Introduction
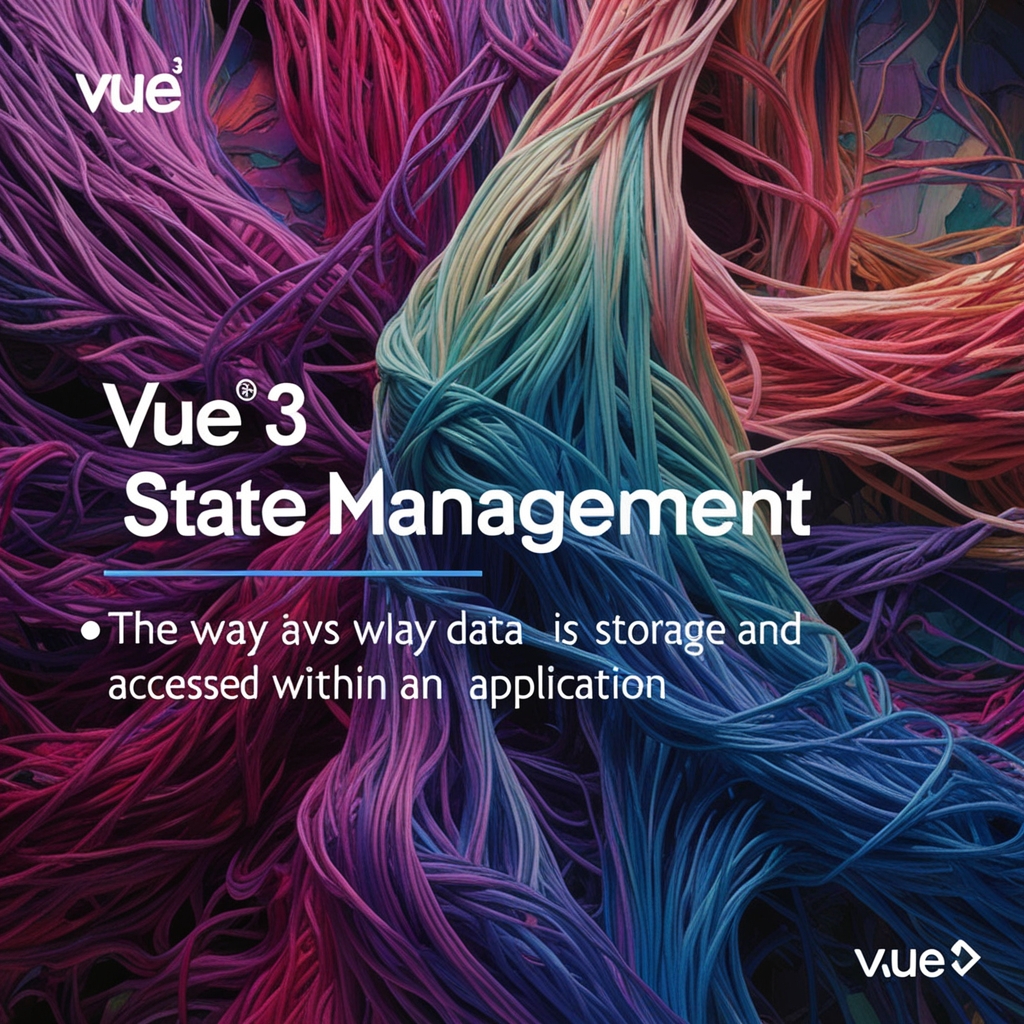
Vue.js has long been a favorite among developers for building interactive user interfaces. With the release of Vue 3, the framework has undergone significant changes, particularly in the realm of state management. This article will explore the evolution of state management from Vue 2 to Vue 3, highlighting new features, best practices, and how these changes impact development workflows.
Understanding State Management
Before diving into the changes in Vue 3, let’s briefly recap what state management is and why it’s crucial in modern web applications.
State management refers to the process of handling an application’s data and ensuring that changes to this data are reflected consistently across the UI. In complex applications, managing state can become challenging, especially when multiple components need to access and modify the same data.
Effective state management solutions aim to:
- Centralize data storage
- Provide a predictable way to modify state
- Ensure data consistency across components
- Improve maintainability and scalability of the application
With this foundation, let’s explore how Vue 3 has revolutionized state management.
Vue 3 vs Vue 2: Key Differences in State Management
Vue 3 introduces several changes that significantly impact how we approach state management. Here are the key differences:
- Composition API: This is perhaps the most significant change in Vue. It provides a more flexible and powerful way to organize component logic, including state management.
- Improved Reactivity System: Vue 3’s reactivity system is now based on ES6 Proxies, making it more efficient and allowing for better tracking of nested objects and arrays.
- Tree-shaking Support: Vue 3’s core has been rewritten to support tree-shaking, allowing unused code to be eliminated from the final bundle, resulting in smaller application sizes.
- TypeScript Integration: Vue 3 is written in TypeScript, providing better type inference and improved IDE support for state management.
- Multiple Root Elements: Vue 3 components can now have multiple root elements, which can simplify state management in certain scenarios.
Let’s delve deeper into these changes and their implications for state management.
Composition API: A Game Changer for State Management
The Composition API is undoubtedly the most significant addition to Vue 3, and it has a profound impact on state management. Unlike the Options API used in Vue 2, the Composition API allows developers to organize code by logical concerns rather than option types.
Here’s a simple example comparing state management in Vue 2 and Vue 3:
Vue 2 (Options API):
export default {
data() {
return {
count: 0
}
},
methods: {
increment() {
this.count++
}
}
}
Vue 3 (Composition API):
import { ref } from 'vue'
export default {
setup() {
const count = ref(0)
function increment() {
count.value++
}
return {
count,
increment
}
}
}
The Composition API offers several advantages for state management:
- Better Code Organization: Related logic can be grouped together, making it easier to understand and maintain complex components.
- Improved Reusability: State management logic can be extracted into composable functions, promoting code reuse across components.
- TypeScript Support: The Composition API works seamlessly with TypeScript, providing better type inference for state and mutations.
- Reactivity on Demand: With the Composition API, you can make specific properties reactive only when needed, potentially improving performance.
Reactive State Management in Vue 3
Vue 3 introduces new reactivity APIs that simplify state management within components and across the application. The key players in this new reactivity system are:
ref
: For creating reactive references to primitive valuesreactive
: For creating reactive objectscomputed
: For creating derived reactive statewatch
andwatchEffect
: For side effects based on state changes
Let’s look at an example that demonstrates these APIs:
import { ref, reactive, computed, watch } from 'vue'
export default {
setup() {
// Reactive primitive
const count = ref(0)
// Reactive object
const user = reactive({
name: 'John Doe',
age: 30
})
// Computed property
const doubleCount = computed(() => count.value * 2)
// Watcher
watch(count, (newValue, oldValue) => {
console.log(`Count changed from ${oldValue} to ${newValue}`)
})
function increment() {
count.value++
}
function updateUser(name, age) {
user.name = name
user.age = age
}
return {
count,
user,
doubleCount,
increment,
updateUser
}
}
}
This example showcases how Vue 3’s reactivity system can be used to manage state within a component. For larger applications, you might want to consider using a state management library like Vuex 4 or Pinia.
Vuex 4: Evolution of the State Management Library
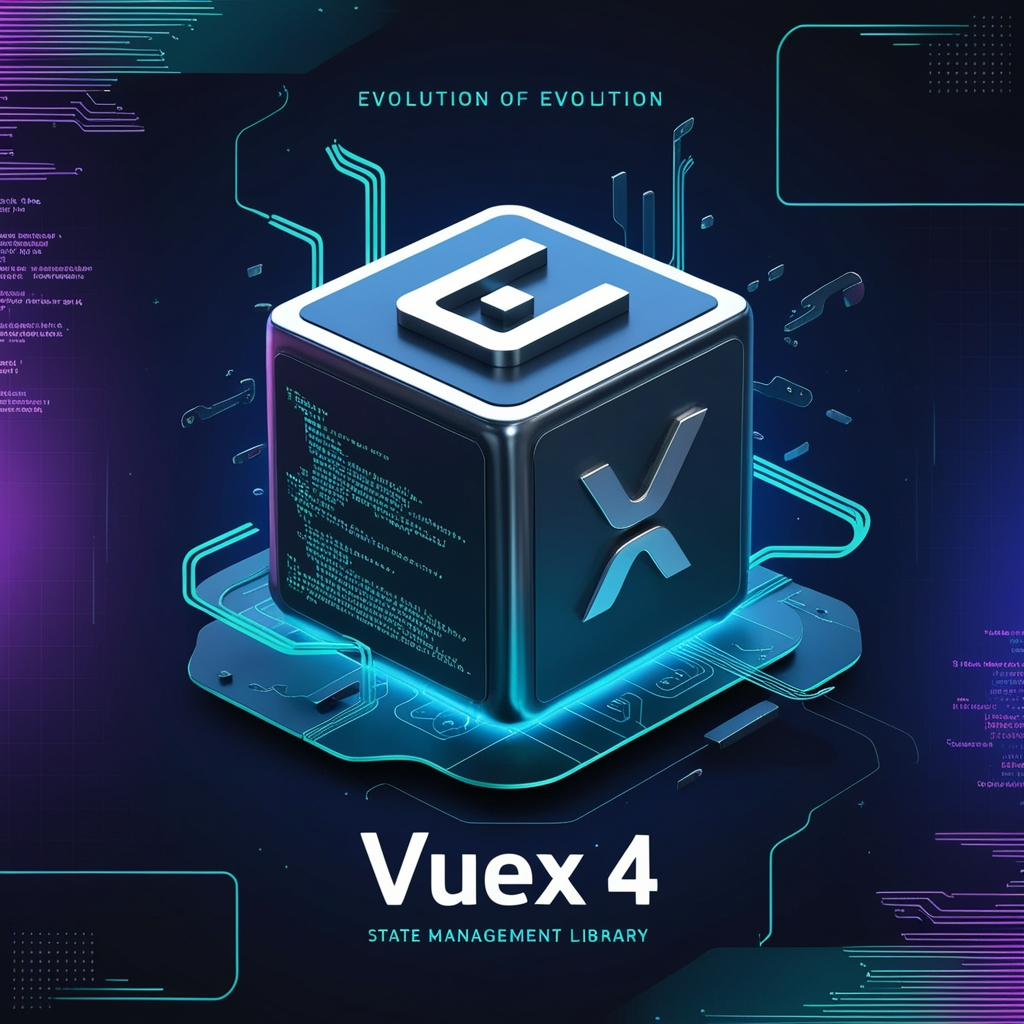
Vuex, the official state management library for Vue, has also evolved with the release of Vue 3. Vuex 4 is designed to work seamlessly with Vue 3 while maintaining most of the concepts from Vuex 3.
Key changes in Vuex 4 include:
- Installation as a Vue 3 Plugin: Vuex 4 is now installed as a plugin using the new
createApp
function. - Composition API Support: Vuex 4 introduces new composition API functions like
useStore
for easier integration with the Composition API. - Improved TypeScript Support: Vuex 4 offers better type inference and autocompletion in TypeScript projects.
Here’s an example of how to use Vuex 4 in a Vue 3 application:
// store.js
import { createStore } from 'vuex'
export default createStore({
state: {
count: 0
},
mutations: {
increment(state) {
state.count++
}
},
actions: {
incrementAsync({ commit }) {
setTimeout(() => {
commit('increment')
}, 1000)
}
},
getters: {
doubleCount: state => state.count * 2
}
})
// main.js
import { createApp } from 'vue'
import App from './App.vue'
import store from './store'
createApp(App).use(store).mount('#app')
// Component.vue
import { useStore } from 'vuex'
import { computed } from 'vue'
export default {
setup() {
const store = useStore()
const count = computed(() => store.state.count)
const doubleCount = computed(() => store.getters.doubleCount)
function increment() {
store.commit('increment')
}
function incrementAsync() {
store.dispatch('incrementAsync')
}
return {
count,
doubleCount,
increment,
incrementAsync
}
}
}
While Vuex 4 maintains compatibility with Vue 3, some developers have found its boilerplate-heavy approach less appealing in the context of the Composition API. This has led to the rise of alternative state management solutions, with Pinia being a notable example.
Pinia: The New Kid on the Block
Pinia is a state management library that has gained significant traction in the Vue 3 ecosystem. Created by Eduardo San Martin Morote, a member of the Vue core team, Pinia is designed to be the successor to Vuex.
Key features of Pinia include:
- Intuitive API: Pinia offers a more straightforward and less verbose API compared to Vuex.
- Excellent TypeScript Support: Pinia is written in TypeScript and provides great type inference out of the box.
- Modular by Design: Pinia encourages a modular approach to state management, making it easier to split and organize stores.
- No Mutations: Unlike Vuex, Pinia doesn’t use mutations, simplifying the state management process.
- Devtools Support: Pinia integrates well with Vue Devtools, providing a great debugging experience.
Here’s an example of how to use Pinia in a Vue 3 application:
// store.js
import { defineStore } from 'pinia'
export const useCounterStore = defineStore('counter', {
state: () => ({
count: 0
}),
getters: {
doubleCount: (state) => state.count * 2
},
actions: {
increment() {
this.count++
},
incrementAsync() {
setTimeout(() => {
this.count++
}, 1000)
}
}
})
// main.js
import { createApp } from 'vue'
import { createPinia } from 'pinia'
import App from './App.vue'
const pinia = createPinia()
createApp(App).use(pinia).mount('#app')
// Component.vue
import { useCounterStore } from './store'
import { storeToRefs } from 'pinia'
export default {
setup() {
const store = useCounterStore()
// Destructure while keeping reactivity
const { count, doubleCount } = storeToRefs(store)
return {
// State and getters
count,
doubleCount,
// Actions
increment: store.increment,
incrementAsync: store.incrementAsync
}
}
}
Pinia’s simplicity and excellent integration with Vue 3’s Composition API have made it a popular choice for many developers.
Best Practices for State Management in Vue 3
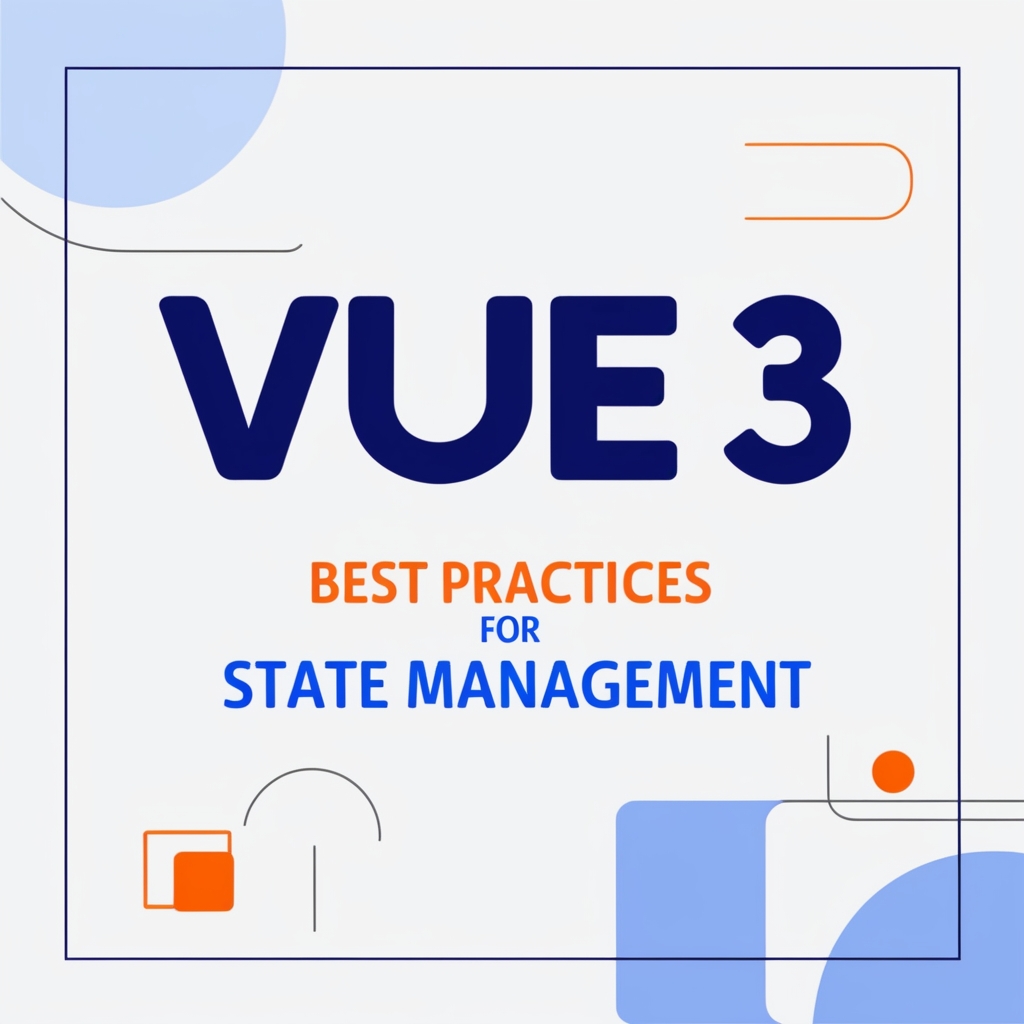
With the introduction of the Composition API and new state management options, some best practices have emerged for managing state in Vue 3 applications:
- Use Composition API for Local State: For component-specific state, leverage the Composition API with
ref
andreactive
. - Extract Reusable Logic: Use composables to extract and reuse state management logic across components.
- Consider Pinia for Global State: For application-wide state, consider using Pinia due to its simplicity and excellent TypeScript support.
- Leverage Vue 3’s Reactivity System: Make use of
computed
,watch
, andwatchEffect
for derived state and side effects. - Keep Components Lightweight: Move complex state management logic out of components and into dedicated stores or composables.
- Use TypeScript: Leverage TypeScript for better type checking and improved developer experience.
- Modularize Stores: Break down large stores into smaller, more focused modules for better maintainability.
- Optimize for Performance: Use
shallowRef
andshallowReactive
for large objects when deep reactivity is not needed.
Real-World Examples
Let’s look at a more complex real-world example that demonstrates state management in a Vue 3 application using Pinia. We’ll create a simple task management application.
First, let’s define our Pinia store:
// taskStore.js
import { defineStore } from 'pinia'
export const useTaskStore = defineStore('tasks', {
state: () => ({
tasks: [],
filter: 'all'
}),
getters: {
filteredTasks() {
if (this.filter === 'all') return this.tasks
const isCompleted = this.filter === 'completed'
return this.tasks.filter(task => task.completed === isCompleted)
},
totalCount: state => state.tasks.length,
completedCount: state => state.tasks.filter(task => task.completed).length
},
actions: {
addTask(title) {
this.tasks.push({ id: Date.now(), title, completed: false })
},
removeTask(id) {
this.tasks = this.tasks.filter(task => task.id !== id)
},
toggleTask(id) {
const task = this.tasks.find(task => task.id === id)
if (task) task.completed = !task.completed
},
setFilter(filter) {
this.filter = filter
}
}
})
Vue 3 with Pinia:
javascriptCopy// store.js
import { defineStore } from 'pinia'
export const useCounterStore = defineStore('counter', {
state: () => ({
count: 0
}),
actions: {
increment() {
this.count++
}
}
})
// Component.vue
import { useCounterStore } from './store'
import { storeToRefs } from 'pinia'
export default {
setup() {
const store = useCounterStore()
const { count } = storeToRefs(store)
return {
count,
increment: store.increment
}
}
}
Conclusion
State management in Vue 3 has evolved significantly, offering developers more flexibility, better performance, and improved tooling. The introduction of the Composition API has changed how we approach state management, allowing for more modular and reusable code.
While Vuex remains a viable option for state management in Vue 3, new libraries like Pinia have emerged to offer simpler and more intuitive APIs that align well with the Composition API’s philosophy.
As you work with Vue 3, consider the following key takeaways:
- Leverage the Composition API for local state management and reusable logic.
- Use Pinia or Vuex 4 for global state management, depending on your project’s needs.
- Take advantage of Vue 3’s improved reactivity system and performance optimizations.
- Embrace TypeScript for better type checking and developer experience.
- Focus on modular, composable state management solutions that align with Vue 3’s design principles.
By understanding these changes and adopting best practices, you can create more maintainable, performant, and scalable Vue 3 applications with efficient state management.
FAQs
- Q: Do I have to use the Composition API for state management in Vue 3? A: No, you don’t have to use the Composition API. Vue 3 still supports the Options API, and you can continue to use it for state management. However, the Composition API offers more flexibility and better code organization, especially for complex components and applications.
- Q: Is Vuex still relevant in Vue 3? A: Yes, Vuex is still relevant and has been updated to work with Vue 3. Vuex 4 is fully compatible with Vue 3 and remains the official state management solution. However, alternatives like Pinia are gaining popularity due to their simpler API and better integration with the Composition API.
- Q: What are the main differences between Vuex and Pinia? A: The main differences are:
- Pinia has a simpler API with no mutations.
- Pinia offers better TypeScript support out of the box.
- Pinia is designed to work seamlessly with the Composition API.
- Pinia allows for easier code splitting and better tree-shaking.
- Q: Can I use Vue 3’s reactivity system for state management without a library? A: Yes, for simpler applications, you can use Vue 3’s reactivity APIs like
reactive()
,ref()
, andcomputed()
to manage state without a dedicated library. You can create a simple store using these APIs and share it across components. - Q: How does state management performance in Vue 3 compare to Vue 2? A: State management in Vue 3 is generally more performant due to the new proxy-based reactivity system, improved virtual DOM, and better tree-shaking support. These improvements allow for more efficient tracking of state changes and faster rendering.