Introduction
GraphQL vs REST, choosing the right API style for your project has become more crucial than ever. Two contenders stand out in this arena: REST (Representational State Transfer) and GraphQL. Both have their strengths and use cases, but which one should you choose for your next project? In this article, we’ll dive deep into the world of APIs, comparing GraphQL and REST, and exploring how they fit into modern backend frameworks like SvelteKit and Express.
Understanding REST
REST, or Representational State Transfer, has been the go-to architectural style for designing networked applications for over two decades. It’s based on a set of constraints that, when applied as a whole, emphasize scalability, simplicity, and independence between components.
Key Characteristics of REST:
- Stateless: Each request from client to server must contain all the information needed to understand and process the request.
- Client-Server: The client and server are separated, allowing each to evolve independently.
- Cacheable: Responses must define themselves as cacheable or non-cacheable.
- Uniform Interface: A uniform way of interacting with a given server regardless of device or type of application.
- Layered System: A client cannot ordinarily tell whether it is connected directly to the end server or to an intermediary along the way.
Example of a REST API Endpoint:
GET /api/users/123
This endpoint would typically return all the information about the user with ID 123.
Understanding GraphQL
GraphQL, developed by Facebook and released in 2015, is a query language for APIs and a runtime for executing those queries with your existing data. It provides a more efficient, powerful, and flexible alternative to REST.
Key Characteristics of GraphQL:
- Single Endpoint: All requests are sent to a single endpoint, typically
/graphql
. - Declarative Data Fetching: Clients can specify exactly what data they need.
- Strong Typing: GraphQL APIs are organized in terms of types and fields, not endpoints.
- Introspection: A GraphQL server can be queried for the types it supports.
- Real-time Updates: GraphQL supports subscriptions for real-time data.
Example of a GraphQL Query:
query {
user(id: "123") {
name
email
posts {
title
}
}
}
This query would return the name and email of the user with ID 123, along with the titles of their posts.
REST vs GraphQL: A Comparison
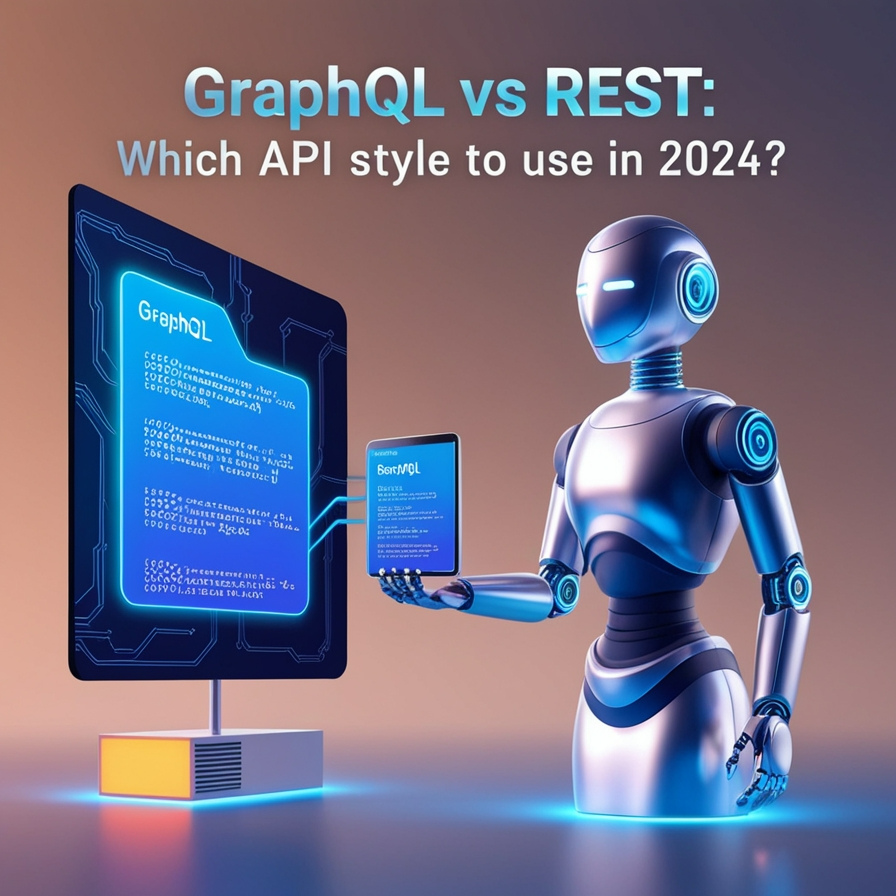
Now that we’ve covered the basics, let’s compare REST and GraphQL across several key factors:
1. Data Fetching
REST:
- Multiple endpoints for different resources
- Often requires multiple requests to fetch related data
- Can lead to over-fetching or under-fetching of data
GraphQL:
- Single endpoint for all data needs
- Can fetch multiple resources in a single request
- Clients specify exactly what data they need, reducing over-fetching
2. Flexibility
REST:
- Fixed structure of endpoints
- Changing data requirements often means creating new endpoints or versions
GraphQL:
- Highly flexible schema
- Easier to evolve API over time without versioning
3. Performance
REST:
- Can be optimized with caching
- Multiple round-trips for complex data requirements
GraphQL:
- Reduces network overhead by allowing batched queries
- Can be challenging to implement efficient caching
4. Learning Curve
REST:
- Well-established, with many developers already familiar
- Simpler to get started with for basic APIs
GraphQL:
- Steeper learning curve, especially for complex schemas
- Requires understanding of GraphQL’s type system and query language
5. Tooling and Ecosystem
REST:
- Mature ecosystem with a wide range of tools
- Excellent browser devtools support
GraphQL:
- Growing ecosystem with powerful developer tools
- Strong typing enables better IDE support and code generation
SvelteKit for Backend: A Modern Approach
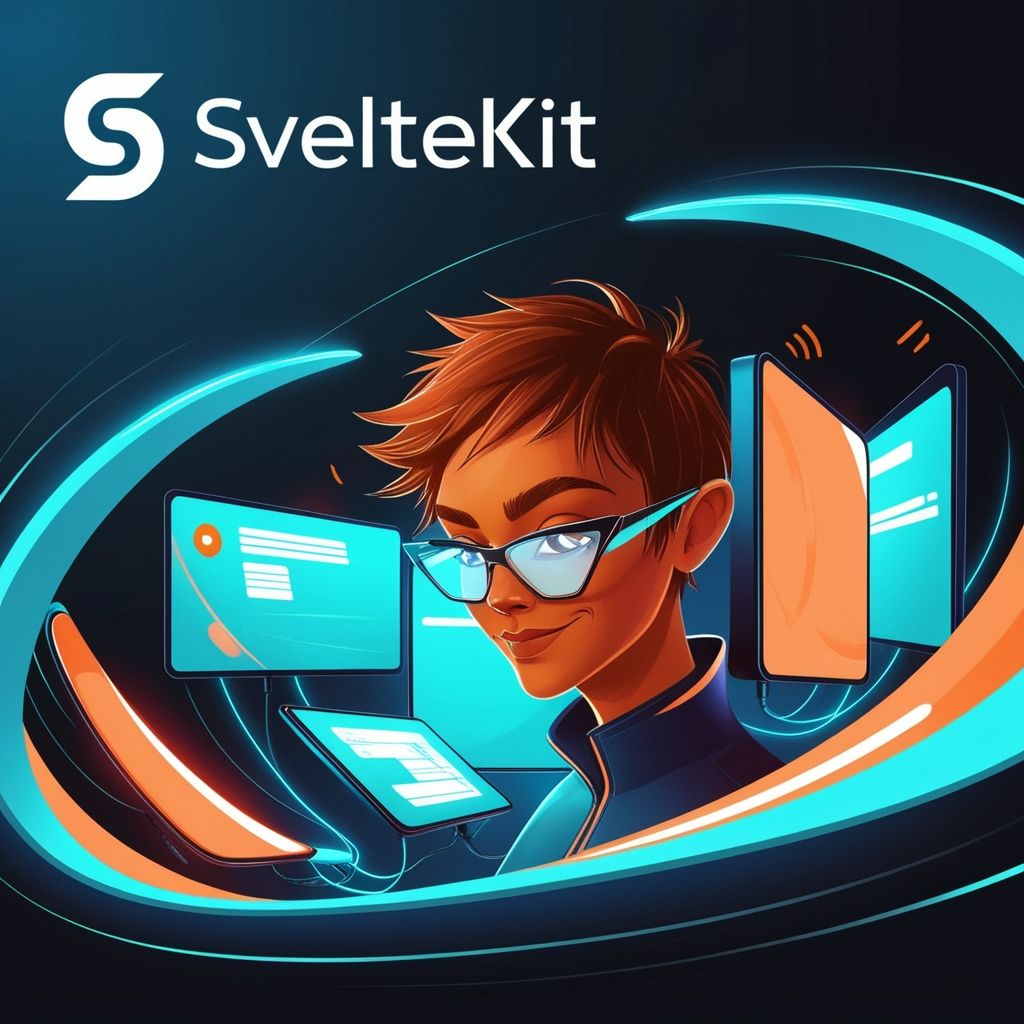
SvelteKit, the official application framework for Svelte, has gained significant traction in the frontend world. But did you know it’s also an excellent choice for building backend APIs? Let’s explore how SvelteKit fits into the GraphQL vs REST debate.
SvelteKit and REST
SvelteKit makes it incredibly easy to create REST APIs. Here’s a simple example of a REST endpoint in SvelteKit:
// src/routes/api/users/[id]/+server.js
export async function GET({ params }) {
const { id } = params;
// Fetch user data based on id
const userData = await fetchUserData(id);
return new Response(JSON.stringify(userData), {
headers: {
'Content-Type': 'application/json'
}
});
}
This creates a GET endpoint at /api/users/:id
that returns user data based on the provided ID.
SvelteKit and GraphQL
While SvelteKit doesn’t have built-in GraphQL support, it’s straightforward to integrate GraphQL using libraries like Apollo Server. Here’s a basic example:
// src/routes/graphql/+server.js
import { ApolloServer } from '@apollo/server';
import { startStandaloneServer } from '@apollo/server/standalone';
import { typeDefs, resolvers } from './schema';
const server = new ApolloServer({
typeDefs,
resolvers,
});
export async function POST({ request }) {
const { url } = await startStandaloneServer(server);
return fetch(url, {
method: 'POST',
headers: request.headers,
body: await request.text(),
});
}
This sets up a GraphQL endpoint at /graphql
that can handle queries and mutations.
SvelteKit vs Express: A Brief Comparison
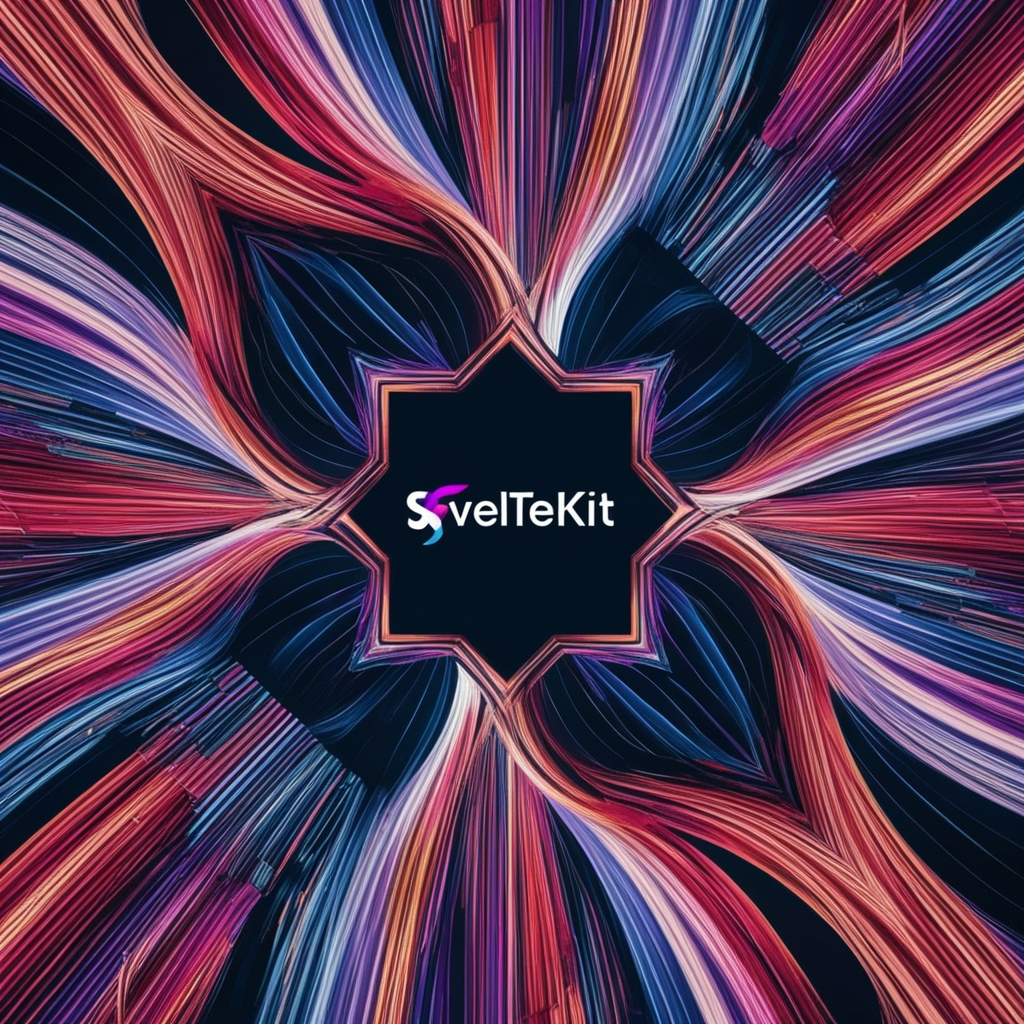
While we’re focusing on API styles, it’s worth comparing SvelteKit to Express, another popular choice for building web applications and APIs.
SvelteKit:
- Full-stack framework with built-in routing and SSR
- Excellent for building both frontend and backend
- File-based routing simplifies API creation
- Built-in optimizations for performance
Express:
- Minimal and flexible Node.js web application framework
- Extensive middleware ecosystem
- More control over server configuration
- Suitable for building microservices
Both can be used to create REST or GraphQL APIs, but SvelteKit offers a more integrated full-stack solution, while Express provides more flexibility and control over the server setup.
Choosing Between GraphQL and REST in 2024
As we look ahead to the rest of 2024, the choice between GraphQL and REST largely depends on your specific project requirements. Here are some guidelines to help you decide:
Choose GraphQL if:
- You’re building a complex application with interrelated data
- Your clients need flexibility in data fetching
- You want to avoid versioning your API
- You’re working with a microservices architecture
- Real-time data updates are a priority (using subscriptions)
Choose REST if:
- You’re building a simple CRUD application
- Your data structure is relatively flat and straightforward
- Caching is a high priority
- You need to leverage existing tools and infrastructure
- Your team is more familiar with REST and you have time constraints
Implementing GraphQL and REST in SvelteKit
Let’s look at more detailed examples of implementing both GraphQL and REST APIs in SvelteKit:
REST API in SvelteKit
// src/routes/api/posts/+server.js
export async function GET({ url }) {
const limit = Number(url.searchParams.get('limit') ?? '10');
const posts = await fetchPosts(limit);
return new Response(JSON.stringify(posts), {
headers: {
'Content-Type': 'application/json'
}
});
}
export async function POST({ request }) {
const data = await request.json();
const newPost = await createPost(data);
return new Response(JSON.stringify(newPost), {
headers: {
'Content-Type': 'application/json'
},
status: 201
});
}
This creates GET and POST endpoints for handling posts. The GET endpoint accepts a limit
query parameter, while the POST endpoint creates a new post.
GraphQL API in SvelteKit
First, let’s define our schema:
// src/lib/graphql/schema.js
import { gql } from 'graphql-tag';
export const typeDefs = gql`
type Post {
id: ID!
title: String!
content: String!
author: User!
}
type User {
id: ID!
name: String!
email: String!
posts: [Post!]!
}
type Query {
posts(limit: Int): [Post!]!
user(id: ID!): User
}
type Mutation {
createPost(title: String!, content: String!, authorId: ID!): Post!
}
`;
export const resolvers = {
Query: {
posts: async (_, { limit = 10 }) => {
return await fetchPosts(limit);
},
user: async (_, { id }) => {
return await fetchUser(id);
}
},
Mutation: {
createPost: async (_, { title, content, authorId }) => {
return await createPost({ title, content, authorId });
}
},
User: {
posts: async (parent) => {
return await fetchPostsByUser(parent.id);
}
},
Post: {
author: async (parent) => {
return await fetchUser(parent.authorId);
}
}
};
Now, let’s set up the GraphQL endpoint:
// src/routes/graphql/+server.js
import { ApolloServer } from '@apollo/server';
import { startStandaloneServer } from '@apollo/server/standalone';
import { typeDefs, resolvers } from '$lib/graphql/schema';
const server = new ApolloServer({
typeDefs,
resolvers,
});
export async function POST({ request }) {
const { url } = await startStandaloneServer(server);
return fetch(url, {
method: 'POST',
headers: request.headers,
body: await request.text(),
});
}
This sets up a GraphQL endpoint at /graphql
that can handle queries and mutations defined in our schema.
Best Practices for API Design in 2024
Regardless of whether you choose GraphQL or REST, here are some best practices to follow:
- Use HTTPS: Always secure your API with HTTPS to protect data in transit.
- Implement Authentication and Authorization: Protect your API from unauthorized access.
- Rate Limiting: Implement rate limiting to prevent abuse of your API.
- Versioning: For REST APIs, consider versioning to maintain backwards compatibility.
- Documentation: Provide clear, comprehensive documentation for your API.
- Error Handling: Implement consistent error handling and provide meaningful error messages.
- Pagination: For large datasets, implement pagination to improve performance.
- Monitoring and Logging: Set up proper monitoring and logging for your API to track usage and diagnose issues.
Conclusion
As we navigate the API landscape in 2024, both GraphQL and REST remain viable options, each with its own strengths and use cases. REST continues to be a solid choice for simpler applications and when working with existing systems, while GraphQL shines in complex, data-intensive applications where flexibility is key.
SvelteKit proves to be an excellent framework for building both types of APIs, offering a modern, efficient approach to full-stack development. Whether you choose GraphQL or REST, and whether you opt for SvelteKit or Express, the key is to understand your project’s specific requirements and choose the tools that best meet those needs.
Remember, the best API is one that serves your users effectively, is easy to maintain, and can evolve with your application. By following best practices and staying informed about the latest developments in API design, you’ll be well-equipped to make the right choice for your projects in 2024 and beyond.
FAQ
- Q: Can I use both GraphQL and REST in the same project?
A: Yes, it’s possible to use both GraphQL and REST in the same project. Some companies use this hybrid approach, using GraphQL for complex data requirements and REST for simpler operations. - Q: Is GraphQL replacing REST?
A: While GraphQL has gained popularity, it’s not replacing REST entirely. Both have their use cases, and REST remains a solid choice for many projects. - Q: How does SvelteKit compare to Next.js for API development?
A: Both SvelteKit and Next.js offer excellent API development capabilities. SvelteKit uses Svelte’s reactive approach and tends to have a smaller bundle size, while Next.js has a larger ecosystem and is based on React. - Q: Can I use GraphQL with a REST backend?
A: Yes, it’s possible to create a GraphQL layer on top of existing REST APIs. This approach can provide the benefits of GraphQL while leveraging existing REST infrastructure. - Q: How does caching work with GraphQL?
A: Caching in GraphQL can be more complex than in REST due to the flexible nature of queries. However, tools like Apollo Client provide sophisticated caching mechanisms for GraphQL. - Q: Is it easier to scale a REST or GraphQL API?
A: Both can be scaled effectively. REST can be easier to cache and scale horizontally, while GraphQL can reduce network overhead and provide more efficient data loading. - Q: How do I handle file uploads in GraphQL?
A: File uploads in GraphQL can be handled using thegraphql-upload
package or by using REST endpoints alongside your GraphQL API. - Q: Can I use TypeScript with SvelteKit for API development?
A: Yes, SvelteKit has excellent TypeScript support, which can be particularly beneficial when developing APIs. - Q: How do I implement real-time features with REST?
A: Real-time features in REST are typically implemented using WebSockets or Server-Sent Events, while GraphQL has built-in support for subscriptions. - Q: Is it possible to generate API documentation automatically for GraphQL and REST?
A: Yes, tools like Swagger can automatically generate documentation for REST APIs, while GraphQL’s introspection feature allows for automatic schema documentation.