Table of Contents
Introduction
In today’s fast-paced software development landscape, efficiency is key. As projects grow in complexity and scale, the need for streamlined processes becomes increasingly crucial. This is where efficient code building and deployment automation come into play, serving as the backbone of modern software development practices.
Code building, the process of transforming source code into executable programs, and deployment automation, the practice of automatically releasing software to production environments, are two critical components that can significantly impact a team’s productivity and a product’s success. By optimizing these processes, developers can focus more on creating innovative features and less on repetitive, time-consuming tasks.
In this comprehensive guide, we’ll explore the intricacies of code building and deployment automation, providing you with the knowledge and tools to enhance your development workflow and unlock new levels of efficiency.
Understanding Code Building
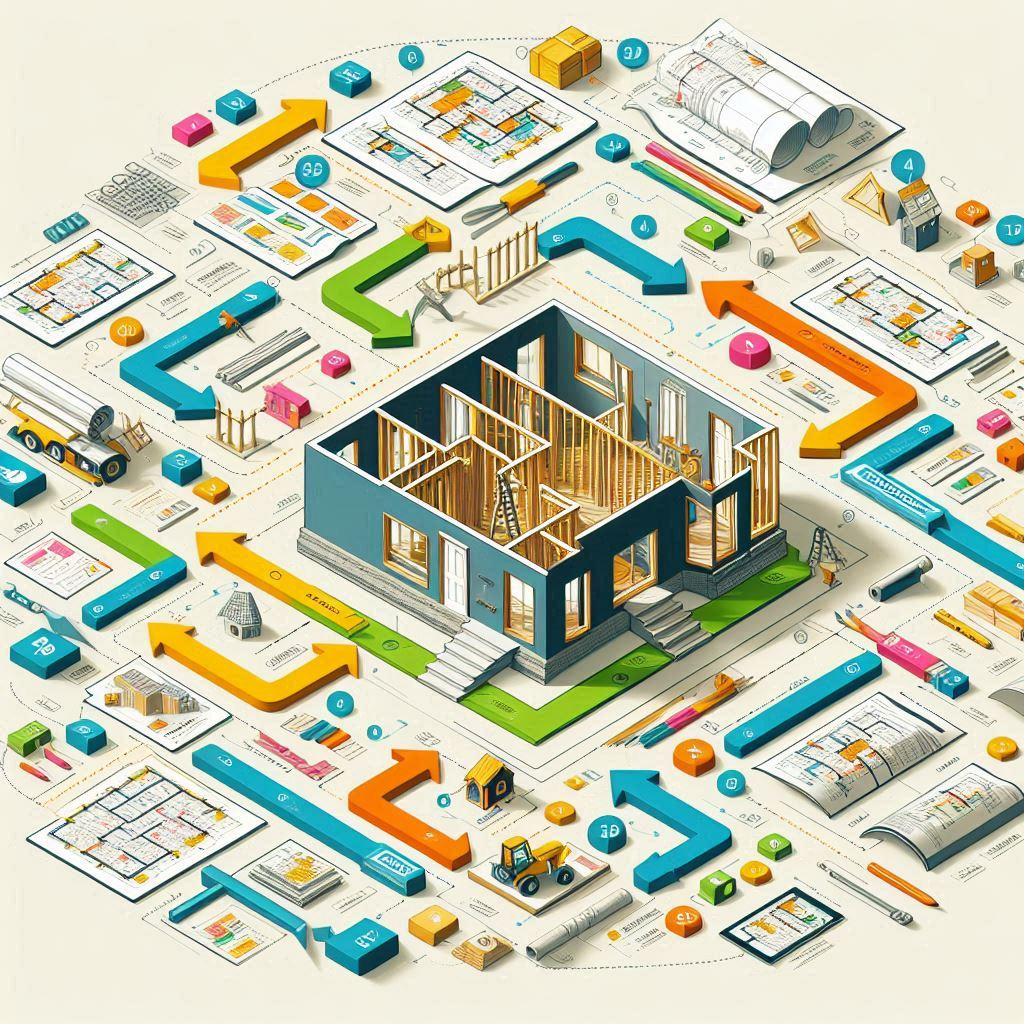
Code building is a fundamental aspect of software development that involves compiling, linking, and packaging source code into a format that can be executed or deployed. This process is essential for transforming human-readable code into machine-executable instructions.
The importance of efficient code building cannot be overstated. A well-optimized build process can:
- Reduce development cycle times
- Improve code quality through consistent builds
- Facilitate easier testing and debugging
- Enable faster release cycles
Common Tools and Build Systems:
Several tools and build systems have emerged to streamline the code building process:
a) Webpack: A popular module bundler for JavaScript applications, Webpack efficiently manages dependencies and assets, optimizing them for production.
b) Babel: This JavaScript compiler allows developers to use the latest ECMAScript features by transpiling code to older, widely-supported versions.
c) Gradle: An open-source build automation tool that supports multiple programming languages and platforms, Gradle is particularly popular in the Java ecosystem.
d) Maven: Another build automation tool primarily used for Java projects, Maven provides a standardized approach to building and managing dependencies.
e) Make: A classic build automation tool that’s still widely used, especially in Unix-based systems and for C/C++ projects.
Understanding these tools and their appropriate use cases is crucial for optimizing your code building process.
Example: Basic Webpack Configuration
const path = require('path');
module.exports = {
entry: './src/index.js',
output: {
filename: 'bundle.js',
path: path.resolve(__dirname, 'dist'),
},
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader',
},
},
],
},
};
This Webpack configuration demonstrates a basic setup for bundling JavaScript files and using Babel for transpilation.
Optimizing Build Processes
Efficient code building is about more than just using the right tools; it’s about implementing strategies to minimize build times and maximize resource utilization. Here are some key techniques to optimize your build processes:
a) Incremental Builds: Instead of rebuilding an entire project from scratch, incremental builds only recompile the parts of the code that have changed since the last build. This can significantly reduce build times, especially for large projects.
b) Caching: Implementing a caching strategy can dramatically speed up subsequent builds. By storing and reusing previously compiled components, you can avoid redundant work and reduce build times.
c) Parallelization: Modern build systems can take advantage of multi-core processors by running multiple build tasks simultaneously. This parallel execution can greatly reduce overall build times.
d) Dependency Management: Efficiently managing and updating project dependencies can prevent unnecessary rebuilds and ensure that only required modules are included in the final build.
e) Code Splitting: For web applications, code splitting involves breaking the application into smaller chunks that can be loaded on demand. This not only improves initial load times but can also optimize the build process.
f) Tree Shaking: This technique, commonly used in JavaScript builds, eliminates dead code (unused exports) from the final bundle, reducing its size and improving load times.
Example: Gradle Parallel Build Configuration
org.gradle.parallel=true
org.gradle.caching=true
org.gradle.configureondemand=true
By implementing these optimization techniques, you can significantly reduce build times, leading to faster development cycles and improved team productivity.
Continuous Integration (CI) and Continuous Deployment (CD)
Continuous Integration and Continuous Deployment (CI/CD) are practices that have revolutionized software development by automating the processes of integrating code changes, running tests, and deploying applications.
Benefits of CI/CD pipelines:
- Faster Time to Market: Automated processes allow for quicker releases and updates.
- Improved Code Quality: Regular integration and testing catch bugs early in the development cycle.
- Increased Collaboration: CI/CD encourages frequent code commits and integration among team members.
- Reduced Risk: Smaller, more frequent releases minimize the risk associated with large-scale deployments.
- Consistency: Automated processes ensure that every build and deployment follows the same steps, reducing human error.
Popular CI/CD Tools:
a) Jenkins: An open-source automation server, Jenkins provides hundreds of plugins to support building, deploying, and automating any project.
b) GitLab CI/CD: Integrated directly into GitLab, this tool offers a complete CI/CD pipeline solution with version control.
c) GitHub Actions: GitHub’s built-in CI/CD solution allows developers to automate workflows directly from their GitHub repositories.
d) CircleCI: A cloud-based CI/CD tool that supports rapid software development and deployment with a focus on speed and reliability.
e) Travis CI: Known for its ease of use, Travis CI is popular among open-source projects and integrates well with GitHub.
Example: GitHub Actions Workflow
name: CI/CD Pipeline
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install dependencies
run: npm ci
- name: Run tests
run: npm test
- name: Build
run: npm run build
Implementing a CI/CD pipeline using these tools can significantly streamline your development process, from code building to deployment.
Automating Deployment
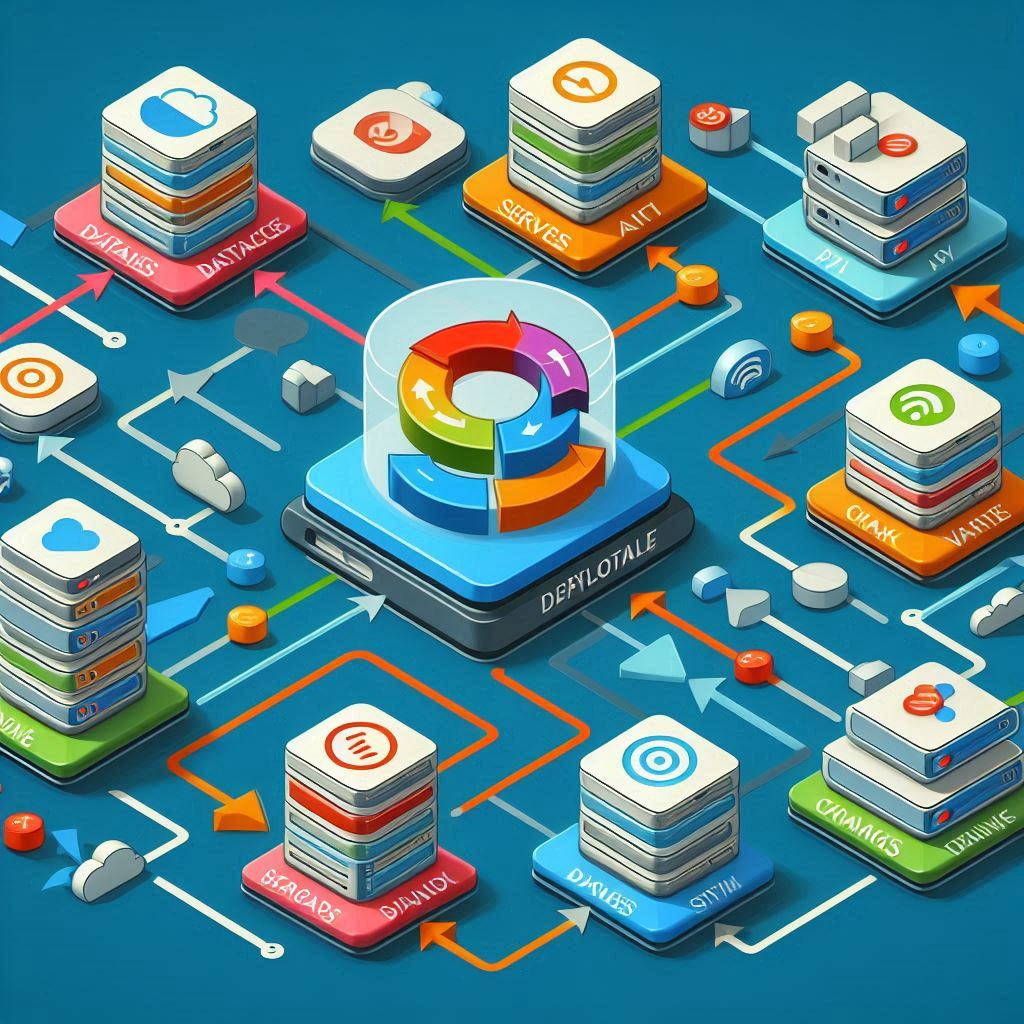
Deployment automation is the practice of using scripts, tools, and workflows to automatically release software to production environments. This process eliminates manual steps, reduces human error, and enables faster, more reliable releases.
Key strategies for deployment automation include:
a) Containerization with Docker: Docker containers package applications with their dependencies, ensuring consistency across different environments and simplifying deployment.
b) Orchestration with Kubernetes: Kubernetes automates the deployment, scaling, and management of containerized applications, providing robust tools for maintaining complex, distributed systems.
c) Infrastructure as Code (IaC): Tools like Terraform and Ansible allow you to define and provision infrastructure using code, ensuring consistency and repeatability in your deployment environments.
d) Blue-Green Deployments: This strategy involves maintaining two identical production environments, allowing you to switch between them for zero-downtime deployments and easy rollbacks.
e) Canary Releases: By gradually rolling out changes to a small subset of users or servers, canary releases allow you to test new features in production with minimal risk.
f) Feature Flags: Implementing feature flags allows you to toggle features on or off without deploying new code, providing flexibility in feature releases and rollbacks.
Example- Docker Compose Configuration
version: '3'
services:
web:
build: .
ports:
- "3000:3000"
environment:
- NODE_ENV=production
depends_on:
- db
db:
image: mongo:latest
volumes:
- ./data:/data/db
By leveraging these deployment automation strategies, you can achieve more frequent, reliable, and efficient releases while minimizing the risk of production issues.
Monitoring and Error Handling
Effective monitoring and error handling are crucial components of any robust code building and deployment automation system. They help ensure the health and stability of your applications throughout the development lifecycle.
Key aspects of monitoring and error handling include:
a) Build Monitoring:
- Track build times and success rates
- Set up alerts for failed builds or unusually long build times
- Use tools like Jenkins Build Monitor or TeamCity to visualize build statuses
b) Deployment Monitoring:
- Implement blue-green deployment monitoring to catch issues before they affect all users
- Use tools like Prometheus or Grafana to monitor system metrics during and after deployments
c) Application Performance Monitoring (APM):
- Utilize APM tools like New Relic or Datadog to track application performance in real-time
- Set up alerts for unusual patterns or performance degradation
d) Log Management:
- Centralize logs from all components of your build and deployment pipeline
- Use log analysis tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk for easier troubleshooting
e) Error Tracking:
- Implement error tracking solutions like Sentry or Rollbar to catch and analyze errors in real-time
- Set up automated error notifications to relevant team members
Best practices for handling errors during deployment:
- Implement automatic rollback mechanisms for failed deployments
- Use feature flags to quickly disable problematic features without full rollbacks
- Establish a clear incident response plan for critical deployment errors
- Conduct post-mortem analyses after significant errors to prevent future occurrences
Example: Prometheus Monitoring Configuration
global:
scrape_interval: 15s
scrape_configs:
- job_name: 'node_exporter'
static_configs:
- targets: ['localhost:9100']
- job_name: 'application'
static_configs:
- targets: ['localhost:8080']
By prioritizing monitoring and error handling in your code building and deployment processes, you can quickly identify and resolve issues, ensuring the stability and reliability of your applications.
Case Studies and Real-World Examples
To illustrate the power of efficient code building and deployment automation, let’s examine how some leading companies have transformed their development workflows:
a) Netflix:
Netflix implemented a sophisticated CI/CD pipeline that allows them to deploy code thousands of times per day. By using tools like Spinnaker for continuous delivery, they’ve achieved rapid, reliable deployments across their global infrastructure.
Key takeaways:
- Embraced microservices architecture for easier deployment
- Implemented canary releases to minimize risk
- Utilized chaos engineering to ensure system resilience
b) Etsy:
Etsy transformed its deployment process from monthly releases to over 50 deploys per day. They achieved this through a combination of continuous integration, automated testing, and a culture that emphasizes small, frequent changes.
Key takeaways:
- Invested in extensive automated testing
- Empowered developers to deploy their own code
- Focused on building a culture of trust and learning from failures
c) Amazon:
Amazon’s deployment process allows them to deploy new code every 11.7 seconds on average. This incredible frequency is achieved through extensive automation and a microservices architecture.
Key takeaways:
- Implemented a “two-pizza team” rule to keep teams small and agile
- Utilized automated deployment pipelines
- Embraced a “you build it, you run it” philosophy
d) Google: Google’s monorepo approach, where most of their code resides in a single repository, required them to develop sophisticated build and test automation. Their custom build system, Bazel, allows for efficient incremental builds across their massive codebase.
Key takeaways:
- Developed custom tools to handle large-scale development
- Implemented extensive automated testing
- Focused on developer productivity through tooling
These case studies demonstrate how efficient code building and deployment automation can transform development workflows, enabling companies to innovate faster and deliver value to users more frequently.
Conclusion
Efficient code building and deployment automation are no longer luxuries in the world of software development β they’re necessities. By optimizing these processes, development teams can:
- Accelerate development cycles
- Improve code quality
- Reduce time-to-market for new features
- Minimize the risk of deployment-related issues
- Enhance overall team productivity
As we’ve explored in this guide, achieving these benefits requires a multifaceted approach:
- Understanding and optimizing the code building process
- Implementing CI/CD pipelines
- Automating deployments with modern tools and strategies
- Prioritizing monitoring and error handling
- Learning from real-world success stories
By adopting these practices and continually refining your processes, you can unlock new levels of efficiency in your software development workflow. Remember, the journey to optimization is ongoing β stay curious, keep learning, and don’t be afraid to experiment with new tools and techniques.
We encourage you to start implementing these practices in your projects today. Whether you’re working on a small personal project or a large-scale enterprise application, the principles of efficient code building and deployment automation can help you deliver better software, faster.
FAQ
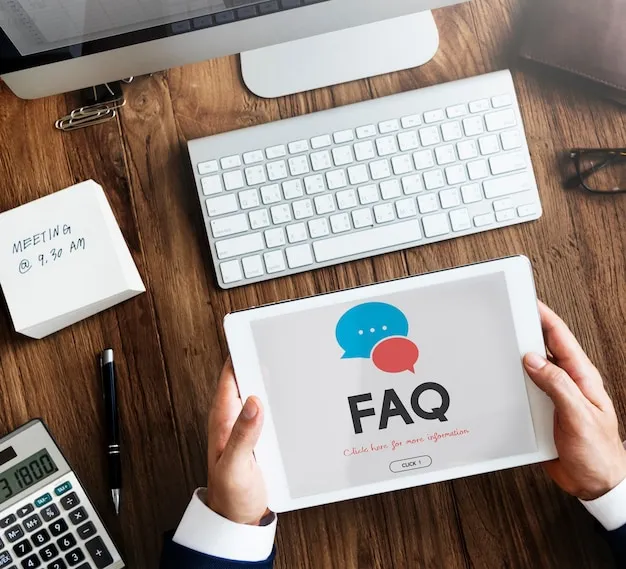
Q1: How long does it typically take to implement a CI/CD pipeline?
A1: The implementation time can vary greatly depending on the complexity of your project and your team’s experience. For a small to medium-sized project, it might take a few weeks to set up a basic pipeline. For larger, more complex projects, it could take several months to fully implement and optimize a CI/CD pipeline.
Q2: Is it worth implementing these practices for small projects?
A2: Absolutely! While the benefits may be more pronounced for larger projects, even small projects can benefit from efficient code building and deployment automation. These practices can save time, improve code quality, and make it easier to scale your project as it grows.
Q3: How can I convince my team or management to invest in improving our build and deployment processes?
A3: Focus on the tangible benefits: faster time-to-market, improved code quality, reduced downtime, and increased developer productivity. Use case studies and industry benchmarks to illustrate the potential impact. Start with small, measurable improvements to demonstrate value.
Q4: What are some common pitfalls to avoid when implementing deployment automation?
A4: Common pitfalls include:
- Over-engineering solutions for simple problems
- Neglecting proper testing in automated pipelines
- Failing to properly secure automated deployment processes
- Not providing adequate training for team members
- Ignoring monitoring and alerting for automated processes
Q5: How often should we review and update our build and deployment processes?
A5: It’s good practice to review your processes quarterly, or whenever there are significant changes to your project or technology stack. Regular reviews help ensure your processes remain efficient and aligned with your project’s evolving needs.
By addressing these frequently asked questions, we hope to provide additional clarity and guidance as you embark on your journey to optimize your code building and deployment processes. Remember, the key to success is continuous improvement and adaptation to your specific needs and challenges.