- Brief Overview of Web Rendering
- The Challenge of Efficient DOM Updates
- React's Clever Solution: Virtual DOM and Reconciliation
- Why This Matters
- Real-World Example
- Understanding the DOM and Reconciliation
- 2.2 Virtual DOM
- 3. Reconciliation in React
- 3.1 What is Reconciliation?
- 3.2 The Reconciliation Process
- Understanding React's Diffing Algorithm and Optimization
- 4. The Diffing Algorithm
- 5. Diffing Process Deep Dive
- 5.1 Tree Diffing
- 5.2 Element Diffing
- 5.3 Component Diffing
- 6. Optimization Techniques
- 6.1 Keys in Lists
- 7. Conclusion
- Future of React Rendering
Brief Overview of Web Rendering
Think of a web page like a tree of boxes. Every element you see – buttons, text, images – is a box in this tree. When you’re browsing websites, your browser creates something called the Document Object Model (DOM), which is basically a map of all these boxes and their relationships.
When something changes on your page (like when someone likes your post), the browser needs to:
- Figure out what changed
- Update the screen to show these changes
- Make sure everything else stays in place
It sounds simple, but here’s where things get interestingโฆ
The Challenge of Efficient DOM Updates
Let’s say you have a list of 100 items, and you just want to change one small thing – like updating a like count. Without any special handling, traditional web development might require the browser to:
- Check the entire list
- Rebuild parts of it
- Re-render everything
- Even update things that haven’t changed!
This is like rebuilding an entire house just to change a light bulb.
React’s Clever Solution: Virtual DOM and Reconciliation
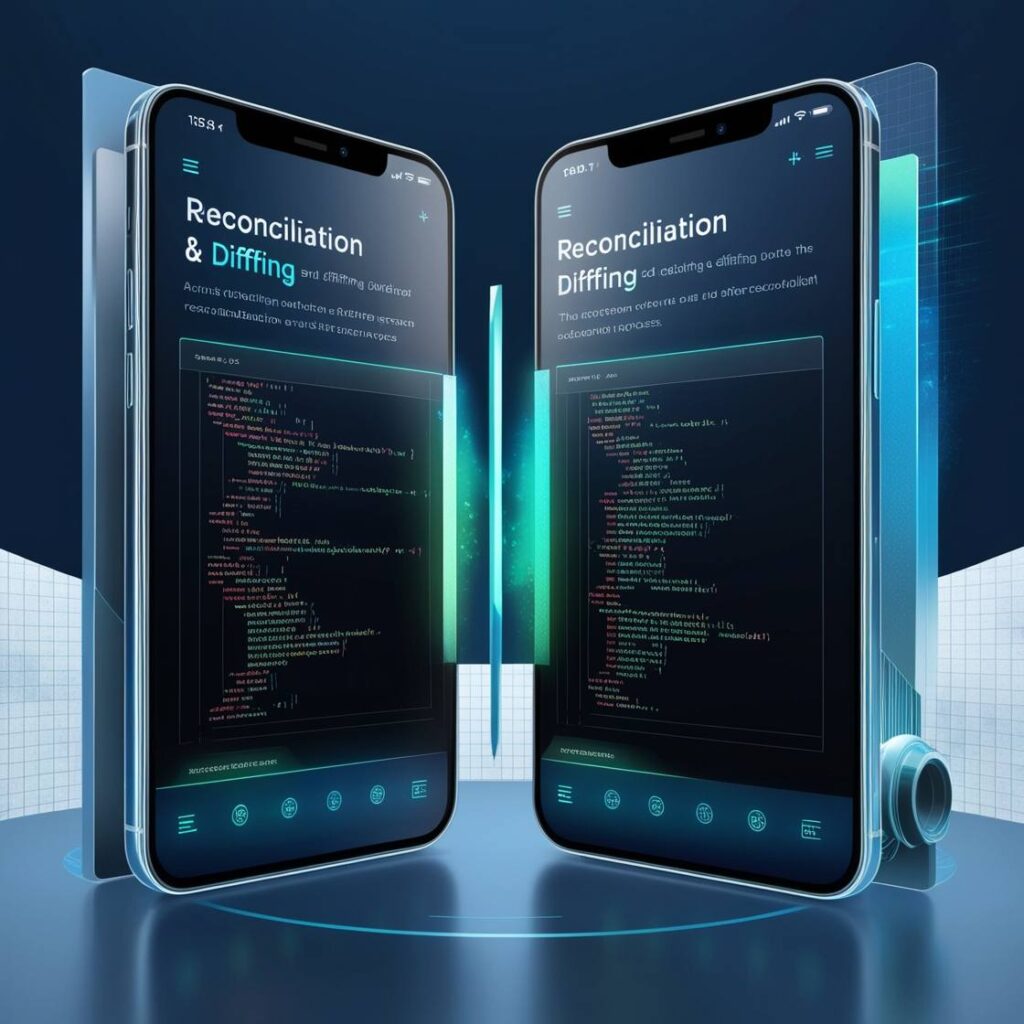
This is where React comes in with a brilliant solution. Instead of working directly with the browser’s DOM (which is slow to update), React creates a lightweight copy in memory called the Virtual DOM.
Here’s how it works:
- Think of it as a blueprint of your page
- It’s much faster to work with than the actual DOM
- React keeps it in memory where changes are lightning-quick
- Smart Updates:
- When something changes, React first updates its Virtual DOM
- Then it compares the Virtual DOM with the previous version
- It finds the minimum number of changes needed
- Only these specific changes are applied to the real DOM
- This is React’s process of figuring out what changed
- It’s like having a super-efficient assistant who knows exactly what needs updating
- No more rebuilding the entire house to change that light bulb!
Why This Matters
This approach means:
- Your web apps run faster
- Less computer resources are used
- Better user experience
- Developers can focus on building features instead of optimizing DOM updates
Real-World Example
Think about a chat application:
- New messages arrive frequently
- People are typing
- Status indicators change
- Read receipts update
Without React’s Virtual DOM and reconciliation:
- Each update might cause the entire chat window to rebuild
- This would make the app slow and possibly glitchy
With React:
- Only the new message gets added to the DOM
- Everything else stays exactly as it is
- The app stays smooth and responsive
Understanding the DOM and Reconciliation
2. Understanding the DOM
What is the Document Object Model?
The DOM is like a family tree for your web page. Every element in your HTML – every button, paragraph, or div – becomes a node in this tree. When you load a webpage, the browser creates this tree structure that represents your entire page.
Here’s a simple example:
<div id="root">
<header>
<h1>Welcome</h1
</header>
<main>
<p>Hello World!</p>
</main>
</div>
This HTML creates a DOM tree that looks like this:
div (root)
โโโ header
โ
โโโ h1 ("Welcome")
โโโ main
โโโ p ("Hello World!")
2.2 Virtual DOM
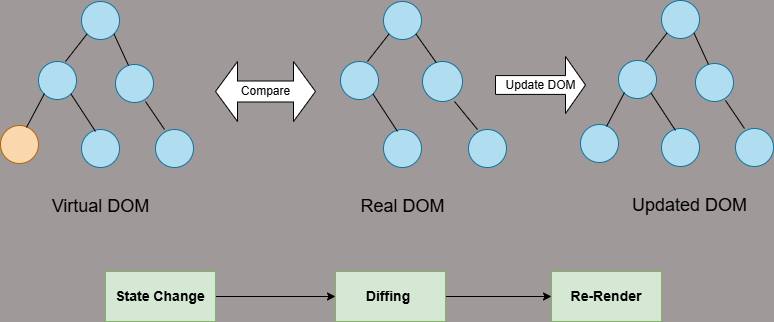
Concept and Purpose
The Virtual DOM is React’s lightweight copy of the real DOM. Think of it as a blueprint that React uses to plan out changes before making them.
Structure and Representation
Here’s how React represents elements in the Virtual DOM:
// Real DOM element
<div class="user-card">
<h2>John Doe</h2>
<p>Software Developer</p>
</div>
// Virtual DOM representation
const virtualElement = {
type: 'div',
props: {
className: 'user-card',
children: [
{
type: 'h2',
props: { children: 'John Doe' }
},
{
type: 'p',
props: { children: 'Software Developer' }
}
]
}
}
Memory Advantages
- Virtual DOM objects are plain JavaScript objects
- They don’t have all the overhead of real DOM nodes
- Changes to Virtual DOM don’t trigger reflows/repaints
- Multiple changes can be batched together
3. Reconciliation in React
3.1 What is Reconciliation?
Definition and Purpose
Reconciliation is React’s way of comparing the Virtual DOM with the real DOM to determine what needs to change. It’s like having a smart assistant that finds the most efficient way to update your UI.
Example of Component Updates
function UserProfile({ user }) {
return (
<div className="profile">
<h2>{user.name}</h2>
<p>{user.bio}</p>
<span>{user.lastActive}</span>
</div>
);
}
// When user data changes:
// 1. React creates new Virtual DOM
// 2. Compares it with previous version
// 3. Updates only what changed
3.2 The Reconciliation Process
Let’s see a practical example of how React handles updates:
class TodoList extends React.Component {
state = {
todos: ['Learn React', 'Master Reconciliation']
};
addTodo = (newTodo) => {
// React handles this update efficiently
this.setState(prevState => ({
todos: [...prevState.todos, newTodo]
}));
};
render() {
return (
<ul>
{this.state.todos.map((todo, index) => (
<li key={index}>{todo}</li>
))}
</ul>
);
}
}
When the state changes:
- React creates a new Virtual DOM tree with the updated todos
- Compares it with the previous Virtual DOM tree
- Identifies that only a new
<li>
needs to be added - Updates only that specific part of the real DOM
Understanding React’s Diffing Algorithm and Optimization
4. The Diffing Algorithm
React’s diffing algorithm works under two key assumptions:
- Elements of different types will produce different trees
- Elements with stable keys will remain stable across renders
Element Type Comparison
React first checks if the element types are the same. For example:
// Old render
<div>
<Counter />
</div>
// New render
<span>
<Counter />
</span>
Here, div โ span triggers a full rebuild because the types are different.
5. Diffing Process Deep Dive
5.1 Tree Diffing
React performs a breadth-first tree traversal, comparing nodes level by level. This is more efficient than checking every possible combination of elements.
5.2 Element Diffing
Props and attributes are compared efficiently:
class Welcome extends React.Component {
render() {
// Only style will update, onClick remains the same
return (
<div
style={{ color: this.props.color }}
onClick={this.handleClick}
>
Hello!
</div>
);
}
}
5.3 Component Diffing
Class vs Functional Components
React treats them similarly for diffing, but they have different optimization options:
// Class Component with PureComponent
class TodoItem extends React.PureComponent {
render() {
return <li>{this.props.text}</li>;
}
}
// Functional Component with memo
const TodoItem = React.memo(({ text }) => (
<li>{text}</li>
));
6. Optimization Techniques
6.1 Keys in Lists
// DON'T: Using non-unique keys
const BadList = () => (
<ul>
{items.map(item => (
<li key={item.category}>
{item.name}
</li>
))}
</ul>
);
// DO: Using unique, stable keys
const GoodList = () => (
<ul>
{items.map(item => (
<li key={`${item.category}-${item.id}`}>
{item.name}
</li>
))}
</ul>
);
7. Conclusion
React’s Virtual DOM is like having a super-efficient assistant who knows exactly how to update your webpage with minimal effort. Instead of rebuilding everything from scratch for each small change, it intelligently updates just what’s necessary. This makes websites faster, more responsive, and more enjoyable to use.
The next time you’re smoothly scrolling through a website, liking posts, or seeing instant updates, remember that React’s Virtual DOM might be working behind the scenes to make your experience seamless and efficient.
Future of React Rendering
- Concurrent Mode
- Server Components
- Automatic batching improvements