Introduction
The \`equals\` method in Java is a fundamental part of the language that developers frequently utilize to compare objects. Understanding how to effectively use this method is crucial for implementing logical equality checks in your Java programs. Unlike the ‘==’ operator, which compares references or memory addresses, the \`equals\` method can be overridden to compare the actual contents of objects. This distinct functionality ensures more precise and meaningful comparisons, particularly in complex applications manipulating custom objects.
Basics of the Equal Method
Definition of the Equal Method
The \`equals()\` method in Java is a procedure defined in the \`Object\` class, which forms the basis of all classes in Java. The primary purpose of this method is to compare two objects for equivalence in terms of their state or data content, rather than their references (memory addresses). When a class does not explicitly override the \`equals()\` method, it inherits the default behavior from the \`Object\` class. This default implementation equates two references if and only if they point to the exact same object, meaning they are identical in every way, including their memory address.
Importance of Object Comparison in Java
Object comparison is a foundational concept in Java programming because it affects how objects are distinguished and manipulated within the program. Accurate and efficient object comparison is crucial in scenarios such as:
– Determining uniqueness in collections that do not allow duplicates, like \`Set\`.
– Checking logical equivalence of instances in conditional structures.
– Managing sessions and user identities in Java-based applications.
Without a robust mechanism to compare objects based on their values, developers would be limited to reference comparison, which can lead to logic errors and inefficient code. For instance, two distinct \`String\` objects can contain identical sequences of characters but will be considered different when using default equality checking. This emphasizes the importance of implementing an appropriate \`equals()\` method that contrasts the actual data held by the objects.
How the Equal Method Works
Syntax and Implementation
The \`equals()\` method can be overridden to compare objects based on their internal states. The syntax for this method is as follows:
public boolean equals(Object obj) {
// Method implementation
}
To override the \`equals()\` method, you must follow certain principles to ensure its correct behavior:
1. Reflexive: For any non-null reference value \`x\`, \`x.equals(x)\` should return \`true\`.
2. Symmetric: For any non-null reference values \`x\` and \`y\`, \`x.equals(y)\` should return \`true\` if and only if \`y.equals(x)\` returns \`true\`.
3. Transitive: For any non-null reference values \`x\`, \`y\`, and \`z\`, if \`x.equals(y)\` returns \`true\` and \`y.equals(z)\` returns \`true\`, then \`x.equals(z)\` should also return \`true\`.
4. Consistent: For any non-null reference values \`x\` and \`y\`, multiple invocations of \`x.equals(y)\` consistently return \`true\` or consistently return \`false\`.
5. Null case: For any non-null reference value \`x\`, \`x.equals(null)\` should return \`false\`.
By adhering to these principles, the \`equals()\` method becomes a reliable tool for assessing object equivalence accurately and consistently.
Comparison with == Operator
It’s essential to distinguish between the \`equals()\` method and the \`==\` operator. While the \`equals()\` method is intended for checking logical equality based on the data content of the objects, the \`==\` operator compares the memory addresses or references of the two objects. Here is what you need to keep in mind:
– Primitive types: For primitive data types (like \`int\`, \`char\`, etc.), \`==\` compares the actual values and is sufficient for equality testing.
– Objects: For objects, \`==\` solely checks if both references point to the same object, which does not necessarily imply equivalence in terms of the state or contents of the objects.
To illustrate, consider two \`String\` objects:
String a = new String("example");
String b = new String("example");
Using \`a == b\` will return \`false\` because \`a\` and \`b\` point to different objects in memory. However, \`a.equals(b)\` will return \`true\` because it compares the strings’ contents, which are identical.
Return Values of the Equal Method
The \`equals()\` method returns a boolean value:
– true: Indicates that the two compared objects are equivalent in terms of their data or state.
– false: Indicates that the objects do not share equivalence.
This method plays a critical role in decision-making processes throughout Java programs, especially when sorting, storing, or retrieving objects is based on logical equality rather than reference equality. Effective use of this method helps ensure that the program behaves as expected when dealing with object interactions and collections management.
Best Practices for Using the Equal Method
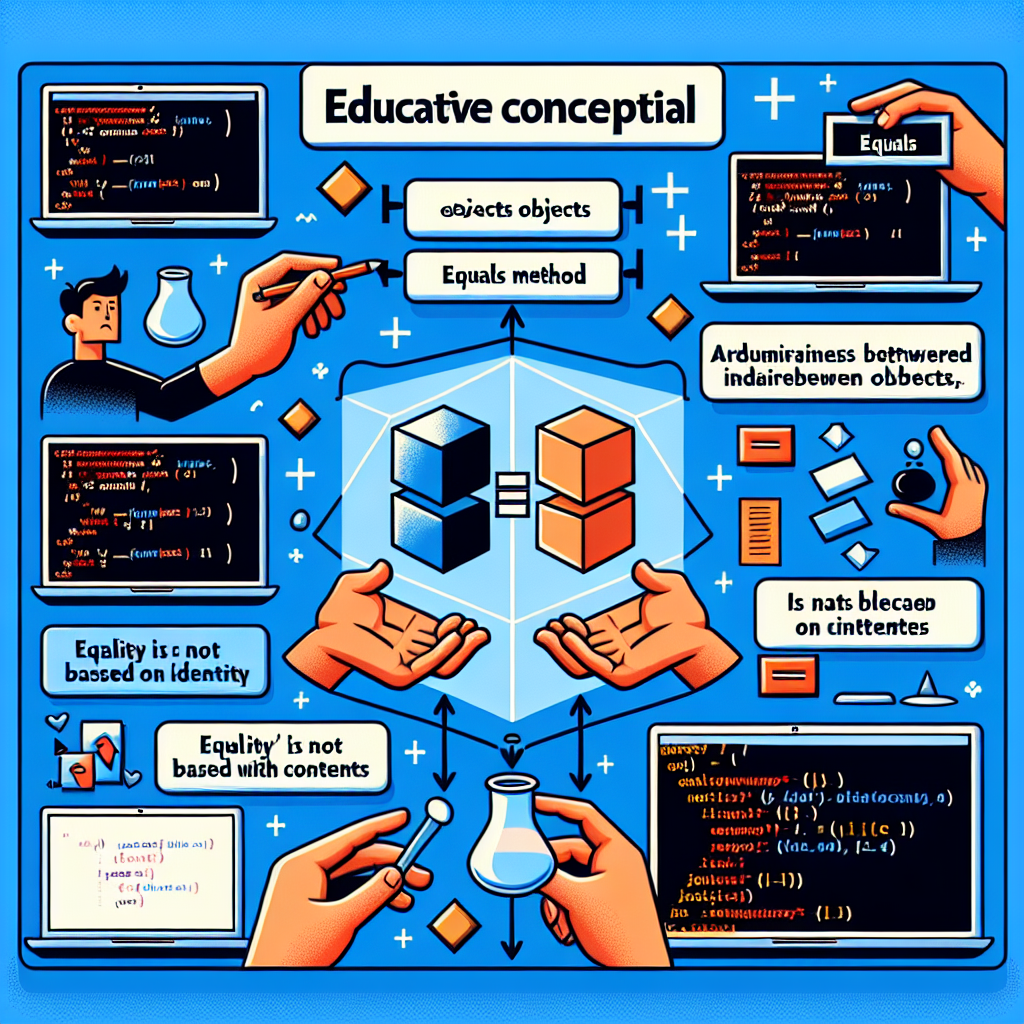
When using the equal method in Java, it’s important to adhere to certain best practices to ensure your code’s reliability and maintainability. The equality method, \`.equals()\`, is critical in Java because it affects how objects are compared, affecting the behavior of lists, maps, and other collections that rely on it for correct operation.
Overriding the Equal Method
When overriding the \`equals()\` method, follow these guidelines:
– Consistency: The method must return the same result as long as the objects compared are unchanged. Inconsistencies can lead to unpredictable behavior in collections.
– Symmetry: For two references, \`a\` and \`b\`, if \`a.equals(b)\` is true, then \`b.equals(a)\` must also be true.
– Transitivity: If \`a.equals(b)\` is true and \`b.equals(c)\` is true, then \`a.equals(c)\` must also be true.
– Reflexivity: Any non-null reference value \`a\` must equal itself (\`a.equals(a)\` should return true).
– Null safety: The \`equals()\` method should return false if the object compared is null, rather than throwing a \`NullPointerException\`.
It is also advisable to override \`hashCode()\` whenever you override \`equals()\`. Consistent hash codes must be produced for objects that are equal, ensuring correct behavior in hash-based collections like \`HashMap\` and \`HashSet\`.
Common Mistakes to Avoid
Several pitfalls could lead to improper behavior of the \`equals()\` method if not adequately managed:
– Comparing incompatible types: Ensure that the objects being compared are instances of the same class or logically compatible classes.
– Ignoring case sensitivity in string comparisons: Depending on the business logic, sometimes ignoring or considering case sensitivity can make a substantial difference.
– Not considering fields from parent classes: If an object inherits properties from a parent class, ensure these are also considered in the equality comparison.
– Overriding equals but not hashCode: This can cause problems in collections that use the hash code, such as \`HashMap\` or \`HashSet\`.
Performance Considerations
Implementing an efficient \`equals()\` method is crucial, especially when dealing with large collections or complex objects:
– Avoid unnecessary comparisons: Start with checking fields that are more likely to differ and less expensive to compare.
– Short-circuit evaluation: Use short-circuiting to exit the method as soon as a difference is found.
– Use of lazy initialization: Delay the calculation of complex fields until necessary, and cache the results if the computation is expensive.
Examples of Using the Equal Method
Image courtesy: Unsplash
To illustrate the proper use and impact of the \`equals()\` method in Java, consider these practical scenarios involving both primitive data types and objects of user-defined classes.
Comparing Primitive Data Types
In Java, primitive data types (like int, float, etc.) are compared using the basic relational operators (\`==\`, \`!=\`, \`<\`, \`>\`, etc.), not the \`equals()\` method. Here’s an example:
int a = 5;
int b = 5;
System.out.println(a == b); // Outputs true
In this case, \`==\` checks if the values are identical, which is sufficient for primitive types. However, when dealing with object wrappers of these primitives (like \`Integer\`, \`Double\`), the \`equals()\` method becomes useful. For instance:
public class IntegerComparisonDemo {
public static void main(String[] args) {
Integer a = new Integer(5);
Integer b = new Integer(5);
System.out.println(a.equals(b)); // Outputs true
}
}
The \`.equals()\` method here checks the equality of the values of the objects, not just their references.
Comparing Objects of User-Defined Classes
For custom classes, you are responsible for overriding the \`equals()\` method to perform a deep comparison based on the fields of the objects. Consider a simple \`Car\` class:
public class Car {
private String make;
private String model;
private int year;
public Car(String make, String model, int year) {
this.make = make;
this.model = model;
this.year = year;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Car car = (Car) obj;
return year == car.year &&
Objects.equals(make, car.make) &&
Objects.equals(model, car.model);
}
@Override
public int hashCode() {
return Objects.hash(make, model, year);
}
}
In this example, the \`equals()\` method checks if the other object is a \`Car\` and then compares its make, model, and year to determine equality. This method ensures that two \`Car\` objects with the same make, model, and year are considered equal, fulfilling the requirements for symmetry, transitivity, and reflexivity.
These examples highlight how critical a correctly implemented \`equals()\` method is in ensuring that your Java applications function as expected, preserving logic integrity and data consistency across your projects.
Conclusion
Understanding the \`equals\` method in Java and its proper implementation is crucial for any Java developer aiming to handle object comparisons effectively within their applications. Through this method, you can ensure logical equality between objects, distinguishing it clearly from the identity comparison provided by the \`==\` operator, which merely checks if two references point to the same object.
Correct usage of the \`equals\` method necessitates adherence to specific guidelines and principles that preserve its contract: reflexivity, symmetry, transitivity, consistency, and the non-null reference requirement, all of which are critical to avoid unexpected behavior and bugs in your code. For instance, violating symmetry by creating an \`equals\` method that isn’t symmetric can lead to confusing results when objects of two different types are compared, potentially causing major logic flaws in an application that relies on sets or maps.
Moreover, when defining an \`equals\` method, especially in a class hierarchy, it is advisable to also override the \`hashCode\` method. This is to ensure that the general contract of the \`hashCode\` method, which states that equal objects must have equal hash codes, remains intact. Neglecting to do so can lead to issues, particularly when objects are inserted into hash-based collections such as \`HashMap\` and \`HashSet\`.
Employing the \`equals\` method also requires careful consideration of its impact on performance. In complex classes with many fields, the \`equals\` method might become a bottleneck if not implemented efficiently. Strategies to enhance performance can include checking fields that are more likely to differ first or those that are faster to compare, and avoiding unnecessary type checking and conversions.
The correct mastery of the \`equals\` method allows for robust, accurate, and efficient object comparisons, which are pivotal in many Java applications. From managing collections effectively to ensuring the logical correctness of custom data types, this method plays a fundamental role in Java programming. Thus, leveraging the principles discussed, alongside a thorough understanding of your application’s requirements, will empower you to use the \`equals}’ method to its fullest potential, ensuring your Java applications are both reliable and maintainable.
FAQ
Image courtesy: Unsplash
What is the difference between == and equals() method in Java?
The == operator in Java checks for reference equality, meaning it checks whether two references point to the same object in memory. In contrast, the equals() method is intended for content comparison. It checks if the values inside the objects are identical, but it’s essential to override it from the Object class to provide custom equality logic based on the fields of your specific classes.
When should I use equals() method?
You should use the equals() method when you need to compare the equality of two object instances based on their data or state. This is common in collections such as ArrayList, HashMap, where object equality is necessary for operations like removing duplicate objects, checking for object existence, or replacing one object with another.
– Comparing data entities: When comparing instances of data containers like custom classes for users, products, etc.
– In collections: Especially useful in set and list operations where uniqueness of elements is a focus.
How do I correctly override the equals() method?
To correctly override the equals() method in Java, follow these key steps:
– Check if the current object is compared to itself using ‘this’.
– Verify that the object is not null and is an instance of the correct class.
– Cast the object to the appropriate type.
– Compare all relevant fields typically involved in your equality check to ensure they match.
For example:
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
MyCustomClass other = (MyCustomClass) obj;
return field1.equals(other.field1) &&
field2.equals(other.field2);
}
Does the equals() method perform a deep comparison?
The default equals() method in the Object class does not perform a deep comparison. It merely checks if two object references point to the same memory location. However, when you override the equals() method, you can define a deep comparison based on the fields that determine object equality. This usually involves comparing each significant field manually unless those fields themselves dictate another reference identity or shallow comparison.