Node.js is a powerful platform for building a variety of web and network applications. In the busy world of software development, performance is key—especially when working in a development environment like Ubuntu 16.04. Optimizing Node.js on Ubuntu can lead to dramatically improved application performance and a smoother development experience. This guide will provide you with a set of practical tips to fine-tune your Node.js applications, ensuring they run at their peak performance. Whether you’re dealing with long server response times, high latency, or just looking for some general improvements, these strategies will help you get the most out of your development environment.
Understanding Node.js Performance on Ubuntu 16.04
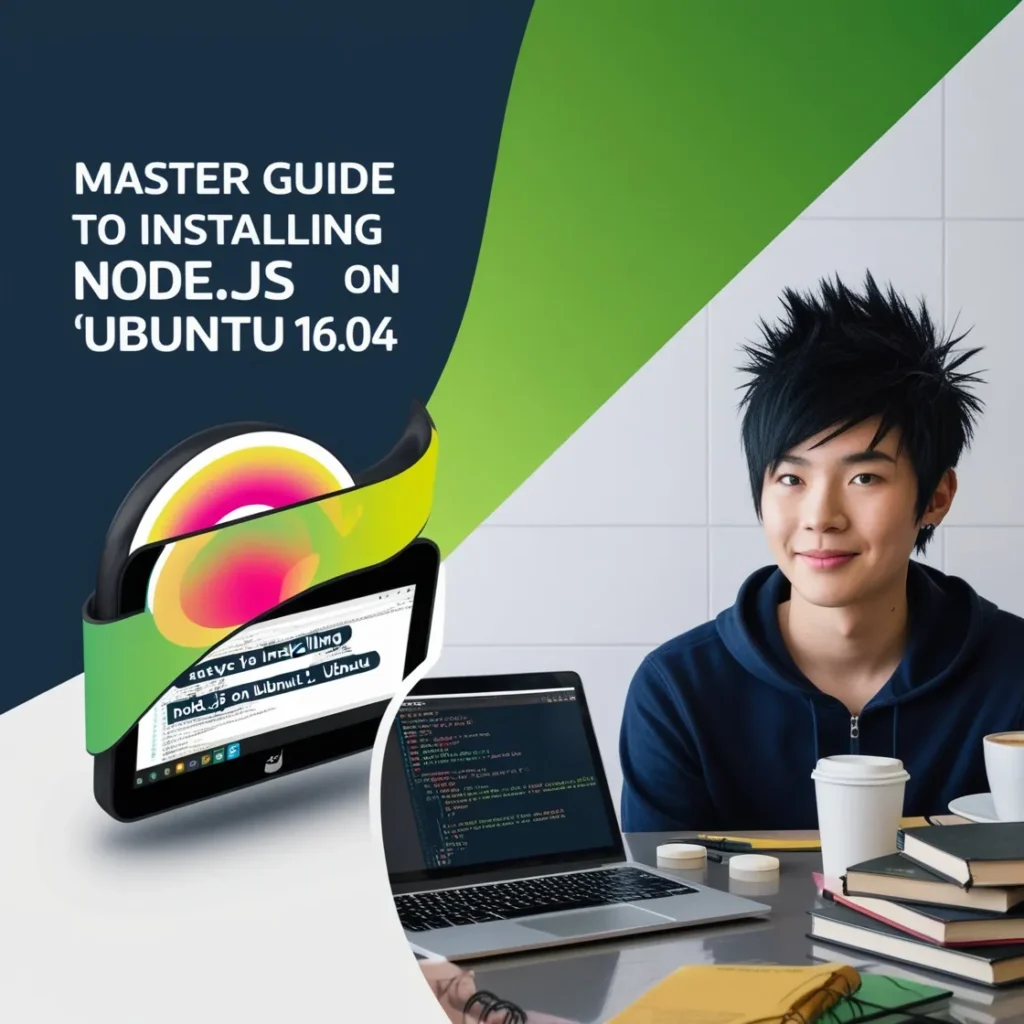
Importance performance optimization
Optimizing Node.js performance is crucial for developers seeking to enhance the efficiency and responsiveness of their applications. Node.js is inherently fast, thanks to its non-blocking I/O model which is well-suited for I/O-bound tasks. However, performance bottlenecks can still occur, especially in CPU-bound tasks or poorly optimized code. By focusing on performance optimization, developers can ensure that their applications are making the best use of system resources, reducing latency, and providing a smoother user experience. Such optimizations not only improve the application’s execution speed but also help in scaling applications effectively to handle more users or requests without requiring proportional increases in hardware resources.
Overview of Ubuntu 16.04
Ubuntu 16.04, also known as Xenial Xerus, is a long-term support (LTS) release from Canonical, supported until April 2021. This stability and extended support make Ubuntu 16.04 a popular choice for developers, particularly in production environments. Ubuntu’s vast repository and compatibility with various software tools, combined with its robustness and security features, make it an ideal platform for developing Node.js applications. Furthermore, the operating system is optimized for performance, which can be leveraged to run Node.js applications efficiently. Understanding the interplay between Node.js and the underlying Linux system on Ubuntu can assist developers in further tweaking their environment for better performance.
Optimizing Node.js Code for Improved Performance
Using asynchronous programming
Node.js’s non-blocking, event-driven architecture is designed to handle asynchronous operations effectively. To maximize Node.js performance, leverage this feature by using asynchronous programming patterns wherever possible. Avoid synchronous functions like \`fs.readFileSync()\` or \`fs.writeFileSync()\` as they halt the execution of your code until the file operation is complete, which can significantly degrade performance. Instead, opt for their asynchronous counterparts, \`fs.readFile()\` and \`fs.writeFile()\`, which allow the Node.js event loop to continue running other code while it handles I/O operations in the background. This approach maximizes throughput and responsiveness in your applications.
Consider using Promises, Async/Await, or libraries like \`async.js\` to manage complex asynchronous workflows. This not only improves performance but also makes your code cleaner and more maintainable.
Implementing code optimizations and best practices
Improving Node.js performance also involves adopting coding best practices and specific optimizations. Here are some strategies to consider:
- Opt for Native Modules: Whenever possible, use native modules over JavaScript modules as they tend to execute faster. This can be particularly useful for tasks that are computationally intensive.
- Reduce Memory Usage: Efficient use of memory is key to improving performance. Avoid global variables, clean up unused objects, and use buffers efficiently to reduce memory footprint.
- Use Tools for Performance Gains: Utilize tools like Node.js’s built-in profiler, \`node –prof\`, to identify slow parts of your code. Employ Google’s V8 profiling tools to dig deeper into CPU usage and function calls.
- Streamline JSON Parsing: If your application processes large volumes of JSON data, consider using stream-based JSON parsers like \`Oboe.js\`, which begin processing data as soon as it starts to arrive, optimizing throughput and memory usage.
- Enhance Garbage Collection: Tune the garbage collector settings using Node flags like \`–max-old-space-size\` and \`–optimizeforsize\` to handle memory more effectively based on your application’s specific needs.
Implementing these coding optimizations and best practices will not only boost the performance of Node.js applications on Ubuntu 16.04 but also improve their scalability and reliability. Regularly reviewing and testing your code for performance issues is key to maintaining optimal efficiency as your application evolves. Remember, the goal is to write clean, efficient, and non-blocking code that fully exploits Node.js’s capabilities on your Ubuntu system.
Resources for Better Node.js Performance
When aiming to enhance Node.js applications, uncovering ways to fully utilize the underlying resources of Ubuntu 16.04 can significantly boost performance. By effectively managing system resources and adjusting configurations, developers can ensure that their Node.js applications run more smoothly and responsively.
Utilizing Node.js cluster module
Node.js executes in a single-thread mode, but you can take advantage of multi-core systems by using the cluster module. This module allows you to create child processes that share server ports and utilize multiple cores. Here’s how it works:
- Forking Workers: The cluster module enables the main process to spawn worker processes, which can all run simultaneously and handle different execution threads. This means your Node.js application can handle more requests at any given time.
- Load Distribution: By distributing incoming connections among multiple instances, the cluster module effectively balances the workload across available CPU cores, preventing any one core from becoming overloaded and potentially slowing down your application.
Implementing the cluster module is relatively straightforward and can drastically improve the throughput of your Node.js applications on Ubuntu 16.04.
Configuring Nginx as a reverse proxy
Using Nginx as a reverse proxy can enhance the performance of Node.js applications by offloading part of the workload to Nginx, which is renowned for its ability to handle a large number of simultaneous connections efficiently. Here are the benefits:
- Caching: Nginx can serve static files directly and cache these responses, reducing the number of requests to the Node.js server.
- Load Balancing: Nginx can distribute incoming traffic to different Node.js instances, optimizing resource utilization and improving responsiveness.
To configure Nginx as a reverse proxy, define a server block in your Nginx configuration file that directs traffic to your Node.js application’s IP address and port. This setup not only optimizes performance but also enhances security by shielding the internal ports and IP addresses of your services.
Managing memory usage efficiently
Effective memory management is crucial for maintaining high performance. Node.js applications, especially those that deal with large amounts of data or high levels of traffic, may experience memory leaks or excessive memory consumption. To manage memory usage:
- Monitoring: Regularly monitor your Node.js applications’ memory usage, which can be done using tools like PM2, Nodetime, or Node Inspector.
- Garbage Collection: Utilize the
\--max-old-space-size\
flag to increase the amount of memory available to Node.js processes if the default limit is exceeded.
Efficient memory management ensures stable performance and prevents crashes or slowdowns due to out-of-memory errors.
Monitoring and Testing Node.js Performance
Monitoring and testing are essential practices to not only assess the performance of your Node.js applications but also to ensure that implemented optimizations effectively enhance the application’s scalability and responsiveness.
To continuously assess the performance of your Node.js applications, integrate performance monitoring tools that can provide insights into various aspects such as response times, system throughput, and error rates. Tools such as New Rellic, Datadog, and Dynatrace offer comprehensive monitoring capabilities that can help identify performance bottlenecks and optimize resource utilization in real-time.
Conducting load testing for Node.js applications
Load testing is critical to understand how your Node.js applications perform under stress. This involves:
- Simulating User Interaction: Use tools like Apache JMeter or Artillery to simulate multiple users accessing your application simultaneously.
- Identifying Bottlenecks: Analyze test results to identify the weakest points in your application that could fail under high traffic.
Regular load testing helps you refine your performance enhancements, ensuring that your Node.js applications on Ubuntu 16.04 can handle real-world use cases effectively. By strategically employing load testing, developers can proactively solve potential issues, leading to smoother deployments and a better user experience.
Conclusion
Optimizing Node.js for better performance on Ubuntu 16.04 is crucial for developers looking to maximize efficiency and enhance the scalability of their applications. Throughout this journey to optimize your Node.js environment, from updating your system and Node.js itself, to fine-tuning runtime configurations and resource management, each step contributes significantly towards a smoother and more robust development experience.
By ensuring that both the Node.js platform and the underlying Ubuntu system are up to date, you safeguard your development environment against performance bottlenecks caused by outdated software. Leveraging tools like Nginx not only improves the handling of concurrent connections but also enhances the overall efficiency of your applications.
Incorporating profiling and monitoring tools into your development process allows you to understand better where optimizations can be most impactful. These tools help identify memory leaks, excessive CPU usage, and other performance issues that could otherwise remain hidden.
Lastly, tuning the garbage collection mechanism and effectively managing asynchronous operations are advanced techniques that require a good grasp of how Node.js works under the hood. These optimizations help in maintaining a balance between performance and resource utilization, ensuring that your Node.js applications run efficiently, even under the stress of high traffic or compute-intensive tasks.
Remember, the key to successful performance optimization lies not only in the implementation of these strategies but also in continuous monitoring and iterative improvement based on real-world usage and feedback. As Ubuntu and Node features continue to evolve, staying informed and ready to adapt your configurations will continue to be an essential part of your development workflow.
By following these guidelines, you are well on your way to achieving a streamlined development process, enabling you to deliver high-quality applications that are both performant and scalable. Whether you’re targeting large-scale enterprise environments or smaller, more dynamic projects, these optimization techniques will empower you to make the most out of your Node.js and Ubuntu capabilities.