Welcome to your step-by-step guide on setting up a phenomenal MERN stack development environment! If you’re gearing up to develop stunning web applications, you’re in the right place. The MERN stack combines MongoDB, Express.js, React, and Node.js—four powerful tools that allow developers to craft dynamic and responsive web applications efficiently. Whether you’re a beginner or a seasoned developer, this guide will walk you through all the necessary steps to get your MERN stack up and running. Let’s dive into the world of full-stack development and turn those ideas into reality!
Installing and Configuring MongoDB
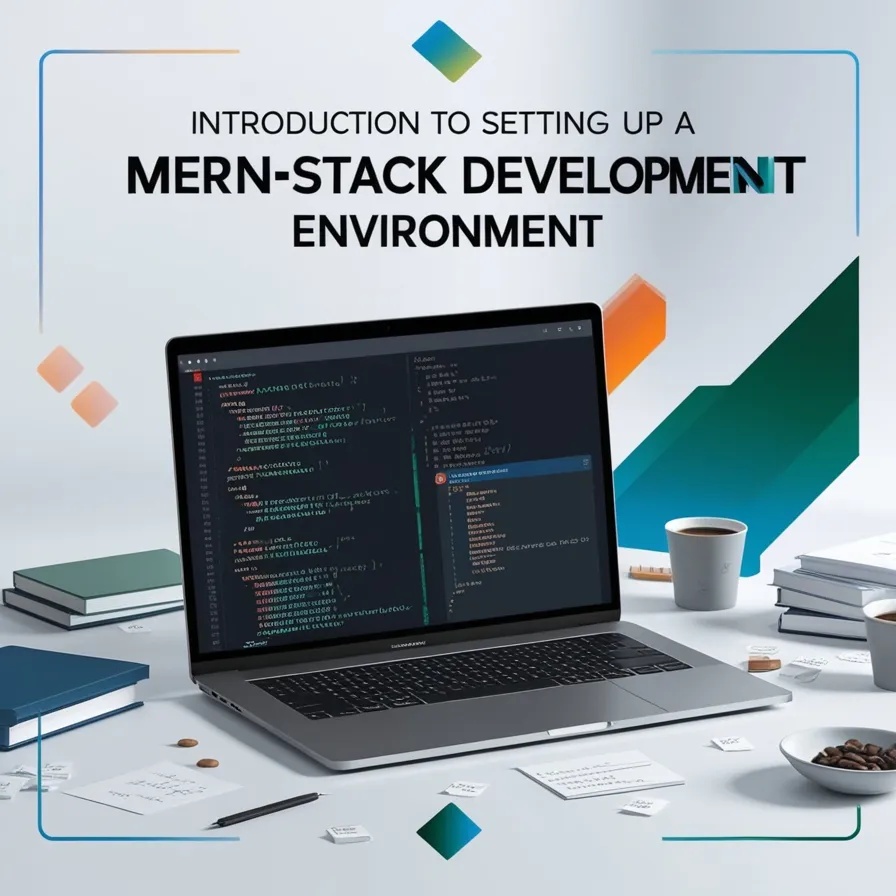
MongoDB is a dynamic, NoSQL database that provides developers with rich features, flexibility, and an excellent scalability factor that fits perfectly with the ever-evolving needs of modern web applications. Despite its complexity, it remains user-friendly for developers, making it a popular choice for storing data in JSON-like documents that can vary in structure. MongoDB is adept at handling large volumes of diverse data, and it integrates seamlessly into our MERN stack, enhancing its power in handling vast, schematically unstructured data clusters.
Step-by-step guide to installing MongoDB
Setting up MongoDB might sound daunting, but it’s easier than you think! Here’s how you can do it:
1. Download MongoDB: Go to the MongoDB official website and download the correct version of MongoDB for your operating system.
2. Install MongoDB: Run the installer and follow the setup wizard. The installer includes MongoDB Compass, which is a handy GUI for MongoDB, though optional.
3. Set up the environment: After installation, set MongoDB’s bin directory to the system’s PATH environment variable. This step is crucial as it allows you to run MongoDB from any command line interface.
4. Running MongoDB: Open your command line interface, type mongodb to start the MongoDB server, and open another command line window and type mongo to start interacting with your MongoDB databases.
5. Create your database: Use the command use yourNewDatabase, to create a new database. MongoDB won’t create the database until data is stored.
And just like that, MongoDB is up and running, ready to store the data you’ll manage from your MERN stack applications!
Configuring Express.js
Express.js, often referred to simply as Express, shoulders the responsibility of handling server-side logic in the MERN stack—bridging the gap between the frontend and the database with smooth, streamlined server-side programming. It’s designed to build web applications efficiently and comes packed with features that make routing, handling HTTP requests, and middleware integration a breeze. Understanding Express.js is crucial because it directly impacts application performance and efficiency.
Setting up Express.js in the MERN stack environment
Integrating Express.js into our MERN environment involves a few precise steps:
- Install Node.js: Ensure you have Node.js installed, as it is necessary for running Express.
- Initiate a Node.js project: Create a new directory for your project and initialize it with \`npm init\`. This process creates a \`package.json\` file that will manage all your project’s dependencies.
- Install Express: Within the project directory, run \`npm install express\` to add Express to your project.
- Set Up the Server: Create an \`index.js\` file, and import express with \`const express = require(‘express’);\`. Initialize your application with \`const app = express();\`.
- Start the Server: Define the port for your server
const PORT = process.env.PORT || 5000;
and then listen to this port withapp.listen(PORT, () => console.log('Server running on port ${PORT}'));
.
Once these steps are followed, your Express.js framework will be configured and ready to serve the incoming requests, making it a robust backend point for your MERN applications.
Exploring middleware in Express.js
In the world of Express.js, middleware functions are essentially the backbone—they have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle. These functions can execute any code, make changes to the request and the response objects, end the request-response cycle, and call the next middleware function. Examples of middleware include functions to log request details, authenticate user requests, and handle errors. Implementing middleware can greatly enhance your app’s performance and security. Here’s how you can apply a simple middleware function:
app.use((req, res, next) => {
console.log('Request URL:', req.originalUrl);
next();
});
This function logs the URL of every request made to the server, then smoothly passes control to the next middleware function in the stack.
Setting up React
React is a powerful front-end library developed by Facebook, designed for building user interfaces, especially for single-page applications where you need data change without reloading the page. In the MERN stack, React plays a critical role in defining the structure and behavior of the front-end. It enhances the user experience by ensuring the UI is highly responsive and dynamic. React’s central feature is its component-based architecture, which allows developers to build encapsulated components that manage their own state, then compose them to make complex UIs. By using React in the MERN stack, developers can create interactive and scalable web applications efficiently.
Installing and configuring React for the development environment
To start using React in your MERN stack development, you first need to set up the environment. Begin with the installation of Node.js and npm (Node Package Manager), which are essential for running and managing React applications. Once Node.js and npm are installed, you can create a new React application using the Create React App command-line interface. Here’s how to do it:
1. Open your terminal.
2. Run npx create-react-app your-project-name
to create a new React project. This command sets up everything you need for a React application, including a development server, webpack configuration, and Babel setups.
3. Navigate into your project directory using cd your-project-place
.
4. Execute \`npm start\` to run the React application. A new browser window should automatically open displaying your new React app.
At this point, your basic React setup is complete, and you can begin to configure additional tools like linters, routers, or state management libraries according to your project requirements.
Utilizing React components for efficient development
React’s component-based architecture is not just a methodology; it’s a practical workflow that enables developers to create reusable and independent pieces of UI. Each component manages its own state and can be nested within other components to build complex applications out of simple building blocks. This structure promotes a solid development approach, making code easier to debug and maintain. Moreover, React’s virtual DOM (Document Object Model) optimizes updates to the actual DOM, which greatly improves performance.
To maximize efficiency, start by breaking down the application UI into smaller components like headers, footers, lists, or modals. Develop these components individually and integrate them into larger views. Utilize React hooks, such as useState and useEffect, for adding state and lifecycle methods to function components. This modularization not only speeds up the development process but also enhances scalability and maintainability of your application.
Installing Node.js
Node.js is an open-source, cross-platform JavaScript runtime environment that allows you to execute JavaScript code outside a web browser. It’s essential for the MERN stack as it runs the server-side JavaScript and hosts the Express.js server. Node.js is built on Chrome’s JavaScript runtime, which helps in building scalable network applications. Node.js uses an event-driven, non-blocking I/O model that makes it lightweight and efficient, perfect for data-intensive real-time applications that run across distributed devices.
Installing Node.js and npm
Installing Node.js and npm is straightforward and crucial for setting up your MERN stack development environment. Here’s how to get started:
- Visit the official Node.js website (nodejs.org).
2. Download the appropriate version for your operating system. Typically, the LTS (Long Term Support) version is recommended for most users.
3. Run the installer and follow the instructions to install both Node.js and npm. Npm is included by default with the Node.js installation.
4. After installation, launch a terminal and enter node -v followed by npm -v to confirm that both Node.js and npm have been successfully installed on your system.
With Node.js and npm installed, you’re now ready to set up the back-end part of your MERN applications or any server-side logic.
Exploring Node.js modules for MERN stack development
Node.js’s ecosystem is famously enriched with a plethora of modules available through npm, which can help in various aspects of Mern stack development. Some of the key modules include:
- Express.js: A minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications.
- Mongoose: An elegant mongodb object modeling for Node.js.
- Body-parser: A body parsing middleware that is extremely useful in handling JSON requests.
- Nodemon: A utility that monitors for any changes in your source and automatically restarts your server.
By leveraging these and other modules, developers can enhance the functionality, manageability, and security of their MERN stack applications, resulting in robust and high-performing web applications.
Integrating MongoDB, Express.js, React, and Node.js
It’s time to weave together the components of the MERN stack: MongoDB, Express.js, React, and Node.js. To ensure a seamless integration, start by connecting your backend Express.js server with MongoDB. Using the mongoose library, easily link your database by updating your connection strings in the main server file.
Next, let’s integrate React with your Node.js/Express.js backend. Create a client folder in your main project directory where you’ll initiate a new React application using the command `npx create-react-app client
`. After setup, modify your client-side `package.json` to include a proxy that directs API requests to your Node.js server, typically as `”proxy”: “http://localhost:5000″`. This step ensures that your React app can interact smoothly with the Express.js server, fetching data effortlessly from the backend.
Make sure to test the connection between all elements. Add a simple API call from the React front-end to verify that data is correctly fetched from MongoDB through the Express.js layer, confirming a successful integration of all stack components.
Testing the MERN stack setup
Testing is a critical phase to ensure your MERN stack setup is perfectly configured. Start by writing simple functional tests for your API endpoints using tools like Mocha and Chai. Ensure each test checks the endpoints for correct responses and error handling capabilities.
On the front end, utilize libraries like Jest alongside React Testing Library to conduct unit and integration tests. Verify that your user interface renders correctly and interacts seamlessly with your back-end services. Do not forget to test the entire flow of data from the front end to the database, checking for any discrepancies or failures.
Lastly, perform end-to-end testing to simulate user behaviors on the client side and observe how the system processes these actions through to the database. This can be done using Cypress or Selenium, providing a thorough validation of your complete MERN stack functionality.
Troubleshooting common setup issues
Even the best setups can encounter bumps along the way, so here’s how to troubleshoot common issues in the MERN stack development:
- Problem connecting to MongoDB: Verify that your MongoDB URI is correct and that your MongoDB server is running. Check firewall settings and authentication details if you’re using MongoDB Atlas.
- API requests returning errors: Ensure that your Express.js routes are correctly defined and match the API calls made from React. Cross-origin resource sharing (CORS) errors can also occur if the backend and frontend servers are on different ports; resolve this by configuring CORS in your Express app.
- React not updating with data from backend: Make sure the proxy setup in your client’s `package.json` is correct, and clear the browser cache or check if the state management in React correctly handles the API data.
Conclusion and Next Steps
Congratulations on setting up your MERN stack! You’ve traveled through the intricacies of configuring databases, servers, and frontend frameworks, integrating complex software, and testing to ensure everything clicks perfectly. Now, armed with a full-stack JavaScript environment, you’re set to build dynamic web applications.
As next steps, consider diving deeper into each component of the MERN stack. Explore advanced MongoDB features, experiment with different React libraries for state management like Redux or Context API, and enhance your Express.js server with additional middleware for better performance. Additionally, familiarize yourself with deployment processes using platforms like Heroku or AWS to get your applications live and running smoothly on the web.
Keep experimenting, keep learning, and most importantly, keep building amazing web experiences with your new MERN stack skills!