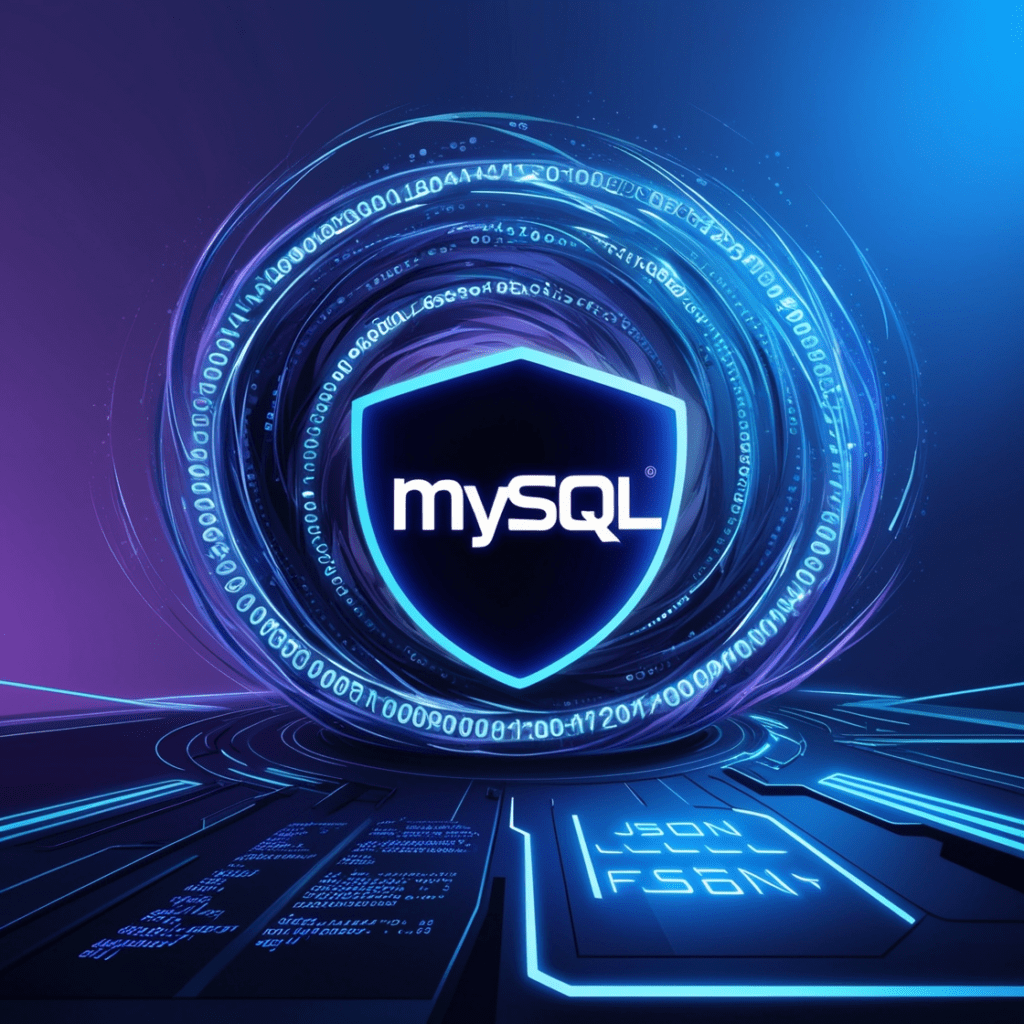
Since MySQL 5.7, the database system has provided robust support for JSON (JavaScript Object Notation) data type and operations. This capability allows developers to store and manipulate semi-structured data directly within MySQL, combining the flexibility of NoSQL databases with the reliability and transaction support of a traditional RDBMS. In this comprehensive guide, we’ll explore how to effectively use MySQL’s JSON functions to handle dynamic data in your applications.
Understanding JSON in MySQL
JSON Data Type Basics
MySQL stores JSON documents in a binary format that enables quick read access to document elements. When you declare a column as JSON, MySQL validates that the values stored are properly formatted JSON documents and automatically converts them to this internal format.
CREATE TABLE products (
id INT PRIMARY KEY,
details JSON
);
INSERT INTO products VALUES (
1,
'{"name": "Smartphone", "brand": "TechCo", "specs": {"color": "black", "storage": 128}}'
);
Advantages of Using JSON in MySQL
- Schema Flexibility: JSON columns can store different sets of fields for different rows
- Structured Querying: MySQL provides functions to query JSON data efficiently
- Type Safety: MySQL validates JSON documents before storage
- Space Efficiency: Binary storage format optimizes space usage
Key JSON Functions in MySQL
JSON_EXTRACT
The JSON_EXTRACT
function is one of the most commonly used JSON functions. It allows you to pull specific values from JSON documents.
SELECT JSON_EXTRACT(details, '$.name') as product_name
FROM products;
-- Alternative syntax using column path operator ->
SELECT details->'$.name' as product_name
FROM products;
JSON_SET, JSON_INSERT, and JSON_REPLACE
These functions allow you to modify JSON documents in different ways:
-- JSON_SET: Sets or replaces values
UPDATE products SET details = JSON_SET(
details,
'$.price', 599.99,
'$.specs.color', 'silver'
)
WHERE id = 1;
-- JSON_INSERT: Only adds values if they don't exist
UPDATE products SET details = JSON_INSERT(
details,
'$.launch_date', '2024-01-15',
'$.price', 599.99 -- Won't override if price exists
);
-- JSON_REPLACE: Only replaces existing values
UPDATE products SET details = JSON_REPLACE(
details,
'$.specs.storage', 256,
'$.nonexistent', 'ignored' -- Won't add new field
);
JSON_REMOVE
Remove elements from a JSON document:
UPDATE products SET details = JSON_REMOVE(
details,
'$.temporary_flag',
'$.specs.deprecated_feature'
);
Working with JSON Arrays
Array Manipulation
MySQL provides several functions specifically for working with JSON arrays:
CREATE TABLE inventory (
id INT PRIMARY KEY,
items JSON
);
INSERT INTO inventory VALUES (
1,
'{"stock": [
{"sku": "ABC123", "quantity": 5},
{"sku": "XYZ789", "quantity": 10}
]}'
);
-- Add to array
UPDATE inventory SET items = JSON_ARRAY_APPEND(
items,
'$.stock',
'{"sku": "NEW456", "quantity": 3}'
);
-- Access array elements
SELECT JSON_EXTRACT(items, '$.stock[0].sku') as first_sku
FROM inventory;
-- Count array elements
SELECT JSON_LENGTH(JSON_EXTRACT(items, '$.stock')) as stock_items
FROM inventory;
Array Searching
-- Find items with specific quantity
SELECT id
FROM inventory
WHERE JSON_CONTAINS(
items,
'{"quantity": 5}',
'$.stock[*]'
);
JSON Table Functions
The JSON_TABLE function transforms JSON data into a relational format, which is particularly useful for reporting and analysis.
SELECT s.*
FROM inventory,
JSON_TABLE(
items->'$.stock[*]',
'$' COLUMNS (
sku VARCHAR(10) PATH '$.sku',
quantity INT PATH '$.quantity'
)
) as s;
Best Practices and Performance Tips
1. Index JSON Fields
Create virtual columns and index them for frequently queried JSON paths:
ALTER TABLE products
ADD COLUMN product_name VARCHAR(100)
GENERATED ALWAYS AS (details->>'$.name') VIRTUAL,
ADD INDEX idx_product_name (product_name);
2. Optimize JSON Document Structure
- Keep JSON documents relatively small
- Avoid deeply nested structures
- Use appropriate data types within JSON
- Consider normalizing frequently queried fields into separate columns
3. Use Appropriate Functions
-- Prefer -> over JSON_EXTRACT for simple paths
SELECT details->'$.name' FROM products;
-- Use ->>, ->, and JSON_UNQUOTE appropriately
SELECT details->>'$.name' as name, -- Unquoted string
details->'$.price' as price -- Preserves JSON type
FROM products;
Real-World Examples
Example 1: Product Catalog with Variants
CREATE TABLE products_catalog (
id INT PRIMARY KEY,
base_info JSON,
variants JSON
);
INSERT INTO products_catalog VALUES (
1,
'{"name": "Classic T-Shirt", "brand": "Fashion Co", "category": "Apparel"}',
'[
{"size": "S", "color": "white", "stock": 100, "price": 19.99},
{"size": "M", "color": "white", "stock": 150, "price": 19.99},
{"size": "L", "color": "white", "stock": 75, "price": 21.99}
]'
);
-- Find available sizes for a specific product
SELECT JSON_EXTRACT(variants, '$[*].size') as available_sizes
FROM products_catalog
WHERE id = 1;
-- Calculate total inventory value
SELECT
id,
JSON_UNQUOTE(base_info->>'$.name') as product_name,
SUM(
CAST(JSON_UNQUOTE(variant->>'$.stock') AS DECIMAL) *
CAST(JSON_UNQUOTE(variant->>'$.price') AS DECIMAL)
) as inventory_value
FROM products_catalog,
JSON_TABLE(
variants,
'$[*]' COLUMNS (
variant JSON PATH '$'
)
) as v
GROUP BY id;
Example 2: User Preferences System
CREATE TABLE user_preferences (
user_id INT PRIMARY KEY,
preferences JSON
);
INSERT INTO user_preferences VALUES (
1,
'{
"theme": "dark",
"notifications": {
"email": true,
"push": false,
"frequency": "daily"
},
"favorites": ["dashboard", "reports", "analytics"]
}'
);
-- Update specific preferences
UPDATE user_preferences
SET preferences = JSON_SET(
preferences,
'$.notifications.push', true,
'$.notifications.frequency', 'weekly'
)
WHERE user_id = 1;
-- Find users with specific preferences
SELECT user_id
FROM user_preferences
WHERE
preferences->>'$.theme' = 'dark'
AND JSON_CONTAINS(
preferences->'$.favorites',
'"dashboard"'
);
Example 3: Event Logging System
CREATE TABLE event_logs (
id INT AUTO_INCREMENT PRIMARY KEY,
timestamp DATETIME DEFAULT CURRENT_TIMESTAMP,
event_data JSON
);
INSERT INTO event_logs (event_data) VALUES (
'{
"type": "user_action",
"action": "login",
"metadata": {
"ip": "192.168.1.1",
"user_agent": "Mozilla/5.0",
"success": true
},
"tags": ["security", "audit"]
}'
);
-- Analysis queries
SELECT
DATE(timestamp) as date,
COUNT(*) as total_events,
COUNT(CASE WHEN event_data->>'$.metadata.success' = 'true' THEN 1 END) as successful_events
FROM event_logs
WHERE
event_data->>'$.type' = 'user_action'
AND event_data->>'$.action' = 'login'
GROUP BY DATE(timestamp);