State management in React applications can sometimes be as straightforward or as complex as the application’s demands. Frontend developers constantly seek tools and practices that streamline this aspect, ensuring both scalability and maintainability. One such recent development is the useOptimistic
custom hook, a tool designed to manage state changes in React with an emphasis on optimistic updates. This approach improves user experience by anticipating state changes before they are confirmed, enhancing the perception of a fast, responsive interface. As we dive into the nuances of using useOptimistic
, we will explore how it functions, its benefits, and how best to implement it in your projects.
Understanding the useOptimistic Hook in React
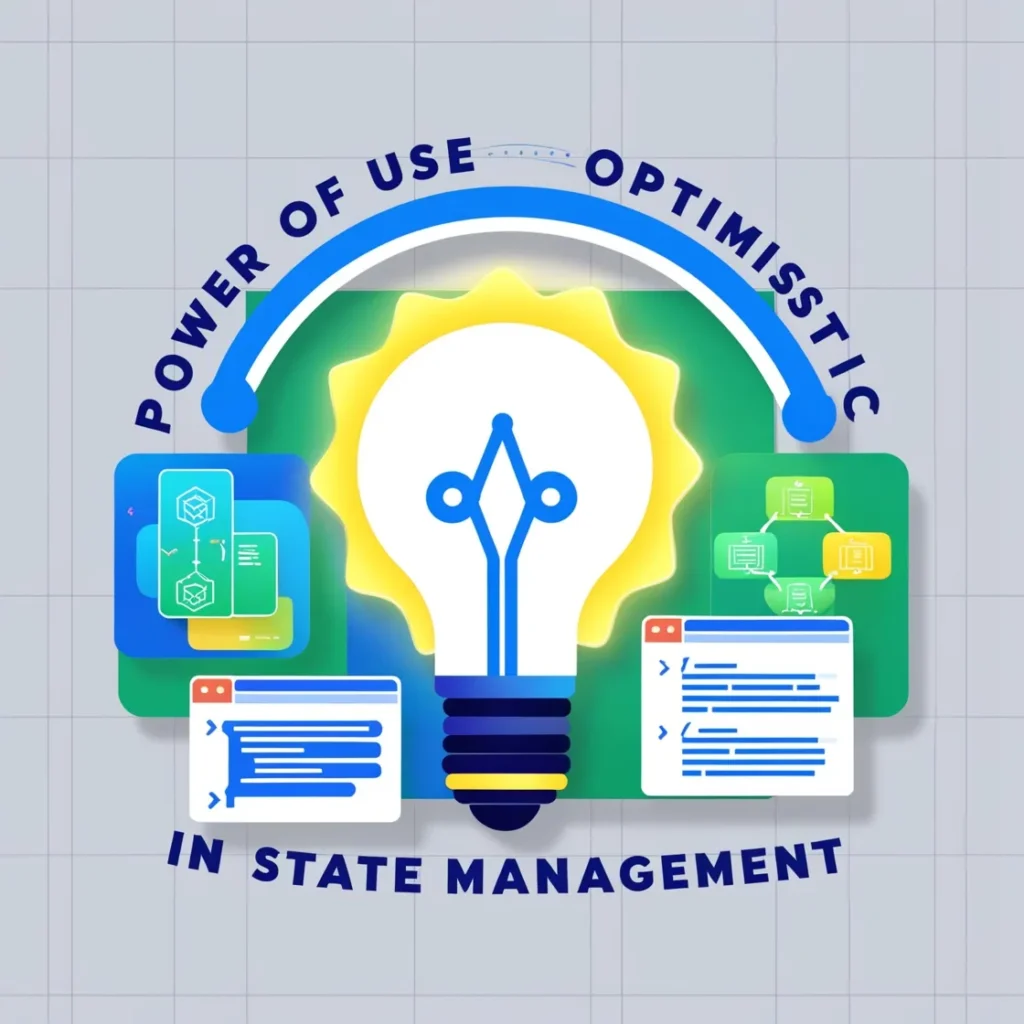
The useOptimistic hook in React is a custom hook designed to enhance frontend performance by preemptively updating the user interface before data changes are confirmed by the backend. This method, typically applied in complex state management scenarios, assumes that actions taken will succeed without errors. The hook temporarily updates the state based on user interactions, providing immediate feedback and a smoother experience.
This approach falls under the broader concept known as optimistic UI, where changes to the user interface are displayed as if they were successful, reverting only if an error occurs. The useOptimistic hook helps manage this pattern by providing a simple and structured way to handle these state transitions within React applications.
Benefits
Incorporating the useOptimistic hook into your React project offers several advantages:
1. Enhanced User Experience: By updating UI elements immediately, users perceive operations as faster, which is crucial in creating a responsive and engaging application.
2. Reduced Server Load: Since the UI updates without waiting for server responses, there is less need for loading states, which can reduce the number of requests made to the server.
3. Simplified Complexity in Handling State Updates: useOptimistic abstracts away some of the complexities involved with managing temporary state changes, making the codebase cleaner and easier to maintain.
4. Greater Resilience: By assuming success and only handling errors as exceptions, applications become more robust and less prone to failures affecting the user interface.
These benefits contribute to more maintainable and user-friendly applications, making useOptimistic a valuable tool in the arsenal of modern React developers.
Implementing useOptimistic effectively
To implement the useOptimistic hook effectively, follow these steps:
1. Identify Appropriate Use Cases: Not all state changes benefit from optimistic updates. Use it for instances where user feedback is critical, such as in form submissions or updating personal data.
2. Handle Reversions Gracefully: Prepare your application to handle situations where the optimistic UI needs to revert to its previous state due to an error. This might involve providing user notifications and ensuring the state is returned accurately.
3. Synchronize with Backend Operations: Ensure that your backend and frontend are synchronized correctly after optimistic updates. This might involve confirming data integrity and consistency once the server responds.
4. Test Thoroughly: Given the complexities of state management in dynamic applications, thorough testing is essential. Ensure that edge cases, especially those involving failed optimistic updates, are rigorously tested.
By following these guidelines, developers can utilize the power of useOptimistic to make their React applications more efficient and pleasant to use.
Best Practices for Optimizing State Management
Reducing latency with useOptimistic
Reducing latency in web applications is crucial for maintaining user satisfaction and engagement. The useOptimistic hook can play a pivotal role in achieving lower latency by allowing state updates to proceed without waiting for server-side confirmations. Here’s how you can leverage useOptimistic to minimize latency:
- Frontend Performance: Implement the useOptimistic hook in scenarios where the delay between the user action and the visual feedback can be perceptible. This is particularly relevant in data-intensive operations.
- Selective Use: Apply the useOptimistic hook selectively to operations where delays are most detrimental to the user experience, such as real-time content editing or highly interactive features.
- Cache Server Responses: Combine the use of useOptimistic with caching strategies to reduce the frequency and duration of server requests, further improving responsiveness.
By integrating these strategies, developers can ensure that their applications are not just functionally rich but also performance-optimized.
Improving user experience through state optimization
The ultimate goal of using hooks like useOptimistic is to enhance the user experience by making applications feel faster and more responsive. Here are several techniques to further this objective:
- Predictive Fetching: Anticipate user actions and fetch data in advance to populate the optimistic states, reducing the apparent wait times for subsequent interactions.
- UI Feedback: Provide clear visual or textual feedback during the optimistic update process. For instance, use subtle animations or messages to indicate that an action is in process and confirm when it’s completed successfully or if it fails.
- Consistency in UI: Ensure that the optimistic updates maintain consistency in the application’s look and feel. Inconsistencies can confuse users and detract from the perceived performance improvements.
By focusing on these areas, developers can maximize the positive impact of the useOptimistic hook on the user experience, leading to applications that are not only functional and fast but also intuitive and robust. Implementing these tools and techniques requires careful planning and thoughtful design but ultimately results in a significantly improved user interface and overall product quality.
Practical Examples of Implemention
Understanding the application of useOptimistic can fundamentally transform the way we approach state management in React applications. Here, we’ll explore this through a real-life example and share effective tips for its integration.
Case study: Real-life application of useOptimistic
Consider a social media platform, where user interactions such as liking a post need to be handled swiftly to ensure a responsive user experience. In such a scenario, the useOptimistic hook can be crucial.
Initially, a user clicks the “like” button on a post. With traditional state update mechanisms, the click would send a request to the server, and only upon receiving a successful response, the UI would update to reflect the change. This can result in a noticeable delay, especially under network latency.
Implementing the useOptimistic hook changes this flow. When a user clicks the “like” button, useOptimistic immediately updates the UI to show that the post has been liked, even before sending the request to the server. Here’s a simplified code snippet to illustrate this:
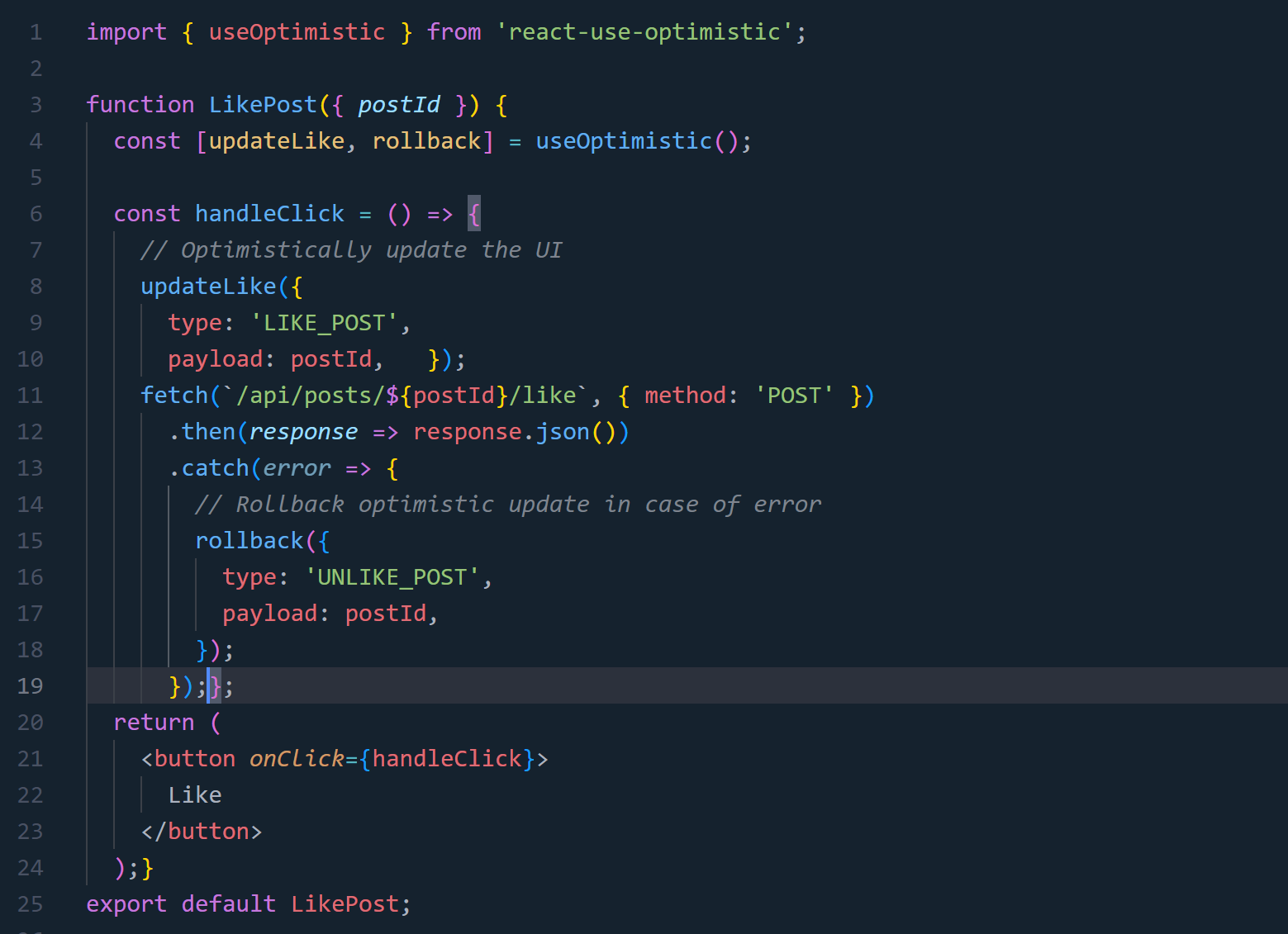
In the example, the useOptimistic hook allows the application to handle state optimistically, creating a smoother and faster user experience. Should the server return an error, the hook also supports reverting the optimistic update, ensuring our data’s integrity.
This approach not only enhances the perceived performance but also helps in maintaining consistent states between the server and client, a crucial aspect in dynamic applications like social media platforms.
Tips for successful integration
Integrating requires understanding its strengths and limitations. Here are some invaluable tips for getting the most out of the useOptimistic hook:
- Understand Your Use Case: Before implementing useOptimistic, ensure it fits your specific scenario. It’s most beneficial in situations where immediate feedback is crucial for user experience but less impactful where users expect some delay, such as in loading data from a server.
- Design for Reversion: Always plan for the possibility of reverting your optimistic updates. Implement error handling that can reliably roll back changes on UI without complicating the user experience.
- Optimize Network Calls: Although useOptimistic improves the responsiveness of an application by updating UI immediately, it doesn’t reduce the network calls to the server. Efficiently manage and minimize these calls to ensure that your application’s performance doesn’t degrade.
- Testing is Key: Rigorous testing is necessary to ensure that the optimistic updates and their potential reversions work correctly under all scenarios. Include tests that simulate network failures and slow network conditions to fully evaluate your implementation.
- Communicate with Users: Sometimes, even with the best implementation, things can go wrong. Ensure to communicate clearly with your users when a problem occurs. For instance, a simple message like “Failed to update, please try again” can prevent confusion and ensure a better user experience.
- Monitor and Iterate: Post-implementation, continually monitor the feature’s performance and user feedback. This data is invaluable for iterating and refining the implementation to better serve your users’ needs.
By following these tips, developers can leverage the useOptimistic hook effectively, making their React applications more robust and user-friendly. Whether you’re building a new project or seeking to improve an existing one, This Hooks offers a powerful tool for enhancing frontend state management, making it an excellent choice for modern web development.
Conclusion
The useOptimistic
hook presents a powerful solution for managing state in React applications with a focus on enhancing user experience through immediate feedback. By intelligently handling optimistic updates, developers can design more responsive and dynamic interfaces. To fully leverage this tool:
- Understand the scenarios best suited for optimistic UI updates.
- Thoroughly manage error states to revert changes when necessary.
- Continuously test to ensure reliability and user satisfaction.
Adopting useOptimistic
into your React project isn’t just about improving performance metrics; it’s about creating seamless, efficient, and highly interactive user experiences. Embrace this nuanced approach to state management and watch as your application not only performs better but also delivers a superior user experience that is noticeable and appreciable.