Table of Contents
In the ever-evolving landscape of web development, creating engaging and responsive email templates has become crucial for effective communication with users. Email 3.0 emerges as a game-changing solution, offering developers a powerful toolkit to craft stunning, dynamic, and highly compatible email templates. This comprehensive guide will walk you through the ins and outs of React Email 3.0, from setup to advanced features, ensuring you’re equipped to leverage its full potential.
1. Introduction
Overview of React Email 3.0
Email 3.0 is the latest iteration of a popular library that brings the power and flexibility of React to email template development. It allows developers to create reusable, component-based email templates that are both easy to maintain and highly customizable.
What is React Email 3.0?
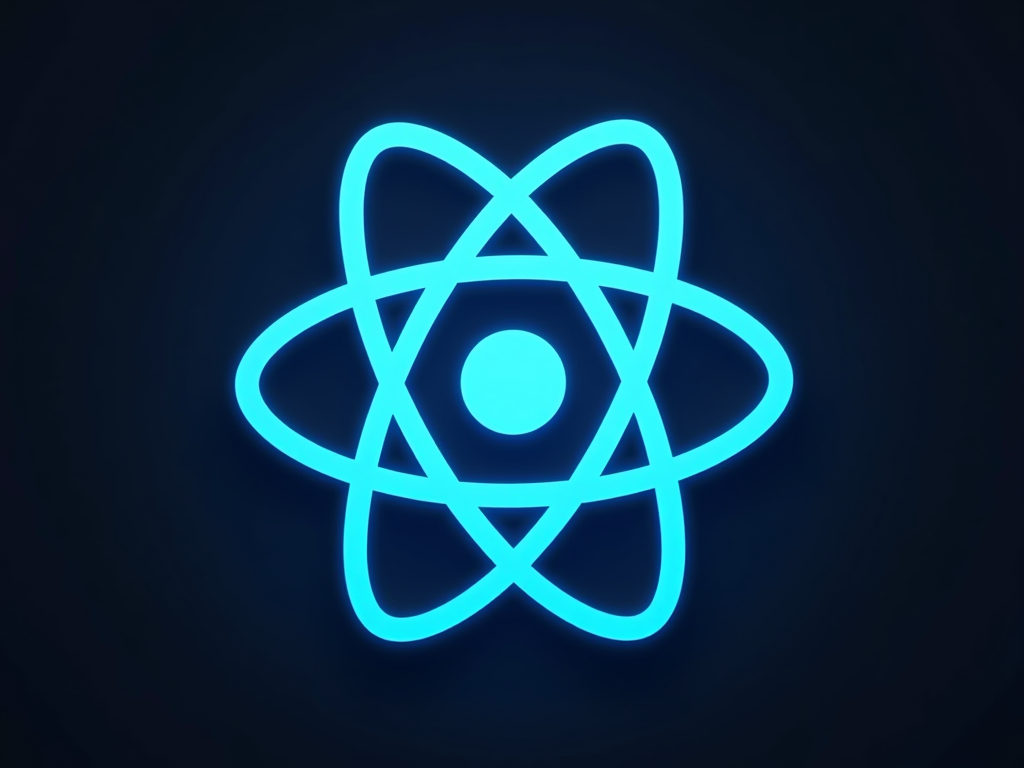
At its core, React Email 3.0 is a framework that enables developers to build email templates using React components. It provides a set of pre-built, email-friendly components and utilities that streamline the process of creating complex, responsive email designs.
Importance of Email Templates in Modern Web Development
In today’s digital age, email remains a critical channel for communication and marketing. Well-designed email templates can:
- Enhance user engagement
- Improve brand consistency
- Increase conversion rates
- Provide a seamless user experience across devices
Why Choose React Email 3.0?
Email 3.0 offers several compelling reasons for adoption:
- Component-based architecture: Reusable components lead to more efficient development and easier maintenance.
- React ecosystem: Leverage the vast React ecosystem and developer tools.
- Improved performance: Optimized rendering and reduced template size.
- Enhanced compatibility: Better support for various email clients.
Key Features and Improvements in Version 3.0
React Email 3.0 introduces several enhancements over its predecessors:
- Improved rendering engine: Faster and more accurate email rendering.
- Enhanced responsive design tools: Easier creation of mobile-friendly emails.
- Extended component library: More pre-built components for common email elements.
- Better TypeScript support: Improved type definitions for enhanced developer experience.
Comparison with Previous Versions
Compared to earlier versions, React Email 3.0 offers:
- Faster rendering times
- Reduced bundle size
- Improved cross-client compatibility
- More intuitive API for component customization
2. Setting Up Email 3.0
Installation and Configuration
Prerequisites
Before diving into React Email 3.0, ensure you have:
- Node.js (version 12 or higher)
- npm or yarn package manager
- Basic knowledge of React and JSX
Step-by-step Installation Guide
To get started with React Email 3.0, follow these steps:
- Create a new React project or navigate to your existing project directory.
- Open your terminal and run:
npm install react-email@3.0.0
or if you’re using yarn:
yarn add react-email@3.0.0
- Once installed, you can import React Email components in your project:
import { Email, Header, Body } from 'react-email';
Basic Project Setup
Creating a New React Project
If you’re starting from scratch, create a new React project:
npx create-react-app my-email-project
cd my-email-project
Integrating React Email 3.0
After installation, you can start using React Email 3.0 in your components:
import React from 'react';
import { Email, Header, Body, Footer } from 'react-email';
function MyEmailTemplate() {
return (
<Email>
<Header>Welcome to React Email 3.0</Header>
<Body>
<p>This is a simple email template built with React Email 3.0</p>
</Body>
<Footer>ยฉ 2024 Your Company</Footer>
</Email>
);
}
export default MyEmailTemplate;
3. Building Your First Email Template
Creating a Simple Email Template
Understanding the Email Template Structure
A typical email template in React Email 3.0 consists of several key components:
<Email>
: The root component that wraps the entire email content.<Header>
: Contains the email header information.<Body>
: The main content of your email.<Footer>
: Optional footer section for additional information.
Writing Reusable Components for Email
One of the strengths of React Email 3.0 is the ability to create reusable components. For example:
function ButtonComponent({ text, url }) {
return (
<a href={url} style={{
padding: '10px 20px',
backgroundColor: '#007bff',
color: 'white',
textDecoration: 'none',
borderRadius: '5px'
}}>
{text}
</a>
);
}
You can then use this component within your email templates:
<Body>
<p>Click the button below to get started:</p>
<ButtonComponent text="Get Started" url="https://example.com" />
</Body>
Styling Emails with Inline CSS
Best Practices for Inline CSS in Email Templates
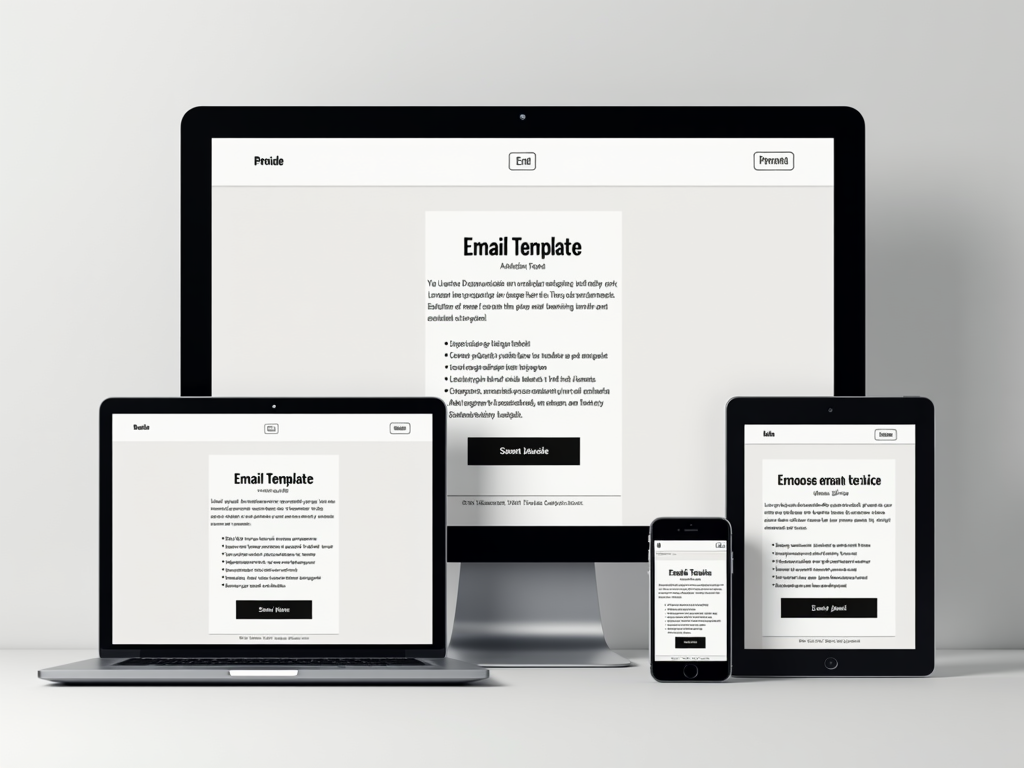
When styling email templates, inline CSS is often necessary for compatibility across email clients. React Email 3.0 makes this process easier:
<p style={{
fontSize: '16px',
color: '#333',
lineHeight: '1.5'
}}>
Welcome to our newsletter!
</p>
Tools and Tips for Maintaining Consistent Styles
- Use CSS-in-JS libraries like styled-components for more manageable styles.
- Create a style guide or theme object to maintain consistency across your email templates.
- Utilize React Email 3.0’s built-in styling props for common elements.
4. Advanced Features in React Email 3.0
Dynamic Content in Emails
Injecting Dynamic Data into Email Templates
React Email 3.0 excels at incorporating dynamic content. Here’s an example:
function DynamicEmailTemplate({ userName, products }) {
return (
<Email>
<Header>Welcome, {userName}!</Header>
<Body>
<h2>Your Recommended Products:</h2>
<ul>
{products.map(product => (
<li key={product.id}>{product.name} - ${product.price}</li>
))}
</ul>
</Body>
</Email>
);
}
Personalizing Emails for Recipients
Personalization can significantly improve engagement. Use props or context to pass user-specific data:
<DynamicEmailTemplate
userName={user.name}
products={user.recommendedProducts}
/>
Responsive Email Design
Techniques for Making Emails Mobile-friendly
React Email 3.0 provides tools to create responsive designs:
- Use percentage-based widths for flexible layouts.
- Implement stackable columns for mobile devices.
- Adjust font sizes and spacing for smaller screens.
Media Queries and Responsive Components
While not all email clients support media queries, you can use them for progressive enhancement:
<style>
{`
@media only screen and (max-width: 600px) {
.mobile-header {
font-size: 20px !important;
}
}
`}
</style>
Optimizing for Email Clients
Compatibility Across Different Email Clients
React Email 3.0 aims to provide better cross-client compatibility. However, always test your emails in various clients to ensure consistent rendering.
Testing Your Templates with Popular Email Clients
Use services like Litmus or Email on Acid to test your templates across multiple email clients and devices.
5. Integrating React Email 3.0 with Email Services
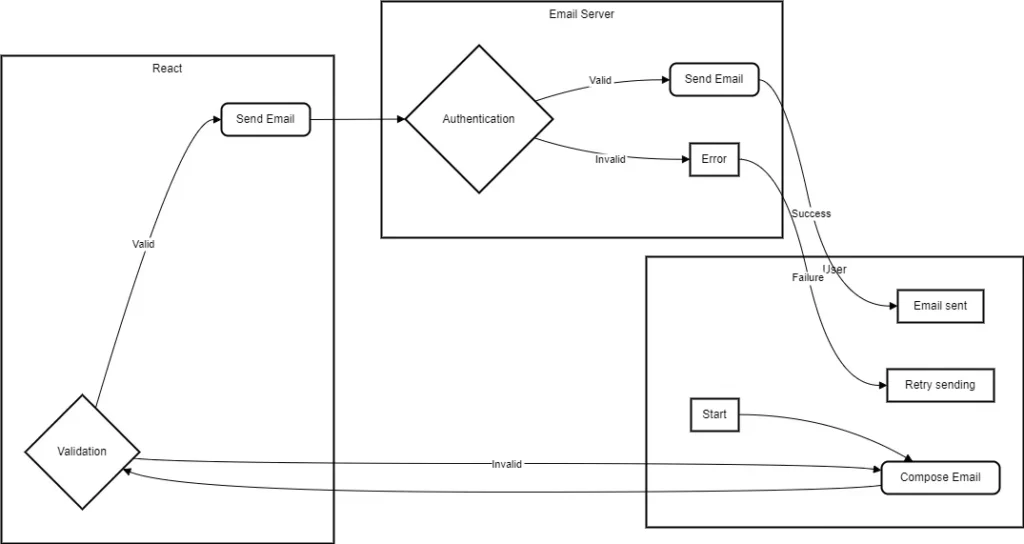
Sending Emails with Node.js
Setting up Nodemailer for Email Delivery
Nodemailer is a popular choice for sending emails from Node.js applications. Here’s a basic setup:
const nodemailer = require('nodemailer');
const { renderToString } = require('react-dom/server');
const MyEmailTemplate = require('./MyEmailTemplate');
async function sendEmail() {
let transporter = nodemailer.createTransport({
host: "smtp.example.com",
port: 587,
secure: false,
auth: {
user: "your_username",
pass: "your_password",
},
});
let info = await transporter.sendMail({
from: '"Your Name" <from@example.com>',
to: "recipient@example.com",
subject: "Hello from React Email 3.0",
html: renderToString(<MyEmailTemplate />),
});
console.log("Message sent: %s", info.messageId);
}
sendEmail().catch(console.error);
Connecting Your Template with Email Services
Popular email services like SendGrid, Mailchimp, or Amazon SES can be integrated similarly. Most provide Node.js SDKs for easy integration.
Handling Email Deliverability
Ensuring High Deliverability Rates
To maintain high deliverability:
- Use authentication protocols like SPF, DKIM, and DMARC.
- Regularly clean your email list.
- Monitor your sender reputation.
Managing Bounces and Spam Filters
Implement bounce handling and regularly update your email list to remove invalid addresses. Use spam testing tools to ensure your content doesn’t trigger spam filters.
6. Best Practices and Tips for React Email 3.0
Performance Optimization
Reducing Template Size for Faster Load Times
- Minimize the use of large images.
- Utilize React Email 3.0’s built-in optimization features.
- Compress assets before including them in your email.
Managing Images and Assets in Emails
- Host images on a CDN for faster loading.
- Use appropriate image formats (JPEG for photos, PNG for graphics with transparency).
- Implement lazy loading for images when possible.
Security Considerations
Avoiding Common Vulnerabilities in Email Templates
- Sanitize user input to prevent XSS attacks.
- Avoid including sensitive information directly in emails.
- Use HTTPS links for all external resources.
Protecting Sensitive Data
- Never include passwords or sensitive tokens in email content.
- Use secure methods for sharing confidential information.
7. Common Pitfalls and Troubleshooting
Debugging Email Templates
Tools and Methods for Identifying and Fixing Issues
- Use browser developer tools to inspect your email structure.
- Implement error boundaries in your React components to catch and handle errors gracefully.
- Utilize React Email 3.0’s debugging features for detailed logging.
Overcoming Common Challenges
Handling Inconsistent Rendering Across Email Clients
- Start with a mobile-first approach and progressively enhance for desktop.
- Use table-based layouts for maximum compatibility.
- Test extensively across different email clients and devices.
8. Case Study: A Real-World Application of React Email 3.0
Example Project Breakdown
Let’s consider a hypothetical e-commerce platform implementing a personalized email campaign using React Email 3.0.
import React from 'react';
import { Email, Header, Body, Footer, Button } from 'react-email';
function PersonalizedCampaignEmail({ user, recentProducts }) {
return (
<Email>
<Header>
<h1 style={{ color: '#333', textAlign: 'center' }}>
Welcome back, {user.firstName}!
</h1>
</Header>
<Body>
<p>We've got some great recommendations based on your recent browsing:</p>
<table style={{ width: '100%' }}>
<tbody>
{recentProducts.map(product => (
<tr key={product.id}>
<td>
<img src={product.imageUrl} alt={product.name} style={{ width: '100px' }} />
</td>
<td style={{ paddingLeft: '20px' }}>
<h3>{product.name}</h3>
<p>{product.description}</p>
<Button href={`https://example.com/product/${product.id}`}>
View Product
</Button>
</td>
</tr>
))}
</tbody>
</table>
</Body>
<Footer>
<p>
You're receiving this email because you're a valued customer of Example Store.
<a href="https://example.com/unsubscribe">Unsubscribe</a>
</p>
</Footer>
</Email>
);
}
Lessons Learned and Best Practices
From this case study, we can derive several best practices:
- Personalization: Utilize user data to create tailored experiences.
- Responsive Design: Ensure the email looks good on both desktop and mobile.
- Clear CTAs: Use prominent, actionable buttons for key actions.
- Balanced Content: Mix text and images for better engagement and deliverability.
- Legal Compliance: Include unsubscribe options and explain why the user is receiving the email.
9. Conclusion
Recap of Key Takeaways
React Email 3.0 offers a powerful, flexible solution for creating dynamic and responsive email templates. Key benefits include:
- Component-based architecture for reusable, maintainable code
- Improved performance and rendering across email clients
- Advanced features for personalization and dynamic content
- Seamless integration with popular email services and Node.js
Future of React Email
As email continues to evolve as a critical communication channel, we can expect React Email to grow in capabilities and adoption. Potential future developments might include:
- Enhanced AI-driven personalization features
- Improved interactivity within email clients that support it
- Better integration with design tools and visual builders
- Continued focus on accessibility and inclusive design
React Email 3.0 represents a significant step forward in email template development, offering developers the tools they need to create engaging, responsive, and highly personalized email experiences.
FAQ Section
What is React Email 3.0 and how is it different from previous versions?
React Email 3.0 is the latest version of the popular email template development library for React. It offers several improvements over previous versions:
- Enhanced rendering engine for faster and more accurate email display
- Expanded component library with more pre-built email elements
- Improved responsive design tools for better mobile compatibility
- Optimized performance with reduced bundle size
- Better TypeScript support for improved developer experience
Can I use React Email 3.0 with any email service provider?
Yes, React Email 3.0 is designed to be compatible with most major email service providers. It generates standard HTML output that can be used with services like SendGrid, Mailchimp, Amazon SES, and others. However, always test your templates with your specific provider to ensure optimal compatibility.
How do I ensure my React Email templates are responsive on mobile devices?
To create responsive email templates with React Email 3.0:
- Use percentage-based widths for flexible layouts
- Implement stackable columns for mobile views
- Adjust font sizes and spacing for smaller screens
- Utilize React Email 3.0’s built-in responsive components
- Test extensively on various devices and email clients
What are some common challenges when building email templates with React Email 3.0?
Common challenges include:
- Ensuring consistent rendering across different email clients
- Balancing design aesthetics with email client limitations
- Managing dynamic content while maintaining performance
- Optimizing images and assets for quick loading
- Implementing complex layouts that work across devices
Is React Email 3.0 SEO-friendly?
While email templates themselves don’t directly impact website SEO, well-designed emails can indirectly benefit your SEO efforts by:
- Increasing engagement and click-through rates to your website
- Improving brand recognition and recall
- Driving traffic to your site, potentially increasing time on site and reducing bounce rates
To optimize your email content for better engagement:
- Use clear, compelling subject lines
- Include relevant, valuable content that encourages clicks
- Ensure all links in your emails are valid and lead to relevant pages
- Use alt text for images to improve accessibility and provide context
Remember, the primary goal of email templates is to engage your audience and drive action, which can indirectly support your overall digital marketing and SEO strategy.