In today’s fast-paced digital world, instant communication is essential. Real-time chat applications play a crucial role for both business and personal use, enhancing interaction and connectivity globally. This guide provides a detailed step-by-step approach to creating a real-time chat app using Express and WebSockets.
Express is a flexible Node.js web application framework designed to build single-page, multi-page, and hybrid web applications. WebSockets enable an interactive communication session between a user’s browser and a server. By combining both, you can develop an efficient real-time chat application that allows users to exchange messages with minimal delay.
To start building a real-time chat application, you need to set up your backend server using Express. This process involves installing the necessary packages and setting up a basic server that will manage message exchanges between clients.
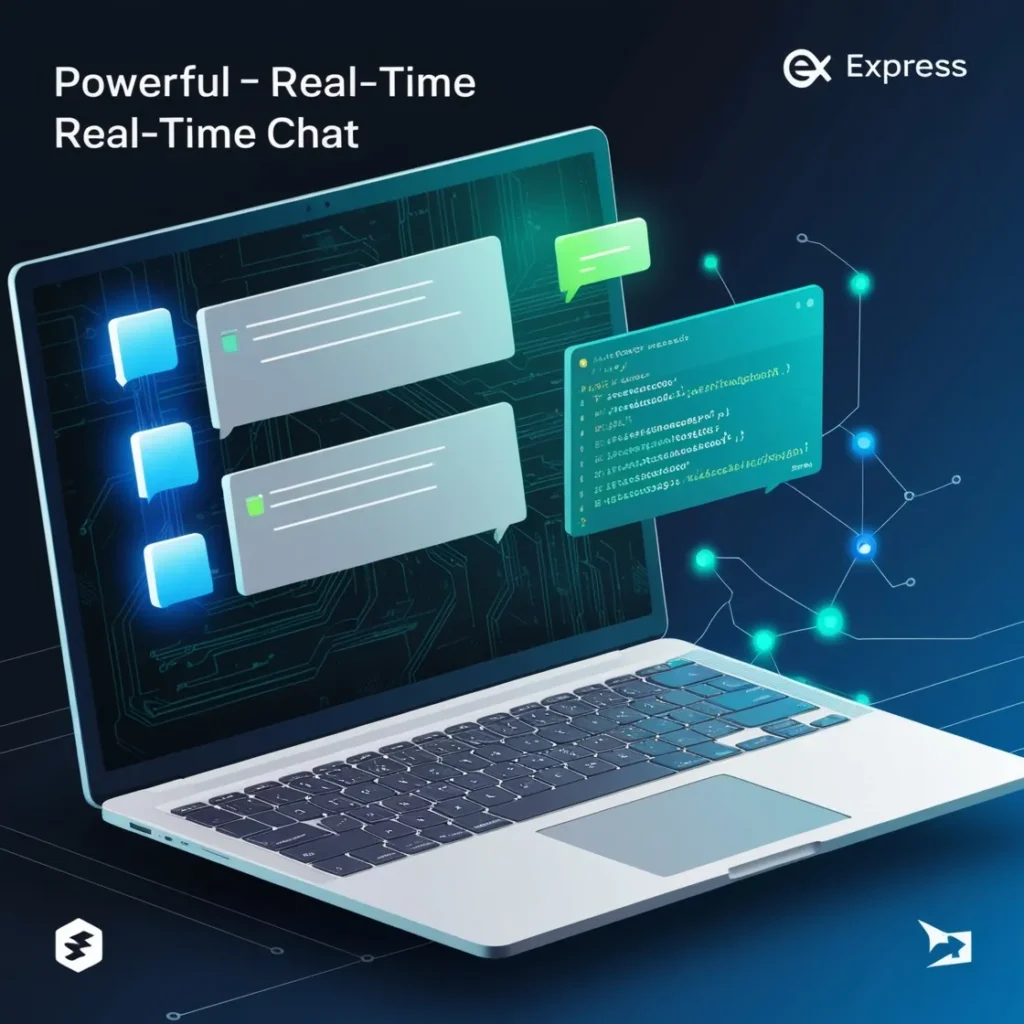
Installing Express
First, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript on the server side. Download and install it from Node.js’s official website (https://nodejs.org/).
With Node.js installed, follow these steps to set up Express:
1. Open your command-line interface and create a new Node.js project:
- `mkdir my-chat-app`: Create a new directory.
- `cd my-chat-app`: Move into your new directory.
- `npm init -y`: Create a new package.json file with default settings.
2. Install Express:
npm install express
This command downloads and installs the latest version of Express and adds it as a dependency in your package.json file.
Creating the Server
With Express installed, create the server:
1. Create a new file named `server.js` in your project directory:
2. Open this file in your preferred text editor and start by importing Express:
const express = require('express');
3. Initialize your Express application:
<br><br>const app = express();
4. To handle real-time communication, make your server listen to incoming requests.
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Your basic server is now set up and running on localhost. It can be accessed by entering `localhost:3000` in your browser’s address bar. In the next sections, you’ll integrate WebSockets with this server and implement functionality to handle and relay chat messages in real-time.
Integrating WebSockets for Real-Time Messaging
Installing WebSockets Library
To integrate WebSockets into your chat application, you must install a suitable WebSocket library. `ws` is a popular choice for Node.js and Express applications due to its simplicity and efficiency.
1. Install `ws`:
npm install ws
Implementing WebSocket Functionality
Next, set up WebSocket on the server side:
1. Import the WebSocket library into your Express application. Create a WebSocket server by attaching it to the existing HTTP server:
This code snippet handles new connections and messages from clients. When a client connects or sends a message, the server logs the event.
Handling web socket events
Properly handling WebSocket events is crucial for smooth communication.
`open`: Confirms the connection is established.
– `message`: Triggered when a message is received from the client.
– `error`: Helps in diagnosing issues with the WebSocket.
– `close`: Important for cleaning up the client’s session when the connection ends.
Building the Chat Interface
Designing the UI
The user interface (UI) of your chat application should be intuitive and engaging. Use HTML and CSS to construct a clear and functional chat window. Consider features such as a text input box, a submit button, and a message display area. Responsive design ensures accessibility across various devices, including desktops, tablets, and smartphones.
Enhanced visual appeal and usability can be achieved using UI frameworks like Bootstrap or Materialize, which provide ready-to-use components.
Handling User Interactions
Effective handling of user interactions is key. Capture user inputs with JavaScript and use WebSockets to send these inputs to the server.
1. Attach an event listener to your form:
document.getElementById('messageForm').addEventListener('submit', function(event) {
event.preventDefault();
const message = document.getElementById('messageInput').value;
ws.send(message); // Send the message through the WebSocket
document.getElementById('messageInput').value = ''; // Clear the input field
});
This script captures the form submission, prevents default behavior, sends the message to the server, and clears the input field.
Testing and Troubleshooting
Testing the Chat Application
Testing is critical for ensuring functionality. Start with unit testing to verify each function, especially those handling WebSockets, operates correctly. Use frameworks like Mocha and Chai for writing tests. Integration testing ensures smooth interaction between client-side and server-side components, with tools like Jest helping automate these tests.
System testing includes performance and load handling. Tools like Loader.io simulate multiple users to test system behavior under stress. Finally, acceptance testing ensures the system meets user requirements, potentially involving beta testers or crowdsourced testing platforms.
Troubleshooting Common Issues
Here are some common issues and possible fixes:
- Connection Issues: Ensure correct WebSocket server address and no firewall or security settings blocking WebSocket connections.
- Message Loss: Implement acknowledgments from the server to track missed messages.
- High Latency: Optimize data payload and use binary data to reduce transmission time.
- Server Crashes: Include error handling in code and use tools like PM2 for process management.
By resolving these issues, you ensure a smoother user experience and maintain the integrity of the chat application.
Conclusion and Next Steps
Building a real-time chat application with Express and WebSockets introduces you to real-time web technologies. Follow the steps outlined to create an efficient and robust chat application. Consider these next steps to enhance your application:
- Add features like voice and video calling.-
- Implement end-to-end encryption for security.
- Integrate AI for automated responses or assistance.
- Scale your application with a microservices architecture or cloud infrastructure for greater scalability and reliability.
- Continuous testing, updating, and refining will keep your application modern and impactful. The skills gained from this project are valuable for numerous other web development projects.