1. Introduction
In the ever-evolving landscape of database management and software development, stored procedures stand out as powerful tools that can significantly enhance the performance, security, and maintainability of your database-driven applications. Whether you’re a seasoned database administrator or a developer looking to optimize your data-centric applications, understanding and mastering stored procedures is crucial for unleashing the full potential of your database systems.
1.1 What are stored procedures?
They are pre-compiled and pre-stored collections of SQL statements that can be executed as a single unit. They are essentially programs stored in the database itself, allowing for complex operations to be encapsulated and easily called from applications or other database objects.
1.2 Brief history and evolution
The concept of stored procedures dates back to the early days of relational database management systems (RDBMS). They were introduced to address the need for reusable code and to improve performance by reducing network traffic between applications and databases. Over time, stored procedures have evolved to become more sophisticated, supporting advanced features like error handling, complex logic, and integration with programming languages.
2. Benefits of Using Stored Procedures
Implementing stored procedures in your database architecture offers numerous advantages that can significantly impact your application’s performance, security, and maintainability.
2.1 Performance improvements
One of the primary benefits of stored procedures is their ability to enhance database performance. Here’s how:
- Pre-compilation: Stored procedures are parsed and optimized when they are first created, resulting in faster execution times for subsequent calls.
- Reduced parsing overhead: Since the execution plan is cached, the database engine doesn’t need to parse and optimize the SQL statements with each execution.
- Batch processing: Complex operations involving multiple SQL statements can be executed as a single batch, reducing round trips between the application and the database.
2.2 Enhanced security
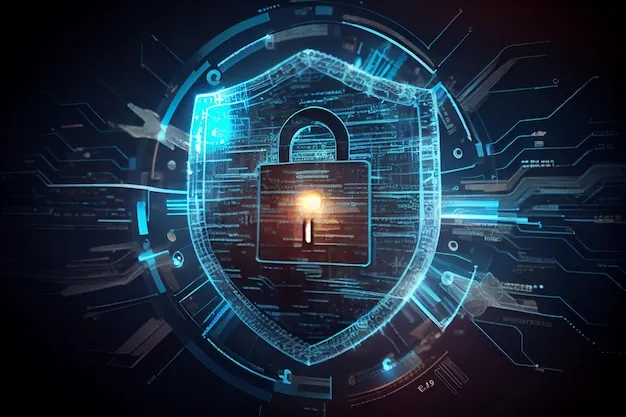
Stored procedures play a crucial role in improving database security:
- Granular access control: They allow you to grant users access to specific procedures without giving them direct access to underlying tables.
- Parameterized queries: By using parameters, stored procedures help prevent SQL injection attacks.
- Encapsulation of business logic: Sensitive operations can be encapsulated within procedures, hiding implementation details from end-users.
2.3 Code reusability and modularity
Stored procedures promote better code organization and reusability:
- Centralized logic: Common database operations can be centralized in procedures, ensuring consistency across applications.
- Easier maintenance: Changes to business logic can be made in one place, affecting all applications that use the procedure.
- Modular design: Complex operations can be broken down into smaller, more manageable procedures.
2.4 Reduced network traffic
By executing operations directly on the database server, stored procedures can significantly reduce network traffic:
- Minimized data transfer: Only the procedure call and its parameters are sent over the network, not entire SQL statements.
- Reduced result sets: Procedures can process data on the server and return only the necessary results, reducing the amount of data transferred.
2.5 Simplified maintenance
Stored procedures contribute to easier database maintenance:
- Version control: Changes to procedures can be tracked and versioned, simplifying rollbacks and updates.
- Centralized updates: Modifying a procedure automatically updates all applications using it, eliminating the need to change client-side code.
- Easier debugging: Many database systems provide tools for debugging stored procedures directly within the database environment.
3. Anatomy of a Stored Procedure
Understanding the structure and components of stored procedures is essential for effective implementation and usage.
3.1 Basic structure
A typical stored procedure consists of the following elements:
CREATE PROCEDURE procedure_name
(parameter1 datatype, parameter2 datatype, ...)
AS
BEGIN
-- SQL statements
-- Business logic
-- Error handling
END;
3.2 Input parameters
Input parameters allow procedures to accept values from the calling application:
CREATE PROCEDURE GetEmployeesByDepartment
@DepartmentID INT
AS
BEGIN
SELECT * FROM Employees WHERE DepartmentID = @DepartmentID;
END;
3.3 Output parameters
Output parameters enable procedures to return multiple values:
CREATE PROCEDURE CalculateStatistics
@InputValue INT,
@Sum INT OUTPUT,
@Average FLOAT OUTPUT
AS
BEGIN
SET @Sum = (SELECT SUM(Value) FROM Statistics WHERE ID <= @InputValue);
SET @Average = (SELECT AVG(Value) FROM Statistics WHERE ID <= @InputValue);
END;
3.4 Return values
Procedures can also return a single integer value using the RETURN statement:
CREATE PROCEDURE CountEmployees
AS
BEGIN
DECLARE @Count INT;
SELECT @Count = COUNT(*) FROM Employees;
RETURN @Count;
END;
4. Creating and Managing Stored Procedures
Effective creation and management of stored procedures are crucial for maintaining a robust database system.
4.1 Syntax across different database systems
While the basic concept remains the same, the syntax for creating stored procedures can vary across different database management systems:
MySQL:
DELIMITER //
CREATE PROCEDURE procedure_name(IN parameter1 INT, OUT parameter2 VARCHAR(50))
BEGIN
-- Procedure body
END //
DELIMITER ;
SQL Server:
CREATE PROCEDURE procedure_name
@parameter1 INT,
@parameter2 VARCHAR(50) OUTPUT
AS
BEGIN
-- Procedure body
END;
Oracle:
CREATE OR REPLACE PROCEDURE procedure_name(
parameter1 IN NUMBER,
parameter2 OUT VARCHAR2
)
IS
BEGIN
-- Procedure body
END;
4.2 Best practices for naming conventions
Adopting consistent naming conventions enhances code readability and maintainability:
- Use descriptive names that reflect the procedure’s purpose (e.g.,
GetCustomerOrders
,UpdateEmployeeSalary
). - Prefix names with the module or application name to avoid conflicts (e.g.,
HR_CalculatePayroll
,Inventory_UpdateStock
). - Use underscores or PascalCase for multi-word names, depending on your organization’s standards.
4.3 Version control and change management
Implementing version control for stored procedures is essential for tracking changes and managing updates:
- Use source control systems like Git to manage procedure scripts.
- Implement a numbering or dating system in procedure names or comments (e.g.,
CreateOrder_v2
,/* Updated: 2024-03-15 */
). - Maintain a change log documenting modifications, reasons for changes, and affected systems.
5. Advanced Techniques
Mastering advanced techniques allows you to create more powerful and flexible stored procedures.
5.1 Dynamic SQL in stored procedures
Dynamic SQL enables the creation of flexible queries that can be constructed at runtime:
CREATE PROCEDURE DynamicSearch
@TableName NVARCHAR(100),
@SearchColumn NVARCHAR(100),
@SearchValue NVARCHAR(100)
AS
BEGIN
DECLARE @SQL NVARCHAR(MAX);
SET @SQL = N'SELECT * FROM ' + @TableName +
N' WHERE ' + @SearchColumn + N' = @Value';
EXEC sp_executesql @SQL, N'@Value NVARCHAR(100)', @Value = @SearchValue;
END;
5.2 Error handling and transaction management
Implementing robust error handling and transaction management is crucial for maintaining data integrity:
CREATE PROCEDURE TransferFunds
@FromAccount INT,
@ToAccount INT,
@Amount DECIMAL(18,2)
AS
BEGIN
BEGIN TRY
BEGIN TRANSACTION;
UPDATE Accounts SET Balance = Balance - @Amount WHERE AccountID = @FromAccount;
UPDATE Accounts SET Balance = Balance + @Amount WHERE AccountID = @ToAccount;
COMMIT TRANSACTION;
END TRY
BEGIN CATCH
ROLLBACK TRANSACTION;
THROW;
END CATCH;
END;
5.3 Cursors and temporary tables
Cursors and temporary tables can be used for complex data manipulation:
CREATE PROCEDURE ProcessLargeDataset
AS
BEGIN
DECLARE @ID INT, @Name NVARCHAR(100);
CREATE TABLE #TempResults (ID INT, ProcessedName NVARCHAR(100));
DECLARE cur CURSOR FOR SELECT ID, Name FROM LargeTable;
OPEN cur;
FETCH NEXT FROM cur INTO @ID, @Name;
WHILE @@FETCH_STATUS = 0
BEGIN
-- Process each row
INSERT INTO #TempResults (ID, ProcessedName)
VALUES (@ID, UPPER(@Name));
FETCH NEXT FROM cur INTO @ID, @Name;
END
CLOSE cur;
DEALLOCATE cur;
SELECT * FROM #TempResults;
DROP TABLE #TempResults;
END;
5.4 Nesting and recursion
Stored procedures can call other procedures or themselves, enabling complex logic and hierarchical data processing:
CREATE PROCEDURE GetEmployeeHierarchy
@EmployeeID INT
AS
BEGIN
WITH EmployeeCTE AS (
SELECT EmployeeID, Name, ManagerID
FROM Employees
WHERE EmployeeID = @EmployeeID
UNION ALL
SELECT e.EmployeeID, e.Name, e.ManagerID
FROM Employees e
INNER JOIN EmployeeCTE ecte ON e.ManagerID = ecte.EmployeeID
)
SELECT * FROM EmployeeCTE;
END;
6. Optimization Strategies
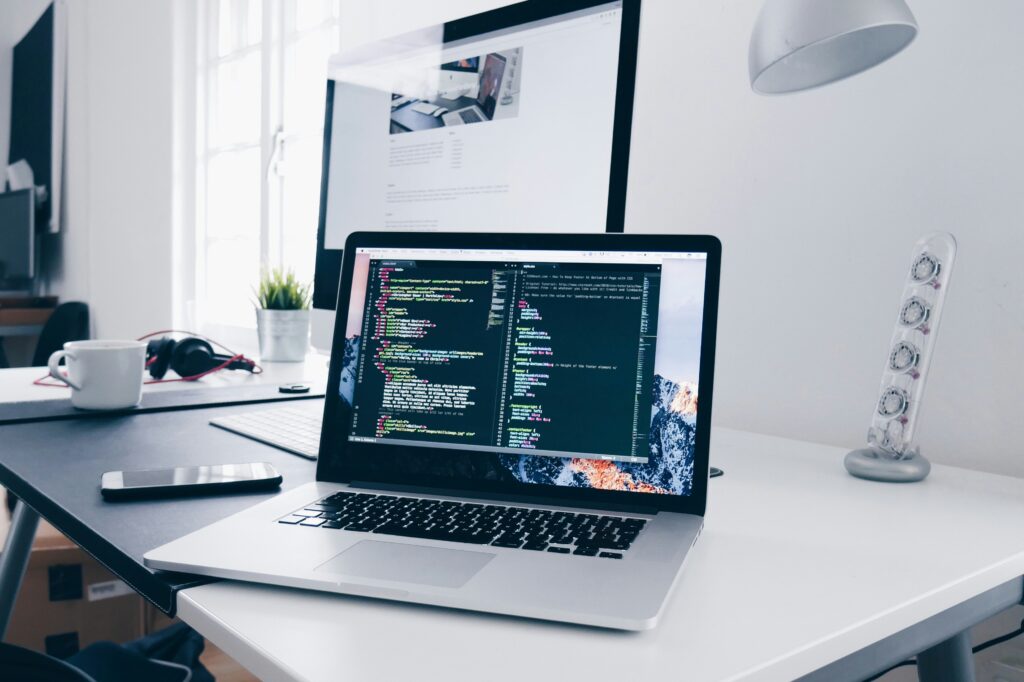
Optimizing stored procedures is crucial for maintaining high performance as your database grows.
6.1 Query optimization within stored procedures
Efficient query writing is essential for optimal procedure performance:
- Use appropriate indexing on frequently queried columns.
- Avoid using
SELECT *
and instead specify only the required columns. - Use JOINs instead of subqueries where possible.
- Utilize table variables or temporary tables for complex intermediate results.
6.2 Indexing considerations
Proper indexing can significantly improve the performance of stored procedures:
- Create indexes on columns frequently used in WHERE clauses and JOIN conditions.
- Consider covering indexes for queries that select a subset of columns.
- Regularly analyze and update statistics to ensure the query optimizer uses the most efficient execution plans.
6.3 Execution plan analysis
Analyzing execution plans helps identify performance bottlenecks:
- Use tools like SQL Server Management Studio’s execution plan viewer or MySQL’s EXPLAIN statement.
- Look for table scans, which might indicate missing indexes.
- Identify expensive operations like sorts or hash joins that could be optimized.
7. Security Aspects
Ensuring the security of stored procedures is paramount in protecting your database and application.
7.1 Role-based access control
Implement granular access control to restrict procedure execution:
-- Create a role
CREATE ROLE SalesRole;
-- Grant execute permission on a specific procedure
GRANT EXECUTE ON GetSalesReport TO SalesRole;
-- Assign the role to a user
EXEC sp_addrolemember 'SalesRole', 'SalesUser';
7.2 SQL injection prevention
Use parameterized queries to prevent SQL injection attacks:
CREATE PROCEDURE SafeSearch
@SearchTerm NVARCHAR(100)
AS
BEGIN
SELECT * FROM Products WHERE ProductName LIKE @SearchTerm + '%';
END;
7.3 Encryption and data protection
Protect sensitive data within stored procedures:
- Use encryption functions for storing and retrieving sensitive data.
- Implement data masking techniques for certain output.
- Consider using always encrypted features provided by some database systems.
8. Stored Procedures vs. Other Database Objects
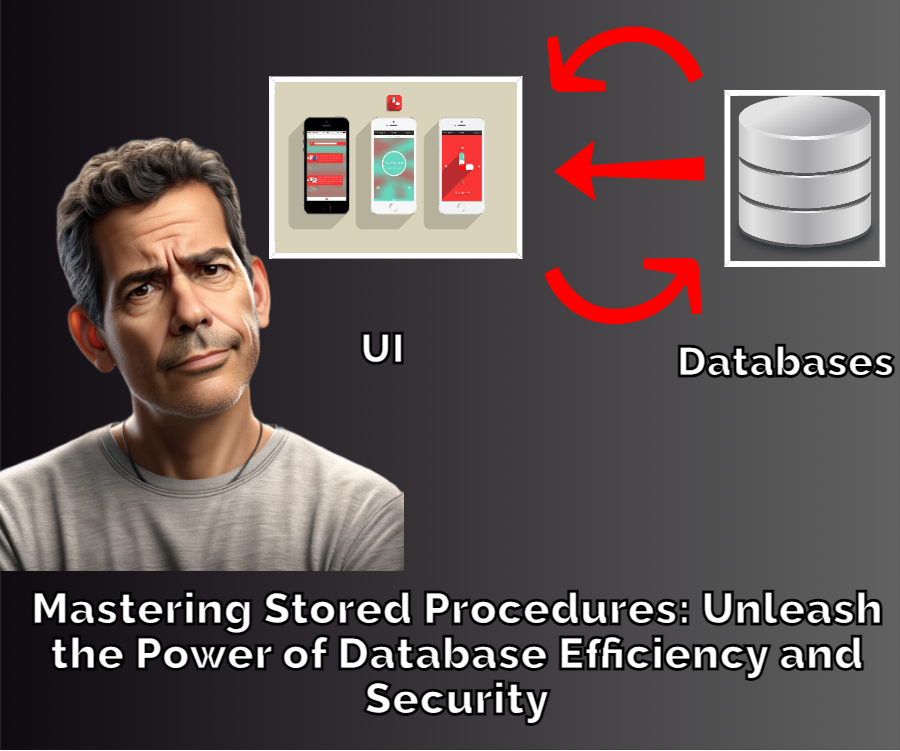
Understanding when to use stored procedures over other database objects is crucial for optimal database design.
8.1 Comparison with views
While both stored procedures and views can encapsulate complex queries, they serve different purposes:
- Views are virtual tables that present data from one or more tables. They are best used for:
- Simplifying complex joins
- Implementing row-level security
- Presenting a consistent interface to data
- Stored Procedures are more versatile and can:
- Perform complex calculations
- Modify data across multiple tables
- Implement business logic and flow control
8.2 Comparison with user-defined functions
User-defined functions (UDFs) and stored procedures have some similarities but distinct use cases:
- UDFs are best for:
- Returning a single value or table
- Use within SELECT statements or WHERE clauses
- Deterministic operations
- Stored Procedures excel at:
- Performing multiple operations
- Modifying data (INSERT, UPDATE, DELETE)
- Returning multiple result sets
8.3 When to use stored procedures
Stored procedures are ideal in scenarios such as:
- Implementing complex business logic that requires multiple steps
- Encapsulating data access for security purposes
- Batch processing large amounts of data
- Creating an API layer for database interactions
9. Stored Procedures in Different Database Systems
While the core concepts remain similar, the implementation of stored procedures can vary across different database management systems.
9.1 MySQL
MySQL supports stored procedures with some unique features:
DELIMITER //
CREATE PROCEDURE GetTopCustomers(IN topN INT)
BEGIN
SELECT CustomerName, TotalPurchases
FROM Customers
ORDER BY TotalPurchases DESC
LIMIT topN;
END //
DELIMITER ;
CALL GetTopCustomers(5);
9.2 SQL Server
SQL Server offers robust support for stored procedures:
CREATE PROCEDURE UpdateProductPrice
@ProductID INT,
@NewPrice DECIMAL(10,2)
AS
BEGIN
UPDATE Products
SET Price = @NewPrice
WHERE ProductID = @ProductID;
SELECT @@ROWCOUNT AS UpdatedRows;
END;
EXEC UpdateProductPrice @ProductID = 1, @NewPrice = 19.99;
9.3 Oracle
Oracle PL/SQL provides powerful features for stored procedures:
CREATE OR REPLACE PROCEDURE CalculateBonus(
p_EmployeeID IN NUMBER,
p_Bonus OUT NUMBER
)
IS
v_Salary NUMBER;
BEGIN
SELECT Salary INTO v_Salary
FROM Employees
WHERE EmployeeID = p_EmployeeID;
p_Bonus := v_Salary * 0.1;
EXCEPTION
WHEN NO_DATA_FOUND THEN
p_Bonus := 0;
END;
DECLARE
v_Bonus NUMBER;
BEGIN
CalculateBonus(101, v_Bonus);
DBMS_OUTPUT.PUT_LINE('Bonus: ' || v_Bonus);
END;
9.4 PostgreSQL
PostgreSQL supports stored procedures with its own unique syntax:
CREATE OR REPLACE PROCEDURE TransferFunds(
sender_id INT,
receiver_id INT,
amount DECIMAL
)
LANGUAGE plpgsql
AS $$
BEGIN
-- Deduct from sender
UPDATE accounts SET balance = balance - amount
WHERE id = sender_id;
-- Add to receiver
UPDATE accounts SET balance = balance + amount
WHERE id = receiver_id;
COMMIT;
END;
$$;
CALL TransferFunds(1, 2, 100.00);
10. Testing and Debugging
Thorough testing and effective debugging are crucial for developing reliable stored procedures.
10.1 Unit testing stored procedures
Implement unit tests to ensure the correctness of your stored procedures:
CREATE PROCEDURE TestCalculateTotal
AS
BEGIN
DECLARE @Result DECIMAL(10,2);
EXEC CalculateTotal 1, '2024-03-15', @Result OUTPUT;
IF @Result <> 150.00
BEGIN
THROW 50000, 'CalculateTotal failed', 1;
END
END;
10.2 Debugging techniques
Use built-in debugging tools and logging for effective troubleshooting:
- Set breakpoints in stored procedures using database management tools.
- Implement error logging within procedures:
CREATE PROCEDURE DebugExample
AS
BEGIN
BEGIN TRY
-- Your code here
PRINT 'Step 1 completed';
-- More code
PRINT 'Step 2 completed';
END TRY
BEGIN CATCH
INSERT INTO ErrorLog (ErrorMessage, ErrorLine, ErrorProcedure)
VALUES (ERROR_MESSAGE(), ERROR_LINE(), ERROR_PROCEDURE());
THROW;
END CATCH
END;
10.3 Performance profiling
Use profiling tools to identify performance bottlenecks:
– Utilize built-in performance analysis tools like SQL Server Profiler or MySQL Performance Schema. – Monitor execution time and resource usage:
DECLARE @StartTime DATETIME, @EndTime DATETIME;
SET @StartTime = GETDATE();
EXEC YourStoredProcedure;
SET @EndTime = GETDATE();
SELECT DATEDIFF(MILLISECOND, @StartTime, @EndTime) AS ExecutionTimeMS;
END;