In the ever-evolving landscape of web development, speed and efficiency are paramount. Enter Vite.js, a build tool that’s revolutionizing the way developers approach both frontend and full-stack applications. In this comprehensive guide, we’ll explore how Vite.js can supercharge your development workflow, making it an indispensable tool for modern web applications.
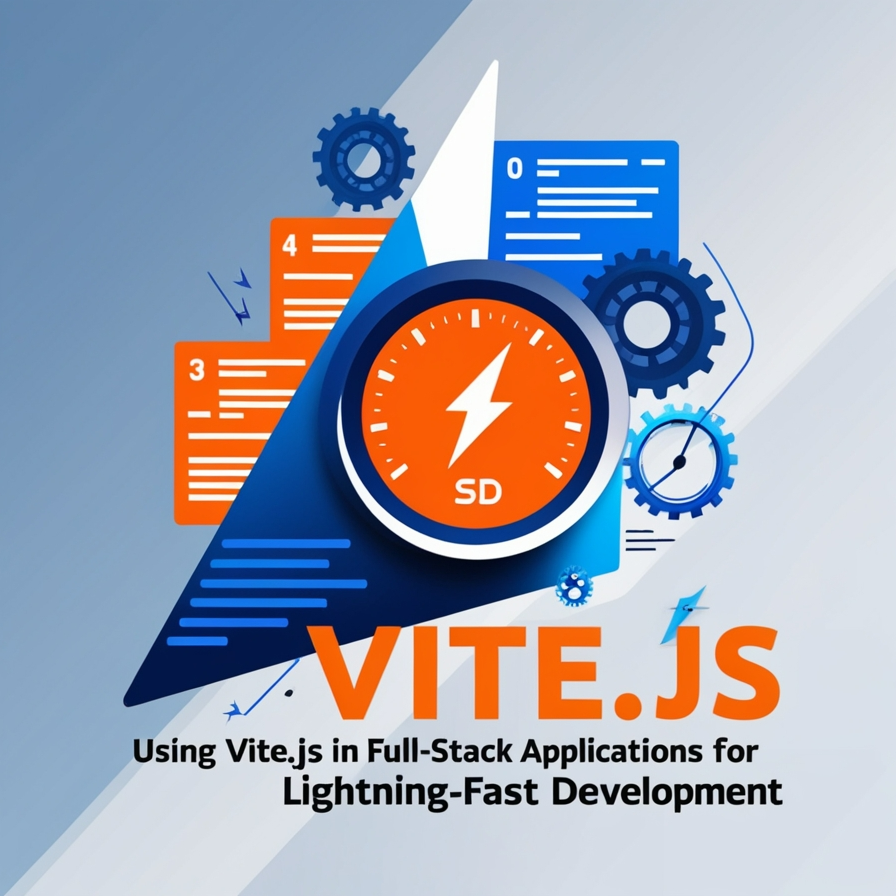
Introduction to Vite.js
Vite.js, pronounced “veet” (French for “quick”), is a next-generation frontend build tool created by Evan You, the mind behind Vue.js. However, don’t let its origins fool you – Vite.js is framework-agnostic and can be used with React, Svelte, or even vanilla JavaScript.
“No-bundle Dev Server + Lightning Fast HMR + Optimized Build” – Vite.js official description
At its core, Vite.js leverages native ES modules in the browser, allowing for an incredibly fast development server. It also employs Rollup for production builds, resulting in highly optimized deployments.
The Power of Vite.js in Full-Stack Development
While Vite.js initially gained popularity as a frontend tool, its capabilities extend far beyond that. In full-stack development, Vite.js shines by:
- Accelerating Development Cycles: With near-instantaneous server start-up and lightning-fast hot module replacement (HMR), developers can iterate quickly on both frontend and backend code.
- Simplifying Build Processes: Vite.js’s out-of-the-box optimizations reduce the need for complex build configurations, allowing developers to focus on writing code rather than managing build tools.
- Enhancing Performance: By leveraging ES modules and efficient code splitting, Vite.js-powered applications often see significant performance improvements in both development and production environments.
- Facilitating Modern Development Practices: Vite.js’s support for TypeScript, CSS pre-processors, and various frameworks makes it an ideal choice for modern, full-stack JavaScript applications.
Setting Up a Full-Stack Project with Vite.js
Let’s walk through setting up a basic full-stack project using Vite.js, Node.js, and Express.
First, create a new Vite.js project:
npm create vite@latest my-fullstack-app -- --template react
cd my-fullstack-app
npm install
Next, let’s add Express for our backend:
npm install express
Create a new file server.js
in the root directory:
const express = require('express');
const path = require('path');
const app = express();
// Serve static files from the React app
app.use(express.static(path.join(__dirname, 'dist')));
// API routes
app.get('/api/hello', (req, res) => {
res.json({ message: 'Hello from the server!' });
});
// The "catchall" handler: for any request that doesn't
// match one above, send back React's index.html file.
app.get('*', (req, res) => {
res.sendFile(path.join(__dirname, 'dist', 'index.html'));
});
const port = process.env.PORT || 5000;
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
Update your package.json
to include scripts for running both the frontend and backend:
{
"scripts": {
"dev": "vite",
"build": "vite build",
"serve": "vite preview",
"start": "node server.js"
}
}
Now you have a basic full-stack setup with Vite.js for the frontend and Express for the backend!
Optimizing Frontend Performance with Vite.js
Vite.js offers several features that can significantly boost your frontend performance:
1. Code Splitting
Vite.js automatically performs code splitting, ensuring that only the necessary code is loaded for each route. This can dramatically improve initial load times, especially for larger applications.
Example of dynamic imports in React with Vite.js:
import React, { Suspense, lazy } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
function App() {
return (
<div>
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</div>
);
}
2. CSS Optimization
Vite.js processes and optimizes your CSS out of the box. It supports CSS modules, preprocessors like Sass, and PostCSS.
Example of using CSS modules in a Vite.js project:
import styles from './Button.module.css'
function Button() {
return <button className={styles.button}>Click me</button>
}
3. Asset Handling
Vite.js optimizes asset loading and provides a straightforward way to import various file types:
import imgUrl from './img.png'
import svgContent from './icon.svg?raw'
Integrating Backend Services with Vite.js
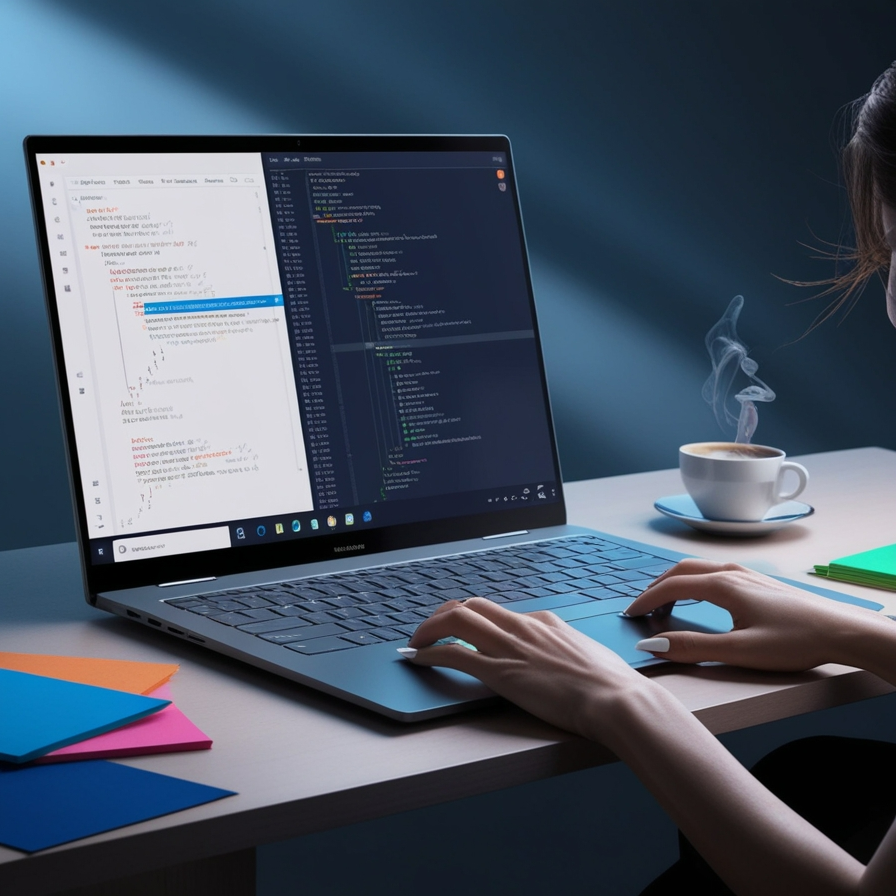
While Vite.js primarily focuses on frontend development, integrating it with backend services is straightforward. Here’s how you can connect your Vite.js frontend with an Express backend:
- Proxy Configuration: During development, you can use Vite’s built-in proxy to forward API requests to your backend server. Add this to your
vite.config.js
:
export default {
server: {
proxy: {
'/api': 'http://localhost:5000'
}
}
}
- API Calls: Use modern JavaScript features like
fetch
or libraries like Axios to make API calls. Here’s an example using React hooks:
import { useState, useEffect } from 'react'
function App() {
const [message, setMessage] = useState('')
useEffect(() => {
fetch('/api/hello')
.then(response => response.json())
.then(data => setMessage(data.message))
}, [])
return <div>{message}</div>
}
- WebSocket Integration: For real-time applications, you can easily integrate WebSockets with Vite.js. Here’s a basic example using
socket.io-client
:
import { useEffect, useState } from 'react'
import io from 'socket.io-client'
function Chat() {
const [socket, setSocket] = useState(null)
useEffect(() => {
const newSocket = io('http://localhost:5000')
setSocket(newSocket)
return () => newSocket.close()
}, [])
// Use the socket for real-time communication
// ...
}
Testing and Debugging in a Vite.js Environment
Vite.js’s speed advantages extend to testing and debugging as well. Here are some tips for effective testing and debugging in a Vite.js project:
1. Unit Testing
Vite.js works seamlessly with popular testing frameworks like Jest and Vitest. Here’s how you can set up Vitest, a Vite-native unit test framework:
npm install -D vitest
Add a test script to your package.json
:
{
"scripts": {
"test": "vitest"
}
}
Create a test file, e.g., sum.test.js
:
import { expect, test } from 'vitest'
import { sum } from './sum'
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3)
})
2. E2E Testing
For end-to-end testing, you can use tools like Cypress or Playwright. Here’s a quick setup for Cypress:
npm install -D cypress
Add a script to your package.json
:
{
"scripts": {
"cypress:open": "cypress open"
}
}
Create a simple Cypress test in cypress/integration/sample_spec.js
:
describe('My First Test', () => {
it('Visits the app', () => {
cy.visit('http://localhost:3000')
cy.contains('Hello, Vite + React!')
})
})
3. Debugging
Vite.js supports source maps out of the box, making debugging a breeze. You can use browser developer tools or IDE debuggers just as you would with any other JavaScript application.
For server-side debugging, you can use tools like node --inspect
or IDE integrations for Node.js debugging.
Deploying Full-Stack Vite.js Applications
Deploying a full-stack Vite.js application involves a few key steps:
- Build the Frontend: Run
npm run build
to create an optimized production build of your Vite.js frontend. - Serve Static Files: Configure your backend (e.g., Express) to serve the static files from the
dist
directory. - Handle Routing: Ensure your server is configured to handle both API routes and frontend routes correctly.
- Environment Variables: Use environment variables for configuration that differs between development and production.
Here’s an example of a simple deployment script for a Heroku-like platform:
{
"scripts": {
"start": "node server.js",
"build": "vite build",
"heroku-postbuild": "npm run build"
}
}
Best Practices and Tips for Vite.js Development
To get the most out of Vite.js in your full-stack projects, consider these best practices:
- Leverage ES Modules: Vite.js is built around ES modules. Use
import
andexport
statements consistently. - Optimize Assets: Use Vite.js’s built-in asset handling features for images, fonts, and other static assets.
- Embrace Hot Module Replacement: Take advantage of Vite.js’s fast HMR to speed up your development workflow.
- Use TypeScript: Vite.js has excellent TypeScript support. Leverage it for better type safety and developer experience.
- Modularize Your Backend: Structure your backend code in a modular way to make it easier to integrate with Vite.js’s frontend setup.
“Simplicity is the ultimate sophistication.” – Leonardo da Vinci
This quote encapsulates the philosophy behind Vite.js. By simplifying the build process and leveraging modern web standards, Vite.js allows developers to create sophisticated applications with less complexity.
Conclusion
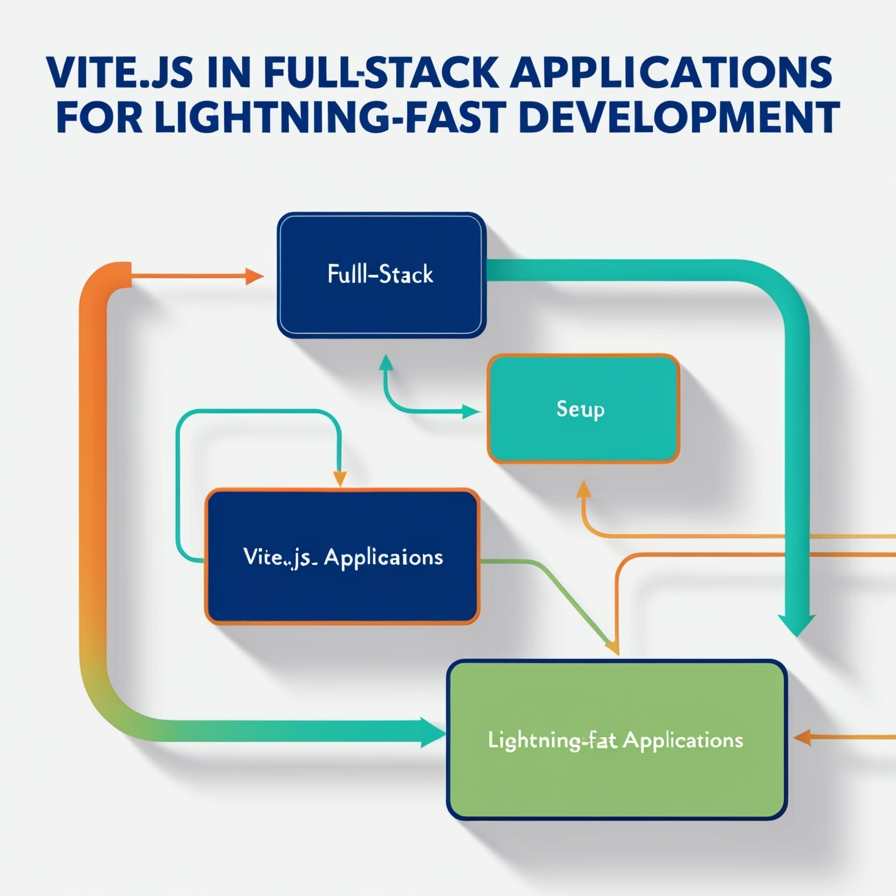
Vite.js represents a significant leap forward in the world of web development tools. Its speed, simplicity, and flexibility make it an excellent choice for full-stack applications. By leveraging Vite.js’s strengths in both frontend and backend development, teams can create high-performance, scalable applications with a streamlined development experience.
As web development continues to evolve, tools like Vite.js that prioritize developer experience without compromising on performance will play an increasingly important role. Whether you’re building a small personal project or a large-scale enterprise application, Vite.js offers the tools and optimizations needed to succeed in today’s fast-paced development landscape.
Embrace the speed and simplicity of Vite.js, and watch your development workflow transform. Happy coding!
FAQs
- Q: Is Vite.js only for Vue.js projects?
A: No, Vite.js is framework-agnostic. While it was created by the creator of Vue.js, it supports React, Preact, Svelte, and even vanilla JavaScript projects out of the box. - Q: How does Vite.js compare to webpack or Create React App?
A: Vite.js generally offers faster build times and a more streamlined configuration process compared to webpack or Create React App. It leverages native ES modules for development, resulting in near-instantaneous server start-up and updates. - Q: Can I use Vite.js with server-side rendering (SSR)?
A: Yes, Vite.js supports server-side rendering. It provides an SSR-specific build command and utilities to help set up SSR projects, though it may require more manual configuration compared to some other frameworks. - Q: Does Vite.js support older browsers?
A: Vite.js produces builds that work in browsers that support native ES Modules, which includes all modern browsers. For older browsers, you can use the @vitejs/plugin-legacy plugin, which automatically generates legacy chunks and corresponding ES language feature polyfills. - Q: Can I migrate my existing project to Vite.js?
A: Yes, it’s possible to migrate existing projects to Vite.js, especially if they’re already using ES modules. Vite.js provides migration guides for popular frameworks. However, the complexity of migration can vary depending on your project’s structure and dependencies.