Table of Contents
Introduction
In the ever-evolving world of web development, JavaScript has remained a cornerstone technology. However, as projects grow in complexity, so does the tendency to rely heavily on external libraries and frameworks. This guide introduces you to the concept of zero dependency JavaScript – a approach that emphasizes lightweight, efficient, and self-contained code.
Zero dependency JavaScript refers to writing JavaScript applications or modules without relying on external libraries or frameworks. This approach focuses on leveraging the core features of the language and browser APIs to create efficient, lightweight, and maintainable code.
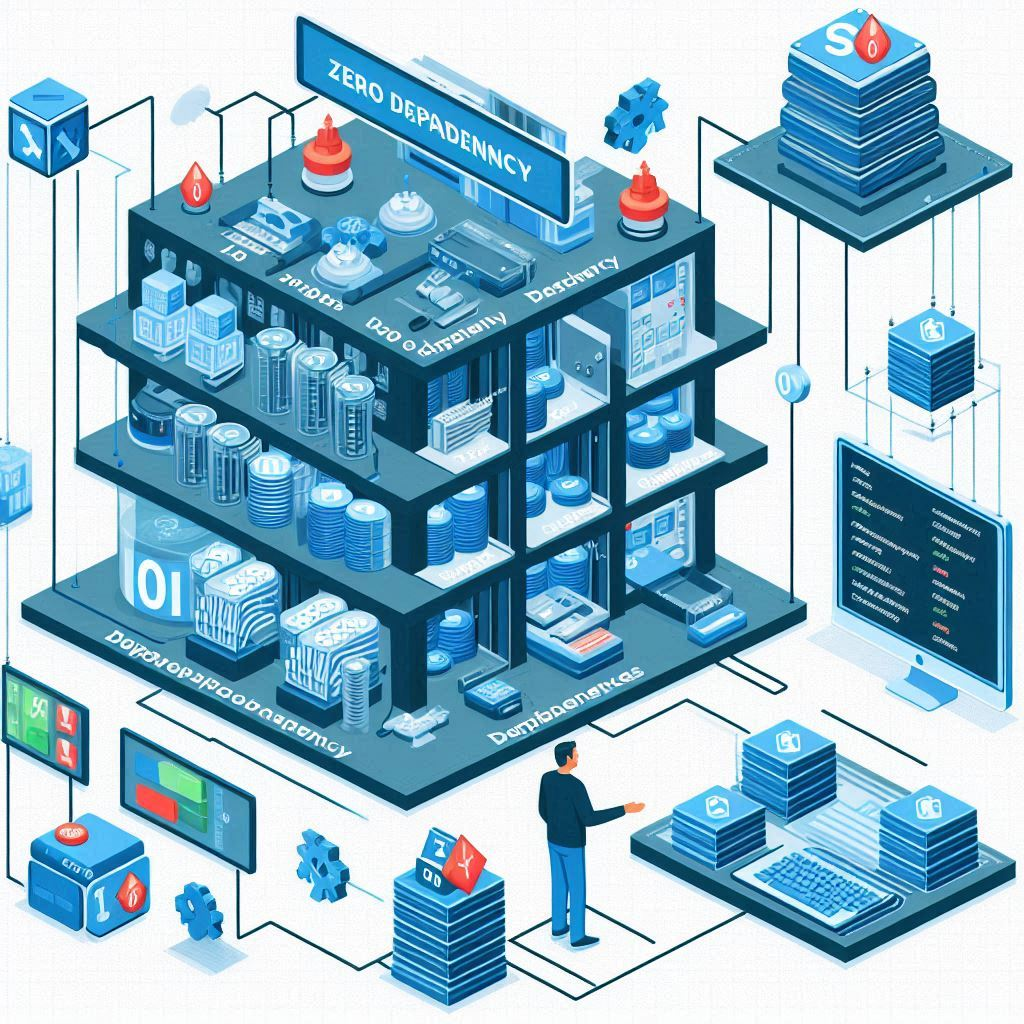
Why Zero Dependency JavaScript Matters
- Performance: Smaller file sizes lead to faster load times.
- Control: You have complete understanding and control over your codebase.
- Security: Fewer dependencies mean a reduced attack surface.
- Learning: It encourages a deeper understanding of JavaScript fundamentals.
This guide is designed for intermediate to advanced JavaScript developers who want to optimize their code, reduce reliance on external libraries, and gain a deeper understanding of the language. Whether you’re building small utilities or considering a lightweight approach for larger projects, this guide will provide valuable insights and practical techniques.
Chapter 1: Understanding Zero Dependency JavaScript
Definition and Principles
Zero dependency JavaScript is an approach to writing JavaScript code that relies solely on the language’s built-in features and APIs, without using external libraries or frameworks. The core principles include:
- Utilizing native JavaScript methods and APIs
- Writing modular and reusable code
- Optimizing for performance and file size
- Enhancing security by reducing external dependencies
Comparison with Frameworks and Libraries
While frameworks and libraries offer convenience and rapid development, zero dependency JavaScript provides:
- Greater control: You have full understanding of every line of code.
- Improved performance: No overhead from unused library features.
- Flexibility: Easier to adapt and modify as project requirements change.
However, it’s important to note that frameworks and libraries still have their place, especially in large-scale applications or when rapid development is prioritized over file size and performance optimizations.
Common Misconceptions
- “Zero dependency means no third-party code at all.”
- Reality: It’s about avoiding runtime dependencies, not development tools.
- “It’s only suitable for small projects.”
- Reality: With proper architecture, it can scale to larger applications.
- “It always results in more code written.”
- Reality: While true for some features, many tasks require less code than using a bloated library.
Chapter 2: Benefits of Zero Dependency JavaScript
Performance Improvements
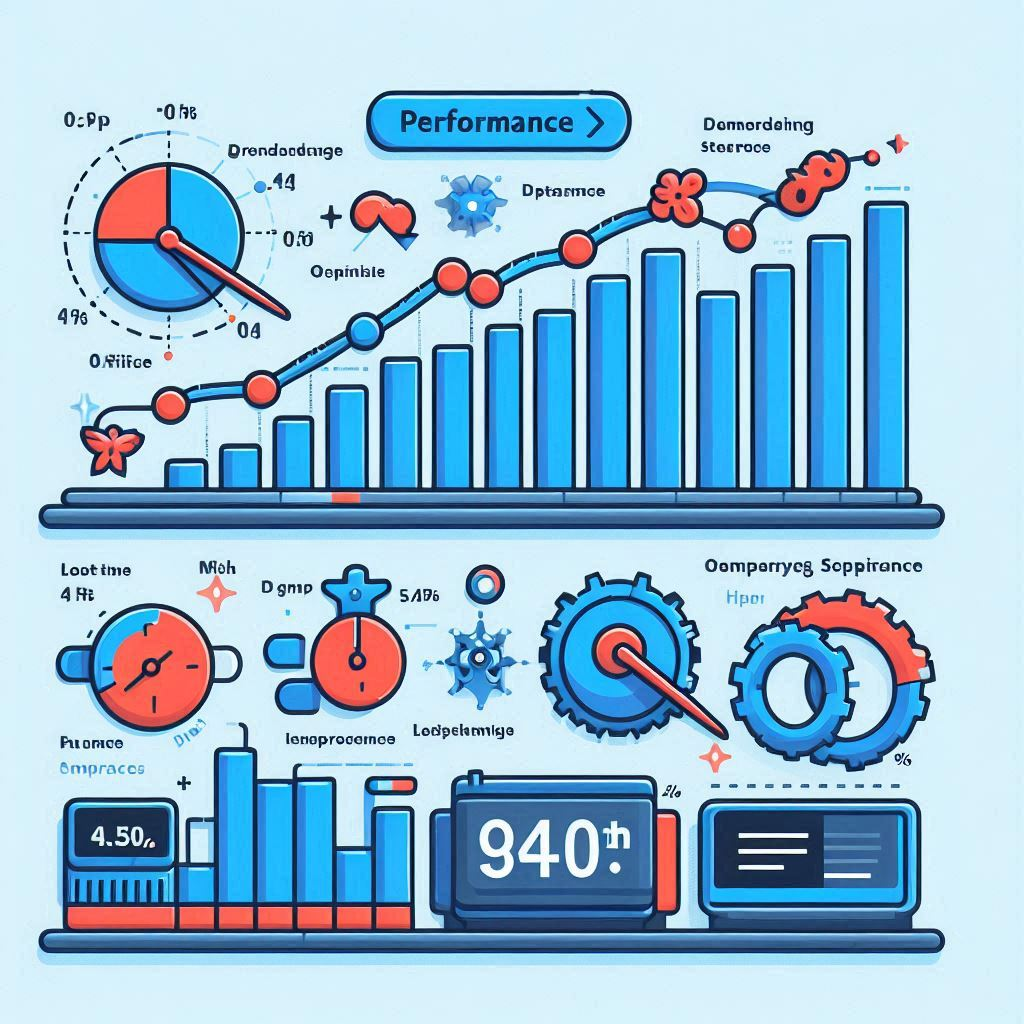
Zero dependency JavaScript often leads to significant performance gains:
- Faster load times: Smaller JavaScript files load and parse quicker.
- Reduced memory usage: Only the necessary code is loaded into memory.
- Improved execution speed: Custom-tailored code can outperform generic library solutions.
Smaller File Sizes
By eliminating unnecessary code, zero dependency JavaScript results in smaller file sizes:
- Reduced bandwidth usage: Beneficial for users with limited data plans.
- Faster downloads: Especially important for users with slower internet connections.
- Improved caching: Smaller files are more cache-friendly, leading to even faster subsequent loads.
Enhanced Security
Reducing dependencies enhances security in several ways:
- Smaller attack surface: Fewer lines of code mean fewer potential vulnerabilities.
- Control over updates: You decide when and how to update your code.
- Easier code audits: A smaller, self-contained codebase is easier to review for security issues.
Easier Debugging and Maintenance
Zero dependency JavaScript often leads to more maintainable code:
- Clear dependency chain: No “black box” external libraries to debug through.
- Simplified updates: No need to manage compatibility with external library versions.
- Better understanding: Developers are more likely to understand code they’ve written themselves.
Chapter 3: When to Use Zero Dependency JavaScript
Appropriate Use Cases
Zero dependency JavaScript is particularly well-suited for:
- Small to medium-sized projects: Where the overhead of a framework isn’t justified.
- Performance-critical applications: When every millisecond counts.
- Educational purposes: To gain a deeper understanding of JavaScript.
- Embedded systems or IoT devices: Where resources are limited.
- Browser extensions: Where file size directly impacts user experience.
Examples of Real-World Applications
- Lightweight analytics tools: Custom-built to track specific metrics without bloat.
- Interactive data visualizations: Using the Canvas API for efficient rendering.
- Custom form validation: Tailored to specific requirements without excess code.
- Simple content management systems: Built with vanilla JavaScript and server-side APIs.
Scenarios Where Dependencies Might Still Be Beneficial
While zero dependency JavaScript has many advantages, there are scenarios where using dependencies might be more appropriate:
- Large-scale applications: Where a consistent architecture (like React or Angular) benefits team collaboration.
- Complex UI components: Where battle-tested libraries can save significant development time.
- Specialized functionalities: Like complex date manipulations or cryptography, where well-maintained libraries are more reliable and efficient.
Chapter 4: Building Blocks of Zero Dependency JavaScript
Core JavaScript Features and APIs
To work effectively with zero dependency JavaScript, it’s crucial to have a strong grasp of core language features and browser APIs:
- DOM manipulation:
document.querySelector
,createElement
, etc. - Event handling:
addEventListener
,removeEventListener
- Fetch API: For making HTTP requests
- Web Storage API:
localStorage
andsessionStorage
- Canvas API: For 2D and 3D graphics
- Web Workers: For background processing
Polyfills and Shims for Cross-Browser Compatibility
While modern browsers support most features natively, you may need polyfills for older browsers:
if (!Array.prototype.includes) {
Array.prototype.includes = function(searchElement, fromIndex) {
// Polyfill implementation
};
}
Working with ES6+ Features Without Dependencies
Modern JavaScript (ES6+) provides powerful features that reduce the need for external libraries:
- Modules: For code organization and encapsulation
- Arrow functions: For concise function syntax
- Template literals: For easy string interpolation
- Destructuring: For extracting values from objects and arrays
- Promises and async/await: For handling asynchronous operations
Chapter 5: Practical Examples
Creating a Simple DOM Manipulation Library
Here’s a basic example of a lightweight DOM manipulation library:
const $ = (selector) => document.querySelector(selector);
const $$ = (selector) => document.querySelectorAll(selector);
const on = (element, event, handler) => {
element.addEventListener(event, handler);
};
const off = (element, event, handler) => {
element.removeEventListener(event, handler);
};
const addClass = (element, className) => {
element.classList.add(className);
};
const removeClass = (element, className) => {
element.classList.remove(className);
};
Building a Custom Event System
A simple pub/sub (publish/subscribe) event system:
class EventEmitter {
constructor() {
this.events = {};
}
on(eventName, callback) {
if (!this.events[eventName]) {
this.events[eventName] = [];
}
this.events[eventName].push(callback);
}
emit(eventName, data) {
const event = this.events[eventName];
if (event) {
event.forEach(callback => {
callback.call(null, data);
});
}
}
off(eventName, callback) {
const event = this.events[eventName];
if (event) {
this.events[eventName] = event.filter(cb => cb !== callback);
}
}
}
Implementing a Basic AJAX Request Handler
A simple AJAX wrapper using the Fetch API:
const ajax = {
get: (url) => fetch(url).then(response => response.json()),
post: (url, data) => fetch(url, {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(data)
}).then(response => response.json())
};
Developing a Small-Scale Templating Engine
A basic templating engine for rendering dynamic content:
function template(str, data) {
return str.replace(/\${(\w+)}/g, (match, key) => {
return data[key] || match;
});
}
// Usage
const tmpl = 'Hello, ${name}!';
const result = template(tmpl, { name: 'World' });
console.log(result); // Outputs: Hello, World!
Chapter 6: Tools and Techniques
Code Organization and Modular Design
Effective code organization is crucial for maintainable zero dependency JavaScript:
- Use ES6 modules: Separate code into logical modules.
- Follow SOLID principles: Write modular, extensible code.
- Implement design patterns: Use appropriate patterns like Singleton, Observer, or Factory.
Performance Optimization Techniques
- Minimize DOM manipulation: Batch updates and use DocumentFragment.
- Use event delegation: Attach event listeners to parent elements.
- Implement lazy loading: Load resources as needed.
- Optimize loops: Use appropriate array methods (map, reduce, filter).
Testing and Debugging Strategies
- Unit testing: Use lightweight testing frameworks like Jest or Mocha.
- Browser developer tools: Leverage console, network tab, and performance profiler.
- Error handling: Implement proper error catching and logging.
Best Practices for Writing Clean and Maintainable Code
- Follow a consistent style guide: Use tools like ESLint to enforce coding standards.
- Write self-documenting code: Use clear variable and function names.
- Comment complex logic: Explain the “why” rather than the “what”.
- Keep functions small and focused: Each function should do one thing well.
Chapter 7: Advanced Concepts
Understanding the Event Loop
The event loop is fundamental to JavaScript’s concurrency model:
- Call stack: Executes synchronous code.
- Task queue: Holds callbacks from asynchronous operations.
- Microtask queue: Handles Promises and certain APIs.
Understanding this helps in writing efficient asynchronous code without external libraries.
Memory Management in JavaScript
JavaScript uses automatic garbage collection, but understanding memory management is crucial:
- Avoid memory leaks: Be cautious with closures and event listeners.
- Use WeakMap and WeakSet: For objects that should be garbage collected.
- Optimize object creation: Use object pools for frequently created/destroyed objects.
Asynchronous Programming with Promises and Async/Await
Promises and async/await provide powerful tools for handling asynchronous operations:
async function fetchData(url) {
try {
const response = await fetch(url);
const data = await response.json();
return data;
} catch (error) {
console.error('Error fetching data:', error);
}
}
Handling Large Datasets Efficiently
When working with large datasets without external libraries:
- Use array methods efficiently: Choose the right method (map, filter, reduce) for the task.
- Implement pagination: Load and process data in smaller chunks.
- Consider Web Workers: Offload heavy computations to background threads.
- Use efficient data structures: Choose appropriate data structures like Map or Set for faster lookups.
FAQ Section
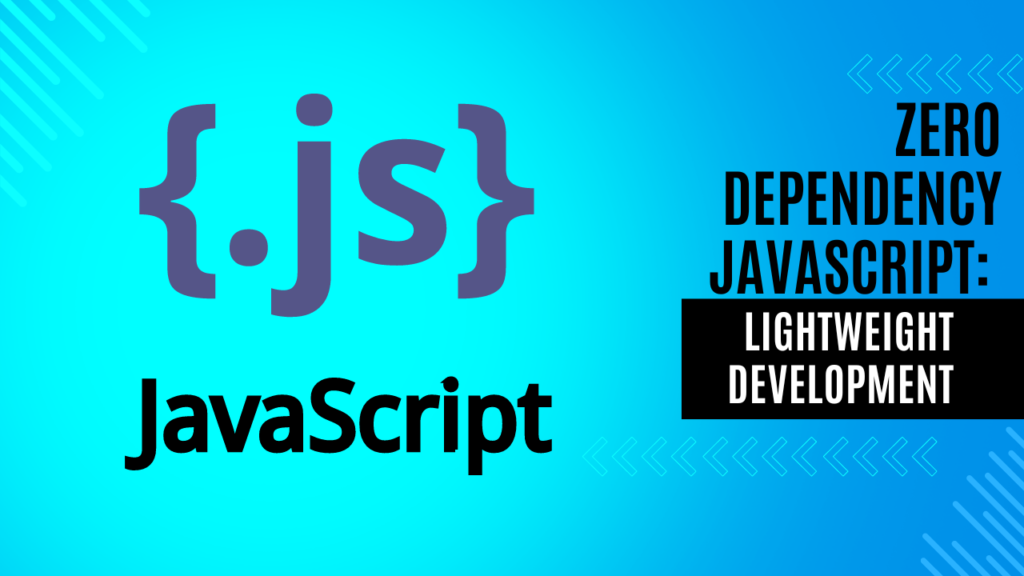
What is zero dependency JavaScript?
Zero dependency JavaScript is an approach to writing JavaScript applications or modules without relying on external libraries or frameworks. It focuses on using the core features of the language and browser APIs to create efficient, lightweight, and self-contained code.
Why should I consider using zero dependency JavaScript?
Consider zero dependency JavaScript for:
- Improved performance and smaller file sizes
- Enhanced security through a reduced attack surface
- Greater control and understanding of your codebase
- Easier debugging and maintenance
What are the main benefits of zero dependency JavaScript?
The main benefits include:
- Faster load times and improved performance
- Smaller file sizes, reducing bandwidth usage
- Enhanced security with a smaller attack surface
- Easier debugging and maintenance
- Deeper understanding of JavaScript fundamentals
Are there any downsides to using zero dependency JavaScript?
Potential downsides include:
- Increased development time for complex features
- Lack of community support compared to popular libraries
- Potential for reinventing the wheel
- May require more thorough testing and edge case handling
How can I start learning zero dependency JavaScript?
To start learning:
- Master core JavaScript features and APIs
- Practice reimplementing common library functions
- Study well-written open-source projects that use minimal dependencies
- Challenge yourself to solve problems without reaching for libraries
What tools can help in developing zero dependency JavaScript applications?
Helpful tools include:
- ESLint for code quality and style enforcement
- Babel for transpiling modern JavaScript features
- Webpack or Rollup for bundling modules
- Jest or Mocha for unit testing
- Browser developer tools for debugging and performance profiling
Can zero dependency JavaScript be used in large-scale applications?
Yes, with proper architecture and design patterns, zero dependency JavaScript can be used in large-scale applications. However, it may require more planning and custom code compared to using established frameworks.
How does zero dependency JavaScript impact performance?
Zero dependency JavaScript often leads to improved performance through:
- Smaller file sizes, resulting in faster load times
- Reduced memory usage
- Elimination of unused code from large libraries
- Potential for more optimized, purpose-built solutions
What are some common use cases for zero dependency JavaScript?
Common use cases include:
- Small to medium-sized web applications
- Browser extensions
- Performance-critical components
- Educational projects for learning JavaScript fundamentals
- Lightweight utilities and tools
Is it possible to convert existing projects to zero dependency JavaScript?
Yes, it’s possible to gradually convert existing projects by:
- Identifying and removing unused dependencies
- Replacing library functions with custom implementations
- Refactoring code to use native JavaScript features
- Carefully testing each change to ensure functionality is preserved
Conclusion
Mastering zero dependency JavaScript opens up a world of lightweight, efficient, and maintainable development. By leveraging the core features of JavaScript and browser APIs, developers can create powerful applications without the overhead of external libraries.
Key takeaways from this guide:
- Zero dependency JavaScript promotes performance, security, and deeper language understanding.
- It’s suitable for various projects, from small utilities to carefully designed larger applications.
- Success with this approach requires strong knowledge of JavaScript fundamentals and browser APIs.
- Proper code organization, testing, and performance optimization are crucial.
- While powerful, it’s important to recognize when external libraries might still be beneficial.
As you continue your journey in JavaScript development, we encourage you to experiment with zero dependency approaches. Start small, perhaps by reimplementing a simple utility function, and gradually tackle more complex challenges. Remember, the goal is not to avoid all dependencies at all costs, but to make informed decisions that lead to efficient, maintainable, and performant code.
Resources for Further Learning
To deepen your understanding of zero dependency JavaScript, consider exploring:
- MDN Web Docs for comprehensive JavaScript and Web API references
- Open source projects that emphasize minimal dependencies
- JavaScript specification (ECMAScript) to understand language features in depth
- Performance optimization guides for web applications
- Advanced JavaScript books focusing on language internals and patterns
By mastering zero dependency JavaScript, you’ll not only write more efficient code but also gain a deeper appreciation for the power and flexibility of the language itself. Happy coding!