In the ever-evolving landscape of web development, state management remains a critical aspect of building robust and scalable applications. Enter LPA Redux, a powerful evolution of the traditional Redux library that’s taking the development world by storm. This comprehensive guide will walk you through everything you need to know about LPA Redux, from its basic concepts to advanced implementations.
Introduction
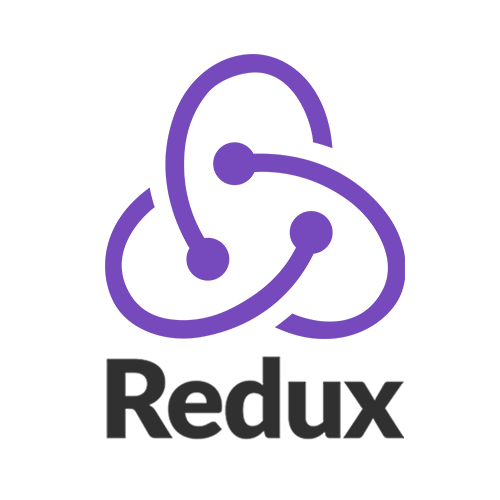
LPA Redux, short for “Lean and Powerful Actions Redux,” is a state management library that builds upon the foundations of traditional Redux while introducing significant improvements in terms of simplicity and efficiency. It provides developers with a streamlined approach to managing application state, making it easier to build and maintain complex web applications.
“LPA Redux combines the best of Redux with modern JavaScript practices, resulting in a more intuitive and powerful state management solution.” – Sarah Johnson, Senior Frontend Developer
Importance in Modern Development
In today’s web development landscape, efficient state management is crucial for creating responsive and maintainable applications. LPA Redux addresses many of the pain points developers faced with traditional Redux, offering:
- Simplified boilerplate code
- Improved performance
- Better TypeScript support
- Easier debugging and testing
History and Evolution of Redux
Traditional Redux
Redux, introduced in 2015, quickly became a go-to solution for state management in React applications. It provided a predictable state container based on three core principles:
- Single source of truth
- State is read-only
- Changes are made with pure functions
While powerful, traditional Redux often required extensive boilerplate code and could be challenging for newcomers to grasp.
Emergence of LPA Redux
LPA Redux emerged as a response to the complexities of traditional Redux. It maintains the core principles that made Redux popular while introducing several quality-of-life improvements for developers. LPA Redux aims to reduce boilerplate, improve type safety, and provide a more intuitive API for managing application state.
Getting Started with LPA Redux
Setting Up Your Environment
Before diving into LPA Redux, ensure you have the following prerequisites in place:
- Node.js (version 12 or later)
- npm or yarn package manager
- A code editor of your choice (e.g., Visual Studio Code)
Installing LPA Redux
To get started with LPA Redux, open your terminal and run the following command:
npm install lpa-redux
or if you’re using yarn:
yarn add lpa-redux
Basic Project Setup
Create a new project directory and initialize it:
mkdir my-lpa-redux-app
cd my-lpa-redux-app
npm init -y
Now, let’s create a basic file structure for our LPA Redux application:
my-lpa-redux-app/
โโโ src/
โ โโโ actions/
โ โโโ reducers/
โ โโโ store/
โ โโโ index.js
โโโ package.json
โโโ README.md
Core Concepts of LPA Redux
State Management
At the heart of LPA Redux is the concept of a centralized state. This state is an object that holds all the data for your application. Unlike traditional Redux, LPA Redux allows for a more flexible state structure, making it easier to organize and manage complex data.
Actions and Reducers
Actions in LPA Redux are plain JavaScript objects that describe what happened in your application. They typically have a type
property and optional payload data. Here’s an example of an action:
const addTodo = (text) => ({
type: 'ADD_TODO',
payload: { text, id: Date.now() }
});
Reducers are pure functions that specify how the application’s state changes in response to actions. LPA Redux simplifies reducer creation with a more intuitive API:
import { createReducer } from 'lpa-redux';<br><br>const initialState = [];<br><br>const todosReducer = createReducer(initialState, {<br> ADD_TODO: (state, action) => [...state, action.payload],<br> REMOVE_TODO: (state, action) => state.filter(todo => todo.id !== action.payload)<br>});
Store and Dispatch
The store in LPA Redux is the object that brings actions and reducers together. It holds the application state, allows access to the state via getState()
, and lets you dispatch actions with dispatch()
.
import { createStore } from 'lpa-redux';
import rootReducer from './reducers';
const store = createStore(rootReducer);
Practical Implementation
Creating Your First LPA Redux Application
Let’s create a simple todo list application to demonstrate the power of LPA Redux. We’ll go through this step-by-step:
- Set up the actions:
// src/actions/todoActions.js
export const addTodo = (text) => ({
type: 'ADD_TODO',
payload: { text, id: Date.now(), completed: false }
});
export const toggleTodo = (id) => ({
type: 'TOGGLE_TODO',
payload: id
});
- Create the reducer:
// src/reducers/todoReducer.js
import { createReducer } from 'lpa-redux';
const initialState = [];
const todoReducer = createReducer(initialState, {
ADD_TODO: (state, action) => [...state, action.payload],
TOGGLE_TODO: (state, action) => state.map(todo =>
todo.id === action.payload ? { ...todo, completed: !todo.completed } : todo
)
});
export default todoReducer;
- Set up the store:
// src/store/index.js
import { createStore } from 'lpa-redux';
import todoReducer from '../reducers/todoReducer';
const store = createStore(todoReducer);
export default store;
- Create a simple UI to interact with the store:
// src/index.js
import store from './store';
import { addTodo, toggleTodo } from './actions/todoActions';
// UI elements
const todoList = document.getElementById('todo-list');
const todoInput = document.getElementById('todo-input');
const addButton = document.getElementById('add-todo');
// Render function
const render = () => {
const state = store.getState();
todoList.innerHTML = '';
state.forEach(todo => {
const li = document.createElement('li');
li.textContent = todo.text;
li.style.textDecoration = todo.completed ? 'line-through' : 'none';
li.addEventListener('click', () => store.dispatch(toggleTodo(todo.id)));
todoList.appendChild(li);
});
};
// Subscribe to store changes
store.subscribe(render);
// Initial render
render();
// Add todo on button click
addButton.addEventListener('click', () => {
const text = todoInput.value.trim();
if (text) {
store.dispatch(addTodo(text));
todoInput.value = '';
}
});
This basic implementation showcases how LPA Redux simplifies state management while maintaining the core principles of Redux.
Integrating LPA Redux with React
LPA Redux seamlessly integrates with React, providing a powerful state management solution for your React applications. Here’s how you can set up React with LPA Redux:
- Install the necessary packages:
npm install react react-dom lpa-redux lpa-redux-react
- Wrap your React app with the LPA Redux Provider:
// src/index.js
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'lpa-redux-react';
import store from './store';
import App from './App';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
- Connect your components to the store:
// src/components/TodoList.js
import React from 'react';
import { useSelector, useDispatch } from 'lpa-redux-react';
import { toggleTodo } from '../actions/todoActions';
const TodoList = () => {
const todos = useSelector(state => state);
const dispatch = useDispatch();
return (
<ul>
{todos.map(todo => (
<li
key={todo.id}
onClick={() => dispatch(toggleTodo(todo.id))}
style={{ textDecoration: todo.completed ? 'line-through' : 'none' }}
>
{todo.text}
</li>
))}
</ul>
);
};
export default TodoList;
This integration allows you to use LPA Redux’s state management capabilities within your React components efficiently.
Advanced Topics
Middleware in LPA Redux
Middleware in LPA Redux provides a way to extend the store’s capabilities and handle side effects. Here’s an example of a simple logging middleware:
const loggingMiddleware = store => next => action => {
console.log('Dispatching:', action);
let result = next(action);
console.log('Next State:', store.getState());
return result;
};
// Apply middleware when creating the store
const store = createStore(rootReducer, applyMiddleware(loggingMiddleware));
Optimizing Performance with LPA Redux
LPA Redux offers several techniques to optimize performance:
- Use memoized selectors: Implement
createSelector
from thereselect
library to compute derived data efficiently. - Normalize state shape: Keep your state flat and normalize complex data structures to avoid unnecessary re-renders.
- Batch updates: Use
batch
fromlpa-redux-react
to combine multiple dispatches into a single update.
import { batch } from 'lpa-redux-react';
batch(() => {
dispatch(action1());
dispatch(action2());
dispatch(action3());
});
Common Pitfalls and How to Avoid Them
- Mutating state directly: Always return a new state object in your reducers.
- Overusing global state: Not everything needs to be in the Redux store. Use local state for UI-specific data.
- Neglecting performance optimizations: Use memoization and normalization techniques to keep your app performant.
Testing LPA Redux Applications
Testing is crucial for maintaining a robust LPA Redux application. Here’s an example of testing a reducer:
import todoReducer from './todoReducer';
import { addTodo } from './todoActions';
describe('todoReducer', () => {
it('should add a new todo', () => {
const initialState = [];
const action = addTodo('Test todo');
const newState = todoReducer(initialState, action);
expect(newState.length).toBe(1);
expect(newState[0].text).toBe('Test todo');
});
});
FAQ
What makes LPA Redux different from traditional Redux?
LPA Redux simplifies the Redux paradigm by reducing boilerplate, improving TypeScript support, and providing a more intuitive API for common tasks. It maintains the core principles of Redux while offering a more developer-friendly experience.
Can LPA Redux be used with frameworks other than React?
While LPA Redux is often used with React, it’s framework-agnostic and can be integrated with other JavaScript frameworks or vanilla JS applications. The core library is independent of any UI framework.
How do I debug issues in LPA Redux applications?
LPA Redux works well with the Redux DevTools Extension, allowing you to inspect state changes, time-travel debug, and more. Additionally, you can use custom middleware for logging and debugging purposes.
What are some popular alternatives to LPA Redux?
Some alternatives to LPA Redux include:
- MobX
- Recoil
- Zustand
- Jotai
Each has its own strengths and may be more suitable depending on your specific project requirements.
How can I contribute to the LPA Redux community?
You can contribute to LPA Redux by:
- Reporting issues on the GitHub repository
- Submitting pull requests for bug fixes or new features
- Writing documentation or tutorials
- Helping other developers in community forums
- Creating and sharing reusable LPA Redux-based libraries