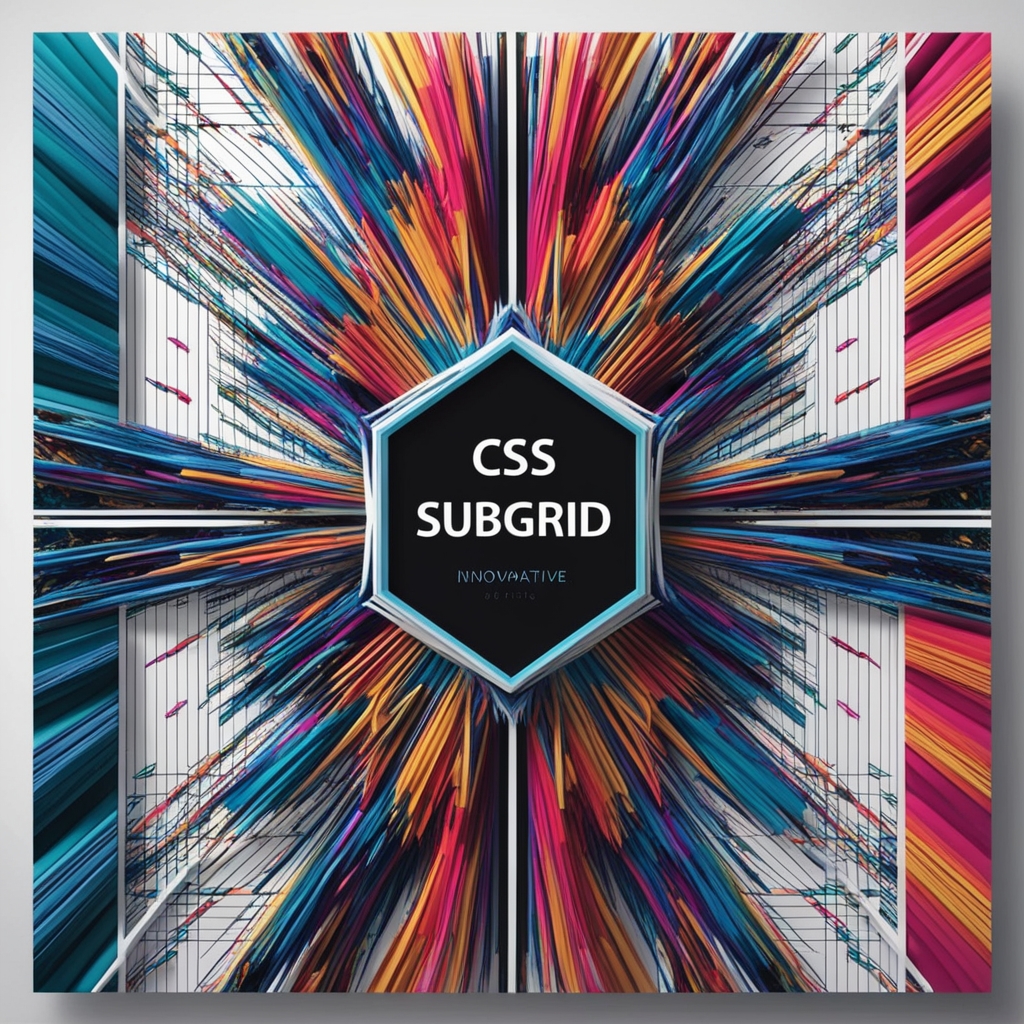
In the ever-evolving world of web design, CSS Grid has become a powerful tool for creating complex layouts with ease. However, as developers, we often find ourselves wrestling with nested grids and alignment issues. Enter CSS Subgrid – a game-changing feature that promises to solve these challenges and revolutionize the way we approach layout design. In this comprehensive guide, we’ll explore CSS Subgrid, its benefits, and how you can leverage it to create more flexible and maintainable layouts.
Table of Contents
Introduction to CSS Subgrid
CSS Grid has been a revolutionary tool for web developers since its introduction, allowing for complex two-dimensional layouts that were previously difficult to achieve. However, as our layouts became more intricate, we encountered limitations when working with nested grids. CSS Subgrid, a part of the CSS Grid Layout Module Level 2 specification, addresses these limitations by enabling nested grid elements to participate in the parent grid’s layout.
Subgrid allows child elements to align with the tracks of their parent grid, creating a more cohesive and flexible layout system. This feature is particularly useful for creating consistent designs across nested components, such as card layouts, form elements, and complex page structures.
The Problem with Nested Grids
Before diving deeper into Subgrid, let’s understand the problem it solves. Consider a typical nested grid scenario:
<div class="parent-grid">
<div class="child-grid">
<div class="grandchild">Content 1</div>
<div class="grandchild">Content 2</div>
</div>
<div class="child-grid">
<div class="grandchild">Content 3</div>
<div class="grandchild">Content 4</div>
</div>
</div>
With traditional CSS Grid, you might style this as follows:
.parent-grid {
display: grid;
grid-template-columns: 1fr 1fr;
gap: 20px;
}
.child-grid {
display: grid;
grid-template-columns: 1fr 1fr;
gap: 10px;
}
While this creates a grid structure, it presents several challenges:
- The child grids are independent of the parent grid, making it difficult to align elements across different nesting levels.
- If you want to ensure that the grandchild elements align with each other across different child grids, you need to manually calculate and set sizes.
- Responsive design becomes more complex, as you need to adjust multiple grid definitions separately.
These issues can lead to inconsistent layouts and increased complexity in your CSS code. This is where CSS Subgrid comes to the rescue.
How CSS Subgrid Works
CSS Subgrid allows a grid container to adopt the track sizing of its parent grid. This means that instead of creating an entirely new grid, a subgrid uses the track definitions of its parent grid. Here’s how you can implement a subgrid:
.parent-grid {
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 20px;
}
.child-grid {
display: grid;
grid-column: span 2; /* Spans two columns of the parent grid */
grid-template-columns: subgrid;
grid-template-rows: subgrid;
}
In this example, the .child-grid
elements will use the column and row definitions of the parent grid. This ensures perfect alignment between the parent and child elements, solving the issues we encountered earlier.
Benefits of Using CSS Subgrid
- Improved Alignment: Subgrid allows for precise alignment of elements across different levels of nesting, creating more cohesive layouts.
- Simplified Code: By inheriting grid properties from the parent, you can reduce the amount of CSS needed to create complex layouts.
- Better Responsiveness: Subgrids automatically adapt to changes in the parent grid, making responsive design easier to implement and maintain.
- Enhanced Flexibility: You can create more dynamic layouts that adapt to content changes without breaking the overall structure.
- Consistent Sizing: Subgrid ensures that elements across different nesting levels maintain consistent sizes, improving the overall design harmony.
CSS Subgrid Tutorial: Getting Started
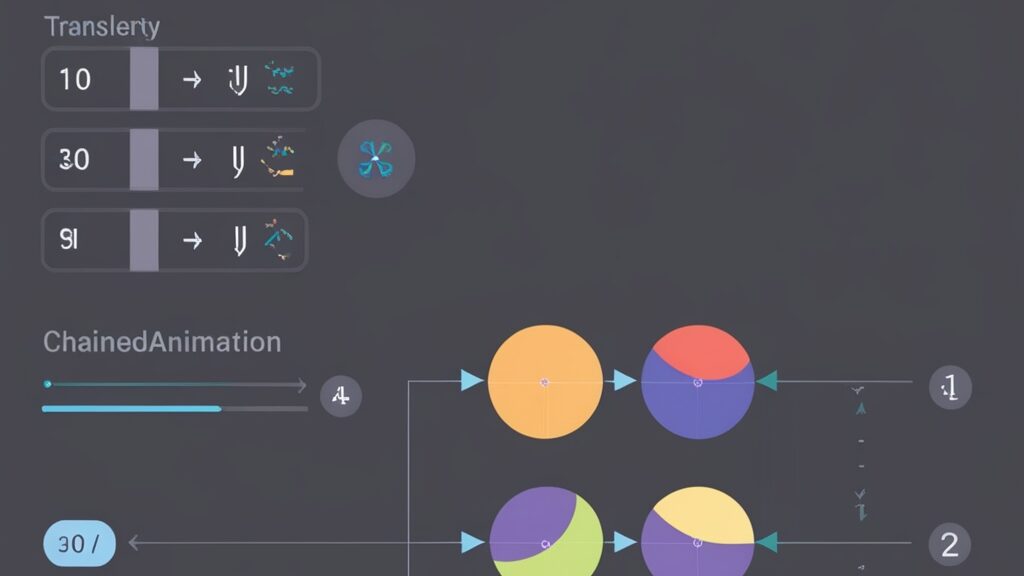
Let’s walk through a step-by-step tutorial to create a basic layout using CSS Subgrid.
Step 1: Set up the HTML structure
<div class="product-grid">
<div class="product-card">
<img src="product1.jpg" alt="Product 1">
<h2>Product Name</h2>
<p>Product description goes here. It can be short or long.</p>
<button>Add to Cart</button>
</div>
<div class="product-card">
<img src="product2.jpg" alt="Product 2">
<h2>Another Product</h2>
<p>This product has a slightly longer description to demonstrate how subgrid handles different content lengths.</p>
<button>Add to Cart</button>
</div>
<!-- Add more product cards as needed -->
</div>
Step 2: Create the parent grid
.product-grid {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(250px, 1fr));
gap: 20px;
padding: 20px;
}
This creates a responsive grid that adjusts the number of columns based on the available space.
Step 3: Implement the subgrid
.product-card {
display: grid;
grid-template-rows: subgrid;
grid-row: span 4; /* Each card spans 4 rows */
background-color: #f0f0f0;
border-radius: 8px;
overflow: hidden;
}
Here, we’re using grid-template-rows: subgrid
to make the product card adopt the row sizing of the parent grid.
Step 4: Style the card contents
.product-card img {
width: 100%;
height: 200px;
object-fit: cover;
}
.product-card h2 {
margin: 10px;
font-size: 1.2em;
}
.product-card p {
margin: 10px;
font-size: 0.9em;
}
.product-card button {
margin: 10px;
padding: 10px 20px;
background-color: #007bff;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
}
These styles will ensure that the content within each product card is presented nicely.
With this setup, you’ll notice that regardless of the content length in each product card, the elements (image, title, description, and button) will align perfectly across all cards in the grid. This is the power of CSS Subgrid in action!
CSS Subgrid Examples
Let’s explore some more advanced examples to showcase the versatility of CSS Subgrid.
Example 1: Form Layout
Forms are an excellent use case for Subgrid, as they often require alignment across multiple fields and labels.
<form class="contact-form">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<label for="message">Message:</label>
<textarea id="message" name="message" required></textarea>
<button type="submit">Send</button>
</form>
.contact-form {
display: grid;
grid-template-columns: max-content 1fr;
gap: 10px 20px;
max-width: 500px;
margin: 0 auto;
}
.contact-form label {
grid-column: 1;
justify-self: end;
}
.contact-form input,
.contact-form textarea,
.contact-form button {
grid-column: 2;
}
.contact-form textarea {
height: 100px;
}
.contact-form button {
justify-self: start;
}
This creates a two-column layout where labels are right-aligned in the first column, and form fields occupy the second column. The subgrid ensures that everything stays aligned even if label lengths vary.
Example 2: Card Layout with Variable Content
Let’s create a more complex card layout that showcases how Subgrid can handle variable content gracefully.
<div class="card-grid">
<div class="card">
<header>
<h2>Card Title 1</h2>
<p class="subtitle">A short subtitle</p>
</header>
<div class="content">
<p>This is some short content.</p>
</div>
<footer>
<button>Action 1</button>
<button>Action 2</button>
</footer>
</div>
<div class="card">
<header>
<h2>Card Title 2 with a Longer Name</h2>
<p class="subtitle">This subtitle is significantly longer to demonstrate flexibility</p>
</header>
<div class="content">
<p>This content is much longer. It contains multiple sentences to show how the subgrid adapts to different content lengths without breaking the layout.</p>
</div>
<footer>
<button>Action</button>
</footer>
</div>
<!-- Add more cards as needed -->
</div>
.card-grid {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(300px, 1fr));
gap: 20px;
padding: 20px;
}
.card {
display: grid;
grid-template-rows: subgrid;
grid-row: span 3;
background-color: #ffffff;
border: 1px solid #e0e0e0;
border-radius: 8px;
overflow: hidden;
box-shadow: 0 2px 4px rgba(0,0,0,0.1);
}
.card header {
background-color: #f0f0f0;
padding: 15px;
}
.card h2 {
margin: 0;
font-size: 1.4em;
}
.card .subtitle {
margin: 5px 0 0;
font-size: 0.9em;
color: #666;
}
.card .content {
padding: 15px;
}
.card footer {
padding: 15px;
background-color: #f9f9f9;
display: flex;
justify-content: flex-start;
gap: 10px;
}
.card button {
padding: 8px 15px;
background-color: #007bff;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
}
In this example, the Subgrid ensures that regardless of the content length in each section (header, content, footer), all cards in the grid maintain a consistent layout. The headers and footers will align across all cards, creating a visually pleasing and organized design.
Best Practices for Using CSS Subgrid
- Use Subgrid for Alignment: Leverage Subgrid when you need to align elements across different nesting levels or when dealing with variable content.
- Combine with Auto-Fit and Minmax: For responsive designs, use
auto-fit
andminmax()
in your parent grid to create flexible layouts that work well with Subgrid. - Plan Your Grid Structure: Before implementing, sketch out your grid structure to determine where Subgrid can be most effective.
- Utilize Grid Gap: Take advantage of the
gap
property in your parent grid to create consistent spacing that propagates through your subgrids. - Consider Accessibility: Ensure that your grid layout makes sense when linearized for screen readers or when CSS is disabled.
- Test with Variable Content: Always test your Subgrid layouts with different content lengths to ensure they remain robust and visually appealing.
- Use Fallbacks: Implement fallback styles for browsers that don’t support Subgrid to ensure a good experience for all users.
Browser Support and Fallbacks
As of 2024, CSS Subgrid is supported in most modern browsers, including Firefox, Chrome, Safari, and Edge. However, it’s always a good practice to provide fallbacks for older browsers or those that don’t support Subgrid.
Here’s an example of how you can create a fallback:
.parent-grid {
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 20px;
}
.child-grid {
display: grid;
grid-column: span 2;
/* Fallback for browsers that don't support subgrid */
grid-template-columns: 1fr 1fr;
gap: 10px;
}
@supports (grid-template-columns: subgrid) {
.child-grid {
grid-template-columns: subgrid;
grid-template-rows: subgrid;
}
}
This approach uses the @supports
rule to apply Subgrid styles only in browsers that support it, while providing a reasonable fallback for others.
Combining Subgrid with Other CSS Features
CSS Subgrid becomes even more powerful when combined with other modern CSS features. Here are some ways to enhance your layouts:
- CSS Custom Properties (Variables): Use CSS variables to create dynamic and themeable grid layouts.
:root {
--grid-columns: 4;
--grid-gap: 20px;
}
.parent-grid {
display: grid;
grid-template-columns: repeat(var(--grid-columns), 1fr);
gap: var(--grid-gap);
}
- CSS Logical Properties: Combine Subgrid with logical properties for better internationalization support.
.child-grid {
display: grid;
grid-template-columns: subgrid;
padding-inline: 10px;
margin-block: 15px;
}