Welcome to the world of Express.js! Whether you’re a beginner stepping into web development or a seasoned developer looking to brush up on your skills, this blog is designed just for you. Express.js is a fast, unopposed, and minimalist web framework for Node.js, perfect for building APIs and web applications. Today, we’ll focus on a crucial skill every web developer should possess—fetching data from an API endpoint using Express.js. By the end of this guide, you’ll understand the basic steps involved in setting up an Express server and how to make API calls effectively. Get ready to elevate your web development prowess with these indispensable skills!
Setting Up Express.js for API Data Fetching
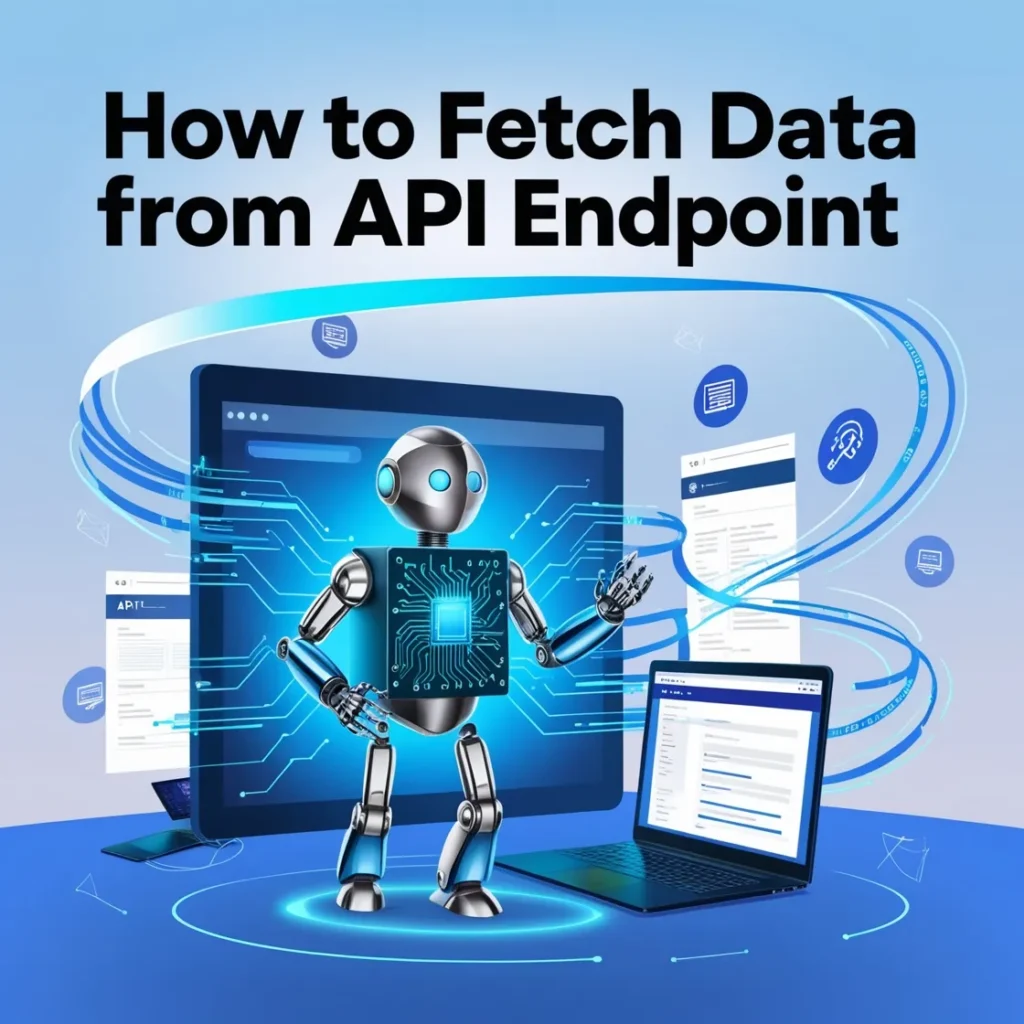
Installing Express.js
Before diving into data fetching, the first thing you need is to set up Express.js, a popular web application framework for Node.js. It’s designed to make developing websites, web apps, and APIs much simpler. To get started, you’ll need Node.js and npm (node package manager) installed on your computer. Once these prerequisites are met, installing Express is straightforward.
Open your terminal, and create a new directory where you want your project to reside. Navigate into your directory, and initialize a new Node.js project by running:
npm init -y
This command creates a ‘package.json’ file in your directory, which will manage all your dependencies and other configurations. Next, install the Express framework by typing:
npm install express
This command fetches the Express.js package from npm and installs it in your project, updating your ‘package.json’ to include Express as a dependency.
Creating an Express.js Server
With Express.js installed, you can now create a simple server. Create a new file named ‘app.js’, and open it in your favorite code editor. Start by requiring the Express module and creating an instance of an Express app:
const express = require('express');
const app = express();
Set up a basic route that your server will respond to. For instance, a GET request to the root route (‘/’) can be handled as follows:
app.get('/', (req, res) => {
res.send('Hello World!');
});
Next, make the server listen on a specific port for requests:
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(\`Server is running on port ${PORT}\`);
});
To test your server, save your ‘app.js’ file and run it from your terminal using Node.js:
node app.js
Navigate to ‘http://localhost:3000’ in your web browser. You should see ‘Hello World!’ displayed, confirming that your server is working correctly.
Making an API Request in Express.js
Understanding HTTP Request Methods
HTTP request methods are crucial in web development, defining the action to be taken towards a specific resource identified by a given URL. The common methods include GET, POST, PUT, DELETE, amongst others. For fetching data from an API, the GET method is most commonly used. It retrieves data from a specified source and has no other effect (i.e., it’s a “safe” method).
Sending a GET Request to API Endpoint
To send a GET request to fetch data from an API endpoint using Express.js, you’ll need another module named ‘axios’, a promise-based HTTP client for the browser and node.js. Install axios via npm:
npm install axios
In your ‘app.js’, require axios and set up a new route specifically for fetching API data:
const axios = require('axios');
app.get('/data', async (req, res) => {
try {
const response = await axios.get('https://api.example.com/data');
res.send(response.data);
} catch (error) {
{
res.status(500).send(error);
}
}
});
Replace ‘https://api.example.com/data’ with the URL of the API you want to fetch data from. This request will contact the API server, request data, and forward it back to the client.
Handling API Response
Handling the response from an API is as vital as sending the request. In the example above, axios.get
asynchronously fetches data from the API and returns a promise. The await
keyword is used to pause your code on this line until the promise settles, and then resumes the async function after fulfillment. When the request is successful, the data will be sent back to the client with res.send(response.data)
;.
It’s important also to handle situations where the API call might fail. In the catch block, the server responds with an error code 500, indicating an internal server error, which informs the client that the request was unsuccessful due to a server-side issue. Here also, you might want to add additional logic to handle different types of errors distinctively, especially those pertaining to the client-side to improve user experience.
By following this guide, you have set up a basic Express.js server capable of fetching data from APIs. This setup serves as a strong foundation for more complex web applications and services you might build in the future, offering a flexible approach to handling HTTP requests and responses efficiently and effectively.
Parsing API Data Using Express.js
Image courtesy: Unsplash
When fetching data from an API using Express.js, parsing the data is a crucial step. This usually involves interpreting the data format returned by the API, which is typically JSON (JavaScript Object Notation). JSON is favored in web development due to its ease of use and its ability to seamlessly integrate with JavaScript, making it ideal for projects using Express.js.
Working with JSON Data
JSON data fetched from an API needs to be parsed to transform it into a JavaScript object for easy manipulation and access within your Express.js application. When you make a request to an API, the response you receive is often in string format. Here is how you can handle JSON data:
1. Use the JSON.parse()
method to convert the JSON string into a JavaScript object.
2. Access the data by referring to the properties of the object. For example, if the data contains a user’s details, you can access the user’s name with \`data.name\`.
Ensure that your API request logic includes error checking when parsing JSON, as this will help to catch any issues that might occur if the data is not in the expected format or is corrupted.
Displaying Fetched Data in Express view
Once the data is parsed, you can easily utilize it within your Express.js views. When using template engines like EJS or Handlebars, displaying data becomes straightforward:
- Pass the parsed data to the view through the rendering function in your route handling.
- Use the templating syntax provided by your chosen engine to display the data. For instance, in EJS, you would include
<%= user.name %>
within your HTML to display the name of the user.
The rendered views can dynamically display data fetched from the API, updating as needed without requiring page refreshes.
Error Handling in Express.js API Data Fetching
Proper error handling is essential in developing robust web applications. Express.js offers several methods for managing errors efficiently, ensuring that your application can handle issues gracefully without crashing and can provide useful feedback to the user.
Implementing Error Handling Middleware
Express.js middleware functions are powerful tools for intercepting and modifying request and response objects. You can create middleware to handle errors that may occur during the API data fetching process. Here’s a simple way to implement error handling middleware:
// Error handling middleware
app.use((err, req, res, next) => {
console.error(err.stack); // Log error message in our server's console
res.status(500).send('Something broke!');
});
This middleware will catch any errors that occur during processing in any route or middleware and will send a generic error message to the client while logging the error detail to your server’s console.
Pro Tip: It’s crucial to define error-handling middleware last, after other app.use() and route calls; this order ensures it catches errors from the entire application.
Displaying Error Sorry Messages to the User
Keeping the user informed about what went wrong can significantly enhance user experience. Implement thoughtful error handling frontend by providing error messages that help users understand the issue without exposing them to technical details that may be confusing or irrelevant.
Here are a few strategies for displaying error messages to users:
- Customize error pages: Create specific templates for different error statuses like 404 (Not Found) and 500 (Internal Server Error). These pages can guide users on what to do next.
- Use alerts or modals to display error messages dynamically. This can be effectively done using JavaScript to alter the user’s view temporarily to display the message.
- Provide suggestions for possible actions users can take in response to the error, such as returning to the homepage, trying the operation again later, or contacting support for help.
Incorporating these strategies helps maintain a seamless user experience, even in the face of errors, by keeping users informed and providing actions they can take to resolve the situation themselves or understand why the error occurred.
Conclusion
Wrapping up, mastering the fetching of data from API endpoints using Express.js not only enhances your backend development skills but also broadens your horizons in creating more dynamic and responsive web applications. By following the steps outlined, from setting up your Express server to making HTTP requests and handling responses, you’re now equipped to integrate real-world data into your projects effectively. Remember, practice is key in web development, so continue experimenting with different APIs to refine your skills and broaden your understanding. Happy coding!
Pingback: REST vs. RESTful API: Understanding Key Differences and Best Practices
Pingback: Importance of API Pagination for Efficient Data Retrieval
Pingback: Zero Dependency JavaScript: Lightweight Development