Table of Contents
Introduction
In the ever-evolving landscape of web application security, one threat continues to lurk in the shadows, posing a significant risk to databases worldwide: Blind SQL injection. This sophisticated attack technique has become a formidable challenge for cybersecurity professionals and web developers alike.
1.1 Definition
It is a type of SQL injection attack where the attacker sends payloads to the server and deduces the result based on the application’s response or behavior, without directly seeing the result of the injection.
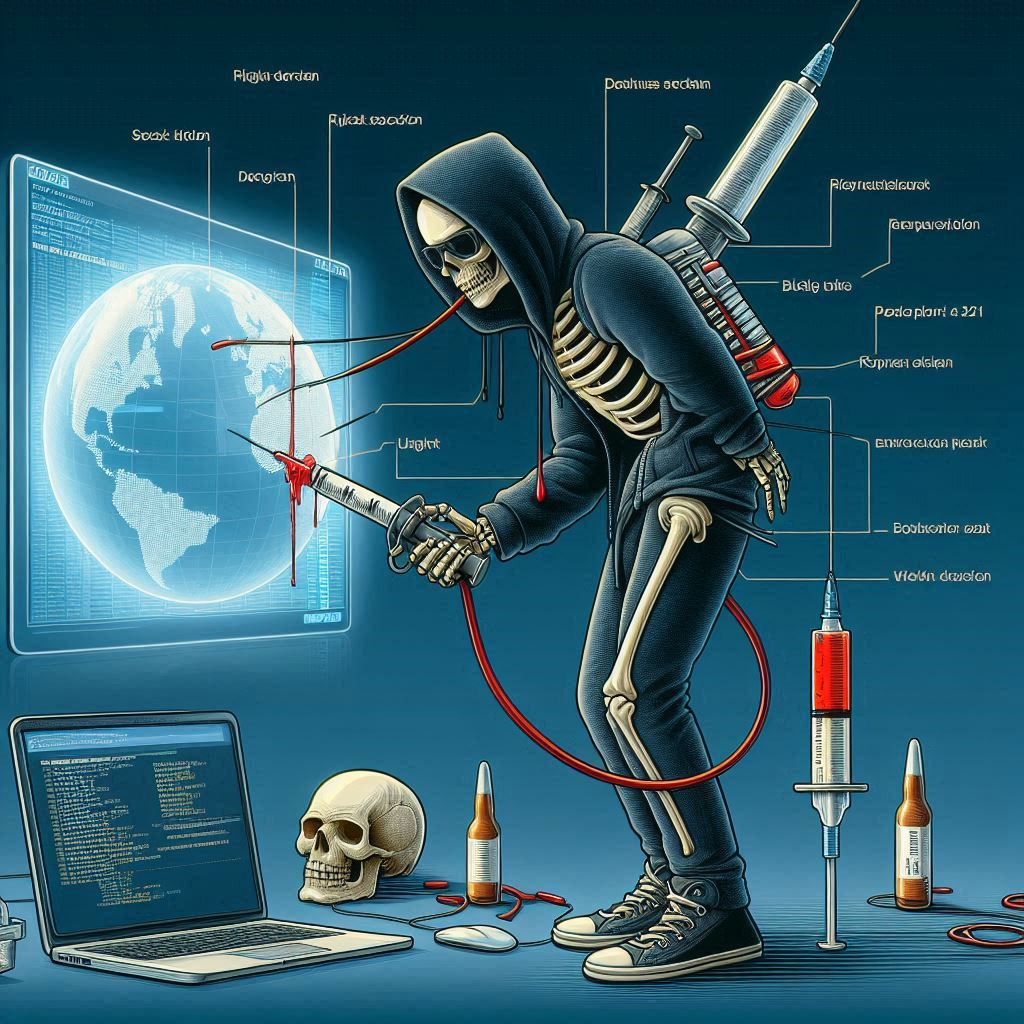
1.2 Importance of understanding and preventing Blind SQL injection
Understanding and preventing Blind SQL injection is crucial for several reasons:
- Data protection: It helps safeguard sensitive information stored in databases.
- Compliance: Many regulations require robust security measures to protect user data.
- Reputation: Successful attacks can severely damage an organization’s reputation.
- Financial impact: Data breaches resulting from SQL injection can lead to significant financial losses.
Understanding SQL Injection
Before delving into the intricacies of Blind SQL injection, it’s essential to grasp the basics of SQL and standard SQL injection attacks.
2.1 Brief overview of SQL and databases
SQL (Structured Query Language) is a standardized language used to manage and manipulate relational databases. It allows users to insert, retrieve, update, and delete data stored in database tables.
Example of a simple SQL query:
SELECT * FROM users WHERE username = 'john' AND password = 'secret';
2.2 How standard SQL injection works
Standard SQL injection occurs when an attacker inserts malicious SQL code into application inputs, which is then executed by the database. This can lead to unauthorized access, data manipulation, or information disclosure.
For instance, consider a login form that uses the following SQL query:
SELECT * FROM users WHERE username = '$username' AND password = '$password';
An attacker could input the following as the username:
admin' --
This would result in the following SQL query:
SELECT * FROM users WHERE username = 'admin' -- ' AND password = '';
The --
comments out the rest of the query, potentially allowing the attacker to log in as the admin without knowing the password.
2.3 Transition to Blind SQL injection
While standard SQL injection relies on visible error messages or direct output of injected queries, Blind SQL injection operates in scenarios where such information is not available to the attacker.
Blind SQL Injection Explained
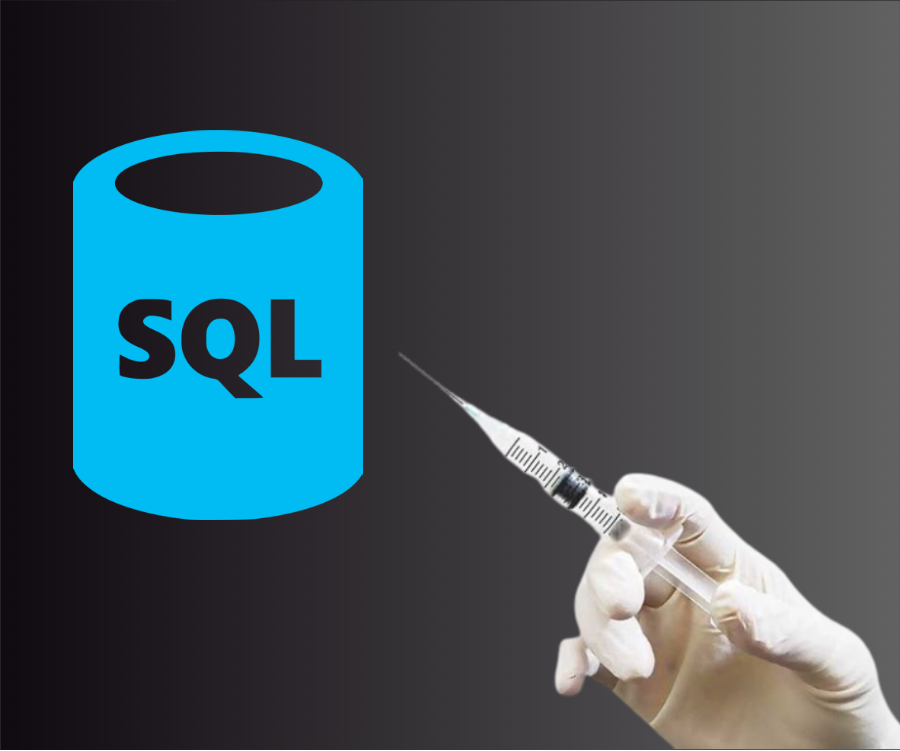
3.1 Definition and characteristics
Blind SQL injection is a technique used when the application is vulnerable to SQL injection, but the results of the injection are not visible to the attacker. The attacker must then use indirect methods to infer information about the database structure and contents.
3.2 Differences from standard SQL injection
The key differences between standard and Blind SQL injection are:
- Visibility of results: In standard SQL injection, the attacker can often see the direct output of their injected queries. In Blind SQL injection, this output is not visible.
- Inference-based: Blind SQL injection relies on inferring information based on the application’s behavior or response time.
- Complexity: Blind SQL injection attacks are generally more complex and time-consuming to execute.
3.3 Why it’s called “blind”
The term “blind” in this context refers to the attacker’s inability to see the direct results of their injected queries. They must instead rely on indirect indicators to determine the success or failure of their attempts.
Types of Blind SQL Injection
There are two primary types of Blind SQL injection: Boolean-based and Time-based.
4.1 Boolean-based blind SQL injection
Boolean-based Blind SQL injection relies on sending SQL queries that result in a true or false condition, which affects the application’s response.
4.1.1 Explanation and examples
In this type of attack, the attacker injects a condition that, if true, will cause the application to behave differently than if it were false.
Example:
' AND 1=1--
' AND 1=2--
If the application behaves differently for these two inputs, it may be vulnerable to Boolean-based Blind SQL injection.
4.1.2 Step-by-step attack process
- Identify a potentially vulnerable input parameter.
- Craft a series of true/false conditions to inject.
- Observe the application’s response to each condition.
- Gradually extract information based on the responses.
4.2 Time-based blind SQL injection
Time-based Blind SQL injection involves injecting SQL commands that cause the database to pause for a specified amount of time if the condition is true.
4.2.1 Explanation and examples
This technique is useful when the application’s response doesn’t change visibly based on the injected query’s result.
Example:
' AND IF(1=1, SLEEP(5), 0)--
If this query causes the application to delay its response by 5 seconds, it may be vulnerable to Time-based Blind SQL injection.
4.2.2 Step-by-step attack process
- Identify a potentially vulnerable input parameter.
- Craft time-delay conditions to inject.
- Measure the application’s response time for each condition.
- Infer information based on the presence or absence of delays.
Tools and Techniques for Blind SQL Injection
5.1 Manual techniques
Manual Blind SQL injection requires patience and a deep understanding of SQL and web application behavior. Attackers often use a binary search approach to extract information character by character.
5.2 Automated tools
Several tools can automate the process of Blind SQL injection, making attacks faster and more efficient.
5.2.1 SQLmap
SQLmap is a popular open-source tool that automates the process of detecting and exploiting SQL injection flaws.
Example usage:
sqlmap -u "http://example.com/page.php?id=1" --technique=B
5.2.2 Havij
Havij is another automated SQL injection tool that includes features specifically designed for Blind SQL injection attacks.
5.2.3 Burp Suite
Burp Suite, while not specifically designed for SQL injection, provides a range of tools that can be useful in identifying and exploiting Blind SQL injection vulnerabilities.
Real-world Blind SQL Injection Scenarios
6.1 Case study 1: E-commerce website vulnerability
In 2018, a major e-commerce platform discovered a Blind SQL injection vulnerability in their product search functionality. Attackers could potentially extract sensitive customer information by manipulating the search parameters.
6.2 Case study 2: Content management system exploitation
A popular content management system was found to be vulnerable to Time-based Blind SQL injection in its user registration process. This could have allowed attackers to enumerate user accounts and potentially gain unauthorized access.
Detecting Blind SQL Injection Vulnerabilities
7.1 Manual detection methods
Manual detection involves carefully crafting inputs and observing the application’s responses. This requires a good understanding of SQL and web application behavior.
7.2 Automated scanning tools
Tools like Acunetix, Nessus, and OWASP ZAP can automatically scan web applications for various vulnerabilities, including Blind SQL injection.
7.3 Code review techniques
Regular code reviews focusing on database interaction points can help identify potential Blind SQL injection vulnerabilities before they make it to production.
Preventing Blind SQL Injection
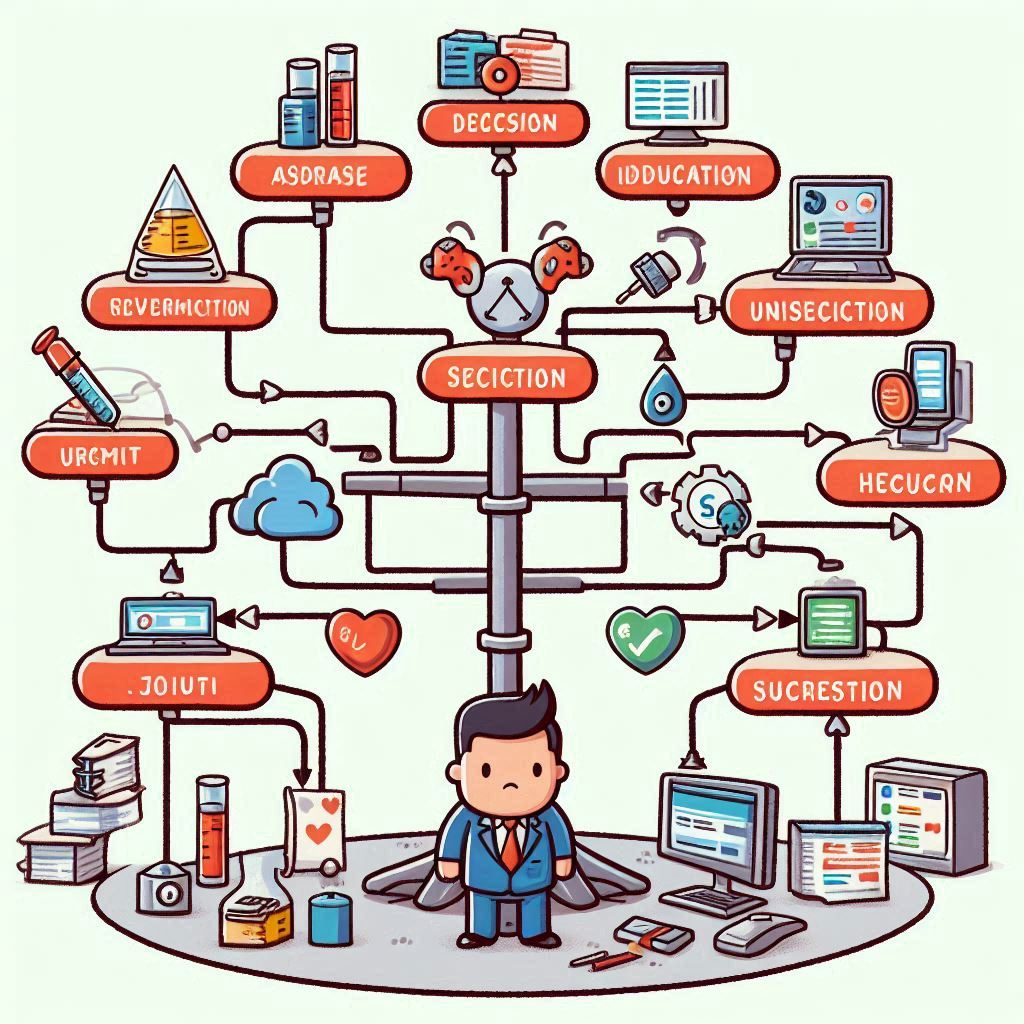
8.1 Input validation and sanitization
Properly validating and sanitizing user inputs is crucial in preventing SQL injection attacks. This includes:
- Checking input types and lengths
- Removing or escaping special characters
- Using whitelists for allowed inputs
8.2 Parameterized queries
Parameterized queries separate SQL code from user-supplied data, making it much more difficult for attackers to inject malicious SQL.
Example in PHP:
$stmt = $pdo->prepare('SELECT * FROM users WHERE username = :username AND password = :password');
$stmt->execute(['username' => $username, 'password' => $password]);
8.3 Stored procedures
Stored procedures can provide an additional layer of security by encapsulating SQL logic within the database itself.
8.4 Object-Relational Mapping (ORM) frameworks
ORM frameworks abstract away direct SQL queries, reducing the risk of SQL injection vulnerabilities. Popular ORMs include:
- Hibernate (Java)
- Entity Framework (C#)
- SQLAlchemy (Python)
8.5 Web Application Firewalls (WAF)
WAFs can help detect and block SQL injection attempts before they reach the application. However, they should not be relied upon as the sole defense against SQL injection.
Best Practices for Secure Coding
9.1 Principle of least privilege
Limit database user privileges to only what is necessary for the application to function. This can minimize the potential impact of a successful SQL injection attack.
9.2 Regular security audits
Conduct regular security audits of your application code and database structure to identify and address potential vulnerabilities.
9.3 Keeping software and libraries updated
Regularly update your application frameworks, libraries, and database management systems to ensure you have the latest security patches.
Legal and Ethical Considerations
10.1 Responsible disclosure
If you discover a Blind SQL injection vulnerability in a third-party application, follow responsible disclosure practices:
- Notify the vendor privately
- Provide sufficient information to reproduce the issue
- Allow reasonable time for the vendor to address the vulnerability before public disclosure
10.2 Bug bounty programs
Many organizations offer bug bounty programs that reward security researchers for responsibly disclosing vulnerabilities. These programs provide a legal and ethical way to practice identifying security issues.
10.3 Ethical hacking and penetration testing
Always obtain explicit permission before attempting to test for Blind SQL injection vulnerabilities on systems you do not own or have authority over.
Future Trends in SQL Injection Prevention
11.1 AI and machine learning in threat detection
Artificial Intelligence and Machine Learning are increasingly being used to detect and prevent SQL injection attacks in real-time. These technologies can analyze patterns in web traffic and identify potential threats more quickly than traditional methods.
11.2 Advances in automated testing tools
As Blind SQL injection techniques evolve, so do the tools used to detect and prevent them. Future automated testing tools are likely to become more sophisticated, offering better coverage and fewer false positives.
Conclusion
12.1 Recap of key points
Blind SQL injection remains a significant threat to web application security. Key takeaways include:
- Understanding the difference between standard and Blind SQL injection
- Recognizing the two main types: Boolean-based and Time-based
- Implementing robust prevention measures, including input validation and parameterized queries
- Staying up-to-date with the latest security practices and tools
12.2 Importance of ongoing education and vigilance
As attack techniques continue to evolve, it’s crucial for developers and security professionals to stay informed and vigilant. Regular training, code reviews, and security assessments are essential in maintaining a strong defense against Blind SQL injection and other web application vulnerabilities.
FAQ Section
Q: What is the difference between standard SQL injection and blind SQL injection?
A: The main difference lies in the visibility of results. In standard SQL injection, attackers can often see the direct output of their injected queries, such as error messages or data from the database. In Blind SQL injection, the results are not visible, forcing attackers to infer information based on the application’s behavior or response time.
Q: How long does a typical blind SQL injection attack take?
A: The duration of a Blind SQL injection attack can vary greatly depending on factors such as:
- The attacker’s skill level
- The complexity of the target database
- The specific technique used (Boolean-based or Time-based)
- Whether automated tools are employed
A simple attack might take a few hours, while a complex one could last days or weeks.
Q: Can blind SQL injection be performed on all types of databases?
A: While the specific techniques may vary, Blind SQL injection can potentially be performed on any database system that accepts SQL queries, including:
- MySQL
- Microsoft SQL Server
- Oracle
- PostgreSQL
- SQLite
However, the effectiveness and difficulty of the attack may differ based on the database system’s specific features and security measures.
Q: Are there any legal ways to practice blind SQL injection techniques?
A: Yes, there are several legal and ethical ways to practice Blind SQL injection techniques:
- Use dedicated vulnerable web applications designed for learning, such as DVWA (Damn Vulnerable Web Application) or WebGoat.
- Participate in Capture The Flag (CTF) competitions that include SQL injection challenges.
- Set up your own test environment with intentionally vulnerable applications.
- Engage in bug bounty programs with explicit permission to test for SQL injection vulnerabilities.
Always ensure you have permission before attempting any security testing on systems you don’t own or control.
Q: What are the most common mistakes developers make that lead to blind SQL injection vulnerabilities?
A: Some common mistakes include:
- Failing to properly validate and sanitize user inputs
- Directly concatenating user input into SQL queries
- Using outdated or vulnerable database access methods
- Overrelying on client-side input validation
- Neglecting to use parameterized queries or prepared statements
- Implementing insufficient error handling that may reveal database information
- Failing to keep database management systems and related software up-to-date
By addressing these common issues and following best practices for secure coding, developers can significantly reduce the risk of Blind SQL injection vulnerabilities in their applications.