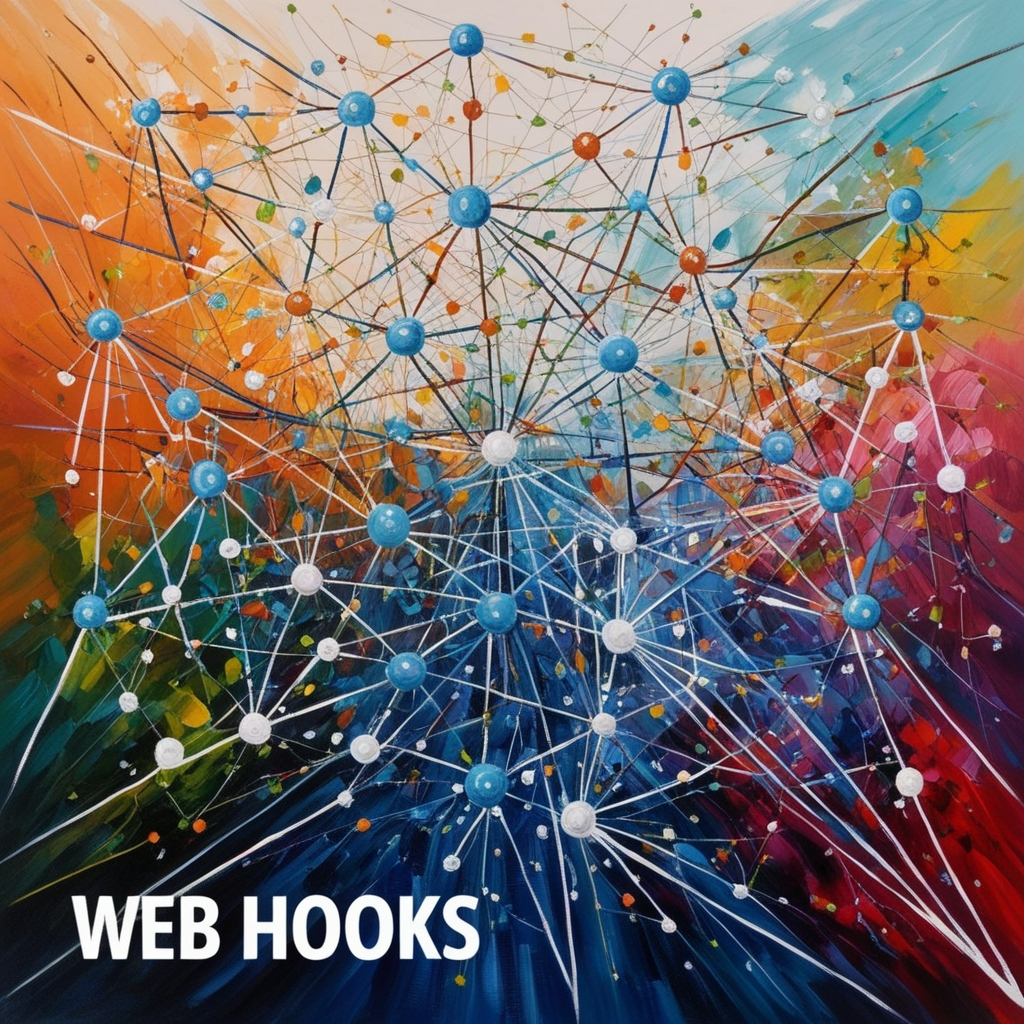
In the ever-evolving landscape of web development, staying ahead of the curve is crucial for developers and businesses alike. One technology that has gained significant traction in recent years is web hooks. These powerful tools are transforming the way applications communicate and interact, paving the way for more efficient, real-time, and scalable web solutions. In this comprehensive guide, we’ll dive deep into the world of web hooks, exploring their functionality, benefits, and practical applications in modern web development.
Table of Contents
Introduction to Web Hooks
Web hooks, also known as HTTP callbacks or reverse APIs, are a mechanism for real-time, event-driven communication between different systems or applications. Unlike traditional APIs where a client constantly polls a server for updates, web hooks allow a server to push data to a client as soon as an event occurs. This paradigm shift in communication has revolutionized the way web applications interact, enabling more efficient and responsive systems.
Key Concepts:
- Event-driven: Web hook are triggered by specific events rather than periodic polling.
- Real-time: Data is pushed immediately when an event occurs, reducing latency.
- Asynchronous: Web hook operate asynchronously, allowing systems to process data independently.
How Web Hooks Work
At their core, web hooks function through a simple yet powerful process:
- Registration: A client application registers a URL (the web hook endpoint) with a service provider.
- Event Trigger: When a specific event occurs on the service provider’s side, it prepares the relevant data.
- HTTP POST Request: The service provider sends an HTTP POST request to the registered URL, containing the event data.
- Processing: The client application receives the data and processes it according to its business logic.
Example:
Let’s consider a scenario where an e-commerce platform wants to be notified when a new order is placed on a third-party marketplace:
# Server-side code (Marketplace)
def process_new_order(order_data):
# Process the order
# ...
# Trigger web hook
webhook_url = "https://ecommerce-platform.com/webhook/new-order"
payload = {
"order_id": order_data["id"],
"total_amount": order_data["total"],
"customer_email": order_data["customer"]["email"]
}
requests.post(webhook_url, json=payload)
# Client-side code (E-commerce Platform)
@app.route("/webhook/new-order", methods=["POST"])
def handle_new_order_webhook():
order_data = request.json
# Process the new order
update_inventory(order_data["order_id"])
send_confirmation_email(order_data["customer_email"])
return "OK", 200
In this example, whenever a new order is placed on the marketplace, it triggers the web hook, sending the order details to the e-commerce platform. The platform then processes the order, updates inventory, and sends a confirmation emailโall in real-time.
Benefits of Using Web Hooks
The adoption of web hook in web development brings numerous advantages:
- Real-time Updates: Web hooks enable instant data transmission, ensuring that applications always have the most up-to-date information.
- Reduced Server Load: By eliminating the need for constant polling, web hook significantly reduce the load on both the client and server systems.
- Improved Efficiency: Web hook allow for more efficient use of resources, as data is only transmitted when necessary.
- Scalability: The event-driven nature of web hooks makes it easier to scale applications and handle high volumes of data.
- Flexibility: Web hooks can be easily integrated into existing systems and adapted to various use cases.
- Better User Experience: Real-time updates lead to a more responsive and interactive user experience.
- Cost-Effective: By reducing unnecessary API calls, web hooks can help lower operational costs associated with data transfer and processing.
Implementing Web Hooks

Implementing web hook in your web development projects involves several key steps:
1. Designing the Web Hook Payload
Carefully consider the data structure you’ll send in your web hook payload. It should include all necessary information while remaining concise. For example:
{
"event_type": "new_comment",
"timestamp": "2024-09-20T14:30:00Z",
"data": {
"comment_id": "12345",
"post_id": "67890",
"author": "johndoe",
"content": "Great article!",
"created_at": "2024-09-20T14:29:55Z"
}
}
2. Setting Up the Web Hook Endpoint
Create an endpoint on your server to receive web hook requests. This typically involves setting up a route in your web application framework. For instance, using Express.js:
const express = require('express');
const app = express();
app.post('/webhook/new-comment', (req, res) => {
const payload = req.body;
// Process the new comment
console.log(`New comment received: ${payload.data.content}`);
// Perform necessary actions (e.g., update database, send notifications)
res.status(200).send('Webhook received successfully');
});
app.listen(3000, () => console.log('Server is running on port 3000'));
3. Implementing Retry Logic
To handle potential failures or downtime, implement a retry mechanism for your web hook:
import requests
from time import sleep
def send_webhook(url, payload, max_retries=3, backoff_factor=2):
for attempt in range(max_retries):
try:
response = requests.post(url, json=payload)
response.raise_for_status()
return response
except requests.exceptions.RequestException as e:
if attempt == max_retries - 1:
raise
sleep_time = backoff_factor ** attempt
print(f"Attempt {attempt + 1} failed. Retrying in {sleep_time} seconds...")
sleep(sleep_time)
4. Handling Web Hook Responses
Ensure your application properly handles responses from the web hook endpoint.
function handleWebhookResponse(response) {
if (response.status === 200) {
console.log('Webhook delivered successfully');
} else if (response.status === 410) {
console.log('Webhook endpoint is no longer valid. Removing from our system.');
removeWebhookEndpoint(response.url);
} else {
console.error(`Webhook delivery failed with status ${response.status}`);
// Implement retry logic or error handling as needed
}
}
Best Practices for Web Hook Design
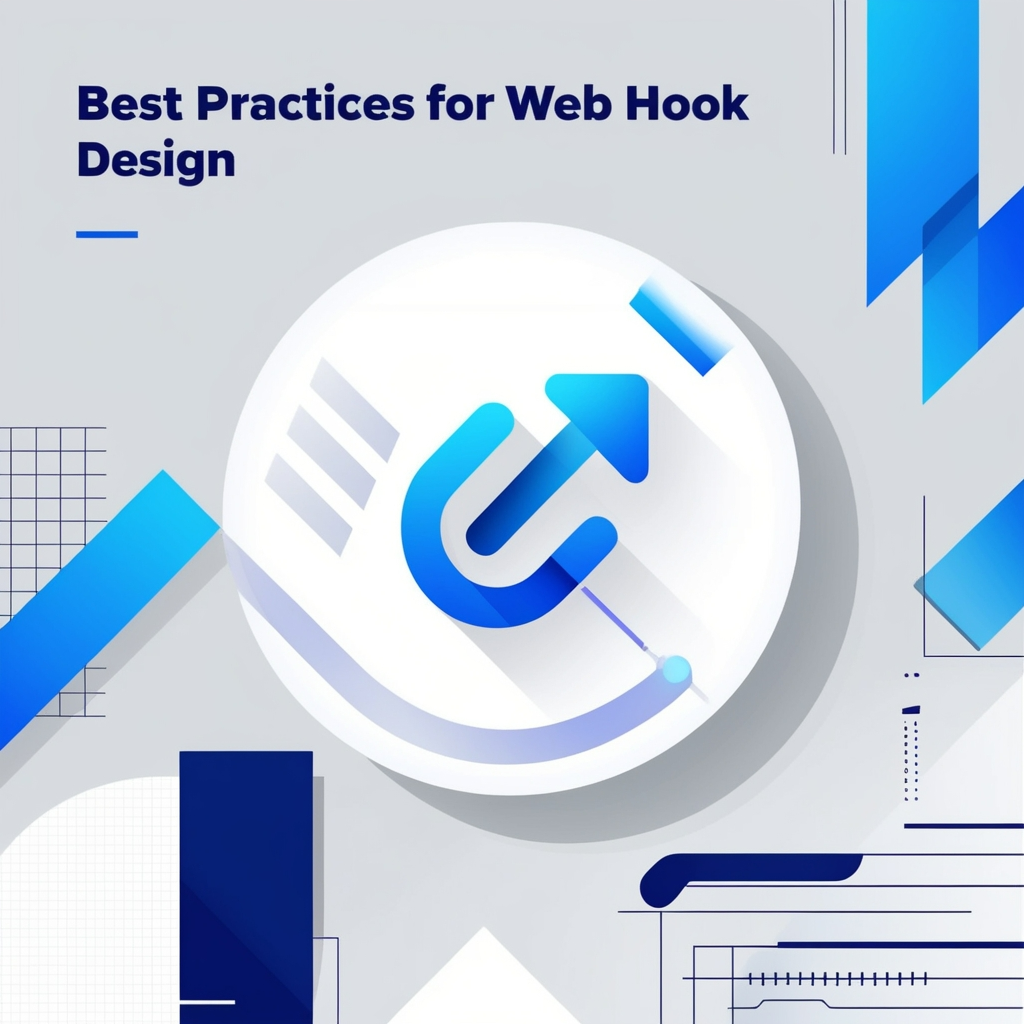
To ensure your web hook are robust, scalable, and developer-friendly, follow these best practices:
- Use HTTPS: Always use HTTPS to encrypt web hook payloads and protect sensitive data.
- Implement Authentication: Use tokens or signatures to verify the authenticity of web hook requests.
- Include Event Types: Clearly specify the type of event in your payload to allow for easy filtering and processing.
- Provide Comprehensive Documentation: Offer detailed documentation on your web hook implementation, including payload structure and expected responses.
- Implement Rate Limiting: To prevent overwhelming the receiving system, implement rate limiting on your web hook requests.
- Use Idempotency Keys: Include unique identifiers in your payloads to prevent duplicate processing in case of retries.
- Offer Configurability: Allow users to choose which events they want to receive web hooks for and at what frequency.
- Implement Versioning: Version your web hook API to ensure backward compatibility as you evolve your system.
Security Considerations
Security is paramount when implementing web hook. Consider the following measures:
- Payload Encryption: Encrypt sensitive data within the payload to protect it during transmission.
- IP Whitelisting: Restrict web hook requests to come only from trusted IP addresses.
- Signature Verification: Implement a signature mechanism to verify the authenticity of incoming web hook requests.
Example of signature verification in Python:
import hmac
import hashlib
def verify_signature(payload, signature, secret):
computed_signature = hmac.new(
secret.encode('utf-8'),
payload.encode('utf-8'),
hashlib.sha256
).hexdigest()
return hmac.compare_digest(computed_signature, signature)
@app.route('/webhook', methods=['POST'])
def handle_webhook():
payload = request.data
signature = request.headers.get('X-Hub-Signature-256')
if not verify_signature(payload, signature, 'your_secret_key'):
abort(403)
# Process the webhook
# ...
Real-World Applications
Web hooks find applications across various domains in web development:
- E-commerce: Real-time inventory updates, order notifications, and shipping status changes.
- Social Media: Instant notifications for new posts, likes, comments, or mentions.
- Payment Systems: Immediate transaction notifications and fraud detection alerts.
- IoT (Internet of Things): real-time data updates from connected devices.
- Continuous Integration/Continuous Deployment (CI/CD): Automated build and deployment notifications.
- Customer Relationship Management (CRM): Instant updates on lead activities or customer interactions.
- Content Management Systems (CMS): Notifications for new content publications or updates.
Case Study: GitHub Web Hooks
GitHub’s web hook system is an excellent example of real-world application. It allows repository owners to set up web hooks that trigger on various events, such as pushes, pull requests, or issue comments. This enables developers to build integrations that respond in real-time to repository activities.
Example of setting up a GitHub web hook using the GitHub API:
import requests
def create_github_webhook(repo_owner, repo_name, webhook_url, events, github_token):
api_url = f"https://api.github.com/repos/{repo_owner}/{repo_name}/hooks"
headers = {
"Authorization": f"token {github_token}",
"Accept": "application/vnd.github.v3+json"
}
payload = {
"name": "web",
"active": True,
"events": events,
"config": {
"url": webhook_url,
"content_type": "json"
}
}
response = requests.post(api_url, json=payload, headers=headers)
return response.json()
# Usage
create_github_webhook(
"octocat",
"Hello-World",
"https://example.com/webhook",
["push", "pull_request"],
"your_github_token"
)
Challenges and Limitations
While web hooks offer numerous benefits, they also come with challenges.
- Reliability: Ensuring all web hook events are delivered and processed can be complex, especially in distributed systems.
- Ordering: Maintaining the correct order of events can be challenging, particularly with high-frequency web hooks.
- Scalability: As the number of webhooks increases, managing and processing them efficiently becomes more demanding.
- Debugging: Troubleshooting issues with web hooks can be difficult due to their asynchronous nature.
- Security: Protecting webhook endpoints from unauthorized access and ensuring data integrity requires careful implementation.
To address these challenges, consider implementing the following solutions:
- Use message queues to handle high volumes of webhook events.
- Implement robust error handling and logging mechanisms.
- Use unique identifiers and timestamps to manage event ordering.
- Regularly audit and update security measures for web hook endpoints.
Future of Web Hooks
As web development continues to evolve, web hooks are poised to play an even more significant role.
- Standardization: Efforts are underway to standardize web hook implementations across different platforms and services.
- Serverless Integration: Web hooks are becoming increasingly integrated with serverless architectures, enabling more scalable and cost-effective solutions.
- AI and Machine Learning: Web hooks will likely be used to trigger AI-powered processes and machine learning models in real-time.
- Edge Computing: As edge computing gains traction, web hooks may be utilized to trigger computations closer to the data source, reducing latency.
- Enhanced Security: Future web hook implementations may incorporate advanced security features such as blockchain-based verification or zero-knowledge proofs.
FAQ
Q1: What is the difference between web hooks and APIs?
A1: While both web hooks and APIs facilitate communication between systems, they operate differently:
- APIs (Application Programming Interfaces) typically follow a request-response model where the client initiates communication by sending a request to the server.
- Web hooks use a publish-subscribe model where the server pushes data to the client when specific events occur, without the client having to request it.
Q2: Are web hooks secure?
A2: Web hooks can be secure when implemented correctly. Key security measures include:
- Using HTTPS for all communications
- Implementing authentication mechanisms (e.g., tokens or signatures)
- Verifying the source of incoming web hook requests
- Encrypting sensitive data in the payload
Q3: How do I handle failed web hook deliveries?
A3: To handle failed deliveries:
- Implement a retry mechanism with exponential backoff
- Log failed attempts for later analysis
- Set up alerts for persistent failures
- Consider implementing a “dead letter queue” for undeliverable web hooks
Q4: Can web hooks be used for real-time applications?
A4: Yes, web hooks are excellent for real-time applications. They allow for immediate data transmission as soon as an event occurs, making them ideal for scenarios requiring instant updates or notifications.
Q5: How do I test web hooks during development?
A5: Testing web hooks during development can be done using various methods:
- Use tools like ngrok or localtunnel to expose your local development server to the internet
- Create a mock server to simulate web hook requests
- Use web hook testing platforms like Webhook.site or RequestBin