In the world of database management, performance is paramount. For MongoDB users, mastering indexing strategies is crucial for achieving optimal query performance and efficient data retrieval. This comprehensive guide will delve into the intricacies of MongoDB indexing, providing you with the knowledge and tools to supercharge your database operations.
Introduction
MongoDB, a popular NoSQL database, offers flexible and scalable solutions for modern applications. However, as data volumes grow, so does the need for efficient querying. This is where indexing comes into play.
Indexing in MongoDB is akin to creating a table of contents for your data. It allows the database to quickly locate and retrieve specific information without scanning the entire collection. The importance of proper indexing cannot be overstated:
- It dramatically reduces query execution time
- It optimizes resource utilization
- It enhances overall application performance
In this article, we’ll explore various indexing strategies, best practices, and advanced techniques to help you maximize MongoDB’s potential.
Understanding MongoDB Indexes
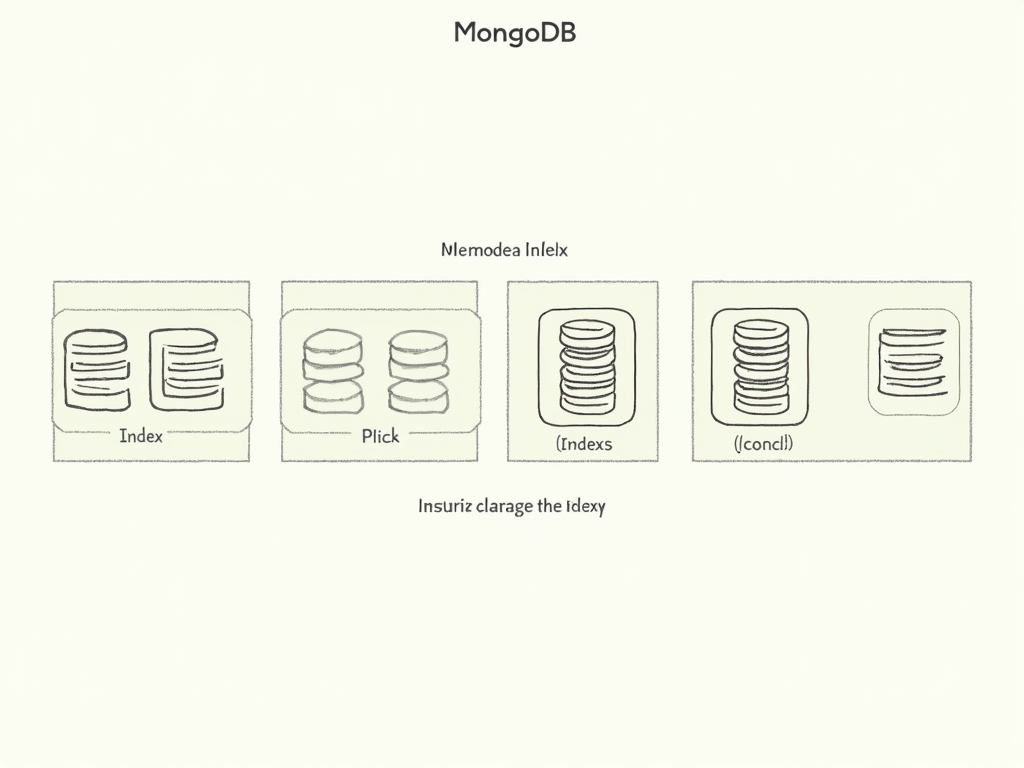
What is an index in MongoDB?
An index in MongoDB is a special data structure that stores a small portion of the collection’s data set in an easy-to-traverse form. It contains the values of specific fields or sets of fields, ordered by those values. This allows MongoDB to efficiently execute queries, sort operations, and aggregations.
Types of indexes available in MongoDB
MongoDB offers several types of indexes to cater to different querying needs:
- Single-field indexes: These are the most basic type of indexes, created on a single field of a document.
- Compound indexes: These indexes include multiple fields, allowing for more complex query optimization.
- Multikey indexes: Used for indexing array fields, these indexes create separate index entries for each element of the array.
- Text indexes: Specifically designed for text search operations, these indexes support searching for string content in a collection.
- Geospatial indexes: These specialized indexes support efficient queries for location-based data.
Creating and Managing Indexes
How to create an index in MongoDB
Creating an index in MongoDB is straightforward. Here’s a basic example:
db.collection.createIndex({ fieldName: 1 })
This command creates an ascending index on the fieldName
field. Use -1
for a descending index.
Managing existing indexes
To view existing indexes on a collection:
db.collection.getIndexes()
Dropping indexes that are no longer needed
Removing unnecessary indexes is crucial for maintaining optimal performance:
db.collection.dropIndex({ fieldName: 1 })
Understanding index prefixes and ordering
Index prefixes refer to the leftmost fields in a compound index. They play a crucial role in query optimization. For example, in a compound index { a: 1, b: 1, c: 1 }
, the prefixes are { a: 1 }
, { a: 1, b: 1 }
, and the full index.
Strategies for Effective Indexing
Analyzing query patterns
Before creating indexes, it’s essential to understand your application’s query patterns. Use MongoDB’s built-in tools like the explain()
method to analyze query performance and identify frequently used fields in your queries.
Choosing the right type of index
Select the appropriate index type based on your query requirements:
- Use single-field indexes for simple queries on one field
- Opt for compound indexes for queries that involve multiple fields
- Consider text indexes for full-text search capabilities
- Implement geospatial indexes for location-based queries
Leveraging compound indexes for complex queries
Compound indexes can significantly improve performance for queries involving multiple fields. Consider this example:
db.users.createIndex({ lastName: 1, firstName: 1, age: -1 })
This index supports queries on:
lastName
lastName
andfirstName
lastName
,firstName
, andage
Partial indexes for specific use cases
Partial indexes only index a subset of documents in a collection, reducing index size and improving insert performance. They’re particularly useful for collections where a small percentage of documents are queried frequently.
db.restaurants.createIndex(
{ cuisine: 1, name: 1 },
{ partialFilterExpression: { rating: { $gt: 5 } } }
)
This index only includes restaurants with a rating greater than 5.
Sparse indexes to handle null values
Sparse indexes only contain entries for documents that have the indexed field, even if it’s null. This can be beneficial for fields that are present in only a subset of documents.
db.users.createIndex({ email: 1 }, { sparse: true })
Optimizing Index Performance
Analyzing and reducing index size
Large indexes can negatively impact performance. Regularly monitor index sizes using:
db.collection.stats().indexSizes
Consider using partial or sparse indexes to reduce size when appropriate.
Indexing strategies for high write operations
For write-heavy applications:
- Limit the number of indexes
- Consider background indexing for large collections
- Use partial indexes to reduce the impact on write performance
Using explain() to analyze query performance
The explain()
method is invaluable for understanding how MongoDB executes queries:
db.users.find({ age: { $gt: 30 } }).explain("executionStats")
This provides detailed information about query execution, including index usage.
Understanding and using covered queries
A covered query is one where all the fields in the query are part of an index, including the projected fields. These queries are highly efficient as MongoDB can return results using only the index.
Avoiding index contention
Index contention occurs when multiple threads attempt to modify the same index simultaneously. To mitigate this:
- Spread writes across multiple collections
- Use compound indexes strategically
- Consider using hashed indexes for high-cardinality fields
Advanced Indexing Techniques
Unique indexes for data integrity
Unique indexes ensure that the indexed fields contain unique values:
db.users.createIndex({ email: 1 }, { unique: true })
TTL indexes for time-based data management
Time-To-Live (TTL) indexes automatically remove documents after a specified amount of time:
db.sessionData.createIndex({ lastModifiedDate: 1 }, { expireAfterSeconds: 3600 })
Geospatial indexes for location-based queries
For applications dealing with geographic data:
db.places.createIndex({ location: "2dsphere" })
This enables efficient geospatial queries.
Text indexes for full-text search
Text indexes support text search queries on string content:
db.articles.createIndex({ content: "text" })
Common Indexing Mistakes and How to Avoid Them
- Over-indexing: Creating too many indexes can slow down write operations. Focus on indexes that support your most common and performance-critical queries.
- Ignoring index cardinality: High-cardinality fields (fields with many unique values) are often good candidates for indexing, while low-cardinality fields may not provide significant benefits.
- Misusing compound indexes: Ensure the order of fields in compound indexes aligns with your query patterns.
- Not analyzing slow queries: Regularly review and optimize slow-running queries using MongoDB’s profiling tools.
Indexing in Sharded Clusters
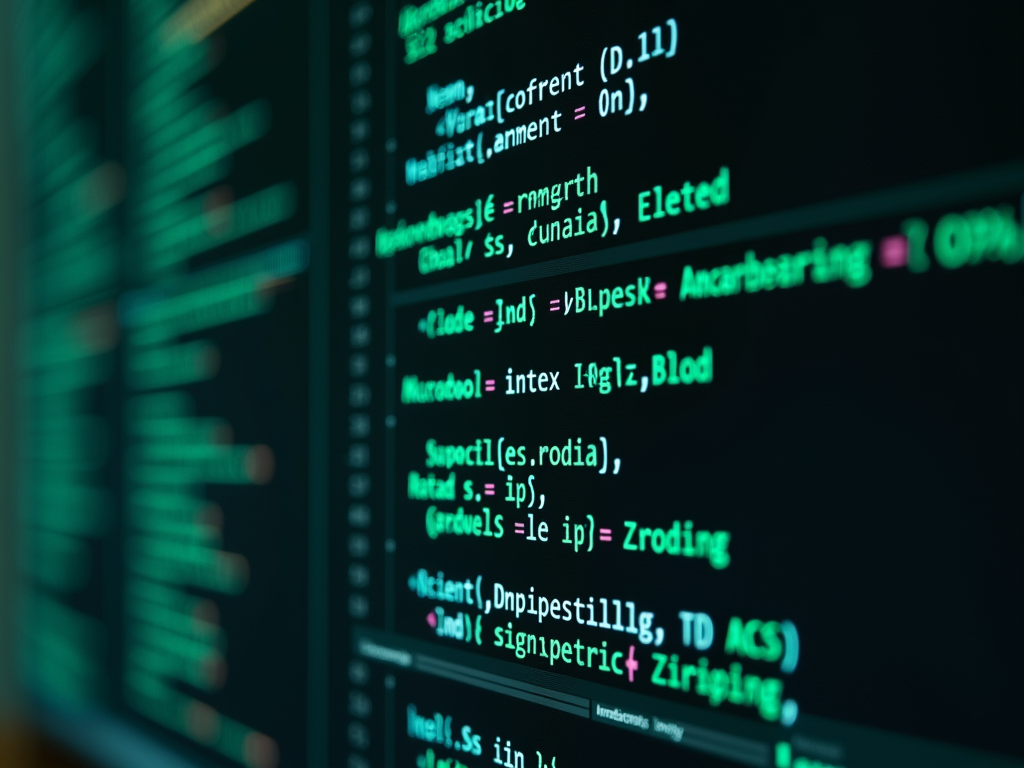
In a sharded environment:
- Ensure the shard key is included in your indexes
- Consider the impact of indexing on data distribution
- Use zone sharding to optimize data locality
Monitoring and Maintaining Indexes
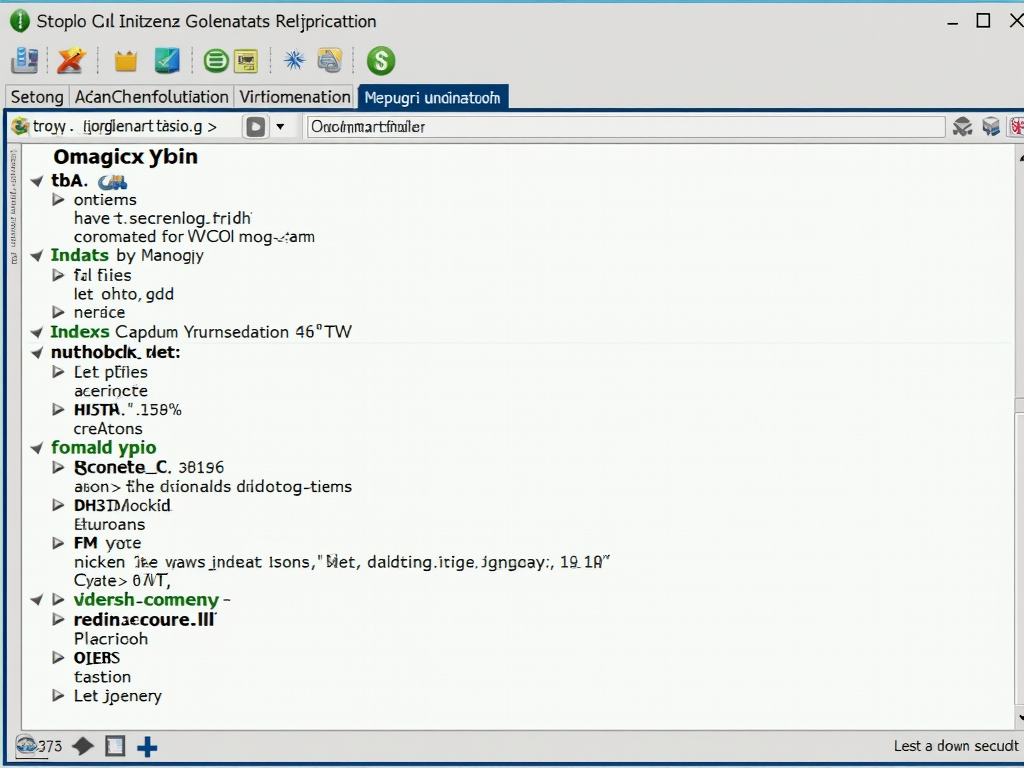
Tools for monitoring index performance
- MongoDB Compass: Provides a visual interface for analyzing index usage
- MongoDB Atlas: Offers advanced monitoring features
db.collection.aggregate()
with$indexStats
: Provides detailed index usage statistics
Regular maintenance tasks for indexes
- Review and remove unused indexes
- Rebuild indexes periodically to reduce fragmentation
- Monitor index size and performance regularly
When to rebuild indexes
Consider rebuilding indexes when:
- There’s significant fragmentation
- After large bulk insert operations
- When upgrading to a new MongoDB version
Automating index management tasks
Use MongoDB’s native scripting capabilities or third-party tools to automate routine index management tasks, ensuring consistent performance over time.
Conclusion
Effective indexing is crucial for maintaining high performance in MongoDB databases. By understanding the various types of indexes, implementing strategic indexing techniques, and following best practices, you can significantly enhance your database’s efficiency and query performance.
Remember these key points:
- Analyze your query patterns before creating indexes
- Use compound indexes for complex queries
- Regularly monitor and maintain your indexes
- Avoid common pitfalls like over-indexing and ignoring cardinality
By applying these strategies and continually optimizing your indexing approach, you’ll be well on your way to achieving peak MongoDB performance.
FAQ Section
Q: What are the best practices for creating indexes in MongoDB?
A: Some key best practices include:
- Create indexes to support your most common and performance-critical queries
- Use compound indexes for queries involving multiple fields
- Consider the order of fields in compound indexes
- Avoid creating unnecessary indexes that aren’t used by your queries
- Monitor and analyze index usage regularly
Q: How do compound indexes improve query performance?
A: Compound indexes can significantly improve performance by:
- Supporting queries that involve multiple fields
- Allowing MongoDB to satisfy queries using only the index, without accessing the documents (covered queries)
- Providing flexibility for various query patterns using index prefixes
Q: When should I use partial indexes?
A: Partial indexes are beneficial when:
- You frequently query a specific subset of your collection
- You want to reduce the size and maintenance overhead of your indexes
- You need to index a large collection but only on a small subset of documents
Q: What tools can I use to monitor my MongoDB indexes?
A: Several tools are available for monitoring MongoDB indexes:
db.collection.stats()
: Provides statistics about a collection, including index sizesexplain()
: Offers detailed information about query execution and index usage- MongoDB Compass: Provides a visual interface for analyzing index performance
- MongoDB Atlas: Offers advanced monitoring features for cloud-hosted databases
Q: How do I avoid over-indexing in MongoDB?
A: To prevent over-indexing:
- Analyze your query patterns and create indexes only for frequently used queries
- Use compound indexes instead of multiple single-field indexes where possible
- Regularly review and remove unused indexes
- Monitor the impact of indexes on write performance
- Consider the trade-offs between query performance and write overhead
By following these guidelines and continuously optimizing your indexing strategy, you can ensure that your MongoDB databases perform at their best, providing fast and efficient data retrieval for your applications.