1. Introduction
React has revolutionized the way we build user interfaces, offering a component-based architecture that promotes reusability and maintainability. At the heart of this flexibility lies a powerful concept: Children Props
. This feature allows developers to create more versatile and reusable components, enhancing the overall structure and efficiency of React applications
.
Definition of Children Props
Children props in React refer to the content passed between the opening and closing tags of a component. This content can be simple text, other React components, or even complex JSX structures.
Importance in React Development
The ability to pass children to components is crucial for creating flexible, reusable UI elements. It allows developers to build wrapper components that can enclose and manipulate their content, leading to more modular and maintainable code.
Overview
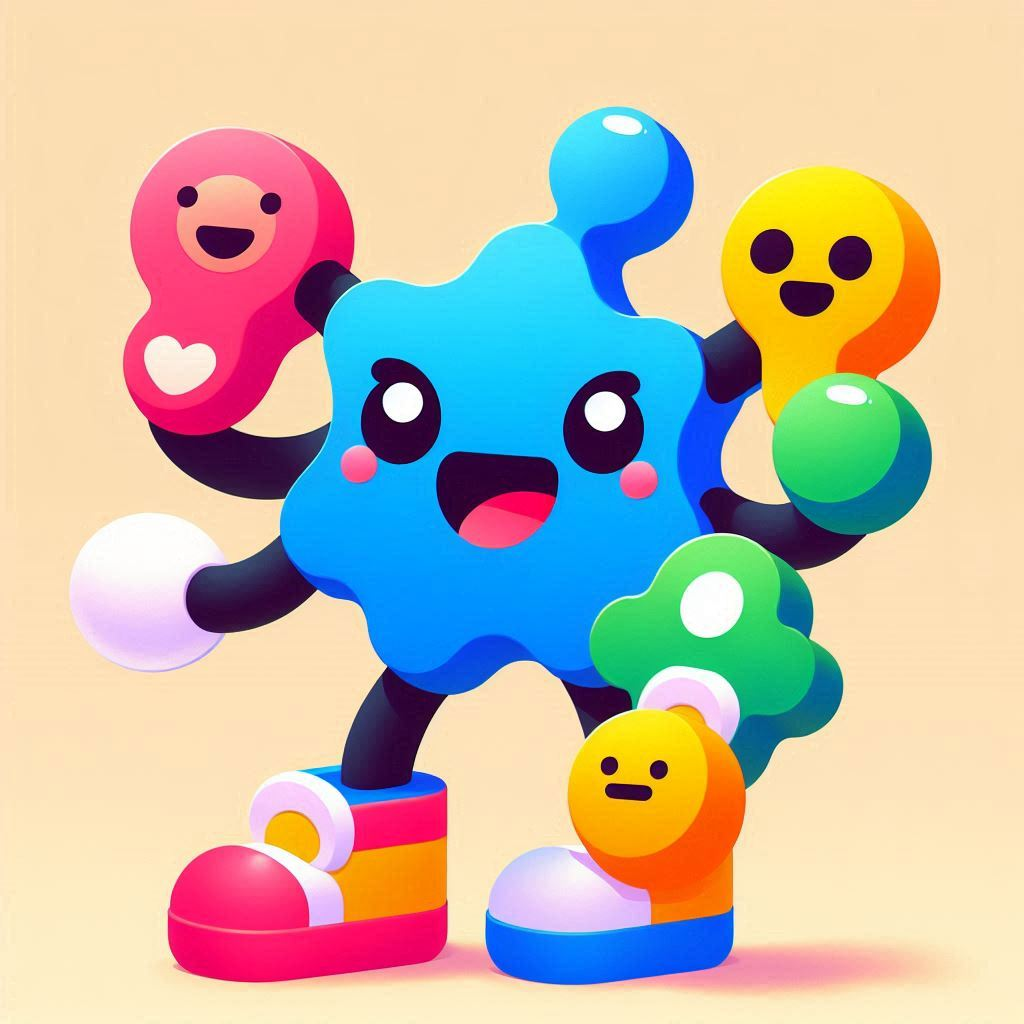
This comprehensive guide will delve into the intricacies of children props
, from basic concepts to advanced techniques. We’ll explore how to implement, optimize, and troubleshoot components using children props, providing real-world examples and best practices along the way.
2. Understanding Children in React
What Are Children Props?
In React, the children prop
is a special prop that is automatically passed to every component. It contains the content between a component’s opening and closing JSX tags.
How Children Props Work
When you use a component in JSX, any content placed between its opening and closing tags becomes the children
prop of that component. This prop can then be accessed and manipulated within the component’s definition.
Benefits of Using Children Props
- Flexibility: Components can accept any content, making them more versatile.
- Reusability: Wrapper components can be created that work with various types of content.
- Composition: Complex UIs can be built by nesting components.
- Separation of Concerns: The parent component doesn’t need to know the details of its children.
3. Basic Implementation of Children Props
Creating a Simple Component with Children Props
Let’s start with a basic example of a component that uses children props:
function Container({ children }) {
return <div className="container">{children}</div>;
}
This Container
component wraps its children in a div with a “container” class.
Passing Children to Components
You can use this component and pass children to it like this:
<Container>
<h1>Welcome</h1>
<p>This is some content inside the container.</p>
</Container>
Example Code Snippets
Here’s a more elaborate example showing how children props can be used to create a flexible layout component:
function Layout({ children, sidebar }) {
return (
<div className="layout">
<div className="main-content">{children}</div>
<div className="sidebar">{sidebar}</div>
</div>
);
}
// Usage
<Layout sidebar={<SidebarContent />}>
<MainPageContent />
</Layout>
This Layout
component accepts both children
and a sidebar
prop, allowing for a flexible two-column layout.
4. Advanced Usage of Children Props
Conditional Rendering with Children Props
Children props can be used in conjunction with conditional rendering to create more dynamic components:
function Alert({ children, type = 'info' }) {
return (
<div className={`alert alert-${type}`}>
{type === 'error' && <strong>Error: </strong>}
{children}
</div>
);
}
// Usage
<Alert type="error">This is an error message.</Alert>
Iterating Over Children
React provides utilities to work with children as a collection. The React.Children
API offers methods like map
and count
:
import React from 'react';
function List({ children }) {
return (
<ul>
{React.Children.map(children, (child, index) => (
<li key={index}>{child}</li>
))}
</ul>
);
}
// Usage
<List>
<span>Item 1</span>
<span>Item 2</span>
<span>Item 3</span>
</List>
Manipulating Children Props
Sometimes, you might need to modify or enhance the children passed to a component. Here’s an example of adding props to child elements:
function WithTooltip({ children, tooltip }) {
return React.Children.map(children, child => {
return React.cloneElement(child, {
title: tooltip
});
});
}
// Usage
<WithTooltip tooltip="This is a tooltip">
<button>Hover me</button>
</WithTooltip>
5. Children Props and Reusability
Creating Reusable Components
Children props are key to creating highly reusable components. Let’s look at a reusable modal component:
function Modal({ isOpen, onClose, children }) {
if (!isOpen) return null;
return (
<div className="modal-overlay">
<div className="modal-content">
<button onClick={onClose}>Close</button>
{children}
</div>
</div>
);
}
// Usage
<Modal isOpen={isModalOpen} onClose={handleCloseModal}>
<h2>Modal Title</h2>
<p>This is the modal content.</p>
</Modal>
Composing Components with Children Props
Children props allow for powerful component composition. Here’s an example of a compound component pattern:
function Tabs({ children }) {
const [activeTab, setActiveTab] = useState(0);
return (
<div className="tabs">
{React.Children.map(children, (child, index) =>
React.cloneElement(child, { isActive: index === activeTab, onClick: () => setActiveTab(index) })
)}
</div>
);
}
function Tab({ children, isActive, onClick }) {
return (
<div className={`tab ${isActive ? 'active' : ''}`} onClick={onClick}>
{children}
</div>
);
}
// Usage
<Tabs>
<Tab>Tab 1 Content</Tab>
<Tab>Tab 2 Content</Tab>
<Tab>Tab 3 Content</Tab>
</Tabs>
Examples of Reusable Patterns
Another common reusable pattern is the render prop pattern, which can be implemented using children props:
function DataFetcher({ children, url }) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
fetch(url)
.then(response => response.json())
.then(data => {
setData(data);
setLoading(false);
});
}, [url]);
return children({ data, loading });
}
// Usage
<DataFetcher url="https://api.example.com/data">
{({ data, loading }) => (
loading ? <p>Loading...</p> : <DisplayData data={data} />
)}
</DataFetcher>
6. Performance Considerations
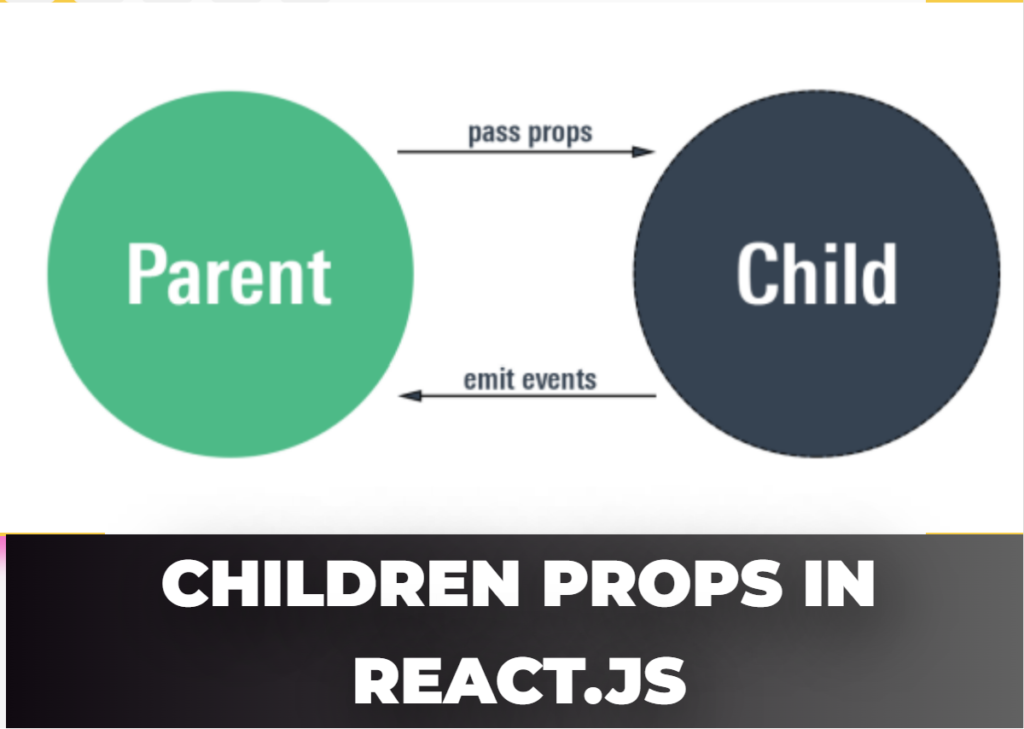
Optimizing Performance with Children Props
When working with children props, it’s important to consider performance, especially when dealing with large component trees or frequent updates.
Avoiding Common Pitfalls
One common pitfall is unnecessary re-rendering. Consider this example:
function Parent() {
const [count, setCount] = useState(0);
return (
<div>
<Button onClick={() => setCount(count + 1)}>Increment</Button>
<ExpensiveComponent>
<span>Count: {count}</span>
</ExpensiveComponent>
</div>
);
}
function ExpensiveComponent({ children }) {
// Some expensive computation here
return <div>{children}</div>;
}
In this case, ExpensiveComponent
will re-render on every count update, even though its own props haven’t changed.
Best Practices for Efficient Rendering
To optimize this, we can use React.memo and/or useMemo:
const ExpensiveComponent = React.memo(({ children }) => {
// Some expensive computation here
return <div>{children}</div>;
});
function Parent() {
const [count, setCount] = useState(0);
const memoizedChildren = useMemo(() => <span>Count: {count}</span>, [count]);
return (
<div>
<Button onClick={() => setCount(count + 1)}>Increment</Button>
<ExpensiveComponent>{memoizedChildren}</ExpensiveComponent>
</div>
);
}
This ensures that ExpensiveComponent
only re-renders when its children actually change.
7. Real-World Examples
Practical Use Cases in Modern Applications
Children props are widely used in real-world applications. Let’s look at a few practical examples:
- Form Components: Creating reusable form layouts and input wrappers.
- Layout Components: Building flexible page layouts and grids.
- Error Boundaries: Implementing error handling and fallback UIs.
Case Studies of Successful Implementations
Many popular React libraries make extensive use of children props. For instance, React Router uses children props to define nested routes:
<Router>
<Route path="/dashboard">
<Dashboard>
<Route path="/dashboard/profile" component={Profile} />
<Route path="/dashboard/settings" component={Settings} />
</Dashboard>
</Route>
</Router>
Detailed Code Walkthroughs
Let’s walk through a more complex example: a customizable data table component.
function DataTable({ children, data }) {
return (
<table>
<thead>
<tr>
{React.Children.map(children, column => (
<th key={column.props.field}>{column.props.header}</th>
))}
</tr>
</thead>
<tbody>
{data.map((row, rowIndex) => (
<tr key={rowIndex}>
{React.Children.map(children, column => (
<td key={column.props.field}>
{column.props.render ? column.props.render(row) : row[column.props.field]}
</td>
))}
</tr>
))}
</tbody>
</table>
);
}
function Column({ field, header, render }) {
// This component doesn't render anything directly
return null;
}
// Usage
<DataTable data={users}>
<Column field="name" header="Name" />
<Column field="email" header="Email" />
<Column
field="status"
header="Status"
render={user => (
<span className={user.status}>{user.status.toUpperCase()}</span>
)}
/>
</DataTable>
This DataTable
component allows for flexible column definitions, including custom rendering for each cell.
8. Testing Components with Children Props
Writing Unit Tests for Components with Children
Testing components that use children props requires special consideration. Here’s an example using Jest and React Testing Library:
import { render, screen } from '@testing-library/react';
test('Container renders children correctly', () => {
render(
<Container>
<h1>Test Heading</h1>
<p>Test paragraph</p>
</Container>
);
expect(screen.getByText('Test Heading')).toBeInTheDocument();
expect(screen.getByText('Test paragraph')).toBeInTheDocument();
});
Tools and Libraries for Testing
Popular tools for testing React components include:
- Jest: A comprehensive testing framework
- React Testing Library: Provides utilities for testing React components
- Enzyme: Offers additional testing utilities, especially for component internals
Example Test Cases
Here’s a more complex test case for our earlier DataTable
component:
test('DataTable renders correct number of rows and columns', () => {
const testData = [
{ id: 1, name: 'John Doe', email: 'john@example.com' },
{ id: 2, name: 'Jane Doe', email: 'jane@example.com' },
];
render(
<DataTable data={testData}>
<Column field="name" header="Name" />
<Column field="email" header="Email" />
</DataTable>
);
expect(screen.getAllByRole('row')).toHaveLength(3); // Header + 2 data rows
expect(screen.getAllByRole('columnheader')).toHaveLength(2);
expect(screen.getByText('John Doe')).toBeInTheDocument();
expect(screen.getByText('jane@example.com')).toBeInTheDocument();
});
9. Troubleshooting and Debugging
Common Issues with Children Props
When working with children props, developers might encounter several common issues:
- Unexpected rendering: When children are not properly typed or validated.
- Performance issues: Due to unnecessary re-renders of complex child components.
- Prop drilling: When passing props through multiple levels of components.
Debugging Tips and Tricks
To debug issues with children props:
- Use React Developer Tools to inspect component props and state.
- Implement logging or debugging statements within your components.
- Use TypeScript to add type checking to your props and catch errors early.
How to Resolve Common Problems
Here’s an example of how to resolve the prop drilling issue using React Context:
const ThemeContext = React.createContext('light');
function ThemedButton({ children }) {
const theme = useContext(ThemeContext);
return <button className={`btn-${theme}`}>{children}</button>;
}
function App() {
return (
<ThemeContext.Provider value="dark">
<Toolbar />
</ThemeContext.Provider>
);
}
function Toolbar() {
return (
<div>
<ThemedButton>Click me</ThemedButton>
</div>
);
}
This approach avoids passing the theme prop through multiple layers of components.
10. Future of Children Props in React
Upcoming Features and Updates
While children props have been a stable feature of React for years, the React team continually works on improvements and new features. Keep an eye on the official React blog and GitHub repository for updates.
How Children Props Fit into React’s Future
As React evolves, children props remain a fundamental concept. They align well with React’s compositional model and are likely to continue playing a crucial role in component design.
Community Contributions and Resources
The React community is vibrant and constantly innovating. Some valuable resources for staying updated include:
- React official documentation
- React community forums and discussion boards
- Open-source React libraries on GitHub
11. Conclusion
Summary of Key Points
Throughout this guide, we’ve explored the power and flexibility of children props in React:
- We’ve seen how they enable the creation of reusable, composable components.
- We’ve delved into advanced usage patterns and performance optimizations.
- We’ve looked at real-world examples and testing strategies.
Final Thoughts on Children Props
Children props are a fundamental feature of React that unlock powerful patterns for component composition and reuse. Mastering their use is crucial for building flexible, maintainable React applications.
Encouraging Further Learning and Exploration
As with any technology, the best way to master children props is through practice. Experiment with different patterns, contribute to open-source projects, and stay curious about new developments in the React ecosystem.
Here’s a set of Frequently Asked Questions (FAQs) with answers related to React’s Children Props:
FAQ
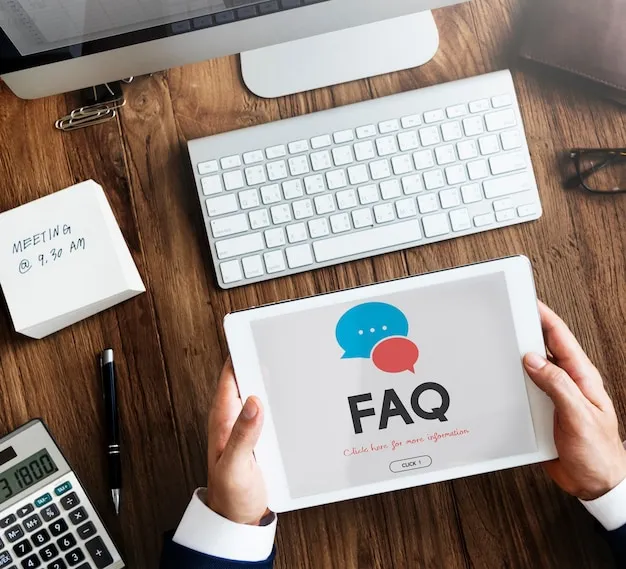
Q1: What are children props in React?
A: Children props in React refer to the content passed between the opening and closing tags of a component. They allow components to receive and render nested elements or components.
Q2: How do I access children props in a React component?
A: You can access children props using the children
property of the props object:
function MyComponent({ children }) {
return <div>{children}</div>;
}
Q3: Can I pass multiple children to a component?
A: Yes, you can pass multiple children to a component. They will be accessible as an array-like object:
<MyComponent>
<p>First child</p>
<p>Second child</p>
</MyComponent>
Q4: How can I modify children props?
A: You can modify children props using React.Children.map()
and React.cloneElement()
:
function ModifyChildren({ children }) {
return React.Children.map(children, child =>
React.cloneElement(child, { className: 'modified' })
);
}
Q5: What’s the difference between props.children and React.Children?
A: props.children
is the content passed to a component, while React.Children
is a utility for working with children
prop, providing methods like map()
, count()
, and toArray()
.
Q6: Can I conditionally render children?
A: Yes, you can conditionally render children:
function ConditionalRender({ children, condition }) {
return condition ? children : null;
}
Q7: How do I type children props with TypeScript?
A: You can type children props in TypeScript like this:
interface Props {
children: React.ReactNode;
}
function MyComponent({ children }: Props) {
return <div>{children}</div>;
}
Q8: Can I pass functions as children?
A: Yes, this is known as the “render prop” pattern:
<DataFetcher>
{(data) => <div>{data.map(item => <p>{item}</p>)}</div>}
</DataFetcher>