In the bustling world of web development, Angular has emerged as a beloved framework for building dynamic and powerful applications. An exciting aspect of Angular is its ability to handle reactivity using signals. But what exactly does that mean for developers like you and me? In simple terms, Angular signals are like little messengers that help manage changes in your application’s data flow. By embracing signals and reactivity, you can create apps that are not only smart but also incredibly responsive. This approach ensures that your web projects run smoothly, reducing bugs and improving user experience. Let’s dive in and explore how Angular signals and reactivity can elevate your development game!
Understanding Angular Signals
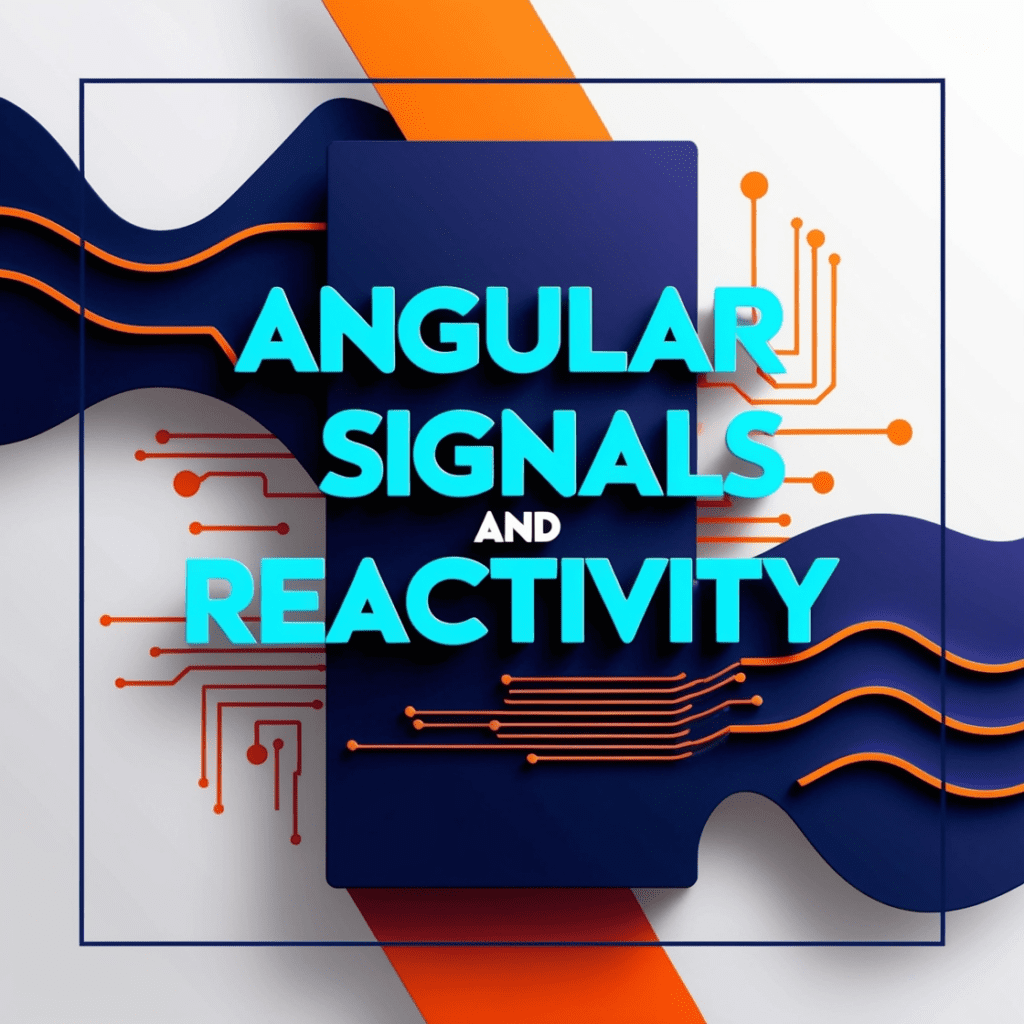
Angular signals are an exciting addition to the toolkit of any Angular developer looking to embrace a more reactive style of programming. But what exactly are these signals, and how do they fit into Angular’s framework?
What are Angular Signals?
Angular signals can be understood as special constructs that help manage data changes and react to these changes proactively. Think of signals as messengers that notify components or services within your Angular application about updates or alterations in the data they depend on. Essentially, a signal represents a piece of state that a system observes for changes. Once a change is detected, related processes are triggered to update their behavior accordingly.
This concept draws inspiration from reactive programming paradigms where the flow of data dictates the application’s behavior. Angular, traditionally imperative in nature, embraces a more reactive approach with signals to better manage the complexity of modern web applications.
How Signals Work in Angular
In technical terms, Angular signals can be likened to observable patterns. They play a pivotal role in creating responsive applications, where each signal is associated with a specific data source or a computed value based on other data sources.
- Definition: Signals are defined through Angular’s framework using special decorators or APIs to declare observable state.
- Subscription: Components or services subscribe to these signals to receive timely updates on changes.
- Listeners: Each signal acts like a broadcaster with various listeners, ensuring the right parts of your application receive the right updates at the right time.
- Propagation: Once a signal changes, an automatic propagation occurs through the system, touching all subscribers dependent on it.
By emphasizing a declarative approach, Angular signals abstract the complexity of tracking state changes manually, smoothing out the development process and reducing potential errors.
Benefits of Using Signals in Angular
Angular signals bring a wealth of advantages to the table, making them an attractive choice for developers aiming to harness the full potential of reactivity.
- Simplified State Management: Signals reduce the need for complex state management systems by allowing a more straightforward way to track and respond to changes.
- Efficient Updates: With reactive updates via signals, Angular applications become more efficient, only re-rendering the necessary components. This optimization can significantly boost performance, especially in large-scale applications.
- Improved Readability: The declarative nature of signals means code is often easier to read and maintain. This readability enhances collaboration among teams and accelerates feature development.
- Minimized Boilerplate Code: Signals inherently reduce the need for additional code, often required for traditional state management, which means a cleaner and more concise codebase.
Moreover, integrating signals into an Angular application allows for a design pattern that closely aligns with how users expect applications to react—fast, seamless, and predictable.
Exploring Reactivity in Angular
Reactivity forms the backbone of modern interactive web experiences, and Angular has enhanced its framework to fully embrace these principles.
Core Concepts of Reactive Programming
Before diving into Angular-specific implementations, let’s unpack some of the core concepts of reactive programming itself:
- Data Streams and Observables: At the heart of reactivity lies the concept of data streams—continuous flows of data or events that can be observed and processed. Reactive systems use observable sequences as a primary abstraction, enabling applications to react to emitted data values over time.
- Bidirectional Data Flow: Unlike the traditional one-way data flow, reactivity supports changes initiated by UI elements that reflect back into the system’s data layer, ensuring an integrated data communication pipeline.
- Composability and Modularity: Reactive programming allows developers to build applications using small, modular units that can be easily composed to achieve complex behaviors.
- Lazy Evaluation: Reactive streams often use lazy evaluation, meaning computations aren’t performed until they are needed. This can lead to more efficient resource usage.
Embracing these principles can transform how developers think about dynamic content and interaction flows within web applications.
Implementing Reactivity in Angular Applications
Implementing reactivity in Angular involves leveraging frameworks and libraries such as RxJS (Reactive Extensions for JavaScript) and Angular’s built-in reactive concepts. Here’s how you can implement reactivity:
- Using Observables: Angular extensively employs observables, a core part of RxJS, to manage asynchronous data streams. Observables can represent events, HTTP requests, or any other data source that you might need to act upon.
- Change Detection: Angular features a powerful change detection mechanism that monitors the model’s state and updates the view accordingly whenever a change is detected. Signals naturally fit into this paradigm by providing a more nuanced update model.
- Reactive Forms: Angular offers reactive forms for form management, which complements reactive programming patterns. With reactive forms, form controls are units of their own, observing and reacting to changes within the form’s data model.
- Async Pipes: Angular’s async pipe simplifies the subscription to observables and automatically handles resource-intensive unsubscribe processes. This pipeline encourages clean and efficient coding practices.
Diving into these tools enables developers to build applications that are not only reactive but efficient and highly responsive to state changes.
Comparing Reactivity with Traditional Programming Models
Compared to traditional programming models, reactive programming represents a paradigm shift that can greatly improve application design and function.
- Stateful vs. Stateless: Traditional programming often relies on explicitly managing the state throughout the application’s lifecycle. In contrast, reactive programming handles state within compositional constructs which automatically manage transitions and lifecycles.
- Pull vs. Push: Traditional programming tends to utilize a pull model where the system queries for needed information. Meanwhile, a reactive push model sends updates immediately when changes occur, reducing latency and ensuring data is always current.
- Synchronous vs. Asynchronous: Many traditional models operate synchronously, which can lead to blocking and inefficiency. Reactive programming, however, is inherently asynchronous, improving responsiveness and resource utilization.
- Complexity and Scalability: Traditional models may struggle when scaling to handle complex asynchronous events or interactions. Reactive programming thrives in such scenarios, offering natural mechanisms to scale efficiently.
By understanding these distinctions and embracing reactivity, developers position themselves to build modern applications that align with contemporary user expectations.
In conclusion, Angular signals and reactivity form a powerful union that empowers developers to craft applications with enhanced performance, maintainability, and user interactivity. By adopting reactive programming techniques, you’ll be well-equipped to navigate the evolving landscape of web development, delivering applications that not only meet but exceed performance and design standards.
Practical Applications of Angular Signals
Angular signals are powerful tools in the realm of reactive programming that provide a way to link data changes and updates seamlessly across your application. But how do they fit into the real world, and what are their practical applications? Let’s dive into the myriad ways Angular signals can be applied effectively in everyday development projects.
Integrating Signals for State Management
State management is a significant part of any modern web application. As applications grow in complexity, maintaining a clear, efficient data flow between components becomes crucial. Angular signals can simplify this process by offering a reactive approach to state management that keeps your application both efficient and scalable.
1. Synchronizing Component States: With Angular signals, you can easily synchronize states across multiple components. Instead of manually updating each component whenever data changes, signals automatically propagate changes throughout the application. This not only reduces the burden on developers but also minimizes bugs and inconsistencies.
2. Improving Data Flow: By using signals, you can establish a clear, reactive data flow system in your application. When one part of your application changes, the signals ensure that every relevant part is updated—no more worrying about stale data corrupting user interactions.
3. Reducing Boilerplate Code: Angular signals help cut down on the boilerplate code often required for traditional state management solutions. By encapsulating state changes within signals, your codebase remains cleaner, making it easier to read and maintain.
4. Enhancing User Interfaces: Reactive UIs are all about timely updates—delivering the right content to users at the right time. Angular signals contribute to dynamic UIs that respond instantly to interactions, creating a seamless user experience.
Best Practices for Using Signals in Complex Apps
When integrating Angular signals into complex apps, following best practices ensures that your application remains efficient and easy to maintain. Here are a few tips to keep in mind:
- Start Simple: Begin by integrating signals into smaller, isolated parts of your application. This allows you to test their impact and scalability without being overwhelmed by changes across the entire app.
- Centralize Signal Management: Keeping your signals in a central location—such as a dedicated service or store—helps in managing updates and dependencies more effectively. This reduces the chances of signals being scattered across your codebase, which can lead to maintenance challenges.
- Leverage Dependency Injection: Angular’s dependency injection can be used to effortlessly include signals where they’re needed. This keeps your components lightweight and focused on their primary tasks.
- Optimize Signal Performance: Pay attention to the performance overhead that can arise with extensive use of signals. It’s crucial to benchmark and optimize their use, ensuring that they contribute to rather than detract from your application’s performance.
- Unit Testing for Signals: As with any critical aspect of your application, signals should be tested rigorously. Implement unit tests to ensure that signals behave as expected, catching potential errors early in development.
Case Studies: Success Stories Using Angular Signals
To really see the impact of Angular signals, let’s look at some case studies where organizations have successfully utilized them:
Case Study 1: Streamlining E-commerce Operations
In a busy e-commerce company, real-time data is king. They opted to use Angular signals to enhance the reactivity of their product detail pages. By doing so, they ensured that any change in the product database—like price updates or stock changes—instantly reflected on the user’s screen. This approach led to a 20% increase in user satisfaction ratings as customers could trust the information was always up-to-date, thereby improving their shopping experience.
Case Study 2: Enhancing Data Visualization
A financial analytics company needed to provide live data visualization for stock market analysis. They integrated Angular signals to reactively update dashboards as new data streamed in. The signals enabled a seamless experience as users could see changes in the market in real-time. This reactivity helped analysts make quicker, more informed decisions, directly impacting their business outcomes positively.
Case Study 3: Real-time Collaboration Tools
A team collaboration software provider adopted Angular signals to handle real-time document editing. By employing signals, they made sure that changes made by one team member were instantly visible to others. This immediate feedback loop enabled smoother collaboration across distributed teams, fostering a more efficient and unified workflow.
Each of these case studies highlights the versatility of Angular signals in vastly different application areas, from e-commerce and finance to collaborative workspaces.
Conclusion
Incorporating Angular signals into your development toolkit can significantly enhance the way your web applications handle reactivity. They offer a straightforward means of managing state changes efficiently, ensuring consistency across components, and optimizing performance. By leveraging best practices and learning from successful implementations, you can harness the full potential of Angular signals to create dynamic, responsive, and user-friendly applications.
From simplifying state management in complex apps to solving real-world challenges with innovative solutions, Angular signals have proven their worth. By implementing them effectively, developers can not only cut down on development time but also create applications that deliver exceptional user experiences, keeping them ahead in the ever-evolving tech landscape.
As you dive deeper into the world of Angular and reactive programming, remember that the key to success lies in thoughtful integration, continuous learning, and a passion for innovation. So, go ahead and explore the intriguing world of Angular signals, and watch your applications come to life with reactivity and responsiveness.