JavaScript debugging is an essential skill for any web developer. Whether you’re a beginner or an experienced programmer, you’ll inevitably encounter bugs in your code. This comprehensive guide will help you understand common JavaScript errors, their causes, and most importantly, how to fix them effectively.
Understanding JavaScript Error Types
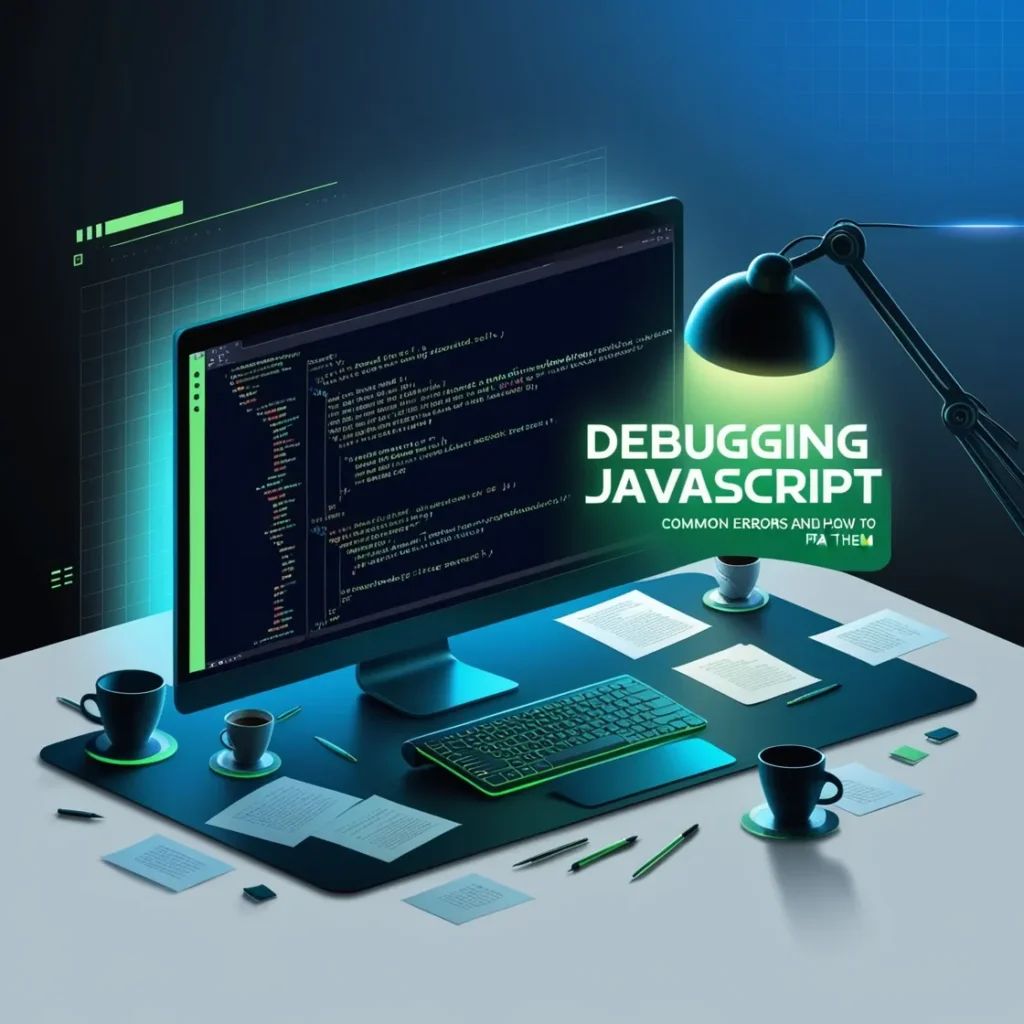
1. Syntax Errors
Syntax errors occur when your code violates JavaScript’s grammar rules. These are typically the easiest to spot as they prevent your code from running altogether.
Common Examples:
// Missing closing parenthesis
function sayHello( {
console.log("Hello!");
// Correct version
function sayHello() {
console.log("Hello!");
}
// Missing quotes in string
let name = John;
// Correct version
let name = "John";
2. Reference Errors
Reference errors happen when you try to use a variable or function that hasn’t been declared or is out of scope.
Common Examples:
// Using an undeclared variable
console.log(unknownVariable);
// Correct version
let unknownVariable = "Now I exist";
console.log(unknownVariable);
// Accessing a variable before declaration due to temporal dead zone
console.log(myVar);
let myVar = "Hello";
// Correct version
let myVar = "Hello";
console.log(myVar);
3. Type Errors
Type errors occur when you try to perform operations on values of the wrong type or access properties of undefined/null values.
Common Examples:
// Trying to call something that isn't a function
const user = { name: "John" };
user.getName();
// Correct version
const user = {
name: "John",
getName() {
return this.name;
}
};
user.getName();
// Accessing properties of undefined
let obj = undefined;
console.log(obj.property);
// Correct version with null check
let obj = undefined;
if (obj) {
console.log(obj.property);
}
Essential Debugging Techniques
1. Using Console Methods Effectively
The console object offers more than just console.log():
// Basic logging
console.log("Basic message");
// Warning
console.warn("Warning message");
// Error
console.error("Error message");
// Info
console.info("Information message");
// Table format for objects
const users = [
{ name: "John", age: 30 },
{ name: "Jane", age: 25 }
];
console.table(users);
// Time execution
console.time("loop");
for(let i = 0; i < 1000000; i++) {}
console.timeEnd("loop");
2. Debugging with Breakpoints
Learn to use the browser’s developer tools for setting breakpoints:
function calculateTotal(items) {
let total = 0;
// You can set a breakpoint on the next line
for(let item of items) {
total += item.price;
}
return total;
}
const cart = [
{ name: "Book", price: 20 },
{ name: "Pen", price: 1 }
];
calculateTotal(cart);
3. Try-Catch Error Handling
Implement proper error handling to make debugging easier:
function fetchUserData(userId) {
try {
// Potentially problematic code
if (!userId) {
throw new Error("User ID is required");
}
// More code...
return userData;
} catch (error) {
console.error("Error fetching user data:", error.message);
// Handle the error appropriately
return null;
}
}
Common JavaScript Gotchas and Solutions
1. Scope Issues
// Problem: Variable scope confusion
function outer() {
var x = 10;
function inner() {
var x = 20; // Creates new variable instead of modifying outer x
console.log(x);
}
inner();
console.log(x);
}
// Solution: Use proper variable referencing
function outer() {
let x = 10;
function inner() {
x = 20; // Modifies outer x
console.log(x);
}
inner();
console.log(x);
}
2. Asynchronous Code Problems
// Problem: Not handling async operations properly
function fetchData() {
let result;
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
result = data;
});
return result; // Will always be undefined
}
// Solution: Use async/await or handle promises properly
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const result = await response.json();
return result;
} catch (error) {
console.error("Error fetching data:", error);
throw error;
}
}
3. Event Handling Issues
// Problem: Event listener memory leaks
function addButtonListener() {
const button = document.querySelector('#myButton');
button.addEventListener('click', function() {
// This creates a new function each time
console.log('Button clicked');
});
}
// Solution: Named function and cleanup
function handleClick() {
console.log('Button clicked');
}
function addButtonListener() {
const button = document.querySelector('#myButton');
button.addEventListener('click', handleClick);
// Cleanup when needed
return () => button.removeEventListener('click', handleClick);
}
Best Practices for Prevention
- Use Strict Mode
'use strict';
// Your code here
- Implement Error Boundaries
class ErrorBoundary {
constructor() {
this.hasError = false;
}
static getDerivedStateFromError(error) {
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
logErrorToService(error, errorInfo);
}
}
- Write Defensive Code
function processUserData(user) {
// Validate input
if (!user || typeof user !== 'object') {
throw new TypeError('Invalid user object');
}
// Use default values
const name = user.name || 'Anonymous';
const age = user.age ?? 0;
// Process data safely
return {
displayName: name.trim(),
isAdult: age >= 18
};
}
Frequently Asked Questions (FAQ)
Conclusion
Debugging JavaScript effectively requires understanding common error types, using the right tools, and implementing preventive measures. By following the practices and solutions outlined in this guide, you’ll be better equipped to handle JavaScript errors and write more reliable code.
Remember that debugging is not just about fixing errors—it’s about understanding why they occur and how to prevent them in the future. Keep practicing these techniques, and they’ll become second nature in your development workflow.