- 1. What is FastAPI?
- 2. Why Choose FastAPI?
- Automatic Documentation
- Built-in Data Validation
- 3. Setting Up Your FastAPI Environment
- Installation
- Creating Your First Application
- Running the Application
- 4. Building Real-World APIs with FastAPI
- Working with Query Parameters
- Authentication and Authorization
- Background Tasks
- Middleware
- 6. Common Errors and Solutions
- 2. Dependency Injection Errors
Hey there, fellow developers! Ready to dive into the world of modern API development? You’re about to discover FastAPI, a game-changing Python framework that’s revolutionizing how we build web APIs. Whether you’re a seasoned developer or just starting your coding journey, this comprehensive guide will help you master FastAPI from the ground up.
1. What is FastAPI?
FastAPI is a modern, fast (high-performance) web framework for building APIs with Python 3.7+ based on standard Python type hints. But what does that really mean? Imagine having a tool that helps you build web services as easily as writing regular Python functions, but with the speed and efficiency of compiled languages like Go or Java.
How Does it Compare to Other Frameworks?
Let’s break down how FastAPI stacks up against other popular Python frameworks:
FastAPI vs Flask:
- Flask is lightweight and flexible but requires additional extensions for many features
- FastAPI includes many features out of the box (validation, serialization, async support)
- FastAPI offers better performance and automatic API documentation
- Both have a similar routing syntax, making it easy for Flask developers to transition
FastAPI vs Django:
- Django is a full-featured web framework with an admin interface, ORM, and templates
- FastAPI focuses specifically on API development
- FastAPI is more lightweight and typically faster for API-only applications
- Django has a larger ecosystem, while FastAPI is more specialized
2. Why Choose FastAPI?
Speed and Performance
FastAPI isn’t just fast in terms of execution speed (though it’s one of the fastest Python frameworks available). It’s also fast when it comes to development time. You’ll write less code and catch errors earlier thanks to its built-in validation system.
Modern Python Features
FastAPI leverages Python’s type hints in a way that feels natural and intuitive:
from typing import Optional
from fastapi import FastAPI
app = FastAPI()
@app.get("/users/")
def read_user(user_id: int, premium: Optional[bool] = None):
return {"user_id": user_id, "premium": premium}
Automatic Documentation
One of FastAPI’s most impressive features is its automatic interactive API documentation. Your API gets two different documentation interfaces for free:
- Swagger UI at
/docs
- ReDoc at
/redoc
Built-in Data Validation
Thanks to Pydantic integration, FastAPI automatically validates your data:
from pydantic import BaseModel, EmailStr
class User(BaseModel):
username: str
email: EmailStr
age: int
@app.post("/users/")
def create_user(user: User):
# Data is already validated when it reaches this function
return {"message": f"User {user.username} created"}
3. Setting Up Your FastAPI Environment
Installation
First, let’s set up your development environment. Open your terminal and run:
pip install fastapi[all]
This command installs FastAPI along with all optional dependencies, including:
- uvicorn – for running the application
- pydantic – for data validation
- starlette – the foundation framework
- python-multipart – for form data handling
- python-jose – for JWT tokens
- passlib – for password hashing
Creating Your First Application
Create a new file called main.py
:
from fastapi import FastAPI
app = FastAPI(
title="My Amazing API",
description="This is a very fancy API",
version="1.0.0"
)
@app.get("/")
async def root():
return {"message": "Welcome to FastAPI!"}
Running the Application
Start your server with:
uvicorn main:app --reload --port 8000
The --reload
flag enables auto-reload during development, so your server updates automatically when you make changes.
4. Building Real-World APIs with FastAPI
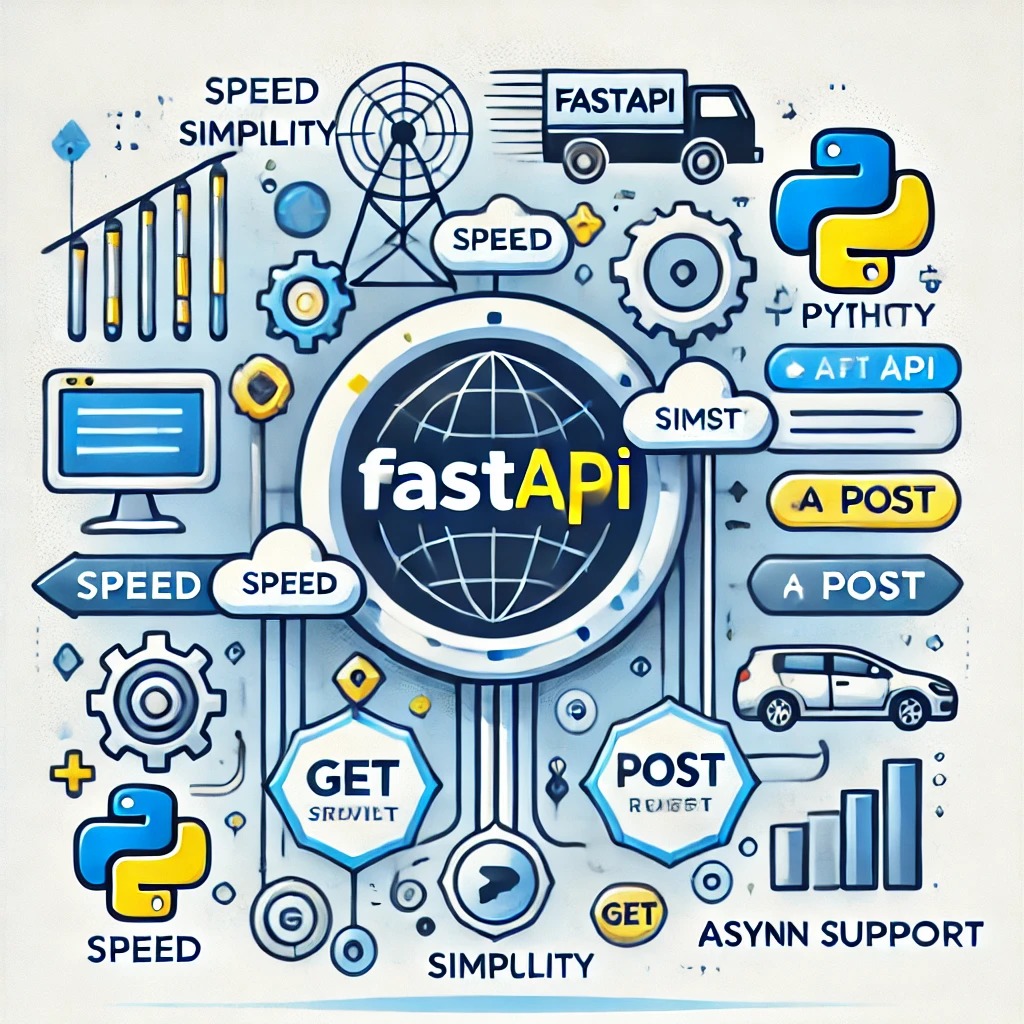
Working with Different HTTP Methods
FastAPI supports all standard HTTP methods. Here’s a complete CRUD example:
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
from typing import List, Optional
app = FastAPI()
# Data model
class Item(BaseModel):
name: str
description: Optional[str] = None
price: float
tax: Optional[float] = None
# In-memory storage
items = {}
# Create
@app.post("/items/")
async def create_item(item: Item):
if item.name in items:
raise HTTPException(status_code=400, detail="Item already exists")
items[item.name] = item
return item
# Read
@app.get("/items/{item_name}")
async def read_item(item_name: str):
if item_name not in items:
raise HTTPException(status_code=404, detail="Item not found")
return items[item_name]
# Update
@app.put("/items/{item_name}")
async def update_item(item_name: str, item: Item):
if item_name not in items:
raise HTTPException(status_code=404, detail="Item not found")
items[item_name] = item
return item
# Delete
@app.delete("/items/{item_name}")
async def delete_item(item_name: str):
if item_name not in items:
raise HTTPException(status_code=404, detail="Item not found")
del items[item_name]
return {"message": "Item deleted"}
Working with Query Parameters
FastAPI makes it easy to handle query parameters with validation:
@app.get("/search/")
async def search_items(
q: str,
max_results: Optional[int] = 10,
sort_by: Optional[str] = None,
category: Optional[str] = None
):
results = [
item for item in items.values()
if q.lower() in item.name.lower() or
(item.description and q.lower() in item.description.lower())
]
if category:
results = [item for item in results if item.category == category]
if sort_by:
results.sort(key=lambda x: getattr(x, sort_by))
return results[:max_results]
Authentication and Authorization
FastAPI provides robust support for security features. Here’s an example using OAuth2 with JWT tokens:
from fastapi import Depends, FastAPI, HTTPException, status
from fastapi.security import OAuth2PasswordBearer, OAuth2PasswordRequestForm
from jose import JWTError, jwt
from passlib.context import CryptContext
from datetime import datetime, timedelta
# Security configurations
SECRET_KEY = "your-secret-key"
ALGORITHM = "HS256"
ACCESS_TOKEN_EXPIRE_MINUTES = 30
pwd_context = CryptContext(schemes=["bcrypt"], deprecated="auto")
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
# Token creation
def create_access_token(data: dict):
to_encode = data.copy()
expire = datetime.utcnow() + timedelta(minutes=ACCESS_TOKEN_EXPIRE_MINUTES)
to_encode.update({"exp": expire})
encoded_jwt = jwt.encode(to_encode, SECRET_KEY, algorithm=ALGORITHM)
return encoded_jwt
# Authentication endpoint
@app.post("/token")
async def login(form_data: OAuth2PasswordRequestForm = Depends()):
# Validate user credentials here
access_token = create_access_token(data={"sub": form_data.username})
return {"access_token": access_token, "token_type": "bearer"}
Background Tasks
FastAPI can handle background tasks efficiently:
from fastapi import BackgroundTasks
def write_notification(email: str, message=""):
with open("log.txt", mode="a") as email_file:
content = f"notification for {email}: {message}\n"
email_file.write(content)
@app.post("/send-notification/{email}")
async def send_notification(email: str, background_tasks: BackgroundTasks):
background_tasks.add_task(write_notification, email, message="Hello World!")
return {"message": "Notification sent in the background"}
Middleware
Adding custom middleware for logging, CORS, or other purposes:
from fastapi import FastAPI, Request
from fastapi.middleware.cors import CORSMiddleware
import time
app = FastAPI()
# Add CORS middleware
app.add_middleware(
CORSMiddleware,
allow_origins=["*"],
allow_credentials=True,
allow_methods=["*"],
allow_headers=["*"],
)
# Custom middleware for request timing
@app.middleware("http")
async def add_process_time_header(request: Request, call_next):
start_time = time.time()
response = await call_next(request)
process_time = time.time() - start_time
response.headers["X-Process-Time"] = str(process_time)
return response
6. Common Errors and Solutions
1. Pydantic Validation Errors
Problem: Data validation fails
Solution:
from fastapi import HTTPException
from pydantic import ValidationError
@app.post("/items/")
async def create_item(item: Item):
try:
return item
except ValidationError as e:
raise HTTPException(status_code=422, detail=str(e))
2. Dependency Injection Errors
Problem: Circular dependencies
Solution: Use Depends()
with async functions and proper error handling:
async def get_db():
try:
db = DBSession()
yield db
finally:
db.close()
@app.get("/items/")
async def read_items(db: Session = Depends(get_db)):
return db.query(Item).all()
7. Best Practices and Tips
- Use Type Hints Consistently
- They improve code readability
- Enable better IDE support
- Help catch errors early
- Implement Proper Error Handling
- Use FastAPI’s HTTPException for API errors
- Create custom exception handlers when needed
- Return meaningful error messages
- Structure Your Application
- Use Router for organizing endpoints
- Separate business logic from route handlers
- Implement dependency injection
- Optimize Performance
- Use async functions for I/O-bound operations
- Implement caching where appropriate
- Use connection pooling for databases
8. Conclusion
FastAPI represents the future of Python web development, combining speed, ease of use, and modern features in one powerful package. Whether you’re building a simple API or a complex microservices architecture, FastAPI provides the tools you need to succeed.
Remember these key takeaways:
- FastAPI is fast in both development and runtime
- Automatic documentation saves time and improves API usability
- Type hints and validation prevent bugs before they happen
- The async support makes it future-proof and scalable
Now that you have a solid understanding of FastAPI, it’s time to start building! Begin with simple endpoints and gradually incorporate more advanced features as you become comfortable with the framework.