findAll() {
return this.users;
}
findOne(id: number) {
return this.users.find(user => user.id === id);
}
create(user: { name: string; email: string }) {
const newUser = { id: this.users.length + 1, ...user };
this.users.push(newUser);
return newUser;
}
}
// src/users/users.controller.ts
import { Controller } from '@nestjs/common';
import { MessagePattern } from '@nestjs/microservices';
import { UsersService } from './users.service';
@Controller()
export class UsersController {
constructor(private readonly usersService: UsersService) {}
@MessagePattern({ cmd: 'findAllUsers' })
findAll() {
return this.usersService.findAll();
}
@MessagePattern({ cmd: 'findOneUser' })
findOne(id: number) {
return this.usersService.findOne(id);
}
@MessagePattern({ cmd: 'createUser' })
create(user: { name: string; email: string }) {
return this.usersService.create(user);
}
}
In this example, we’ve created a simple user microservice with methods to find all users, find a single user, and create a new user. The @MessagePattern
decorator is used to define message patterns that the microservice will respond to.
To run this microservice, we need to update our main.ts
file:
// src/main.ts
import { NestFactory } from '@nestjs/core';
import { MicroserviceOptions, Transport } from '@nestjs/microservices';
import { AppModule } from './app.module';
async function bootstrap() {
const app = await NestFactory.createMicroservice<MicroserviceOptions>(
AppModule,
{
transport: Transport.TCP,
options: {
host: '127.0.0.1',
port: 8877,
},
},
);
await app.listen();
}
bootstrap();
This configuration sets up our microservice to use TCP as the transport layer, listening on port 8877.
Communication Between Microservices
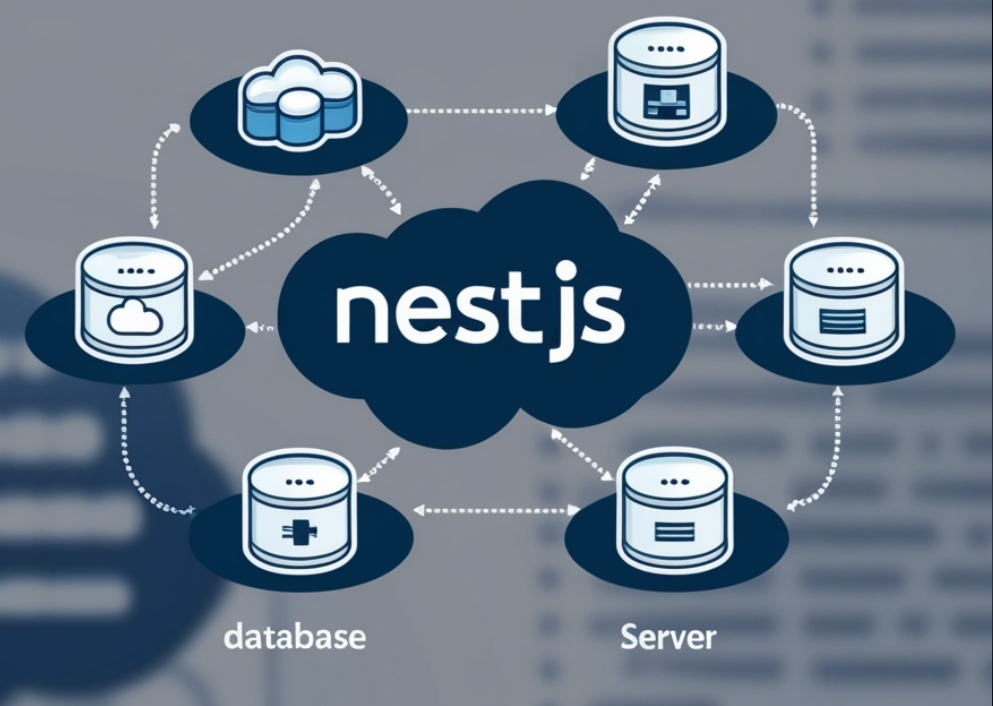
One of the key aspects of a microservices architecture is inter-service communication. NestJS provides several options for communication between microservices, including:
- TCP
- Redis
- MQTT
- gRPC
- RabbitMQ
Let’s create another microservice that will communicate with our user microservice. We’ll create an order microservice that needs to fetch user information.
First, generate the new module, controller, and service:
nest generate module orders
nest generate controller orders
nest generate service orders
Now, let’s implement the order microservice:
// src/orders/orders.service.ts
import { Injectable } from '@nestjs/common';
import { ClientProxy, ClientProxyFactory, Transport } from '@nestjs/microservices';
@Injectable()
export class OrdersService {
private client: ClientProxy;
constructor() {
this.client = ClientProxyFactory.create({
transport: Transport.TCP,
options: {
host: '127.0.0.1',
port: 8877,
},
});
}
async createOrder(userId: number, productId: number) {
const user = await this.client.send({ cmd: 'findOneUser' }, userId).toPromise();
if (!user) {
throw new Error('User not found');
}
// Create order logic here
const order = { id: Math.random(), userId, productId };
return { order, user };
}
}
// src/orders/orders.controller.ts
import { Controller, Post, Body } from '@nestjs/common';
import { OrdersService } from './orders.service';
@Controller('orders')
export class OrdersController {
constructor(private readonly ordersService: OrdersService) {}
@Post()
async createOrder(@Body() createOrderDto: { userId: number; productId: number }) {
return this.ordersService.createOrder(createOrderDto.userId, createOrderDto.productId);
}
}
In this example, the order microservice communicates with the user microservice to fetch user information before creating an order. This demonstrates how microservices can work together to fulfill business requirements.
Implementing API Gateways
As your microservices architecture grows, you may want to implement an API Gateway to provide a single entry point for clients and handle cross-cutting concerns like authentication, logging, and request routing.
NestJS can be used to create an API Gateway that communicates with your microservices. Here’s a simple example:
// src/app.module.ts
import { Module } from '@nestjs/common';
import { ClientsModule, Transport } from '@nestjs/microservices';
import { AppController } from './app.controller';
import { AppService } from './app.service';
@Module({
imports: [
ClientsModule.register([
{
name: 'USER_SERVICE',
transport: Transport.TCP,
options: {
host: '127.0.0.1',
port: 8877,
},
},
{
name: 'ORDER_SERVICE',
transport: Transport.TCP,
options: {
host: '127.0.0.1',
port: 8878,
},
},
]),
],
controllers: [AppController],
providers: [AppService],
})
export class AppModule {}
// src/app.controller.ts
import { Controller, Get, Post, Body, Inject } from '@nestjs/common';
import { ClientProxy } from '@nestjs/microservices';
@Controller()
export class AppController {
constructor(
@Inject('USER_SERVICE') private userClient: ClientProxy,
@Inject('ORDER_SERVICE') private orderClient: ClientProxy,
) {}
@Get('users')
async getUsers() {
return this.userClient.send({ cmd: 'findAllUsers' }, {});
}
@Post('orders')
async createOrder(@Body() createOrderDto: { userId: number; productId: number }) {
return this.orderClient.send({ cmd: 'createOrder' }, createOrderDto);
}
}
This API Gateway provides a unified interface for clients to interact with both the user and order microservices.
Testing NestJS Microservices
Testing is crucial in any software development process, and microservices are no exception. NestJS provides excellent support for unit testing, integration testing, and end-to-end testing of microservices.
Here’s an example of how to write a unit test for our user service:
// src/users/users.service.spec.ts
import { Test, TestingModule } from '@nestjs/testing';
import { UsersService } from './users.service';
describe('UsersService', () => {
let service: UsersService;
beforeEach(async () => {
const module: TestingModule = await Test.createTestingModule({
providers: [UsersService],
}).compile();
service = module.get<UsersService>(UsersService);
});
it('should be defined', () => {
expect(service).toBeDefined();
});
it('should return all users', () => {
const users = service.findAll();
expect(users.length).toBe(2);
expect(users[0].name).toBe('John Doe');
});
it('should create a new user', () => {
const newUser = { name: 'Test User', email: 'test@example.com' };
const createdUser = service.create(newUser);
expect(createdUser.id).toBeDefined();
expect(createdUser.name).toBe(newUser.name);
expect(createdUser.email).toBe(newUser.email);
});
});
For integration testing, you can use NestJS’s @nestjs/testing
module to create a test module that includes your microservice components. Here’s an example of an integration test for our user controller:
// src/users/users.controller.spec.ts
import { Test, TestingModule } from '@nestjs/testing';
import { UsersController } from './users.controller';
import { UsersService } from './users.service';
describe('UsersController', () => {
let controller: UsersController;
let service: UsersService;
beforeEach(async () => {
const module: TestingModule = await Test.createTestingModule({
controllers: [UsersController],
providers: [UsersService],
}).compile();
controller = module.get<UsersController>(UsersController);
service = module.get<UsersService>(UsersService);
});
it('should be defined', () => {
expect(controller).toBeDefined();
});
it('should return all users', async () => {
const result = [{ id: 1, name: 'John Doe', email: 'john@example.com' }];
jest.spyOn(service, 'findAll').mockImplementation(() => result);
expect(await controller.findAll()).toBe(result);
});
it('should create a new user', async () => {
const newUser = { name: 'Test User', email: 'test@example.com' };
const result = { id: 3, ...newUser };
jest.spyOn(service, 'create').mockImplementation(() => result);
expect(await controller.create(newUser)).toBe(result);
});
});
For end-to-end testing of microservices, you can create a test application that communicates with your microservices and verifies the entire flow. Here’s an example:
// test/users.e2e-spec.ts
import { Test, TestingModule } from '@nestjs/testing';
import { INestApplication } from '@nestjs/common';
import { Transport, ClientProxy, ClientsModule } from '@nestjs/microservices';
import { AppModule } from '../src/app.module';
describe('Users Microservice (e2e)', () => {
let app: INestApplication;
let client: ClientProxy;
beforeAll(async () => {
const moduleFixture: TestingModule = await Test.createTestingModule({
imports: [
AppModule,
ClientsModule.register([
{ name: 'USER_SERVICE', transport: Transport.TCP, options: { port: 8877 } },
]),
],
}).compile();
app = moduleFixture.createNestApplication();
app.connectMicroservice({
transport: Transport.TCP,
options: { port: 8877 },
});
await app.startAllMicroservices();
await app.init();
client = app.get('USER_SERVICE');
await client.connect();
});
afterAll(async () => {
await app.close();
client.close();
});
it('should create a new user', async () => {
const newUser = { name: 'E2E Test User', email: 'e2e@example.com' };
const createdUser = await client.send({ cmd: 'createUser' }, newUser).toPromise();
expect(createdUser).toHaveProperty('id');
expect(createdUser.name).toBe(newUser.name);
expect(createdUser.email).toBe(newUser.email);
});
it('should return all users', async () => {
const users = await client.send({ cmd: 'findAllUsers' }, {}).toPromise();
expect(Array.isArray(users)).toBe(true);
expect(users.length).toBeGreaterThan(0);
});
});
These tests ensure that your microservices are working correctly both individually and as part of the larger system.
Deploying NestJS Microservices
Deploying microservices can be more complex than deploying monolithic applications due to the distributed nature of the architecture. Here are some approaches and best practices for deploying NestJS microservices:
- Containerization with Docker:
Containerize each microservice using Docker. This ensures consistency across different environments and makes it easier to manage dependencies. Example Dockerfile for a NestJS microservice:
FROM node:14-alpine
WORKDIR /usr/src/app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
EXPOSE 8877
CMD ["npm", "run", "start:prod"]
- Container Orchestration with Kubernetes:
Use Kubernetes to manage the deployment, scaling, and operations of your containerized microservices. Example Kubernetes deployment for a NestJS microservice:
apiVersion: apps/v1
kind: Deployment
metadata:
name: user-microservice
spec:
replicas: 3
selector:
matchLabels:
app: user-microservice
template:
metadata:
labels:
app: user-microservice
spec:
containers:
- name: user-microservice
image: your-registry/user-microservice:latest
ports:
- containerPort: 8877
- Serverless Deployment:
For certain microservices, a serverless approach using platforms like AWS Lambda or Google Cloud Functions can be beneficial. - CI/CD Pipelines:
Implement robust CI/CD pipelines to automate the testing, building, and deployment of your microservices. - Service Discovery and Configuration Management:
Use tools like Consul or etcd for service discovery and configuration management in your microservices architecture. - Monitoring and Logging:
Implement comprehensive monitoring and logging solutions to track the health and performance of your microservices. Tools like Prometheus, Grafana, and ELK stack (Elasticsearch, Logstash, Kibana) can be helpful.
Best Practices and Common Pitfalls
When building microservices with NestJS, keep these best practices in mind:
- Single Responsibility Principle: Each microservice should have a clearly defined and limited scope of functionality.
- Database per Service: Maintain separate databases for each microservice to ensure loose coupling and independent scalability.
- Asynchronous Communication: Use asynchronous communication patterns when possible to improve system resilience and scalability.
- API Versioning: Implement API versioning to manage changes and updates to your microservices without breaking existing clients.
- Circuit Breakers: Implement circuit breakers to handle failures gracefully and prevent cascading failures across your microservices.
- Centralized Logging and Monitoring: Implement a centralized logging and monitoring solution to gain visibility into your distributed system.
- Automated Testing: Maintain a comprehensive suite of automated tests, including unit tests, integration tests, and end-to-end tests.
Common pitfalls to avoid:
- Over-engineering: Don’t create microservices for every small functionality. Start with a monolith and split into microservices as needed.
- Ignoring Data Consistency: Be mindful of data consistency challenges in distributed systems and implement appropriate patterns (e.g., Saga pattern) to manage transactions across microservices.
- Neglecting Security: Implement proper authentication and authorization mechanisms across your microservices.
- Poor Error Handling: Implement robust error handling and provide meaningful error messages to aid in debugging and troubleshooting.
- Inadequate Documentation: Maintain up-to-date documentation for each microservice, including API contracts and deployment procedures.
Conclusion
Building microservices with NestJS offers a powerful and flexible approach to developing scalable, maintainable applications. By leveraging NestJS’s built-in support for microservices, developers can create robust distributed systems with ease.
Throughout this article, we’ve explored the fundamentals of microservices architecture, compared NestJS with Express, and walked through the process of building, testing, and deploying NestJS microservices. We’ve also discussed best practices and common pitfalls to help you succeed in your microservices journey.
As you continue to explore and implement microservices with NestJS, remember that the key to success lies in careful planning, continuous learning, and adapting to the specific needs of your project. Embrace the flexibility and scalability that microservices offer, but also be mindful of the additional complexities they introduce.
FAQs
- Q: What are the main advantages of using NestJS for microservices over Express?
A: NestJS provides built-in support for microservices, a modular architecture, dependency injection, and TypeScript support out of the box. These features make it easier to build and maintain complex microservices compared to Express. - Q: How does NestJS handle communication between microservices?
A: NestJS supports various transport layers for microservices communication, including TCP, Redis, MQTT, gRPC, and RabbitMQ. It provides a unified interface for sending and receiving messages between services. - Q: Can I mix NestJS microservices with services built using other technologies?
A: Yes, NestJS microservices can communicate with services built using other technologies as long as they use compatible communication protocols. - Q: How do I handle database transactions across multiple microservices?
A: Handling transactions across microservices is challenging. You can use patterns like the Saga pattern or implement eventual consistency depending on your specific requirements. - Q: Is it possible to use GraphQL with NestJS microservices?
A: Yes, NestJS has excellent support for GraphQL. You can implement a GraphQL API gateway that communicates with your microservices to resolve queries and mutations. - Q: How do I ensure the security of my NestJS microservices? A: Implement authentication and authorization mechanisms, use HTTPS for communication, implement rate limiting, and follow security best practices for Node.js applications. NestJS provides guards and interceptors that can help in implementing security measures.
- Q: What’s the best way to handle errors in a NestJS microservices architecture? A: Implement centralized error handling, use exception filters in NestJS, implement retry mechanisms for transient failures, and use circuit breakers to handle persistent failures gracefully.