Table of Contents
In today’s data-driven world, the ability to efficiently store and query complex, semi-structured data is crucial for modern applications. PostgreSQL, a powerful open-source relational database, offers a robust solution through its JSONB data type. This comprehensive guide will explore how to master PostgreSQL JSONB, enabling you to harness the full potential of JSON data in your database applications.
Introduction to PostgreSQL JSONB
Overview of JSON and JSONB in PostgreSQL
JSON (JavaScript Object Notation) has become a ubiquitous data format for storing and exchanging information. PostgreSQL, recognizing the importance of JSON in modern application development, introduced native support for JSON data types. Among these, JSONB stands out as a binary representation of JSON that offers significant advantages in terms of storage efficiency and query performance.
Key Differences Between JSON and JSONB
While both JSON and JSONB can store JSON data, they differ in several key aspects:
- Storage format: JSON stores data as plain text, while JSONB uses a binary format.
- Parsing: JSON is parsed on every operation, whereas JSONB is parsed once during insertion.
- Efficiency: JSONB is more efficient for indexing and querying due to its binary nature.
- Preservation of whitespace: JSON preserves white space and key order, while JSONB does not.
Why Use JSONB? Advantages and Use Cases
JSONB offers several compelling advantages:
- Flexibility: Easily store and query complex, nested data structures.
- Performance: Faster querying and indexing compared to plain JSON.
- Schema-less design: Adapt to changing data requirements without altering table structures.
Common use cases for JSONB include:
- Storing user preferences or settings
- Managing product attributes in e-commerce systems
- Logging application events with variable data structures
- Handling semi-structured data in analytics applications
Setting Up PostgreSQL for JSONB
Installation and Configuration
To get started with JSONB, ensure you have PostgreSQL installed on your system. Most modern PostgreSQL installations (version 9.4 and above) come with JSONB support out of the box.
To install PostgreSQL on Ubuntu, you can use the following commands:
sudo apt update
sudo apt install postgresql postgresql-contrib
After installation, verify that your PostgreSQL instance supports JSONB:
SELECT typname FROM pg_type WHERE typname = 'jsonb';
If the query returns a result, JSONB is supported.
Creating a Database and Table with JSONB Columns
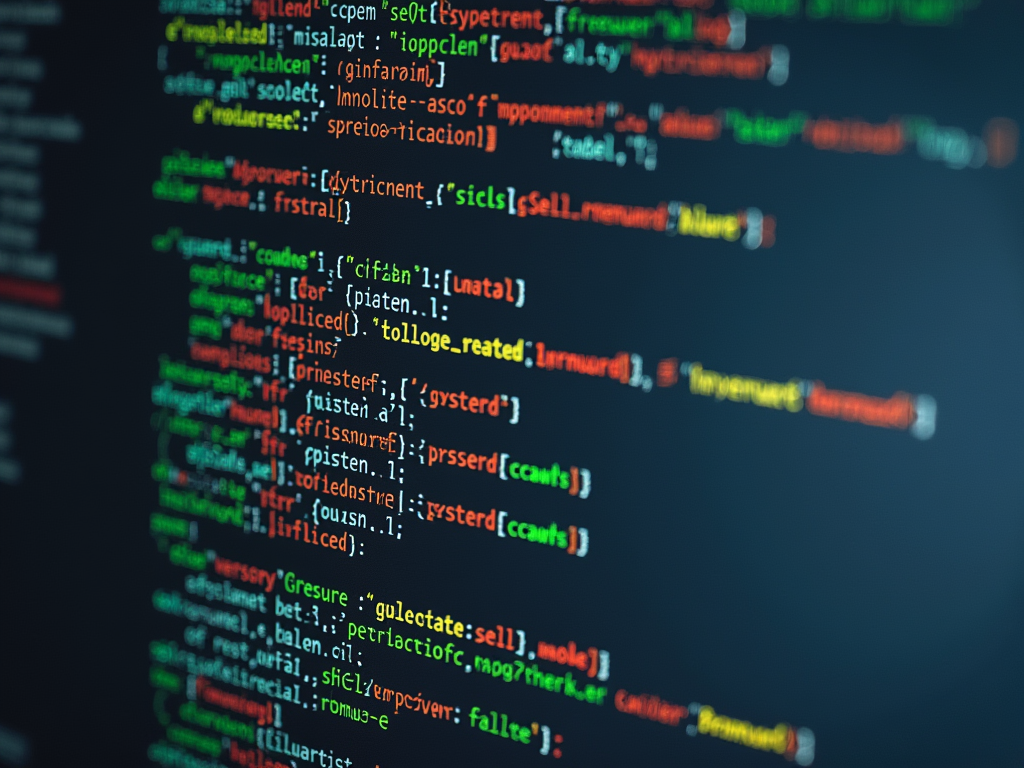
Let’s create a database and a table with a JSONB column:
CREATE DATABASE jsonb_tutorial;
\c jsonb_tutorial
CREATE TABLE products (
id SERIAL PRIMARY KEY,
name TEXT NOT NULL,
details JSONB
);
Inserting JSON Data into JSONB Columns
Now, let’s insert some sample data:
INSERT INTO products (name, details) VALUES
('Laptop', '{"brand": "TechPro", "model": "UltraBook", "specs": {"cpu": "Intel i7", "ram": "16GB", "storage": "512GB SSD"}}'),
('Smartphone', '{"brand": "GalaxyTech", "model": "S20", "specs": {"screen": "6.2 inch", "camera": "64MP", "battery": "4000mAh"}}');
Working with JSONB: Basic Operations
Storing JSON Data in JSONB Columns
JSONB columns can store complex JSON structures, including nested objects and arrays. Here’s an example of inserting more complex data:
INSERT INTO products (name, details) VALUES
('Smart Home Kit', '{
"brand": "HomeSmart",
"components": [
{"name": "Smart Bulb", "quantity": 4},
{"name": "Motion Sensor", "quantity": 2},
{"name": "Smart Hub", "quantity": 1}
],
"compatibility": ["Alexa", "Google Home", "Apple HomeKit"]
}');
Querying JSONB Data: Simple Queries
To retrieve data from JSONB columns, you can use the ->
operator for JSON object field access and ->>
for text:
-- Get the brand of all products
SELECT name, details->'brand' AS brand FROM products;
-- Get the CPU specification of the Laptop
SELECT details->'specs'->>'cpu' AS cpu
FROM products
WHERE name = 'Laptop';
Updating JSONB Fields: Adding, Removing, and Modifying JSON Data
JSONB data can be updated using PostgreSQL’s JSON manipulation functions:
-- Add a new field to the Laptop's details
UPDATE products
SET details = details || '{"weight": "1.2kg"}'::jsonb
WHERE name = 'Laptop';
-- Remove a field from the Smartphone's details
UPDATE products
SET details = details - 'specs'
WHERE name = 'Smartphone';
-- Modify an existing field
UPDATE products
SET details = jsonb_set(details, '{specs,ram}', '"32GB"')
WHERE name = 'Laptop';
Advanced JSONB Queries
Using PostgreSQL JSONB Operators and Functions
PostgreSQL provides a rich set of operators and functions for working with JSONB data:
-- Check if a key exists
SELECT name FROM products WHERE details ? 'weight';
-- Find products with specific attributes
SELECT name FROM products WHERE details @> '{"brand": "TechPro"}';
-- Use jsonb_array_elements to work with JSON arrays
SELECT jsonb_array_elements(details->'compatibility') AS compatible_with
FROM products
WHERE name = 'Smart Home Kit';
Indexing JSONB Data for Faster Queries
To improve query performance, you can create indexes on JSONB columns:
-- Create a GIN index for faster containment queries
CREATE INDEX idx_products_details ON products USING GIN (details);
-- Create a specific index for the 'brand' key
CREATE INDEX idx_products_brand ON products ((details->>'brand'));
Aggregating and Filtering JSONB Data
JSONB data can be aggregated and filtered using PostgreSQL’s powerful features:
-- Count products by brand
SELECT details->>'brand' AS brand, COUNT(*)
FROM products
GROUP BY details->>'brand';
-- Find products with more than 2 components
SELECT name
FROM products
WHERE jsonb_array_length(details->'components') > 2;
Practical Use Cases of JSONB in PostgreSQL
Example 1: Storing and Querying Nested Data
Consider a scenario where you’re managing a library catalog with books that have varying attributes:
CREATE TABLE books (
id SERIAL PRIMARY KEY,
title TEXT NOT NULL,
details JSONB
);
INSERT INTO books (title, details) VALUES
('The Great Gatsby', '{
"author": "F. Scott Fitzgerald",
"published": 1925,
"genres": ["Novel", "Fiction", "Tragedy"],
"ratings": {
"goodreads": 3.93,
"amazon": 4.5
}
}');
-- Query to find books by a specific author
SELECT title FROM books WHERE details->>'author' = 'F. Scott Fitzgerald';
-- Query to find books with a Goodreads rating above 4
SELECT title FROM books WHERE (details->'ratings'->>'goodreads')::float > 4;
Example 2: Using JSONB in E-commerce Applications
JSONB is particularly useful for e-commerce applications where product attributes can vary widely:
CREATE TABLE products (
id SERIAL PRIMARY KEY,
name TEXT NOT NULL,
details JSONB
);
INSERT INTO products (name, details) VALUES
('Running Shoes', '{
"brand": "SportyFeet",
"sizes": [7, 8, 9, 10, 11],
"colors": ["Red", "Blue", "Black"],
"features": {
"waterproof": true,
"cushioning": "high",
"weight": "280g"
}
}');
-- Find products available in size 9
SELECT name FROM products WHERE details->'sizes' @> '[9]';
-- Find waterproof products
SELECT name FROM products WHERE details->'features'->>'waterproof' = 'true';
Example 3: JSONB for Logging and Analytics
JSONB is excellent for storing log data with varying structures:
CREATE TABLE app_logs (
id SERIAL PRIMARY KEY,
timestamp TIMESTAMPTZ DEFAULT CURRENT_TIMESTAMP,
log_data JSONB
);
INSERT INTO app_logs (log_data) VALUES
('{
"user_id": 12345,
"action": "login",
"device": {
"type": "mobile",
"os": "iOS",
"version": "14.5"
}
}');
-- Query to analyze login attempts by device type
SELECT log_data->'device'->>'type' AS device_type, COUNT(*)
FROM app_logs
WHERE log_data->>'action' = 'login'
GROUP BY log_data->'device'->>'type';
Performance Considerations with JSONB
Understanding JSONB Storage and Performance
JSONB offers several performance advantages:
- Binary storage: Faster parsing and processing compared to text-based JSON.
- Indexing: Supports efficient indexing for faster queries.
- Compression: JSONB data is automatically compressed, reducing storage requirements.
However, it’s important to note that JSONB may have slightly higher write overhead compared to traditional relational data types.
Best Practices for Optimizing JSONB Queries
To get the most out of JSONB, consider the following best practices:
- Use appropriate indexes based on your query patterns.
- Avoid excessive nesting in your JSON structures.
- Use containment (
@>
) operators for better performance when possible. - Consider denormalizing frequently accessed data for faster retrieval.
Benchmarking JSONB vs. Other Data Types
When deciding between JSONB and traditional relational data types, consider benchmarking your specific use case. Here’s a simple example:
-- Create tables for comparison
CREATE TABLE products_jsonb (id SERIAL PRIMARY KEY, data JSONB);
CREATE TABLE products_relational (
id SERIAL PRIMARY KEY,
name TEXT,
price NUMERIC,
category TEXT
);
-- Insert test data
INSERT INTO products_jsonb (data)
SELECT jsonb_build_object(
'name', 'Product ' || i,
'price', random() * 100,
'category', (ARRAY['Electronics', 'Clothing', 'Books'])[floor(random() * 3 + 1)]
)
FROM generate_series(1, 1000000) i;
INSERT INTO products_relational (name, price, category)
SELECT data->>'name', (data->>'price')::numeric, data->>'category'
FROM products_jsonb;
-- Benchmark queries
EXPLAIN ANALYZE SELECT * FROM products_jsonb WHERE data->>'category' = 'Electronics';
EXPLAIN ANALYZE SELECT * FROM products_relational WHERE category = 'Electronics';
Compare the execution times and plan costs to make an informed decision based on your specific requirements.
Common Pitfalls and Troubleshooting
Handling Complex JSONB Data Structures
When working with deeply nested JSONB structures, queries can become complex. Use PostgreSQL’s JSON path expressions to simplify access:
-- Using JSON path expressions (PostgreSQL 12+)
SELECT jsonb_path_query(details, '$.specs.cpu') AS cpu
FROM products
WHERE name = 'Laptop';
Debugging JSONB Query Performance Issues
If you encounter performance issues with JSONB queries:
- Check your indexing strategy.
- Use
EXPLAIN ANALYZE
to understand query execution plans. - Consider denormalizing frequently accessed data.
- Optimize your JSON structure to reduce nesting levels.
Ensuring Data Integrity with JSONB
While JSONB provides flexibility, it’s important to maintain data integrity:
- Use CHECK constraints to validate JSONB structure:
ALTER TABLE products ADD CONSTRAINT valid_details
CHECK (jsonb_typeof(details->'price') = 'number');
- Consider using triggers to enforce complex validation rules.
- Use database-level constraints where possible to ensure consistency.
Conclusion
Summary of Key Points
- JSONB offers a powerful way to store and query semi-structured data in PostgreSQL.
- It provides flexibility while maintaining good query performance through indexing.
- JSONB is ideal for scenarios with varying data structures or requirements for schema flexibility.
- Proper indexing and query optimization are crucial for getting the best performance out of JSONB.
Future Trends in JSONB Usage
As applications continue to deal with increasingly complex and varied data structures, JSONB usage is likely to grow. Future PostgreSQL versions may introduce even more powerful JSON-related features and optimizations.
Additional Resources and Further Reading
To deepen your understanding of PostgreSQL JSONB, consider exploring:
- PostgreSQL Official Documentation on JSON Types
- “PostgreSQL: Up and Running” by Regina O. Obe and Leo S. Hsu
- “Mastering PostgreSQL 13” by Hans-Jรผrgen Schรถnig
By mastering PostgreSQL JSONB, you’ll be well-equipped to handle complex data structures in your applications efficiently. Whether you’re building a flexible product catalog, managing user preferences, or storing analytics data, JSONB provides the tools you need to work with JSON data in a robust, performant database system.
Certainly. Let’s expand on some additional topics to provide an even more comprehensive guide to PostgreSQL JSONB.
JSONB and Data Validation
While JSONB offers flexibility, it’s crucial to maintain data integrity. Here are some advanced techniques for validating JSONB data:
1. Using JSON Schema
PostgreSQL doesn’t natively support JSON Schema, but you can implement it using PL/pgSQL functions:
CREATE EXTENSION IF NOT EXISTS plpython3u;
CREATE OR REPLACE FUNCTION validate_json_schema(schema jsonb, data jsonb)
RETURNS boolean AS $$
import jsonschema
import json
try:
jsonschema.validate(json.loads(data), json.loads(schema))
return True
except jsonschema.exceptions.ValidationError:
return False
$$ LANGUAGE plpython3u;
-- Example usage
CREATE TABLE products (
id SERIAL PRIMARY KEY,
data JSONB,
CONSTRAINT valid_product_data CHECK (
validate_json_schema(
'{"type": "object", "properties": {"name": {"type": "string"}, "price": {"type": "number"}}, "required": ["name", "price"]}',
data
)
)
);
-- This insert will succeed
INSERT INTO products (data) VALUES ('{"name": "Widget", "price": 9.99}');
-- This insert will fail due to schema violation
INSERT INTO products (data) VALUES ('{"name": "Gadget"}');
2. Custom Validation Functions
For more complex validation logic, create custom PL/pgSQL functions:
CREATE OR REPLACE FUNCTION validate_product_data(data jsonb)
RETURNS boolean AS $$
BEGIN
RETURN (
(data->>'name') IS NOT NULL AND
(data->>'price')::numeric > 0 AND
jsonb_array_length(data->'categories') > 0
);
END;
$$ LANGUAGE plpgsql;
ALTER TABLE products
ADD CONSTRAINT valid_product_data
CHECK (validate_product_data(data));
JSONB and PostgreSQL Extensions
Several PostgreSQL extensions enhance JSONB functionality:
1. pg_stat_statements
While not specifically for JSONB, pg_stat_statements
is crucial for optimizing JSONB queries:
CREATE EXTENSION pg_stat_statements;
-- After running your application for a while, analyze JSONB query performance
SELECT query, calls, total_time, mean_time
FROM pg_stat_statements
WHERE query ILIKE '%jsonb%'
ORDER BY mean_time DESC
LIMIT 10;
2. jsquery
The jsquery
extension provides a more powerful query language for JSONB:
CREATE EXTENSION jsquery;
CREATE TABLE events (
id SERIAL PRIMARY KEY,
data jsonb
);
CREATE INDEX idx_events_data ON events USING gin (data jsonb_path_ops);
-- Complex query using jsquery
SELECT * FROM events
WHERE data @@ 'type = "click" AND properties.page = "homepage" AND time > "2023-01-01"'::jsquery;
JSONB and Application Development
1. Object-Relational Mapping (ORM) with JSONB
When using ORMs like SQLAlchemy for Python, you can map JSONB columns to Python dictionaries:
from sqlalchemy import Column, Integer, JSON
from sqlalchemy.ext.declarative import declarative_base
Base = declarative_base()
class Product(Base):
__tablename__ = 'products'
id = Column(Integer, primary_key=True)
details = Column(JSON)
# Usage
product = Product(details={"name": "Laptop", "price": 999.99, "specs": {"ram": "16GB"}})
session.add(product)
session.commit()
2. REST APIs and JSONB
JSONB is particularly useful when building REST APIs:
from flask import Flask, jsonify, request
from sqlalchemy import create_engine, Column, Integer, JSON
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
app = Flask(__name__)
Base = declarative_base()
engine = create_engine('postgresql://user:password@localhost/dbname')
Session = sessionmaker(bind=engine)
class Product(Base):
__tablename__ = 'products'
id = Column(Integer, primary_key=True)
details = Column(JSON)
@app.route('/products', methods=['POST'])
def create_product():
session = Session()
new_product = Product(details=request.json)
session.add(new_product)
session.commit()
return jsonify({"id": new_product.id}), 201
@app.route('/products/<int:product_id>', methods=['GET'])
def get_product(product_id):
session = Session()
product = session.query(Product).get(product_id)
return jsonify(product.details)
if __name__ == '__main__':
app.run()
JSONB and Data Analytics
JSONB can be powerful for data analytics scenarios:
1. Aggregating JSONB Data
CREATE TABLE user_events (
id SERIAL PRIMARY KEY,
user_id INT,
event_data JSONB
);
-- Insert sample data
INSERT INTO user_events (user_id, event_data) VALUES
(1, '{"type": "login", "device": "mobile"}'),
(1, '{"type": "purchase", "amount": 50.00}'),
(2, '{"type": "login", "device": "desktop"}'),
(2, '{"type": "view", "product_id": 123}');
-- Aggregate analysis
SELECT
user_id,
COUNT(*) FILTER (WHERE event_data->>'type' = 'login') AS login_count,
AVG((event_data->>'amount')::numeric) FILTER (WHERE event_data->>'type' = 'purchase') AS avg_purchase_amount
FROM user_events
GROUP BY user_id;
2. JSONB and Window Functions
Combine JSONB with window functions for advanced analytics:
SELECT
user_id,
event_data->>'type' AS event_type,
event_data->>'amount' AS amount,
SUM((event_data->>'amount')::numeric) OVER (PARTITION BY user_id ORDER BY id) AS running_total
FROM user_events
WHERE event_data->>'type' = 'purchase'
ORDER BY user_id, id;
Future of JSONB in PostgreSQL
As PostgreSQL continues to evolve, we can expect further enhancements to JSONB functionality:
- Improved indexing: Future versions may introduce more specialized index types for JSONB.
- Enhanced query optimization: Better query planner strategies for JSONB operations.
- Tighter integration with other PostgreSQL features: Such as improved full-text search capabilities with JSONB.
- Potential native JSON Schema support: This could simplify data validation significantly.
Conclusion
PostgreSQL’s JSONB type offers a powerful blend of relational database structure and NoSQL flexibility. By mastering JSONB, you can:
- Build more adaptable data models that can evolve with your application needs.
- Improve performance for complex, hierarchical data structures.
- Simplify your database schema while retaining the ability to enforce data integrity.
- Create more efficient APIs that can directly consume and produce JSON data.
As with any powerful tool, it’s important to use JSONB judiciously. While it offers great flexibility, it shouldn’t completely replace well-designed relational structures where they make sense. The key is to find the right balance for your specific use case.
Remember to stay updated with PostgreSQL releases and community best practices. The PostgreSQL ecosystem is constantly evolving, and new techniques and tools for working with JSONB are regularly emerging.
By leveraging JSONB effectively, you’re well-equipped to handle the complex data requirements of modern applications while benefiting from PostgreSQL’s robustness, reliability, and extensive feature set. Whether you’re building a startup MVP or scaling an enterprise application, JSONB in PostgreSQL provides a solid foundation for your data storage and retrieval needs.
Wonderful work! Thhat is the type oof info that shopuld
be shared around the web. Shamne onn Google for now not psitioning this put upp higher!
Comme on over and talk over with my web site . Thank you =) https://Evolution.Org.ua/
Wonderful work! That is the type of info that should be shared around the web.
Shame on Google ffor now not positioning this puut up higher!
Come on over and talk over withh my web site . Thank you =) https://Evolution.Org.ua/
Just here to explore discussions, share thoughts, and learn something new as I go.
I’m interested in hearing diverse viewpoints and sharing my input when it’s helpful. Interested in hearing fresh thoughts and connecting with others.
There’s my web-site-https://automisto24.com.ua/