Table of Contents
In today’s digital landscape, web application security is paramount. With cyber threats evolving rapidly, developers must stay ahead of potential vulnerabilities. Enter Helmet.js โ a powerful tool in the Node.js ecosystem that helps secure your Express applications with ease. This comprehensive guide will delve into the world of Helmet.js, exploring its features, benefits, and implementation strategies.
What is Helmet.js?
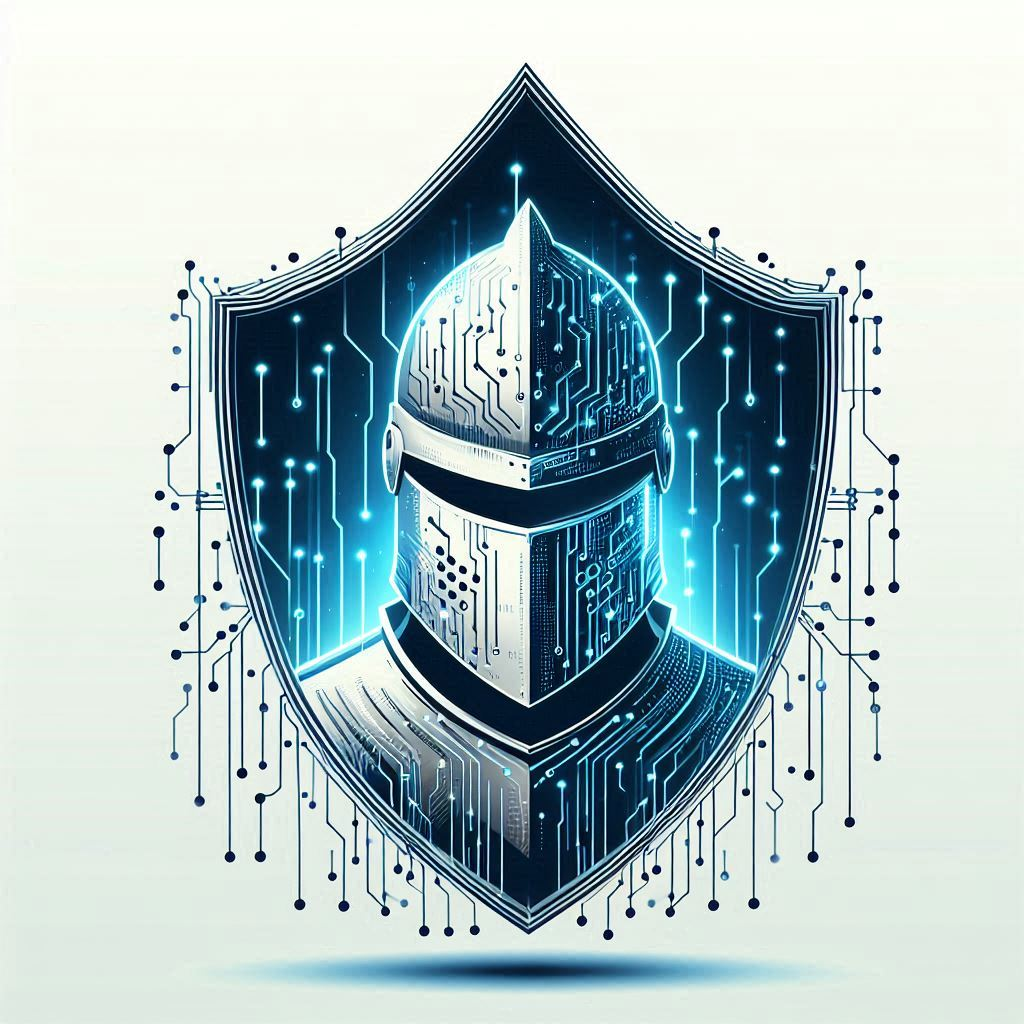
Helmet.js is a collection of 15 smaller middleware functions that set HTTP response headers. It acts as a layer of protection for your Express applications, helping to secure them by setting various HTTP headers. These headers are crucial in preventing common web vulnerabilities such as cross-site scripting (XSS), clickjacking, and other injection attacks.
Key Features:
- Easy integration with Express.js applications
- Modular design allowing for customized security configurations
- Regular updates to address new security threats
- Wide community support and active maintenance
Why Use Helmet.js?
Security should never be an afterthought in web development. Helmet.js provides a simple yet effective way to enhance your application’s security posture. Here’s why you should consider using Helmet.js:
- Simplified Security Implementation: Instead of manually configuring multiple security headers, Helmet.js does the heavy lifting for you.
- Protection Against Common Vulnerabilities: It guards against well-known web vulnerabilities out of the box.
- Performance Optimized: Helmet.js is designed to have minimal impact on your application’s performance.
- Customizable: You can easily enable or disable specific protections based on your application’s needs.
- Compliance: Many of the headers set by Helmet.js are recommended by security standards and can help in compliance efforts.
Getting Started with Helmet.js
Let’s dive into how you can start using Helmet.js in your Node.js applications. The process is straightforward and can be completed in just a few steps.
Installation
First, you need to install Helmet.js via npm (Node Package Manager). Open your terminal and run:
npm install helmet
Basic Usage
Once installed, you can use Helmet.js in your Express application like this:
const express = require('express');
const helmet = require('helmet');
const app = express();
// Use Helmet!
app.use(helmet());
// Your routes and other middleware...
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
This simple setup applies all of Helmet’s default protections to your application.
Core Functionalities of Helmet.js
Helmet.js provides several core functionalities, each addressing specific security concerns. Let’s explore some of the key protections:
1. Content Security Policy (CSP)
CSP helps prevent cross-site scripting (XSS) and other code injection attacks. It specifies which dynamic resources are allowed to load.
app.use(
helmet.contentSecurityPolicy({
directives: {
defaultSrc: ["'self'"],
scriptSrc: ["'self'", "'unsafe-inline'"],
styleSrc: ["'self'", "'unsafe-inline'"],
imgSrc: ["'self'", "data:", "https:"],
},
})
);
2. X-XSS-Protection
This header enables the browser’s built-in filters to prevent XSS attacks.
app.use(helmet.xssFilter());
3. Strict-Transport-Security
HSTS forces browsers to use HTTPS for future requests, enhancing transport layer security.
app.use(
helmet.hsts({
maxAge: 31536000,
includeSubDomains: true,
preload: true,
})
);
4. X-Frame-Options
This protection helps prevent clickjacking attacks by disabling iframes.
app.use(helmet.frameguard({ action: 'deny' }));
5. Referrer-Policy
Controls the Referer header when navigating to other pages.
app.use(helmet.referrerPolicy({ policy: 'strict-origin-when-cross-origin' }));
These are just a few examples of the protections Helmet.js offers. Each middleware can be configured individually to suit your application’s specific needs.
Advanced Usage and Configuration
While the default configuration of Helmet.js provides excellent protection, you might need to fine-tune it for your specific use case. Here are some advanced configurations:
Custom CSP Directives
You can create a more granular Content Security Policy:
app.use(
helmet.contentSecurityPolicy({
directives: {
defaultSrc: ["'self'"],
scriptSrc: ["'self'", "'unsafe-inline'", 'example.com'],
objectSrc: ["'none'"],
upgradeInsecureRequests: [],
},
})
);
Handling Legacy Browsers
Some older browsers might not support certain headers. You can configure Helmet.js to handle these cases:
app.use(
helmet({
contentSecurityPolicy: {
useDefaults: true,
directives: {
upgradeInsecureRequests: null,
},
},
})
);
Disabling Specific Middleware
If a particular middleware conflicts with your application, you can disable it:
app.use(
helmet({
contentSecurityPolicy: false,
})
);
Best Practices for Implementing Helmet.js
To get the most out of Helmet.js, consider these best practices:
- Keep Helmet.js Updated: Regularly update to the latest version to benefit from new security features and patches.
- Understand Your Headers: Take time to understand what each header does and how it affects your application.
- Test Thoroughly: After implementing Helmet.js, thoroughly test your application to ensure it functions correctly with the new security measures.
- Use in Conjunction with HTTPS: Helmet.js works best when your application is served over HTTPS. Use it alongside proper SSL/TLS configuration.
- Customize Based on Needs: Don’t rely solely on default settings. Customize Helmet.js based on your application’s specific requirements.
- Monitor and Log: Implement logging and monitoring to track any security-related issues that arise.
Helmet.js vs. Other Security Middleware
While Helmet.js is excellent, it’s not the only security middleware available for Node.js applications. Let’s compare it with some alternatives:
Helmet.js vs. Lusca
Lusca, developed by PayPal, is another popular security middleware:
- Helmet.js: More focused on HTTP headers, regularly updated.
- Lusca: Offers some features Helmet doesn’t, like CSRF protection.
Helmet.js vs. Custom Middleware
Some developers prefer writing custom middleware:
- Helmet.js: Easy to use, well-maintained, community-backed.
- Custom Middleware: Offers maximum flexibility but requires more expertise and maintenance.
Helmet.js vs. Web Application Firewalls (WAF)
WAFs like ModSecurity provide broader protection:
- Helmet.js: Application-level protection, easy to integrate into Node.js apps.
- WAFs: More comprehensive but complex to set up and maintain.
Common Pitfalls and How to Avoid Them
Even with Helmet.js, there are some common security pitfalls to watch out for:
- Overreliance on Defaults: Always review and customize Helmet.js settings for your specific needs.
- Ignoring Other Security Aspects: Helmet.js is not a silver bullet. Combine it with other security practices like input validation and proper authentication.
- Misconfiguration of CSP: A too-strict Content Security Policy can break functionality. Test thoroughly after implementation.
- Neglecting Regular Updates: Failing to keep Helmet.js updated can leave your application vulnerable to new threats.
- Inconsistent Use Across Environments: Ensure consistent Helmet.js configuration across development, staging, and production environments.
Future of Helmet.js and Web Security
As web technologies evolve, so do security threats and protections. Here’s what we might expect in the future of Helmet.js and web security:
- Integration with AI/ML: Future versions might incorporate machine learning to adapt to new threats dynamically.
- Enhanced API Security: As API-first architectures become more common, expect Helmet.js to offer more API-specific protections.
- Improved Performance: Continuous optimization to reduce the performance impact of security measures.
- Broader Ecosystem Integration: Closer integration with other Node.js security tools and frameworks.
- Quantum-Ready Security: As quantum computing advances, we may see new headers and protections to address quantum-related vulnerabilities.
Conclusion
Helmet.js stands as a crucial tool in the modern web developer’s arsenal. Its ease of use, coupled with robust security features, makes it an indispensable part of building secure Node.js applications. By implementing Helmet.js and following best practices, you significantly enhance your application’s resistance to common web vulnerabilities.
Remember, security is an ongoing process. Stay informed about the latest threats and regularly update your security measures, including Helmet.js. With the right approach and tools like Helmet.js, you can create web applications that are not only feature-rich but also secure and trustworthy.
Start securing your Node.js applications with Helmet.js today, and take a proactive step towards a safer web ecosystem!