Introduction
React Router has long been a staple in the React ecosystem, primarily known for its role in handling navigation in single-page applications (SPAs). However, this powerful library has much more to offer beyond its conventional use. In this article, we’ll explore innovative and unconventional ways to leverage React Router, unlocking its full potential to enhance your React applications.
Before we dive into the advanced techniques, let’s briefly recap what React Router is and its common uses:
React Router is a standard routing library for React applications. It enables the creation of single-page applications with dynamic, client-side routing. Typically, developers use React Router to:
- Define routes for different components
- Handle navigation between pages
- Manage URL parameters and query strings
- Create nested routes
While these are essential functions, React Router’s capabilities extend far beyond basic navigation. Let’s explore some innovative uses that can take your React applications to the next level.
Dynamic Component Loading
One of the most powerful features of React Router is its ability to facilitate dynamic component loading, also known as code splitting or lazy loading. This technique can significantly improve your application’s performance by loading components only when they’re needed.
Benefits of Dynamic Component Loading with React Router
- Faster initial load times
- Reduced bundle size
- Improved performance on low-end devices
- Better user experience
Implementing Dynamic Component Loading
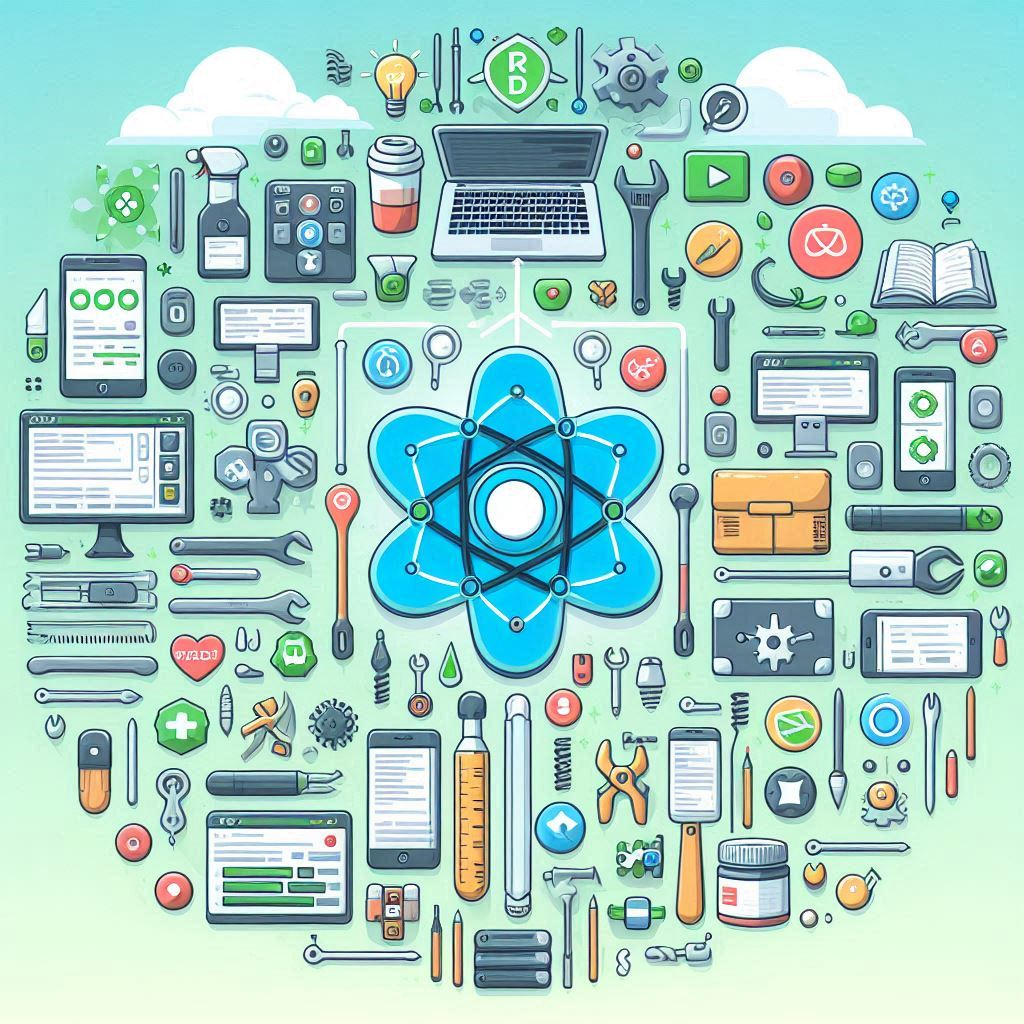
Here’s an example of how you can implement dynamic component loading using React Router:
import React, { lazy, Suspense } from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
const Home = lazy(() => import('./components/Home'));
const About = lazy(() => import('./components/About'));
const Contact = lazy(() => import('./components/Contact'));
function App() {
return (
<Router>
<Suspense fallback={<div>Loading...</div>}>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</Switch>
</Suspense>
</Router>
);
}
export default App;
In this example, we use the lazy()
function to dynamically import components. The Suspense
component provides a fallback UI while the lazy-loaded components are being loaded.
State Management with React Router
While libraries like Redux and MobX are popular choices for state management in React applications, React Router can also be utilized for managing certain aspects of your application’s state.
How React Router Can Be Used for State Management
- URL as a source of truth
- Storing state in route parameters
- Using location state for temporary data
Here’s an example of how you can use React Router for state management:
import React from 'react';
import { useHistory, useLocation } from 'react-router-dom';
function SearchComponent() {
const history = useHistory();
const location = useLocation();
const searchParams = new URLSearchParams(location.search);
const query = searchParams.get('q') || '';
const handleSearch = (event) => {
const newQuery = event.target.value;
history.push(`/search?q=${newQuery}`);
};
return (
<div>
<input
type="text"
value={query}
onChange={handleSearch}
placeholder="Search..."
/>
<p>Search results for: {query}</p>
</div>
);
}
export default SearchComponent;
In this example, we use the URL query parameter to store the search query. This approach allows users to share and bookmark specific search results easily.
Using React Router for Conditional Rendering
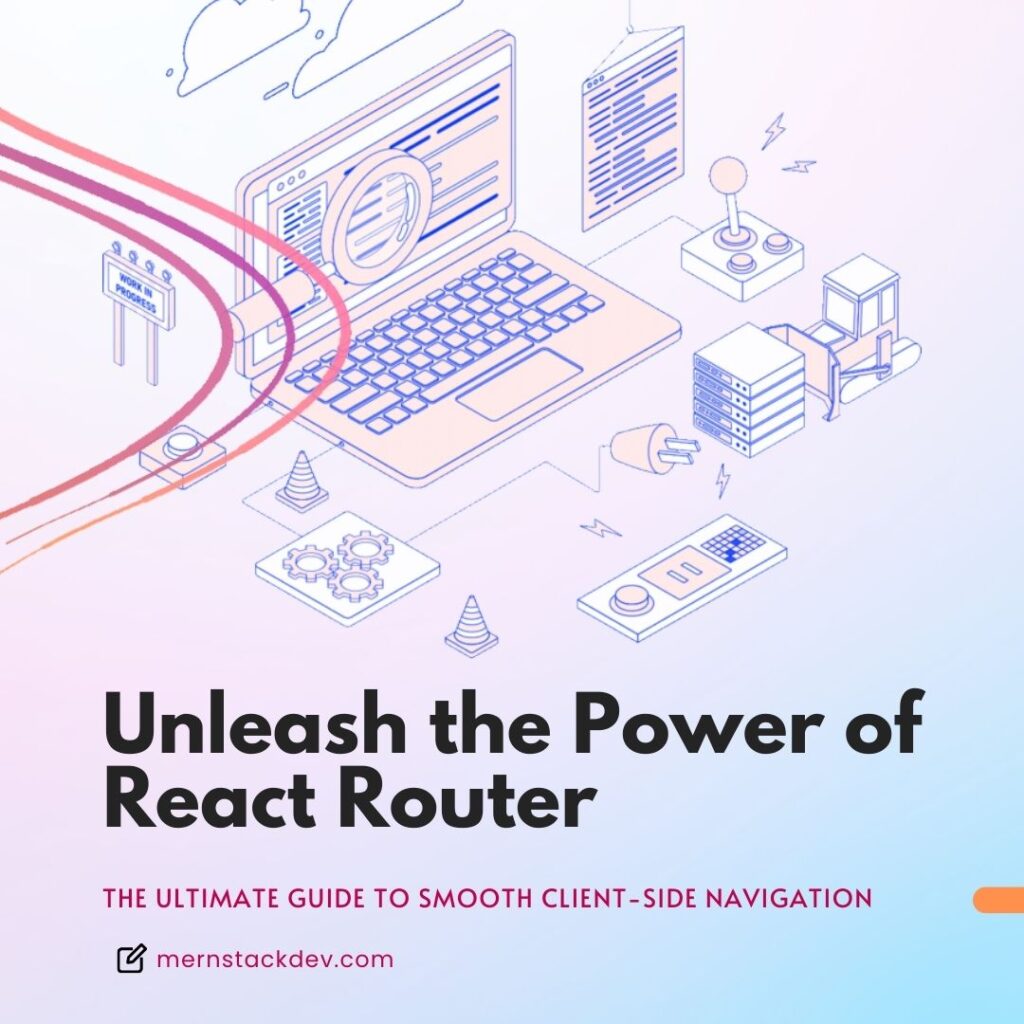
Conditional rendering is a common pattern in React applications, and React Router can be leveraged to implement this pattern in a clean and efficient manner.
Implementing Conditional Rendering with React Router
React Router’s Switch
component and the exact
prop can be used to create sophisticated conditional rendering logic:
import React from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
function App() {
return (
<Router>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/users/:id" component={UserProfile} />
<Route path="/users" component={UserList} />
<Route component={NotFound} />
</Switch>
</Router>
);
}
export default App;
In this example, the Switch
component ensures that only one route is rendered at a time. The order of routes matters, with more specific routes placed before more general ones.
Integrating React Router with Redux
While React Router can handle some aspects of state management, for complex applications, you might want to integrate it with a more robust state management solution like Redux.
Steps to Integrate React Router with Redux
- Install necessary dependencies
- Create a connected router component
- Use
connected-react-router
middleware - Dispatch navigation actions
Here’s a basic example of how to integrate React Router with Redux:
import React from 'react';
import { Provider } from 'react-redux';
import { ConnectedRouter } from 'connected-react-router';
import { Route, Switch } from 'react-router-dom';
import configureStore, { history } from './configureStore';
const store = configureStore();
function App() {
return (
<Provider store={store}>
<ConnectedRouter history={history}>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</Switch>
</ConnectedRouter>
</Provider>
);
}
export default App;
This setup allows you to dispatch navigation actions from your Redux actions, keeping your routing in sync with your application state.
Role-Based Access Control
Implementing role-based access control (RBAC) is crucial for many applications, and React Router can be an excellent tool for this purpose.
Implementing RBAC with React Router
You can create a higher-order component (HOC) or a custom route component to handle role-based access:
import React from 'react';
import { Route, Redirect } from 'react-router-dom';
function PrivateRoute({ component: Component, roles, ...rest }) {
return (
<Route
{...rest}
render={props => {
const currentUser = authService.getCurrentUser();
if (!currentUser) {
return <Redirect to="/login" />;
}
if (roles && !roles.includes(currentUser.role)) {
return <Redirect to="/unauthorized" />;
}
return <Component {...props} />;
}}
/>
);
}
export default PrivateRoute;
You can then use this PrivateRoute
component in your main routing configuration:
<Switch>
<Route exact path="/" component={Home} />
<PrivateRoute path="/admin" roles={['ADMIN']} component={AdminDashboard} />
<PrivateRoute path="/user" roles={['USER', 'ADMIN']} component={UserDashboard} />
</Switch>
This approach ensures that only users with the appropriate roles can access certain routes.
Creating Wizard Forms with React Router
Wizard forms, or multi-step forms, are a common pattern in web applications. React Router can be used to create a clean and intuitive wizard form interface.
Step-by-Step Guide to Create Wizard Forms Using React Router
- Define routes for each step of the form
- Create a component for each step
- Use React Router’s history to navigate between steps
- Store form data in a shared state or context
Here’s a basic example of how to implement a wizard form with React Router:
import React, { useState } from 'react';
import { BrowserRouter as Router, Route, Switch, useHistory } from 'react-router-dom';
function WizardForm() {
const [formData, setFormData] = useState({});
const history = useHistory();
const handleNext = (stepData) => {
setFormData({ ...formData, ...stepData });
history.push('/step2');
};
return (
<Router>
<Switch>
<Route exact path="/">
<Step1 onNext={handleNext} />
</Route>
<Route path="/step2">
<Step2 formData={formData} />
</Route>
</Switch>
</Router>
);
}
function Step1({ onNext }) {
const [name, setName] = useState('');
const handleSubmit = (e) => {
e.preventDefault();
onNext({ name });
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
placeholder="Enter your name"
/>
<button type="submit">Next</button>
</form>
);
}
function Step2({ formData }) {
return <h1>Welcome, {formData.name}!</h1>;
}
export default WizardForm;
This example demonstrates a simple two-step wizard form using React Router. You can extend this pattern to create more complex multi-step forms.
SEO Optimization with React Router
Search Engine Optimization (SEO) is crucial for single-page applications, and React Router can play a significant role in improving your app’s SEO.
Techniques for SEO Optimization Using React Router
- Use semantic URLs
- Implement server-side rendering (SSR)
- Utilize React Helmet for dynamic meta tags
- Create a sitemap
Here’s an example of how you can use React Router with React Helmet for better SEO:
import React from 'react';
import { Route } from 'react-router-dom';
import { Helmet } from 'react-helmet';
function SEORoute({ path, component: Component, title, description }) {
return (
<Route
path={path}
render={props => (
<>
<Helmet>
<title>{title}</title>
<meta name="description" content={description} />
</Helmet>
<Component {...props} />
</>
)}
/>
);
}
function App() {
return (
<Router>
<Switch>
<SEORoute
exact
path="/"
component={Home}
title="Home | My App"
description="Welcome to My App, a React application"
/>
<SEORoute
path="/about"
component={About}
title="About Us | My App"
description="Learn more about My App and our team"
/>
</Switch>
</Router>
);
}
export default App;
This approach allows you to set dynamic meta tags for each route, improving your application’s SEO.
Frequently Asked Questions (FAQ)
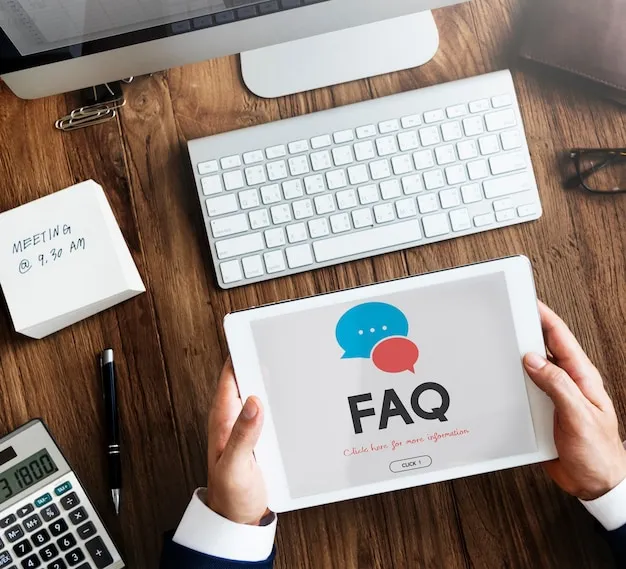
What is React Router?
React Router is a standard routing library for React applications. It enables developers to build single-page applications with dynamic, client-side routing.
Can React Router be used for state management?
While React Router is primarily a routing library, it can be used for certain aspects of state management, particularly for state that is closely tied to the URL or navigation.
How does React Router help with SEO?
React Router can help with SEO by allowing you to create semantic URLs, implement server-side rendering, and dynamically update meta tags for each route.
What are some common issues with React Router and how to fix them?
Common issues include nested routes not rendering correctly, browser back button not working as expected, and route parameters not updating. These can often be resolved by proper route configuration, using the Switch
component, and leveraging hooks like useParams
and useLocation
.
Can React Router be used with other libraries like Redux?
Yes, React Router can be integrated with state management libraries like Redux. Libraries such as connected-react-router
can help synchronize your routing with your Redux store.
Conclusion
React Router is a powerful and versatile library that extends far beyond its primary use for navigation. By leveraging its advanced features, you can enhance your React applications in numerous ways:
- Implement dynamic component loading for improved performance
- Utilize it for certain aspects of state management
- Create sophisticated conditional rendering logic
- Implement role-based access control
- Build intuitive wizard forms
- Optimize your application for search engines
As you continue to work with React Router, don’t be afraid to think outside the box and explore new ways to use this flexible tool. The examples provided in this article are just the beginning – there are countless other innovative uses waiting to be discovered.
We encourage you to experiment with these techniques in your own projects and share your unique uses of React Router with the community. By pushing the boundaries of what’s possible, we can all contribute to the evolution of React development practices.
Have you found an innovative way to use React Router in your projects? Share your experiences in the comments below or on social media using #ReactRouterInnovations. Your insights could inspire the next breakthrough in React development!