Node.js has emerged as a powerful tool for building a variety of applications, from web servers to networking tools to real-time applications. With its easy scalability and high performance, it leverages JavaScript’s ubiquity and the vast npm ecosystem, making it an indispensable skill in the modern developer toolkit. For beginners aiming to enter the world of software development, understanding Node.js is both rewarding and essential. This guide provides a strategic syllabus tailored for beginners to efficiently start their journey in learning Node.js.
Understanding Node.js
What is Node.js and syllabus for beginners?
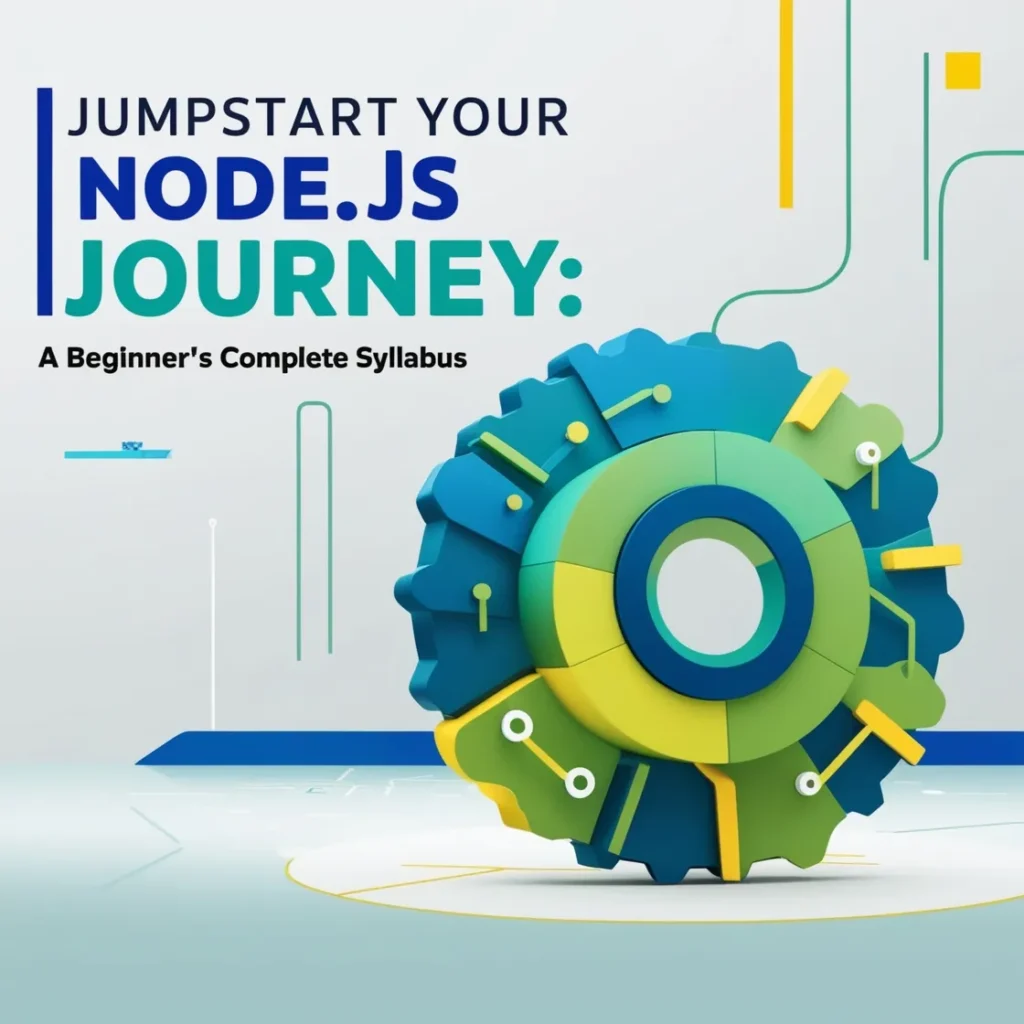
Node.js is an open-source, cross-platform, back-end JavaScript runtime environment that runs on the V8 engine and executes JavaScript code outside a web browser. Essentially, it allows developers to use JavaScript to write command-line tools and for server-side scripting—running scripts server-side to produce dynamic web page content before the page is sent to the user’s web browser. Therefore, Node.js represents a “JavaScript everywhere” paradigm, unifying web application development around a single programming language rather than different languages for server-side and client-side scripts.
Why Node.js is so popular among beginners
The popularity of Node.js among beginners can largely be attributed to several key factors:
- Simplicity: JavaScript is one of the most straightforward languages for newbie programmers to learn. Node.js extends this by enabling server-side coding in the same language.
- Efficiency: Built on the Google V8 JavaScript engine, Node.js is extremely efficient and well-suited for handling data-intensive real-time applications that run across distributed devices.
- Community and Support: With one of the largest ecosystems of open-source libraries available through the npm registry, beginners have extensive resources to solve problems and enhance their learning.
- Versatility: Node.js is incredibly versatile, perfect for prototyping and agile development as well as building super-fast and scalable applications like online games, chatbots, and more.
- Node.js’s non-blocking, event-driven architecture optimizes throughput and scalability in web applications with many input/output operations, making it a smart choice for beginners looking to make a significant impact in their coding careers.
Setting up Environment
Installing Node.js
To begin your journey with Node.js, the first step is to install the Node.js environment on your computer. It is available for download on the official Node.js website and is compatible with most operating systems, including Windows, macOS, and Linux. Make sure to download the LTS (Long Term Support) version, as it’s more stable and well-supported for beginners. After downloading, run the installer and follow the prompts to install Node.js and npm (Node package manager), which is vital for managing your Node.js applications.
Choosing a text
Selecting a text editor is crucial, as it will be your primary tool for writing code. There are several popular text editors that are well-suited for Node.js development. Visual Studio Code (VS Code) is highly recommended due to its built-in support for JavaScript and a vast ecosystem of extensions that simplify Node.js development. Other good options include Sublime Text and Atom, both of which offer a clean, user-friendly interface and robust functionality tailored for beginners. Whichever editor you choose, ensure it supports JavaScript syntax highlighting and can integrate with Node.js for a smoother workflow.
Basic Concepts
Asynchronous Programming
Asynchronous programming is a core concept in Node.js, allowing it to perform non-blocking operations. Unlike traditional synchronous programming, where tasks are executed sequentially, asynchronous programming executes tasks concurrently, making Node.js highly efficient for I/O-heavy operations. Understanding callbacks, promises, and async/await patterns is fundamental in mastering Node.js. These constructs help manage asynchronous operations and ensure your apps remain responsive and performant.
Event Loop
The event loop is what allows Node.js to perform non-blocking I/O operations, despite the fact that JavaScript is single-threaded. It is at the heart of Node.js architecture. Understanding the event loop involves recognizing how tasks are queued and processed in Node.js. The event loop continuously checks the queue of events and processes them asynchronously, handling connections and I/O requests efficiently. A solid grasp of how the event loop functions is crucial for developing scalable Node.js applications that can handle a large number of simultaneous operations.
Core Modules
Node.js offers a variety of built-in modules that are essential for back-end development. These core modules include functionalities necessary for file system access, network communications, and path utilities, among others. Understanding these modules provides a strong foundation in Node.js capabilities.
fs module
The fs module in Node.js is fundamental for handling file system operations such as read/write files, interact with directories, and stream files. Beginners can start by learning to read files asynchronously using \`fs.readFile()\` and then move on to more complex file operations such as writing to files using \`fs.writeFile()\`. Handling errors and knowing when to use synchronous methods are crucial skills in real-world applications.
http module
The http module is one of the cornerstones of Node.js, allowing developers to build web servers and handle HTTP requests and responses. Starting with creating a simple server using \`http.createServer()\`, beginners can explore how to set headers, status codes, and handle GET and POST requests. This module is vital for developing API services and understanding the basics of web communications.
path module
The path module provides utilities for working with file and directory paths. It is particularly useful for ensuring that applications behave consistently across different operating systems. Beginners should focus on methods like \`path.join()\` and \`path.resolve()\` to manipulate and access file paths safely, which is especially crucial for projects involving file-based operations.
NPM (Node Package Manager)
NPM is an indispensable tool for modern Node.js development which serves as a registry and also aids efficiently in managing dependencies of various Node.js projects.
Introduction to NPM
NPM not only hosts the largest ecosystem of open-source libraries but also provides command-line utility to install Node.js packages, do version management, and manage dependencies. Beginners should understand how to navigate the NPM package library and learn commands like \`npm init\` and \`npm install\`.
Using NPM for package Effective package management is crucial in handling Node.js projects, ensuring they are up-to-date and secure. Beginners should start by learning how to install packages using \`npm install`, and then delve into managing package versions and updating them using \`npm update`. Understanding \`package.json\`, the configuration file for projects that includes metadata about the project, is a key aspect of mastering NPM.
Creating a Simple Node.
Setting up a project
To set up a Node.js project, begin by installing Node.js and npm (node package manager) on your computer. After installation, you can create a new directory for your project and initialize it with a ‘package.json’ file by running \`npm init` from your terminal. This file will manage all the dependencies and scripts related to your project. To maintain a structured and accessible project environment, consider setting up a version control system like Git.
Writing your first Node.js script
Write your first simple Node.js script by creating a new file, typically named ‘app.js’ or ‘index.js’. In this file, you can start with a basic “Hello World” program:
console.log('Hello World');
This program will print ‘Hello Out’ when run in the Node.js environment. You can expand this by importing required modules using \`require\`, defining functions, or managing data.
Running the application
To run your Node.js application, navigate to your project directory in the terminal and type:
node app.js
This command will execute the script you wrote in the ‘app.js’ file. If your script is error-free, you will see whatever output your code is designed to display, such “Hello World” in the initial example.
Handling HTTP Requests
Creating a server
Node.js makes creating a server straightforward using its built-in ‘http’ module. To create a server, include the http module and use its \`createServer\` method:
const http = require('http');
const server = http.createServer((request, response) => {
response.end('Server Working');
});
server.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
This code snippet will start a server listening on port 3000, and it will respond with “Server Working” for every request.
Handling routes
handling routes involves determining the incoming HTTP request’s URL and method, and then serving the appropriate content or data. Use the ‘url’ module to parse the request URL and a simple conditional or switch statement to handle different routes:
const http = require('http');
const url = require('url');
const server = http.createServer((request, response) => {
const parsedUrl = url.parse(request.url, true);
if (parsedUrl.pathname === '/') {
response.end('Home Page');
} else if (parsedUrl.pathname === '/about') {
response.end('About Us');
} else {
response.writeHead(404);
response.end('Not Found');
}
});
server.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
This server will respond to home and about routes by serving text responses and will give a ‘Not Found’ message for all other routes.
Working with Data
Introduction to databases
Understanding how to work with databases is crucial for any backend software, including those built on Node.js. Databases can be broadly categorized into SQL and NoSQL databases. SQL databases, like MySQL and PostgreSQL, organize data into defined tables and relationships, and they use SQL (Structured Query Query Language) for database access. NoSQL databases, such as MongoDB, store data in more flexible formats like JSON and are beneficial for applications that require rapid development and scaling. For beginners, grasping the basic concepts of databases and how they integrate with Node.js is essential for building robust applications.
Using MongoDB with Node.js
MongoDB, a popular NoSQL database, is a great choice for Node.js applications due to its JSON-like storage format and scalability. To use MongoDB with Node.js, you first set up your MongoDB server or use a cloud-based service like MongoDB Atlas. Then, incorporate the Node.js MongoDB driver or a higher-level library like Mongoose in your project. Mongoose abstracts database operations and provides schema validation, which is fundamental for structuring and querying data effectively. By mastering MongoDB usage in Node.js, developers can build dynamic and flexible applications efficiently.
Error Handling
Types of errors in Node.js
Error handling is a critical part of Node.js development. Errors in Node.js can be broadly separated into operational errors and programmer errors. Operational errors are part of the system’s normal operation, like failed network requests or file access rights, while programmer errors are bugs in the code, such as type mismatches or accessing undefined variables. Understanding the types of errors can help in implementing more effective error handling strategies in your applications.
Implementing error help in your applications
Effective error handling in Node.js not only prevents your application from crashing but also ensures it behaves correctly under unforeseen circumstances. A common strategy in Node.js is to use the traditional “try…catch” method, which allows you to catch exceptions synchronously. Additionally, Node.js embraces asynchronous error handling with the “error-first” callback pattern, where callbacks receive an error object as the first argument. For promises, error handling is achieved using the \`.catch()\` method. Implementing these error-handling patterns correctly is key to building resilient Node.js applications.
Testing Node.js Applications
importance of testing
Testing is a crucial phase in the development of any application, including those built with Node.js. It ensures that the code behaves as expected and helps identify bugs before the software is released into production. Proper testing elevates the quality of the application, which translates into higher user satisfaction and reduced maintenance costs over time. For beginners, understanding the ins and outs of testing is beneficial for building robust and scalable Node.js applications.
Tools for testing Node.js applications
Node.js offers several tools that make the testing process efficient and comprehensive. Here are a few popular ones:
- Mocha: A flexible testing framework that supports both behavior-driven development (BDD) and test-driven Driven Development (TDD). Mocha is known for its extensibility and compatibility with other libraries, like Chai for assertions.
- Jest: Created by Facebook, Jest is often praised for its zero-configuration setup and instant feedback. It’s particularly powerful for large-scale applications due to its capabilities for snapshot testing and built-in test coverage tools.
- AVA is : A modern testing framework designed for asynchronous testing, perfect for handling Node.js native promise-based and async/await functionalities. AVA executes tests concurrently, enabling faster testing cycles.
- Supertest: Ideal for testing HTTP APIs, Supertest works well with Node.js to simulate server requests and responses, allowing developers to assert conditions based on HTTP status codes and response payloads.
Deploying Node.js Applications
Deployment options
When it comes to deploying Node.js applications, several options are available:
- Heroku is : A popular Platform as a Service (PaaS) that allows developers to deploy, manage, and scale applications easily. Heroku supports Node.js naturally and simplifies many aspects of application deployment.
- AWS Elastic Beanstalk: For those looking into AWS services, Elastic Beanstalk automatically handles the deployment, from capacity provisioning and load balancing to auto-scaling.
- DigitalOcean: Known for its simplicity and high performance, DigitalOcean offers droplets, which are customizable virtual servers that can host Node.js applications.
- Docker: Using Docker, developers can create containers that package up an application with all its parts, such as libraries and dependencies, and ship it as one package.
Best practices for deployment
Deploying Node.js applications effectively involves more than just selecting a deployment platform. Here are some best practices:
Continuous Integration/Continuous Deployment (CI/CD): Implementing CI/CD pipelines helps automate the process from code commit to production, ensuring that each integration is verified by an automated build.
Environment Variables: Use environment variables to store configuration options and sensitive information. This practice keeps your application’s configuration separate from its codebase and simplifies configuration changes across different environments.
Load Testing: Before going live, conduct load testing to determine how your application behaves under stress. This can help you identify and rectify performance bottlenecks.
Logging and Monitoring: Setup logging and monitoring to track the application’s performance and health post-deployment. Tools like Winston for logging and Prometheus for monitoring can be invaluable for maintaining good service quality.
Conclusion
In conclusion, embarking on learning Node.js as a beginner can seem daunting at first, but with a structured syllabus that includes understanding the basics, mastering essential frameworks, experimenting with databases, exploring APIs, and implementing best practices in security, you can gradually build your competence. Remember, the key to mastering Node.js—or any programming language or technology—is consistent practice and real-world application. Use projects to challenge your skills and solidify your understanding. Additionally, the community around Node.js is vibrant and supportive, offering endless resources, such as tutorials, forums, and open-source projects, which can be incredibly beneficial as you navigate your learning journey. Begin with the basics, stay curious, and keep learning; the world of Node.js awaits you.
Pingback: Navigating the MERN Stack Roadmap: A Step-by-Step Guide