Table of Contents
Introduction
In the vast landscape of web development, understanding and implementing various HTTP methods is crucial for creating robust and efficient applications. Among these methods, POST routes stand out as a fundamental component for handling data submission and server-side processing. This blog post will delve deep into the world of POST routes, exploring their importance, implementation, and best practices.
“POST is the Swiss Army knife of HTTP methods. It’s versatile, powerful, and essential for any web developer’s toolkit.” – Anonymous Web Developer
Understanding HTTP Methods
Before we dive into the specifics of POST routes, let’s take a moment to understand the broader context of HTTP methods.
Brief Introduction to HTTP Methods
HTTP (Hypertext Transfer Protocol) defines a set of request methods to indicate the desired action to be performed for a given resource. The most commonly used HTTP methods are:
- GET
- POST
- PUT
- DELETE
- PATCH
Differences Between GET, POST, PUT, DELETE, etc.
Each HTTP method serves a specific purpose:
- GET: Retrieves data from the server
- POST: Submits data to be processed by the server
- PUT: Updates an existing resource on the server
- DELETE: Removes a specific resource from the server
- PATCH: Partially modifies an existing resource
When to Use POST Routes
POST routes are particularly useful when:
- Submitting form data
- Creating new resources on the server
- Sending sensitive information (as POST data is not visible in the URL)
- Uploading files
- Performing actions that change the server’s state
Setting Up Your Development Environment
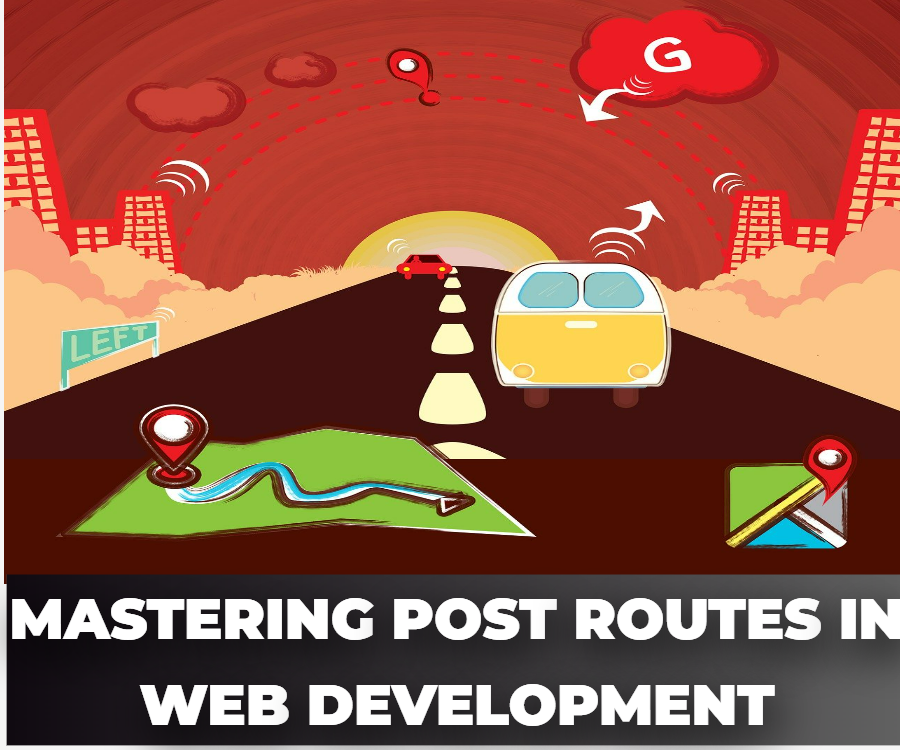
To get started with implementing POST routes, you’ll need to set up your development environment.
Required Tools and Software
- Node.js
- npm (Node Package Manager)
- A text editor or IDE (e.g., Visual Studio Code, Sublime Text)
- Postman (for testing API endpoints)
Installing Node.js and Express
First, download and install Node.js from the official website (https://nodejs.org). Once installed, you can use npm to install Express, a popular web application framework for Node.js.
Open your terminal and run:
npm install express
Initializing a New Project
Create a new directory for your project and initialize it with npm:
mkdir post-routes-demo
cd post-routes-demo
npm init -y
This will create a package.json
file in your project directory.
Basic Implementation of POST Routes
Now that we have our environment set up, let’s create a simple POST route using Express.js.
Creating a Simple POST Route with Express.js
Create a new file called app.js
in your project directory and add the following code:
const express = require('express');
const app = express();
const port = 3000;
// Middleware to parse JSON bodies
app.use(express.json());
// Middleware to parse URL-encoded bodies
app.use(express.urlencoded({ extended: true }));
// Define a POST route
app.post('/submit-form', (req, res) => {
const { name, email } = req.body;
res.send(`Form submitted successfully! Name: ${name}, Email: ${email}`);
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
Handling Form Data
In the example above, we’ve created a POST route that handles form submissions. The express.json()
and express.urlencoded()
middleware allow us to parse JSON and URL-encoded data from the request body.
To test this route, you can use Postman or create a simple HTML form:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Form Submission</title>
</head>
<body>
<form action="http://localhost:3000/submit-form" method="POST">
<input type="text" name="name" placeholder="Your Name" required>
<input type="email" name="email" placeholder="Your Email" required>
<button type="submit">Submit</button>
</form>
</body>
</html>
Advanced Usage of POST Routes
As you become more comfortable with basic POST routes, you can explore more advanced usage scenarios.
Validating Data in POST Requests
Data validation is crucial for maintaining the integrity of your application. Here’s an example of how you can validate incoming data using a library like joi
:
const Joi = require('joi');
app.post('/register', (req, res) => {
const schema = Joi.object({
username: Joi.string().alphanum().min(3).max(30).required(),
email: Joi.string().email().required(),
password: Joi.string().pattern(new RegExp('^[a-zA-Z0-9]{3,30}$')).required()
});
const { error } = schema.validate(req.body);
if (error) {
return res.status(400).send(error.details[0].message);
}
// Process the registration...
res.send('User registered successfully');
});
Handling JSON and URL-Encoded Data
Express.js makes it easy to handle different types of data. We’ve already seen how to use express.json()
and express.urlencoded()
middleware. Here’s an example of how to handle both types in a single route:
app.post('/api/data', (req, res) => {
if (req.is('json')) {
// Handle JSON data
const { name, age } = req.body;
res.json({ message: `Received JSON data for ${name}, age ${age}` });
} else if (req.is('urlencoded')) {
// Handle URL-encoded data
const { title, description } = req.body;
res.json({ message: `Received form data: ${title} - ${description}` });
} else {
res.status(415).send('Unsupported Media Type');
}
});
Security Considerations
Security should always be a top priority when working with POST routes.
Securing POST Routes with Middleware
One way to add an extra layer of security is by using middleware to authenticate requests:
const authenticateUser = (req, res, next) => {
const authToken = req.headers['authorization'];
if (authToken === 'secret-token') {
next();
} else {
res.status(401).send('Unauthorized');
}
};
app.post('/secure-endpoint', authenticateUser, (req, res) => {
res.send('Access granted to secure endpoint');
});
Preventing Cross-Site Request Forgery (CSRF)
CSRF attacks can be mitigated using tokens. Here’s a simple example using the csurf
package:
const csrf = require('csurf');
const csrfProtection = csrf({ cookie: true });
app.use(cookieParser());
app.get('/form', csrfProtection, (req, res) => {
res.render('form', { csrfToken: req.csrfToken() });
});
app.post('/process', csrfProtection, (req, res) => {
res.send('Data is being processed');
});
Sanitizing User Input
Always sanitize user input to prevent XSS attacks. You can use libraries like xss
to clean user input:
const xss = require('xss');
app.post('/comment', (req, res) => {
const cleanComment = xss(req.body.comment);
// Store the cleaned comment in the database
res.send('Comment posted successfully');
});
Working with Databases
POST routes are often used to insert or update data in a database.
Connecting to a Database
Here’s an example of connecting to a MongoDB database using Mongoose:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/myapp', {
useNewUrlParser: true,
useUnifiedTopology: true
});
const db = mongoose.connection;
db.on('error', console.error.bind(console, 'connection error:'));
db.once('open', function() {
console.log('Connected to MongoDB');
});
Inserting Data via POST Requests
Once connected, you can create a schema and model to insert data:
const userSchema = new mongoose.Schema({
name: String,
email: String,
age: Number
});
const User = mongoose.model('User', userSchema);
app.post('/users', async (req, res) => {
try {
const newUser = new User(req.body);
await newUser.save();
res.status(201).send(newUser);
} catch (error) {
res.status(400).send(error);
}
});
Testing POST Routes
Testing is crucial for ensuring the reliability of your POST routes.
Writing Unit Tests for POST Routes
Here’s an example of how to write a unit test for a POST route using Jest and Supertest:
const request = require('supertest');
const app = require('../app'); // Your Express app
describe('POST /users', () => {
it('should create a new user', async () => {
const res = await request(app)
.post('/users')
.send({
name: 'John Doe',
email: 'john@example.com',
age: 30
});
expect(res.statusCode).toEqual(201);
expect(res.body).toHaveProperty('_id');
expect(res.body.name).toEqual('John Doe');
});
});
Using Tools Like Postman and Jest
Postman is excellent for manual testing and creating test suites for your API. You can create collections of requests and run them as part of your CI/CD pipeline.
Jest, on the other hand, is perfect for automated unit and integration testing. It provides a rich set of matchers and mocking capabilities.
Troubleshooting Common Issues
Even experienced developers encounter issues with POST routes from time to time.
Debugging POST Route Errors
Common errors include:
- 404 Not Found: Check your route definition and URL
- 400 Bad Request: Verify the request body format
- 500 Internal Server Error: Check your server-side code for exceptions
Use logging statements and tools like Morgan to help identify where errors are occurring:
const morgan = require('morgan');
app.use(morgan('dev'));
Tips for Efficient Troubleshooting
- Use meaningful error messages
- Implement proper error handling middleware
- Use debugging tools like Node.js debugger or VS Code’s built-in debugger
- Monitor your application logs
Real-World Examples
Let’s look at some real-world applications of POST routes.
Use Case: User Authentication
app.post('/login', async (req, res) => {
const { username, password } = req.body;
try {
const user = await User.findOne({ username });
if (!user) {
return res.status(401).send('Invalid username or password');
}
const isMatch = await bcrypt.compare(password, user.password);
if (!isMatch) {
return res.status(401).send('Invalid username or password');
}
const token = jwt.sign({ id: user._id }, 'your-secret-key', { expiresIn: '1h' });
res.json({ token });
} catch (error) {
res.status(500).send('Server error');
}
});
Use Case: File Upload
const multer = require('multer');
const upload = multer({ dest: 'uploads/' });
app.post('/upload', upload.single('file'), (req, res) => {
if (!req.file) {
return res.status(400).send('No file uploaded');
}
res.send('File uploaded successfully');
});
Future Trends in POST Routes
As web development continues to evolve, so do the ways we use POST routes.
Upcoming Features and Updates
- Increased use of GraphQL mutations as an alternative to traditional REST POST endpoints
- Enhanced security features in popular frameworks
- Better integration with serverless architectures
How POST Routes Fit into the Future of Web Development
While new technologies emerge, POST routes remain a fundamental part of web development. They continue to be essential for handling form submissions, API interactions, and data processing in modern web applications.
Conclusion
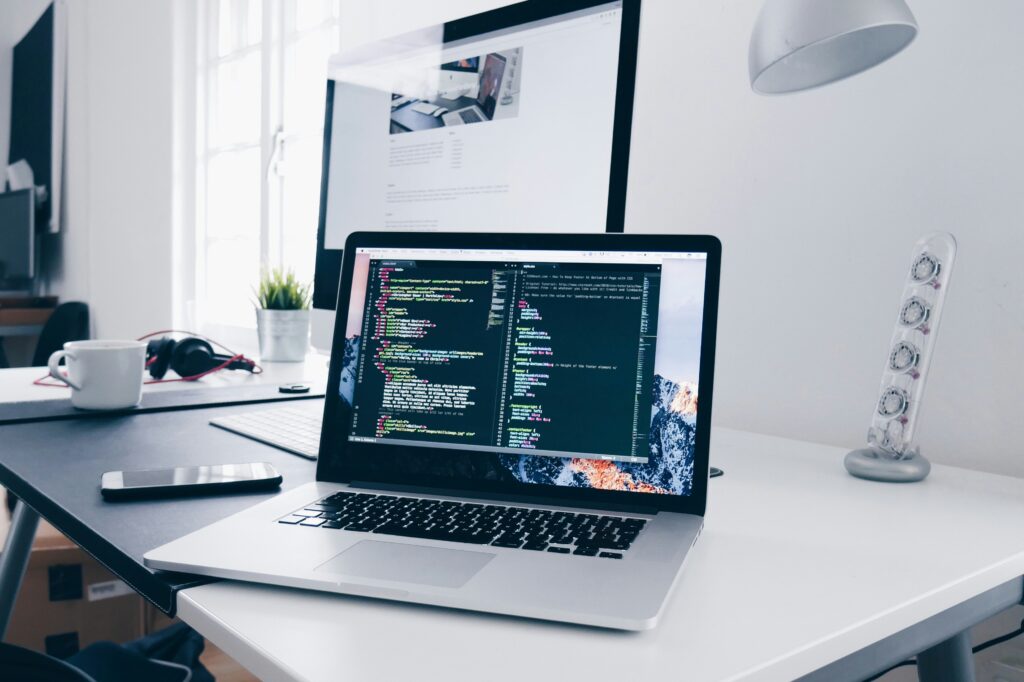
POST routes are a crucial component of web development, enabling secure data submission and server-side processing. By mastering POST routes, you’ll be well-equipped to build robust, efficient, and secure web applications.
Remember these key points:
- Use POST routes for submitting data and changing server state
- Always validate and sanitize user input
- Implement proper error handling and security measures
- Test your routes thoroughly
- Stay updated with the latest trends and best practices
As you continue your journey in web development, keep exploring new ways to leverage POST routes and other HTTP methods to create powerful, user-friendly applications.
“The art of programming is the art of organizing complexity, of mastering multitude and avoiding its bastard chaos as effectively as possible.” – Edsger W. Dijkstra