As a developer, you’re constantly learning and growing. But sometimes, the best lessons come from mistakes – those moments that make you cringe and vow, “Never again!” In this comprehensive guide, we’ll explore ten common mistakes that developers often make but wish they hadn’t. By understanding these pitfalls, you can save yourself time, frustration, and potentially even your job. Let’s dive in and learn how to become a better, more efficient coder.
1. Neglecting Version Control: The Silent Killer of Collaboration
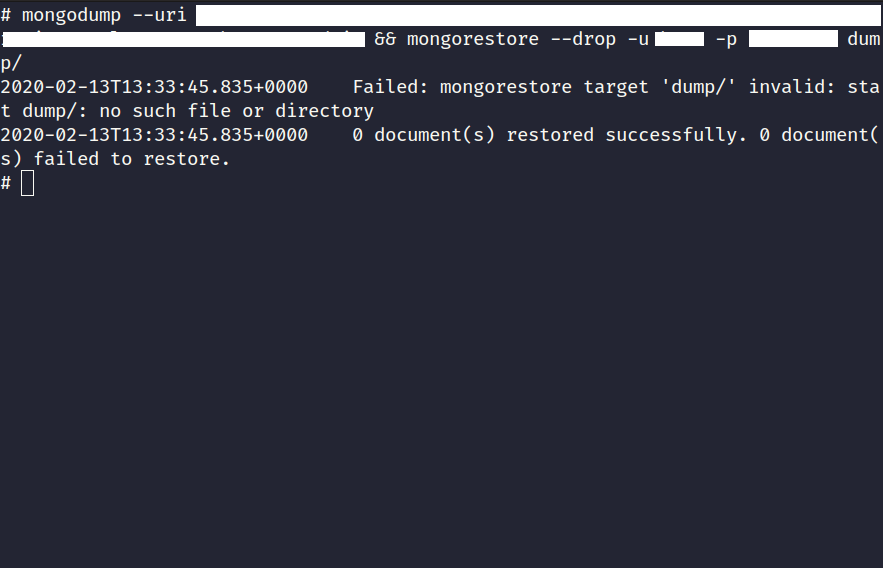
Picture this: you’ve just spent hours perfecting a feature, only to realize your colleague made conflicting changes. Without proper version control, you’re in for a world of pain.
Why It’s a Mistake
Version control is the backbone of collaborative coding. It allows multiple developers to work on the same project simultaneously, track changes, and revert to previous versions if needed. Neglecting version control can lead to:
- Lost code
- Conflicting changes
- Difficulty in tracking who made what changes
- Inability to roll back to a stable version
How to Avoid It
Embrace Git or another version control system from the start of your project. Here’s a quick example of how to get started with Git:
# Initialize a new Git repository
git init
# Add files to staging
git add .
# Commit changes
git commit -m "Initial commit"
# Create a new branch for a feature
git checkout -b new-feature
# Push changes to remote repository
git push origin new-feature
By making version control a habit, you’ll save yourself countless headaches and make collaboration a breeze.
2. Ignoring Code Documentation: Your Future Self Will Thank You
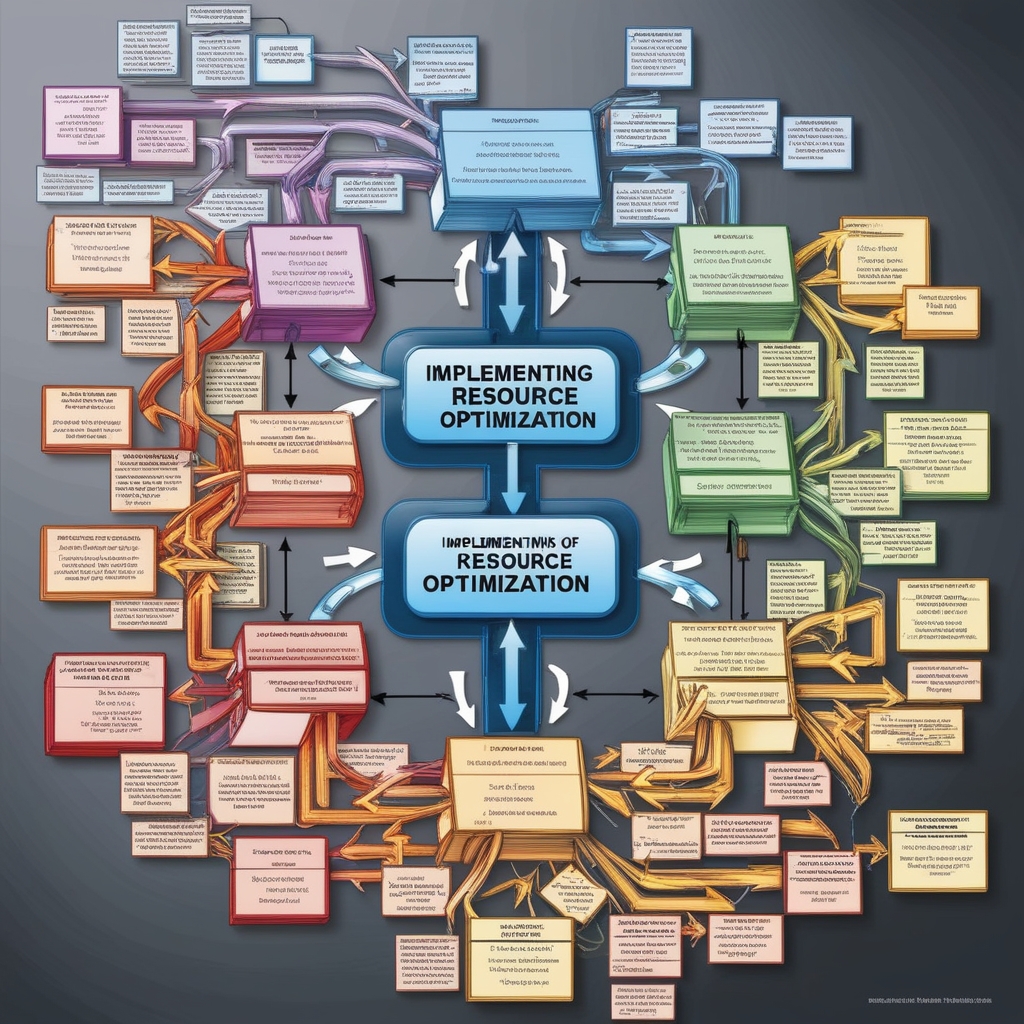
We’ve all been there – diving into a codebase with little to no documentation, feeling like we’re deciphering an ancient language. Don’t be that developer who leaves others (or your future self) in the dark.
Why It’s a Mistake
Poor documentation leads to:
- Increased onboarding time for new team members
- Difficulty in maintaining and updating code
- Frustration and wasted time trying to understand complex logic
- Higher likelihood of introducing bugs during updates
How to Avoid It
Make documentation a part of your coding process. Use clear, concise comments and create README files for your projects. Here’s an example of good documentation in Python:
def calculate_discount(price: float, discount_percentage: float) -> float:
"""
Calculate the discounted price of an item.
Args:
price (float): The original price of the item.
discount_percentage (float): The discount percentage (0-100).
Returns:
float: The discounted price.
Raises:
ValueError: If discount_percentage is not between 0 and 100.
Example:
>>> calculate_discount(100, 20)
80.0
"""
if not 0 <= discount_percentage <= 100:
raise ValueError("Discount percentage must be between 0 and 100")
discount_amount = price * (discount_percentage / 100)
return price - discount_amount
By providing clear documentation, you’re not only helping others but also creating a valuable reference for yourself.
3. Overlooking Security: Don’t Be the Weak Link
In an age of increasing cyber threats, security should be at the forefront of every developer’s mind. Yet, it’s often an afterthought.
Why It’s a Mistake
Neglecting security can lead to:
- Data breaches
- Financial losses
- Loss of user trust
- Legal consequences
How to Avoid It
Incorporate security best practices into your development process:
- Use HTTPS for all web applications
- Implement proper authentication and authorization
- Sanitize user inputs to prevent SQL injection and XSS attacks
- Keep dependencies up to date
Here’s an example of how to sanitize user input in PHP:
<?php
// Unsafe way
$username = $_POST['username'];
$query = "SELECT * FROM users WHERE username = '$username'";
// Safe way using prepared statements
$username = $_POST['username'];
$stmt = $pdo->prepare("SELECT * FROM users WHERE username = :username");
$stmt->execute(['username' => $username]);
?>
By making security a priority, you protect your users, your company, and your reputation.
4. Premature Optimization: Don’t Solve Problems You Don’t Have
It’s tempting to optimize every little detail of your code from the get-go. But as the saying goes, “Premature optimization is the root of all evil.”
Why It’s a Mistake
Obsessing over optimization too early can:
- Overcomplicate your code
- Waste time on insignificant improvements
- Make your code harder to read and maintain
- Distract from more important architectural decisions
How to Avoid It
Focus on writing clean, readable code first. Optimize only when you have identified actual performance bottlenecks through profiling. Here’s an example of how to profile Python code:
import cProfile
import pstats
def function_to_profile():
# Your code here
pass
cProfile.run('function_to_profile()', 'profile_stats')
# Analyze the results
p = pstats.Stats('profile_stats')
p.sort_stats('cumulative').print_stats(10)
By profiling your code, you can identify where optimization efforts will have the most impact, rather than guessing.
5. Ignoring Error Handling: Embrace the Exceptions
We all want our code to work perfectly, but the reality is that errors happen. Ignoring proper error handling is like driving without a seatbelt – it’s fine until it’s not.
Why It’s a Mistake
Poor error handling can lead to:
- Unexpected crashes
- Difficulty in debugging issues
- Poor user experience
- Security vulnerabilities
How to Avoid It
Implement robust error handling throughout your code. Use try-except blocks (or their equivalent in your language) and provide meaningful error messages. Here’s an example in JavaScript:
async function fetchUserData(userId) {
try {
const response = await fetch(`https://api.example.com/users/${userId}`);
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const data = await response.json();
return data;
} catch (error) {
console.error('Failed to fetch user data:', error.message);
// Handle the error appropriately (e.g., show a user-friendly message)
throw error; // Re-throw if you want calling code to handle it
}
}
By handling errors gracefully, you create more robust applications and provide a better experience for your users.
6. Neglecting Code Reviews: Your First Line of Defense
Code reviews are often seen as a bottleneck, but they’re actually one of the most valuable tools in a developer’s arsenal.
Why It’s a Mistake
Skipping code reviews can result in:
- Undetected bugs making it to production
- Inconsistent coding styles across the project
- Missed opportunities for knowledge sharing
- Accumulation of technical debt
How to Avoid It
Make code reviews a non-negotiable part of your development process. Use pull requests and encourage constructive feedback. Here’s an example of how to create a pull request on GitHub:
# Create a new branch
git checkout -b feature-branch
# Make your changes and commit them
git add .
git commit -m "Implement new feature"
# Push the branch to GitHub
git push origin feature-branch
# Then, go to GitHub and create a pull request from your branch
By embracing code reviews, you’ll catch issues early, improve code quality, and foster a culture of continuous learning.
7. Reinventing the Wheel: Don’t Ignore Existing Solutions
As developers, we love to build things from scratch. But sometimes, this desire can lead us astray.
Why It’s a Mistake
Reinventing the wheel can:
- Waste time on solving already-solved problems
- Introduce bugs that have already been fixed in existing solutions
- Result in less optimized or less secure code
- Increase maintenance burden
How to Avoid It
Before starting a new component or feature, research existing libraries and frameworks. Use package managers to easily incorporate well-maintained solutions. Here’s an example using npm for a JavaScript project:
# Initialize a new project
npm init -y
# Install a popular date manipulation library
npm install moment
# Use it in your code
const moment = require('moment');
console.log(moment().format('MMMM Do YYYY, h:mm:ss a'));
By leveraging existing solutions, you can focus on building the unique aspects of your application.
8. Ignoring Scalability: Plan for Success
It’s easy to get caught up in making your application work for the present, but what happens when success strikes and your user base grows exponentially?
Why It’s a Mistake
Failing to consider scalability can lead to:
- Performance bottlenecks as user numbers increase
- Difficulty in adding new features
- Increased costs for infrastructure
- Potential downtime during peak usage
How to Avoid It
Design your applications with scalability in mind from the start. Consider using microservices architecture, implement caching, and design efficient database schemas. Here’s an example of implementing caching in Node.js using Redis:
const redis = require('redis');
const client = redis.createClient();
async function getCachedData(key) {
return new Promise((resolve, reject) => {
client.get(key, (error, data) => {
if (error) reject(error);
resolve(data);
});
});
}
async function setCachedData(key, value, expirationInSeconds) {
return new Promise((resolve, reject) => {
client.setex(key, expirationInSeconds, value, (error, result) => {
if (error) reject(error);
resolve(result);
});
});
}
// Usage
async function getUserData(userId) {
const cacheKey = `user:${userId}`;
let userData = await getCachedData(cacheKey);
if (!userData) {
userData = await fetchUserDataFromDatabase(userId);
await setCachedData(cacheKey, JSON.stringify(userData), 3600); // Cache for 1 hour
} else {
userData = JSON.parse(userData);
}
return userData;
}
By thinking about scalability early, you’ll be prepared for growth and success.
9. Underestimating the Importance of Testing: Quality Assurance is Key
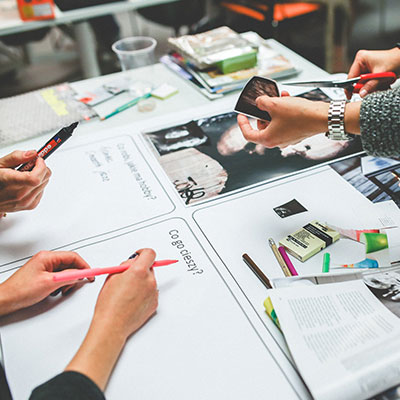
“It works on my machine” is a phrase that strikes fear into the hearts of developers everywhere. Neglecting proper testing is a surefire way to encounter this dreaded situation.
Why It’s a Mistake
Insufficient testing can result in:
- Bugs making it to production
- Regressions when adding new features
- Difficulty in refactoring code
- Decreased confidence in deployments
How to Avoid It
Implement a comprehensive testing strategy that includes unit tests, integration tests, and end-to-end tests. Use test-driven development (TDD) when appropriate. Here’s an example of a unit test in Python using pytest:
# File: calculator.py
def add(a, b):
return a + b
# File: test_calculator.py
import pytest
from calculator import add
def test_add():
assert add(2, 3) == 5
assert add(-1, 1) == 0
assert add(0, 0) == 0
def test_add_large_numbers():
assert add(1000000, 2000000) == 3000000
def test_add_floating_point():
assert abs(add(0.1, 0.2) - 0.3) < 1e-9 # Account for floating-point precision
By prioritizing testing, you’ll catch issues early, improve code quality, and deploy with confidence.
10. Neglecting Continuous Learning: The Tech World Waits for No One
In the fast-paced world of technology, standing still is equivalent to moving backward. Failing to keep up with new technologies and best practices is a mistake that can seriously impact your career.
Why It’s a Mistake
Neglecting continuous learning can lead to:
- Becoming obsolete in your field
- Missed opportunities for career advancement
- Difficulty in solving modern problems efficiently
- Decreased job satisfaction and motivation
How to Avoid It
Make learning a part of your daily routine. Set aside time for reading articles, watching tutorials, and experimenting with new technologies. Here’s a simple learning plan you can adapt:
- Subscribe to developer newsletters and blogs
- Attend local meetups or online webinars
- Contribute to open-source projects
- Set a goal to learn one new technology or concept each month
- Share your knowledge through blog posts or presentations
Remember, the goal is progress, not perfection. Even dedicating 30 minutes a day to learning can make a significant difference over time.
Conclusion: Embracing Growth and Improvement
As we’ve explored these ten common mistakes, it’s clear that being a great developer is about more than just writing code. It’s about embracing best practices, thinking ahead, and continuously improving your skills.
By avoiding these pitfalls, you’ll not only become a better developer but also a more valuable team member and a more confident professional. Remember, everyone makes mistakes – the key is to learn from them and use that knowledge to grow.
So, take these lessons to heart, apply them in your daily work, and watch as your code quality improves, your projects succeed, and your career flourishes. Happy coding!