Welcome to the dynamic world of web development with Node.js! If you’re looking to supercharge the performance of your applications, you’re in the right place. Node.js, known for its efficiency and speed, becomes even more powerful with the use of Express middleware. In this blog, we’ll dive deep into how middleware can enhance your Node.js applications, ensuring they run smoother and faster. Whether you’re a beginner or an experienced developer, the insights shared here will help you optimize your projects in ways you never thought possible. Get ready to transform your application’s performance with some middleware magic!
Understanding Express Middleware
Understanding Express middleware is crucial for any developer looking to create efficient, robust web applications using Node.js. Middleware functions are those functions that have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle. These functions can perform a variety of tasks such as executing code, making changes to the request and the response objects, ending the request-response cycle, and calling the next middleware function in the stack.
What is Express Middleware?
Express middleware are functions that execute during the lifecycle of a request to the Express server. Each middleware has access to the HTTP request and response objects and can modify, add, or manipulate these objects to perform tasks like parsing data, logging, sending a response, or even preventing further execution by sending an error response. Middleware functions are the backbone of an Express application as they allow developers to modularize their code and add powerful features to their application efficiently.
How Does Middleware Work in Node.js?
In Node.js, middleware functions literally “sit in the middle” of the request-response cycle. They intercept incoming requests, perform the necessary operations, and pass the requests along to the next middleware or route handler until a response is sent back to the client. Middleware functions can be configured to execute only under certain conditions, and they can be stacked in multiple layers, allowing for a highly customizable and controlled request-handling process. Here’s a simple way of understanding how middleware works in the context of the Express framework:
1. Request Handling: A middleware function will receive the request object and perform actions like validating user input or parsing body data.
2. Route Handling: Based on the request, middleware can decide to send responses directly or pass control to the next appropriate function.
3. Response Handling: After the request has been fully handled, middleware can modify the response before it’s sent out, like setting headers or compressing the response content.
Understanding and leveraging middleware effectively allows developers to add a powerful layer of processing which helps in preparing the data before it reaches the final route logic or after the primary processing is done.
Benefits of Utilizing Middleware for Catching Performance Optimization
Middleware not only helps in managing the request and response flow inside an application, but it also provides significant benefits in optimizing performance for Node.js applications. Here’s how middleware can practically help in enhancing your application’s effectiveness and efficiency.
Improved Code Execution
Middleware can drastically improve the execution of your code. By delegating specific tasks to middleware, such as error handling, data validation, and session management, you decouple these responsibilities from your main application logic. This means lesser code in your primary application logic and fewer conditions to check during each execution cycle, thus boosting the application’s performance. Middleware allows you to:
- Execute preprocessing or cleanup tasks efficiently.
- Filter out unnecessary data before it hits your route logic.
- Manage async tasks more effectively by using asynchronous middleware.
This management and delegation optimize the request-handling process and save valuable server resources, contributing to quicker response times and improved application performance.
Enhanced Scalability
Using middleware effectively allows your Node.js applications to become more scalable. Middleware can handle tasks such as rate limiting, load balancing, and cache management, which are essential for managing high loads and scaling operations. By offloading these responsibilities to middleware, your application can serve more users without degradation in performance. Consider these benefits:
- Load Balancing: Distribute incoming requests efficiently across multiple instances or servers.
- Rate Limiting: Prevent any single user from overloading the server by making too many requests in a short amount of time.
- Cache Management: Store previously fetched data, so future requests for the same data can be served faster.
Scalability doesn’t just mean handling more users; it also means your application can handle increased complexity more smoothly.
Boosted Overall Performance
Overall application performance can be significantly boosted through optimal use of middleware. Middleware that compresses request and response bodies, minifies files, handles compression, and optimizes images can reduce the load time and increase the speed of your application. Efficient use of middleware cuts down unnecessary processing on the server, reduces latency, and enhances user experience with faster load times. Middleware can:
- Minify CSS, JavaScript, and HTML files to reduce their size and thus, their load times.
- Compress data sent over the network to decrease response times and bandwidth usage.
- Implement efficient logging that doesn’t intrude on the application’s performance.
By harnessing the power of middleware, developers can ensure that their Node.js applications are not only functional but also quick and responsive even under significant loads or complex data requests.
In conclusion, Express middleware plays a crucial role in optimizing the performance of Node.js applications. From improving code execution and enhancing scalability to boosting the overall efficiency, middleware offers a plethora of benefits that can help developers build superfast and scalable web applications. As we dive deeper into specific middleware and techniques to optimize performance, understanding and leveraging these functionalities can transform how your application performs under various conditions.
Practical Tips for Efficient Middleware Implementation
Image courtesy: Unsplash
When it comes to boosting your Node.js application’s performance with Express middleware, implementation strategies can make or break your application’s efficiency. Whether you are new to Express or looking to optimize an existing app, the following tips will help you implement middleware in the most effective way possible.
Prioritizing Middleware Functions
The order in which you stack your middleware functions is crucial for optimal performance and functionality. Middleware functions in Express are executed sequentially, so the sequence can affect both the response time and the outcome of your application. To prioritize correctly:
- Place middleware that terminates the request-response cycle at the top. This includes middleware that serves static files or responses, as they can avoid unnecessary processing.
- Authentication checks should follow, ensuring that resources are not wasted on processing requests from unauthorized users.
- Complex business logic or processor-intensive tasks should be positioned after preliminary checks to avoid unnecessary operations on requests that might be rejected later.
Understanding and planning the order of your middleware stack will drastically enhance the efficiency and speed of your application.
Utilizing Built-in Express Middleware
Express comes equipped with a range of built-in middleware functions that you can leverage straight out of the box to improve performance:
- express.static: Serve static files such as images, CSS files, and JavaScript files efficiently.
- express.json(): This is a built-in middleware function in Express which parses incoming requests with JSON payloads. It streamlines data processing and reduces the need for external dependencies.
- express.urlencoded(): Parses incoming requests with URL-encoded payloads, which is handy for handling form submissions.
These tools are finely tuned for performance and can handle a significant share of functionality without necessitating the overhead of additional dependencies.
Custom Middleware Development
Sometimes, the specific needs of your application will require custom solutions. Developing your own middleware functions offers tailored performance optimizations:
- Focus on minimalism: Write lean middleware functions that perform only necessary tasks to keep the codebase efficient.
- Optimize asynchronous operations: Use asynchronous APIs in your custom middleware to prevent blocking the event loop, especially for I/O operations.
- Reuse logic: If certain functionality is needed across various parts of the application, encapsulate it into middleware to avoid code duplication and reduce errors.
Developing middleware specifically for your application’s needs can significantly boost performance by addressing the unique challenges your application might face.
Real-World Examples of Middleware in Action
Seeing middleware put into practice can provide clarity and inspire your implementation strategies. Here are three common middleware applications that most Node.js using Express projects will benefit from:
Logging Middleware
Logging middleware functions are essential for monitoring and debugging applications. They provide insights into application flow, user behavior, and can help identify performance bottlenecks. Here’s a simple example:
function logRequest(req, res, next) {
console.log(${new Date().toISOString()}
${req.method) request made to ${req.url}\');
Settings next(); } ALTERNATIVE TEXT
app.use(logRequestId);
This function logs the date, request method, and URL accessed, thus, offering a timeline of actions performed in your app which can be crucial for tracing issues when they arise.
Authentication Middleware
Authentication is a security essential, and implementing it as middleware ensures it’s processed before hitting your main business logic, thereby safeguarding sensitive operations. Here’s how you might set it up:
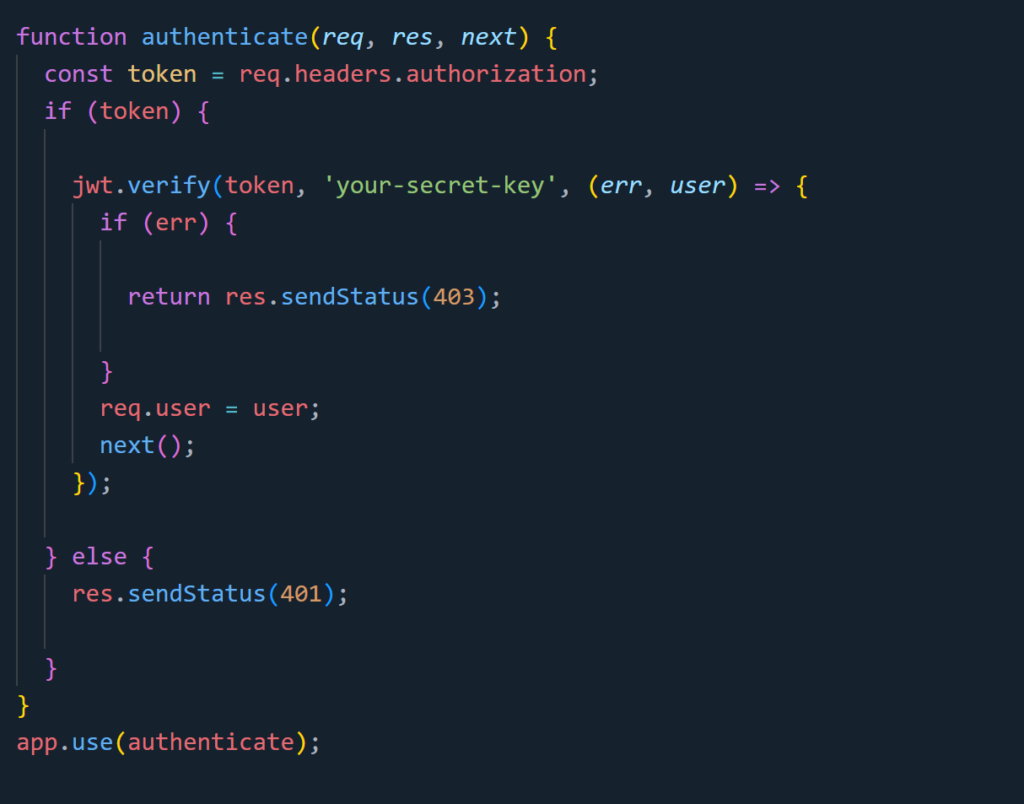
This middleware checks for a JSON Web Token (JWT), verifies it, and either continues with the request or rejects it based on the authentication status. It’s a robust method to protect APIs.
Error Handling Middleware
Proper error handling can save an application from crashing and improve the user’s experience by providing meaningful error responses. Here’s a straightforward way to implement error-handling middleware:
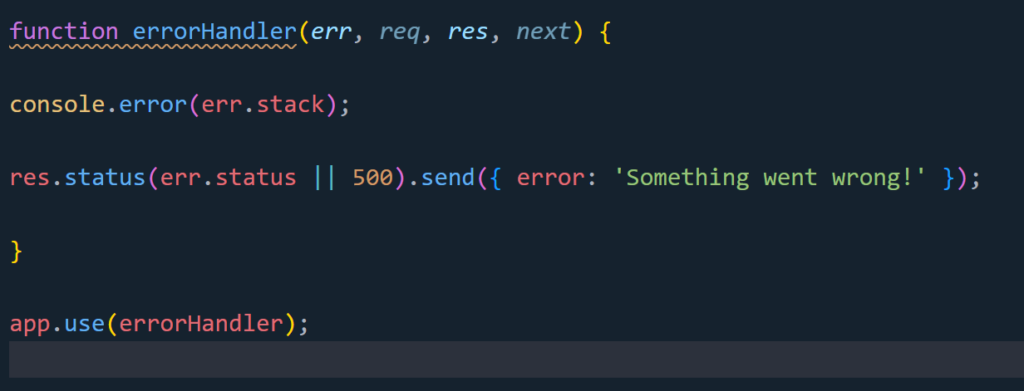
This middleware catches errors thrown anywhere in the stack that haven’t been handled previously and provides a user-friendly error message, instead of letting the application crash.
Through these examples, we can see how middleware not only supports the functionality of your Node.js application but also enhances its reliability and performance. Implementing middleware effectively requires foresight and planning, but with the right approach, the benefits are substantial. Whether you’re serving static files, parsing data, or securing your application, middleware in Express makes it easier and more efficient, paving the way towards a cleaner, faster, and more secure application.
Ensuring your middleware is efficient and effective is key to bolstering your Node.js application’s performance. By adhering to some core best practices, you can dramatically improve how middleware interacts within your Express app. Let’s dive into these transformative methods!
Keeping Middleware Lightweight
When it comes to enhancing the speed and responsiveness of your Node.js applications, the weight of your middleware plays a crucial role. Here are a few pointers to keep your middleware lean and mean:
- Focus on Essentials: Include only necessary functionalities in your middleware. Avoid bloating your middleware with excessive logic or features that could be handled elsewhere.
- Optimize Code: Regularly review and refactor your middleware code. Efficient, well-written code executes faster and consumes less server resources.
- Asynchronous Operations: Utilize asynchronous operations whenever possible. This prevents the blocking of the Node.js event loop, allowing other code to run while waiting on operations that take time, such as API calls or database transactions.
- Use Compression: Implementing compression middleware, like \`compression()\`, helps reduce the size of the request and response objects traveling between the server and client, speeding up transmission times and improving load times.
Keeping each piece of middleware focused on a single task not only improves performance but also makes it easier to maintain and debug your application.
Middleware Chaining for Sequential Execution
Middleware chaining is a powerful feature in Express that allows you to run multiple middleware functions in a specific sequence. This capability can be incredibly beneficial in managing the flow of data and the execution of code. Here are key practices for effective middleware chaining:
- Define Clear Execution Paths: Make sure the order of middleware is intentionally planned so that each function prepares the request object for the next middleware. This ensures a logical and efficient flow of processing.
- Limit Chain Length: While middleware chaining is useful, overly long chains can become difficult to trace and debug. Keep your chains concise.
- Error Handling: Always include error handling in your chains. If one middleware function fails, it should not halt the entire application or create unhandled exceptions.
- Shared Logic Extraction: If several middleware functions use the same piece of logic, consider extracting that logic into a separate function or module. This reduces redundancy and keeps your codebase clean.
Efficient middleware chaining not only streamizes your code execution but also enhances error management and debugging capability.
Error Handling Strategies within Autonomous Middleware
Robust error handling within middleware is vital to maintaining the stability and reliability of your application. Here are some strategies to effectively manage errors within your middleware:
- Use Middleware-specific Try/Catch: Wrap functionalities prone to errors in try/catch blocks specifically within your middleware functions. This localizes error handling and makes your application more resilient to anomalies.
- Leverage Next with Error Objects: When an error occurs in middleware, use the \`next()\` function to pass an error object to the next error handling middleware. This propagates the error properly and allows centralized error processing.
- Logging: Implement logging mechanisms to record errors as they occur. This can help in diagnosing issues post-mortem and aids in monitoring the health of the application.
- Graceful Fallbacks: Design middleware to degrade gracefully when an error occurs. This ensures that the user experience is minimally impacted, and essential functions of your application are maintained.
Implementing these error handling strategies not only protects your application from unexpected failures but also helps in maintaining a smooth user experience by dealing with problems swiftly and efficiently.
Conclusion
After diving deep into the world of Express middleware, it’s clear that these tools are not just helpers but crucial elements in enhancing the performance of your Node.js applications. By strategically implementing middleware functions, you can manage requests efficiently, streamline data processing, and significantly boost your application’s speed and reliability. Remember, the key to successful middleware management is in its thoughtful arrangement and selective deployment:
– Prioritize Essential Middleware: Only use those that are pivotal for your app’s operations.
– Monitor Performance: Regularly check how middleware affects load times and overall performance.
– Customize When Necessary: Don’t hesitate to write custom middleware if existing solutions don’t meet your specific needs.
In conclusion, middleware in Express isn’t just about adding functionality; it’s about enhancing performance in ways that are both measurable and meaningful. Incorporate these tools wisely, and you’ll see a notable improvement in your app’s efficiency and user satisfaction. Embrace middleware, the unsung hero of web development, and push your Node.js projects to new heights!
Pingback: Production Environment Essentials: From Setup to Security
Pingback: Authentication with Passport.js: A Guide to PassportJS Integration