In the ever-evolving landscape of backend development, Node.js continues to stand out as a powerhouse for building scalable and efficient server-side applications. As we navigate through 2024, it’s crucial for backend developers to have a solid grasp of Node.js fundamentals to stay competitive and deliver high-performance solutions.
This comprehensive guide will delve into the core concepts that every Node.js developer should master. From event-driven architecture to performance optimization, we’ll cover everything you need to know to excel in Node.js development.
1. Event-Driven Architecture
At the heart of Node.js lies its event-driven architecture, a programming paradigm that forms the foundation of its non-blocking I/O model. This approach allows Node.js to handle multiple operations concurrently, making it ideal for building scalable network applications.
Understanding Event-Driven Programming
Event-driven programming is a paradigm where the flow of the program is determined by events such as user actions, sensor outputs, or messages from other programs. In Node.js, this translates to a system where actions are triggered in response to specific events.
How Node.js Leverages Event-Driven Architecture
Node.js uses an event loop to manage and execute callbacks associated with events. This allows it to handle many connections concurrently without the need for multi-threading.
Example:
const EventEmitter = require('events');
class MyEmitter extends EventEmitter {}
const myEmitter = new MyEmitter();
myEmitter.on('event', () => {
console.log('An event occurred!');
});
myEmitter.emit('event');
In this example, we create a custom event emitter that listens for an ‘event’ and logs a message when it occurs. The emit
method triggers the event.
Benefits and Challenges
Benefits:
- Highly scalable
- Efficient for I/O-bound operations
- Promotes loose coupling between components
Challenges:
- Can lead to callback hell if not managed properly
- Requires careful error handling
- May not be ideal for CPU-intensive tasks
2. Asynchronous Programming
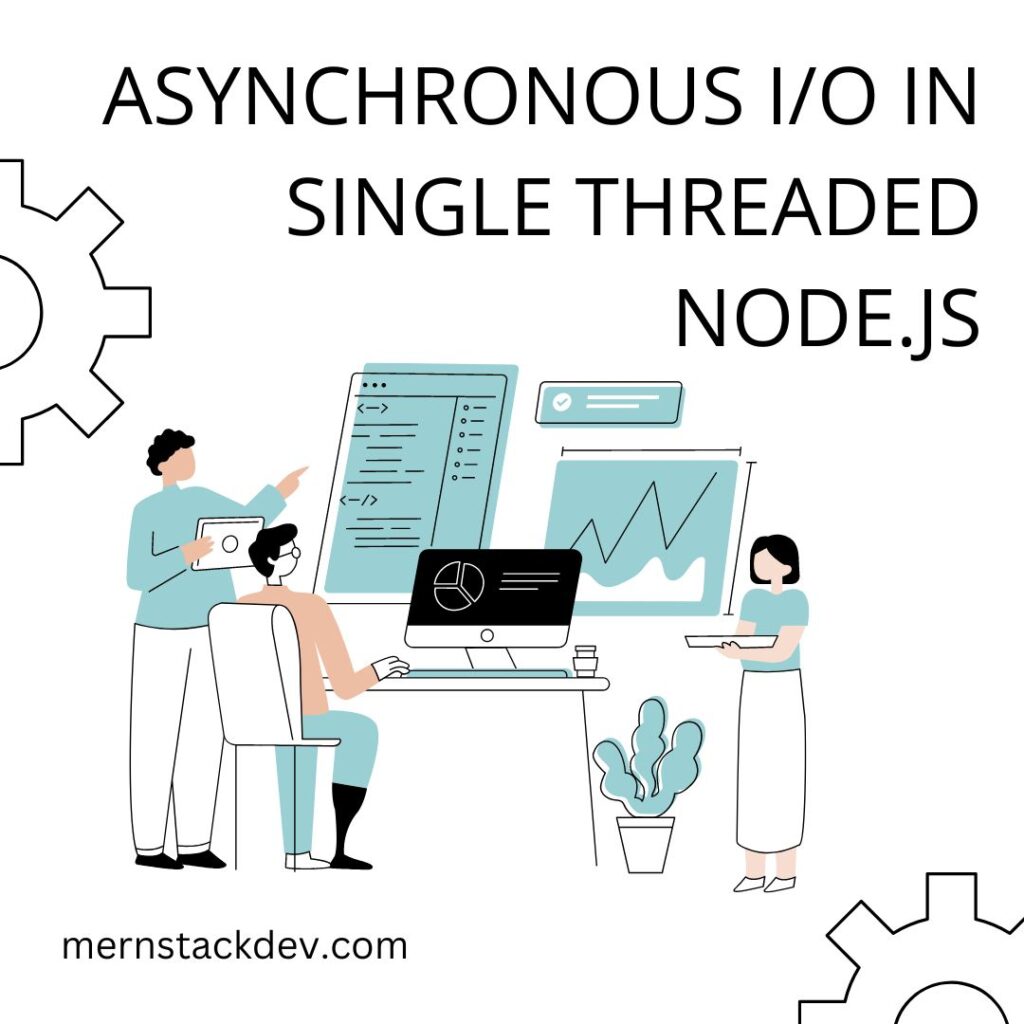
Asynchronous programming is a cornerstone of Node.js, allowing it to perform non-blocking operations efficiently. Understanding this concept is crucial for writing performant Node.js applications.
Callback Functions
Callbacks are functions passed as arguments to other functions, which are then invoked when an asynchronous operation completes.
Example:
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File contents:', data);
});
Promises and Async/Await
Promises provide a more structured way to handle asynchronous operations, allowing for better error handling and chaining of operations. Async/await is syntactic sugar built on top of promises, making asynchronous code look and behave more like synchronous code.
Example using Promises:
const readFilePromise = (filename) => {
return new Promise((resolve, reject) => {
fs.readFile(filename, 'utf8', (err, data) => {
if (err) reject(err);
else resolve(data);
});
});
};
readFilePromise('example.txt')
.then(data => console.log('File contents:', data))
.catch(err => console.error('Error reading file:', err));
Example using Async/Await:
async function readFile(filename) {
try {
const data = await readFilePromise(filename);
console.log('File contents:', data);
} catch (err) {
console.error('Error reading file:', err);
}
}
readFile('example.txt');
3. The Node.js Event Loop
The event loop is the secret sauce that allows Node.js to perform non-blocking I/O operations despite JavaScript being single-threaded. Understanding how the event loop works is crucial for writing efficient Node.js applications.
How the Event Loop Handles Asynchronous Operations
- The event loop starts running when Node.js starts.
- It checks if there are any pending timers, I/O operations, or setImmediate() callbacks.
- If there are, it executes them in order.
- If there are no more tasks, the event loop waits for new events.
“The event loop is what allows Node.js to perform non-blocking I/O operations — despite the fact that JavaScript is single-threaded — by offloading operations to the system kernel whenever possible.” – Node.js official documentation
Real-World Example
Consider a web server handling multiple requests:
const http = require('http');
const server = http.createServer((req, res) => {
if (req.url === '/') {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Home Page');
} else if (req.url === '/slow-page') {
setTimeout(() => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Slow Page');
}, 5000);
}
});
server.listen(3000, () => {
console.log('Server running on port 3000');
});
In this example, the ‘/slow-page’ route simulates a slow operation using setTimeout()
. The event loop allows the server to continue handling other requests while waiting for the timeout to complete.
4. Modules and NPM
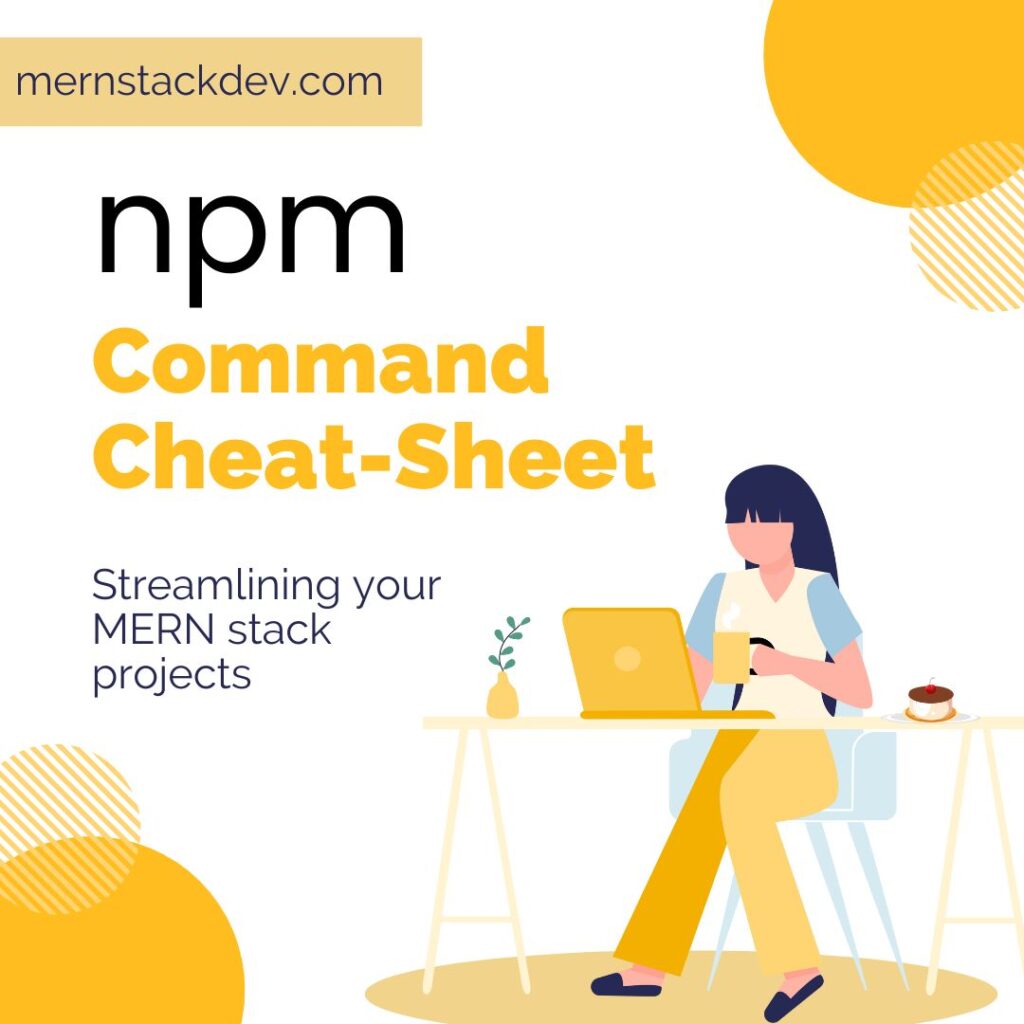
Node.js uses a modular system to organize and reuse code. Understanding how to work with modules and the Node Package Manager (NPM) is essential for every Node.js developer.
Understanding Node.js Modules
Node.js supports two module systems: CommonJS and ES6 modules.
CommonJS Example:
// math.js
module.exports = {
add: (a, b) => a + b,
subtract: (a, b) => a - b
};
// app.js
const math = require('./math');
console.log(math.add(5, 3)); // Output: 8
ES6 Module Example:
// math.mjs
export const add = (a, b) => a + b;
export const subtract = (a, b) => a - b;
// app.mjs
import { add, subtract } from './math.mjs';
console.log(add(5, 3)); // Output: 8
Role and Importance of NPM
NPM is the world’s largest software registry, allowing developers to share and reuse code packages. It’s an essential tool for managing dependencies in Node.js projects.
Installing and Managing Packages
To install a package:
npm install package-name
To save it as a dependency in your package.json
:
npm install package-name --save
Creating and Publishing Your Own Modules
To create and publish your own NPM package:
- Create your module
- Initialize a new NPM package:
npm init
- Write your code and tests
- Publish to NPM:
npm publish
5. File System (fs) Module
The fs
module provides a way to interact with the file system on your computer. It’s a crucial module for many Node.js applications, especially those dealing with data persistence or file manipulation.
Common File Operations
Reading a File:
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File contents:', data);
});
Writing to a File:
fs.writeFile('output.txt', 'Hello, World!', (err) => {
if (err) {
console.error('Error writing file:', err);
return;
}
console.log('File written successfully');
});
Handling File Streams
For large files, it’s more efficient to use streams:
const readStream = fs.createReadStream('large-file.txt');
const writeStream = fs.createWriteStream('output.txt');
readStream.pipe(writeStream);
readStream.on('end', () => {
console.log('Read and write completed');
});
6. Networking with Node.js
Node.js excels at networking tasks, making it a popular choice for building web servers and APIs.
Creating HTTP Servers
Here’s a basic HTTP server using the built-in http
module:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, World!');
});
server.listen(3000, () => {
console.log('Server running on port 3000');
});
Building a Simple API
Let’s create a basic API with routing:
const http = require('http');
const server = http.createServer((req, res) => {
if (req.url === '/api/users' && req.method === 'GET') {
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ users: ['John', 'Jane', 'Bob'] }));
} else {
res.writeHead(404, { 'Content-Type': 'text/plain' });
res.end('Not Found');
}
});
server.listen(3000, () => {
console.log('API server running on port 3000');
});
7. Express.js Framework
Express.js is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications.
Setting up a Basic Express Server
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(port, () => {
console.log(`Express server running on port ${port}`);
});
Routing and Middleware
Express makes it easy to define routes and use middleware:
app.use(express.json()); // Middleware to parse JSON bodies
app.get('/api/users', (req, res) => {
res.json({ users: ['John', 'Jane', 'Bob'] });
});
app.post('/api/users', (req, res) => {
const newUser = req.body.name;
// Add user to database
res.status(201).json({ message: `User ${newUser} created` });
});
8. Working with Databases
Most backend applications require interaction with databases. Node.js can work with various databases, both SQL and NoSQL.
Connecting to MongoDB using Mongoose
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/myapp', {
useNewUrlParser: true,
useUnifiedTopology: true
});
const db = mongoose.connection;
db.on('error', console.error.bind(console, 'connection error:'));
db.once('open', function() {
console.log('Connected to MongoDB');
});
Performing CRUD Operations
const userSchema = new mongoose.Schema({
name: String,
email: String
});
const User = mongoose.model('User', userSchema);
// Create
const newUser = new User({ name: 'John Doe', email: 'john@example.com' });
newUser.save((err) => {
if (err) return console.error(err);
console.log('User saved');
});
// Read
User.find({ name: 'John Doe' }, (err, users) => {
if (err) return console.error(err);
console.log(users);
});
// Update
User.updateOne({ name: 'John Doe' }, { email: 'newemail@example.com' }, (err) => {
if (err) return console.error(err);
console.log('User updated');
});
// Delete
User.deleteOne({ name: 'John Doe' }, (err) => {
if (err) return console.error(err);
console.log('User deleted');
});
9. Error Handling
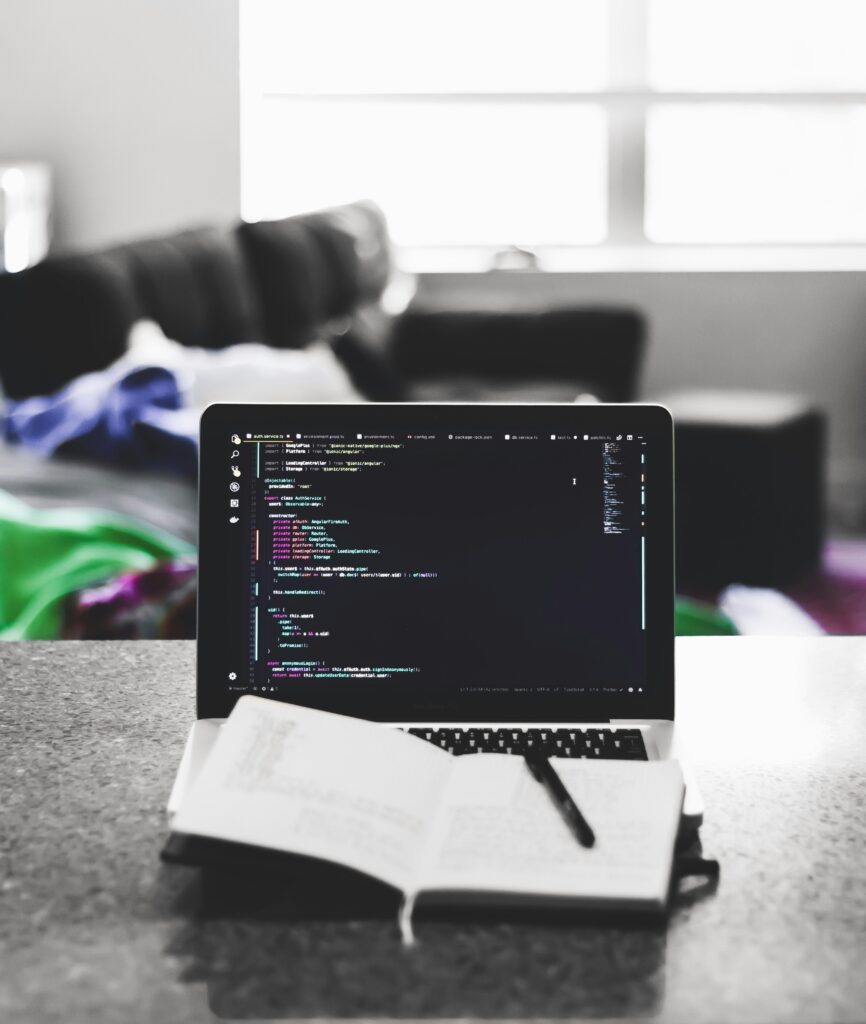
Proper error handling is crucial for building robust Node.js applications. It helps in debugging and provides a better user experience.
Types of Errors
- Synchronous Errors: Can be handled with try/catch blocks.
- Asynchronous Errors: Require callback functions or promise rejections to handle.
Using try/catch
try {
// Code that might throw an error
throw new Error('Something went wrong');
} catch (error) {
console.error('Caught an error:', error.message);
}
Handling Asynchronous Errors
function asyncOperation(callback) {
setTimeout(() => {
try {
// Simulating an error
throw new Error('Async error');
} catch (error) {
callback(error);
}
}, 1000);
}
asyncOperation((error) => {
if (error) {
console.error('Async operation failed:', error.message);
}
});
Using Error Events
const EventEmitter = require('events');
class MyEmitter extends EventEmitter {}
const myEmitter = new MyEmitter();
myEmitter.on('error', (err) => {
console.error('An error occurred:', err.message);
});
myEmitter.emit('error', new Error('Something went wrong'));
10. Testing in Node.js
Testing is an integral part of software development, ensuring that your code works as expected and helps catch bugs early.
Setting up a Testing Environment
First, install the necessary testing libraries:
npm install mocha chai --save-dev
Writing and Running Tests
Create a test file, e.g., test/math.test.js
:
const expect = require('chai').expect;
const math = require('../math');
describe('Math module', () => {
it('should add two numbers correctly', () => {
expect(math.add(2, 3)).to.equal(5);
});
it('should subtract two numbers correctly', () => {
expect(math.subtract(5, 2)).to.equal(3);
});
});
Run the tests using Mocha:
npx mocha
11. Performance Optimization
Optimizing Node.js applications is crucial for handling high traffic and ensuring a smooth user experience.
Caching Strategies
Implement caching to reduce database queries and improve response times:
const NodeCache = require('node-cache');
const myCache = new NodeCache();
app.get('/api/data', (req, res) => {
const cacheKey = 'apiData';
const cachedData = myCache.get(cacheKey);
if (cachedData) {
return res.json(cachedData);
}
// Fetch data from database or external API
const data = fetchDataFromSource();
// Set cache
myCache.set(cacheKey, data, 3600); // Cache for 1 hour
res.json(data);
});
Load Balancing and Clustering
Use the cluster
module to take advantage of multi-core systems:
const cluster = require('cluster');
const http = require('http');
const numCPUs = require('os').cpus().length;
if (cluster.isMaster) {
console.log(`Master ${process.pid} is running`);
// Fork workers.
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
cluster.on('exit', (worker, code, signal) => {
console.log(`worker ${worker.process.pid} died`);
});
} else {
// Workers can share any TCP connection
// In this case it is an HTTP server
http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello World\n');
}).listen(8000);
console.log(`Worker ${process.pid} started`);
}
Profiling and Monitoring
Use tools like node-clinic
or New Relic
to profile and monitor your Node.js applications in production.
FAQ
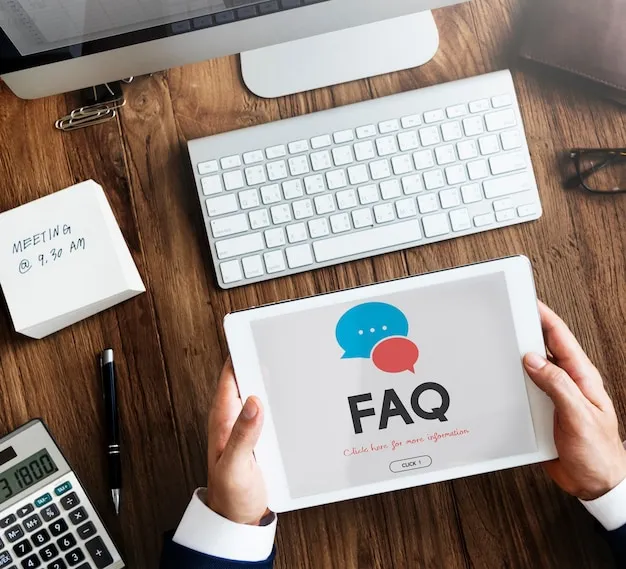
- What is the event loop in Node.js?
The event loop is a mechanism that allows Node.js to perform non-blocking I/O operations despite JavaScript being single-threaded. It handles the execution of callbacks, scheduling of timers, and processing of events. - How does Node.js handle asynchronous operations?
Node.js uses callbacks, promises, and async/await to handle asynchronous operations. These mechanisms allow code execution to continue while waiting for I/O operations to complete. - How do I manage dependencies in Node.js projects?
Use NPM (Node Package Manager) to manage dependencies. Add dependencies to yourpackage.json
file and usenpm install
to install them. - How do I connect Node.js to a database?
Use appropriate database drivers or ORMs. For example, usemongoose
for MongoDB orsequelize
for SQL databases. Establish a connection in your Node.js application using the connection string provided by your database. - What are common techniques for error handling in Node.js?
Common techniques include using try/catch blocks for synchronous code, handling errors in callbacks, using.catch()
with promises, and implementing global error handlers for uncaught exceptions. - How can I optimize the performance of my Node.js application?
Optimize performance by implementing caching, using clustering to take advantage of multiple CPU cores, minimizing database queries, and using asynchronous operations where possible. Regular profiling and monitoring can help identify bottlenecks.
Conclusion
Mastering Node.js is an ongoing journey that requires continuous learning and practice. This guide has covered the essential concepts that every backend developer should know in 2024, including:
- Event-driven architecture
- Asynchronous programming
- The Node.js event loop
- Working with modules and NPM
- File system operations
- Networking and building APIs
- Using Express.js for web applications
- Database integration
- Error handling and testing
- Performance optimization
By understanding these core concepts, you’ll be well-equipped to build efficient, scalable, and robust backend applications with Node.js. Remember that the Node.js ecosystem is constantly evolving, so it’s crucial to stay updated with the latest developments and best practices.
As you continue your Node.js journey, consider exploring more advanced topics such as:
- Microservices architecture
- Serverless computing with Node.js
- Real-time applications using WebSockets
- GraphQL APIs
- Containerization and deployment strategies
“The only way to do great work is to love what you do.” – Steve Jobs
Embrace the challenges and opportunities that come with Node.js development. Contribute to open-source projects, participate in the Node.js community, and never stop learning. Your skills and knowledge will grow with each project you undertake.
Remember, mastering Node.js is not just about understanding the technology itself, but also about applying best practices, writing clean and maintainable code, and solving real-world problems efficiently. As you gain experience, you’ll develop an intuition for when to use certain Node.js features and how to architect your applications for maximum performance and scalability.
Stay curious, keep coding, and enjoy the process of becoming a Node.js expert!
\